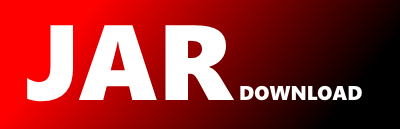
org.semanticwb.SWBPortal Maven / Gradle / Ivy
Show all versions of SWBPortal Show documentation
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org.mx
*/
package org.semanticwb;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URLEncoder;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Properties;
import java.util.Random;
import java.util.StringTokenizer;
import java.util.concurrent.ConcurrentHashMap;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import javax.servlet.Filter;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.semanticwb.SWBUtils.IO;
import org.semanticwb.css.parser.Attribute;
import org.semanticwb.css.parser.CSSParser;
import org.semanticwb.css.parser.Selector;
import org.semanticwb.model.FriendlyURL;
import org.semanticwb.model.Language;
import org.semanticwb.model.ResourceType;
import org.semanticwb.model.Rule;
import org.semanticwb.model.SWBContext;
import org.semanticwb.model.User;
import org.semanticwb.model.UserGroup;
import org.semanticwb.model.UserRepository;
import org.semanticwb.model.WebSite;
import org.semanticwb.platform.SWBMessageCenter;
import org.semanticwb.platform.SemanticModel;
import org.semanticwb.portal.PFlowManager;
import org.semanticwb.portal.SWBAdminFilterMgr;
import org.semanticwb.portal.SWBMessageProcesor;
import org.semanticwb.portal.SWBMonitor;
import org.semanticwb.portal.SWBResourceMgr;
import org.semanticwb.portal.SWBServiceMgr;
import org.semanticwb.portal.SWBTemplateMgr;
import org.semanticwb.portal.SWBUserMgr;
import org.semanticwb.portal.access.SWBAccessIncrement;
import org.semanticwb.portal.access.SWBAccessLog;
import org.semanticwb.portal.api.SWBResource;
import org.semanticwb.portal.db.SWBDBAdmLog;
import org.semanticwb.portal.indexer.SWBIndexMgr;
import org.semanticwb.portal.resources.ImageGallery;
import org.semanticwb.portal.services.SWBCloud;
import org.semanticwb.portal.util.CaptchaUtil;
import org.semanticwb.portal.util.ContentStyles;
import org.semanticwb.portal.util.SWBSoundEngine;
import org.semanticwb.repository.Workspace;
import org.semanticwb.util.JarFile;
import org.semanticwb.util.db.GenericDB;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.arthurdo.parser.HtmlStreamTokenizer;
import com.arthurdo.parser.HtmlTag;
// TODO: Auto-generated Javadoc
/**
* Executes initialization operations for the environment necesary to provide
* Portal services, so users, templates, publication flows, resources, services,
* indexes, and access and data bases can be managed.
*
* Realiza operaciones de inicialización del ambiente necesario para
* proporcionar servicios de Portal, a fin de poder administrar usuarios,
* plantillas, flujos de publicación, recursos, servicios, indizado, así
* como de bitácoras de acceso y de base de datos.
*
* @author Javier Solís
* @author Jorge Jiménez
* @version 1.0
*/
public class SWBPortal {
/**
* Holds the names and values for the variables declared in
* {@literal web.properties} file.
*
* Almacena los nombres y valores de las variables declaradas en el archivo
* {@literal web.properties}.
*/
private static Properties props = null;
/**
* Holds the names and values for the variables declared in
* {@literal security.properties} file.
*
* Almacena los nombres y valores de las variables declaradas en el archivo
* {@literal security.properties}.
*/
private static Properties secProps = null;
/**
* Stores the context path's value configured for this portal instance.
*
* Almacena el valor de la ruta de contexto configurada para esta instancia
* de portal.
*/
private String contextPath = "/";
/**
* Stores the work directory's web path value
*
* Almacena el valor de la ruta web del directorio de trabajo
.
*/
private static String webWorkPath = "";
/**
* The WORKPAT h_ relative.
*/
public static String WORKPATH_RELATIVE = "relative";
/**
* The WORKPAT h_ absolute.
*/
public static String WORKPATH_ABSOLUTE = "absolute";
/**
* The WORKPAT h_ remote.
*/
public static String WORKPATH_REMOTE = "remote";
/**
* Stores the work directory's path.
*
* Almacena la ruta del directorio de trabajo.
*/
private static String workPath = "";
/**
* Store the work path type Almcaena el tipo de ruta de trabajo.
*/
private static String workPathType = WORKPATH_RELATIVE;
/**
* Holds a reference to the {@code ServletContext} used by this web
* application.
*
* Mantiene una referencia hacia el objeto {@code ServletContext} utilizado
* por esta aplicación.
*/
private static ServletContext servletContext = null;
/**
* Holds a reference to the {@code Filter} used by this web application.
*
* Mantiene una referencia hacia el objeto {@code Filter} utilizado por esta
* aplicación.
*/
private static Filter virtualHostFilter = null;
/**
* Indicates whether this instance of portal has an existing database
* related to it.
*
* Indica si esta instancia de portal tiene relacionada una base de datos
* existente.
*/
private static boolean haveDB = false;
/**
* Indicates whether the database related to this portal instance has the
* necessary tables to work with.
*
* Indica si la base de datos relacionada a esta instancia de portal tiene
* las tablas necesarias para trabajar.
*/
private static boolean haveDBTables = false;
/**
* Indicates whether or not to use a database.
*
* Indica si usar o no una base de datos.
*/
private static boolean useDB = true;
/**
* Indicates if this portal instance is client of another one.
*
* Indica si esta instancia de portal es o no cliente de otra.
*/
private static boolean client = false;
/**
* Indicates if this portal instance is stand alone.
*
* Indica si esta instancia de portal esta sola (no en balanceo).
*/
private static boolean standalone = true;
/**
* Creates the messages to the log files.
*
* Crea los mensajes hacia los archivos de bitácora.
*/
private static Logger log = SWBUtils.getLogger(SWBPortal.class);
/**
* Holds the only one instance of this object in the application.
*
* Mantiene la única instancia de este objeto en la
* aplicación
*/
private static SWBPortal instance = null;
/**
* Contains all the anchors found in the HTML code analized.
*
* Contiene todas las anclas encontradas en el código HTML
* analizado.
*/
private static HashMap hAnchors = null;
/**
* Contains the names and content of all files within SWBAdmin.jar and
* dojo.jar files.
*
* Contiene los nombres y el contenido de todos los archivos dentro de los
* archivos SWBAdmin.jar y dojo.jar.
*/
private static ConcurrentHashMap admFiles = null;
/**
* Is the manager for the user objects in this portal.
*
* Es el administrador de los objetos de usuarios en este portal.
*/
private static SWBUserMgr usrMgr;
/**
* Is the manager for the publication flows in this portal.
*
* Es el administrador de los flujos de publicación en este
* portal.
*/
private static PFlowManager pflowMgr;
/**
* Is the monitor in this portal.
*
* Es el monitor en este portal.
*/
private static SWBMonitor monitor = null;
/**
* Is the manager for the resource objects in this portal.
*
* Es el administrador de los objetos de recursos en este portal.
*/
private static SWBResourceMgr resmgr = null;
/**
* Is the manager for the template objects in this portal.
*
* Es el administrador de los objetos de plantillas en este portal.
*/
private static SWBTemplateMgr templatemgr = null;
/**
* Is the manager for the service objects in this portal.
*
* Es el administrador de los objetos de servicios en este portal.
*/
private static SWBServiceMgr servicemgr = null;
/**
* Is the manager for the admin filters.
*
* Es el administrador de los filtros de administracion.
*/
private static SWBAdminFilterMgr adminfiltermgr = null;
/**
* Is the manager for the database log objects in this portal.
*
* Es el administrador de los objetos de bitácoras de base de datos
* en este portal.
*/
private static SWBDBAdmLog admlog = null;
/**
* Is the message center in this portal.
*
* Es el centro de mensajes en este portal.
*/
private static SWBMessageCenter msgcenter = null;
/**
* The procesor.
*/
private static SWBMessageProcesor procesor = null;
/**
* Is the manager for the access logs in this portal.
*
* Es el administrador de las bitácoras de acceso en este portal.
*/
private static SWBAccessLog acclog = null;
/**
* Is the manager for the access increment object in this portal.
*
* Es el administrador del incremento de accesos a objetos en este
* portal.
*/
private static SWBAccessIncrement accInc = null;
/**
* Is the manager for the index objects in this portal.
*
* Es el administrador de los objetos de indizado en este portal.
*/
private static SWBIndexMgr indmgr = null;
/**
* GeoIp Service Interface
*/
private static com.maxmind.geoip.LookupService geoip = null;
/**
* Link to AWS Services
*/
private static SWBCloud cloudServices = null;
/**
* Creates an object of this class, calling method {@code createInstance(ServletContext, Filter) with.
*
* @param servletContext the {@code ServletContex} agregated to the new instance
*
* @return SWBPortal the new instance of this component
* {@code Filter}'s value equal to {@code null}.
*
* Crea un objeto de esta clase, llamando al método {@code createInstance(ServletContext, Filter)
* con el valor de {@code Filter} igual a {@code null}.
*/
static public synchronized SWBPortal createInstance(ServletContext servletContext) {
return createInstance(servletContext, null);
}
/**
* Creates an object of this class with the {@code ServletContext} and
* {@code Filter} indicated.
*
* Crea un objeto de esta clase con el {@code ServletContext} y
* {@code Filter} indicados.
*
* @param servletContext the {@code ServletContex} agregated to the new
* instance
* @param filter the {@code Filter} assigned to the new instance's virtual
* host filter
* @return SWBPortal the new instance of this component
*/
static public synchronized SWBPortal createInstance(ServletContext servletContext, Filter filter) {
if (instance == null) {
SWBPortal.virtualHostFilter = filter;
instance = new SWBPortal();
SWBPortal.servletContext = servletContext;
String dbfile = SWBUtils.getApplicationPath() + "/swbadmin/geoip/GeoIP.dat";
try {
SWBPortal.geoip = new com.maxmind.geoip.LookupService(dbfile, com.maxmind.geoip.LookupService.GEOIP_MEMORY_CACHE);
Runtime.getRuntime().addShutdownHook(new Thread() {
@Override
public void run() {
SWBPortal.geoip.close();
}
});
} catch (IOException ioe) {
log.warn("can't find GeoIP.dat file", ioe);
SWBPortal.geoip = null;
}
}
return instance;
}
/**
* Constructor that initializes the environment of its new instance by
* calling method {@code init()}
*
* Constructor que inicia el ambiente de su nueva instancia llamando al
* método {@code init()}
.
*/
private SWBPortal() {
log.event("Initializing SemanticWebBuilder Portal...");
init();
}
/**
* Prepares the environment necessary to provide site and content management
* services. This involves the following operations:
* - configuring the values for workpath and SMTPServer environment
* variables, and some other variables from SWBPlatform
* - verifing the existence of the database and its tables
* - loading of classes indicated in startup.properties file
* - executing method {@code loadSemanticModels}
* - creating the administration site, if it does not exist yet
* - creating the onthology editor site, if it does not exist yet
* - creating the administration user repository, if it does not exist
* yet
* - creating the global site, if it does not exist yet
* - creating the default user repository, if it does not exist yet
* - creating the log tables
* - initializing the objects: {@code SWBUserMgr}, {@code SWBMonitor},
* {@code SWBResourceMgr}, {@code PFlowManager}, {@code SWBTemplateMgr},
* {@code SWBServiceMgr}, {@code SWBDBAdmLog}, {@code SWBMessageCenter},
* {@code SWBAccessLog}, {@code SWBAccessIncrement} and {@code SWBIndexMgr},
* wich can be accesed directly from this class, and {@code RuleMgr}
* - loading of files in SWBAdmin.jar and dojo.jar
*
*
* Prepara el ambiente necesario para proveer servicios de
* administración de sitios y contenido. Esto involucra las
* siguientes operaciones:
*
* - configurar valores de variables de ambiente de SWBPortal y
* {@link SWBPlatform} como: la ruta del directorio de trabajo o el dominio
* de servidor SMTP
* - verificar la existencia de una base de datos y sus tablas
* - cargar las clases indicadas en el archivo startup.properties
* - executar el método {@code loadSemanticModels}
* - crear el sitio de administración, si aún no existe
* - crear el sitio de edición de ontologías, si aún
* no existe
* - crear el repositorio de usuarios del sitio de administración,
* si aún no existe
* - crear el sitio global, si aún no existe
* - crear el repositorio de usuarios por defecto, si aún no
* existe
* - crear las tablas de base de datos para la bitácora
* - inicializar los objetos: {@code SWBUserMgr}, {@code SWBMonitor},
* {@code SWBResourceMgr}, {@code PFlowManager}, {@code SWBTemplateMgr},
* {@code SWBServiceMgr}, {@code SWBDBAdmLog}, {@code SWBMessageCenter},
* {@code SWBAccessLog}, {@code SWBAccessIncrement} y {@code SWBIndexMgr},
* que se pueden acceder directamente de esta clase, y {@code RuleMgr}
* - cargar los archivos contenidos en SWBAdmin.jar y dojo.jar
*
*
*/
private void init() {
props = SWBUtils.TEXT.getPropertyFile("/web.properties");
try {
secProps = SWBUtils.TEXT.getPropertyFile("/security.properties");
} catch (NullPointerException npe) {
secProps = null;
}
workPath = getEnv("swb/workPath");
SWBUtils.EMAIL.setSMTPSsl(Boolean.parseBoolean(getEnv("swb/smtpSsl", "false")));
SWBUtils.EMAIL.setSMTPServer(getEnv("swb/smtpServer"));
SWBUtils.EMAIL.setSMTPPort(Integer.parseInt(getEnv("swb/smtpPort", "0")));
SWBUtils.EMAIL.setSMTPTls(Boolean.parseBoolean(getEnv("swb/smtpTls", "false")));
SWBUtils.EMAIL.setSMTPUser(getEnv("swb/smtpUser"));
SWBUtils.EMAIL.setSMTPPassword(getEnv("swb/smtpPassword"));
SWBUtils.EMAIL.setAdminEmail(getEnv("af/adminEmail"));
standalone = getEnv("swb/clientServer", "SASC").equalsIgnoreCase("SASC");
client = !getEnv("swb/clientServer", "SASC").equalsIgnoreCase("Server") && !getEnv("swb/clientServer", "SASC").equalsIgnoreCase("SAS") && !standalone;
try {
//TODO:revisar sincronizacion
//if (confCS.equalsIgnoreCase("Client")) remoteWorkPath = (String) AFUtils.getInstance().getEnv("swb/remoteWorkPath");
workPath = (String) getEnv("swb/workPath", "/work");
if (workPath.startsWith("file:")) {
workPath = (new File(workPath.substring(5))).toString();
workPathType = WORKPATH_ABSOLUTE;
} else if (workPath.startsWith("http://")) {
workPath = (URLEncoder.encode(workPath));
workPathType = WORKPATH_REMOTE;
} else {
workPath = SWBUtils.getApplicationPath() + workPath;
workPathType = WORKPATH_RELATIVE;
}
} catch (Exception e) {
log.error("Can't read the context path...", e);
workPath = "";
}
//Insercion de parametros a Platform
SWBPlatform platform = SWBPlatform.createInstance();
SWBPlatform.setPortalLoaded(true);
platform.setPlatformWorkPath(workPath);
platform.setStatementsCache(getEnv("swb/ts_statementsCache", "false").equals("false"));
String persistType = getEnv("swb/triplepersist", SWBPlatform.PRESIST_TYPE_DEFAULT);
platform.setPersistenceType(persistType);
platform.setAdminDev(getEnv("swb/adminDev", "false").equalsIgnoreCase("true"));
platform.setAdminFile(getEnv("swb/adminFile", "/swbadmin/rdf/SWBAdmin.nt"));
platform.setOntEditFile(getEnv("swb/ontEditFile", "/swbadmin/rdf/SWBOntEdit.nt"));
platform.setProperties(props);
platform.setSecurityProperties(secProps);
try {
SWBUtils.DB.setDefaultPool(getEnv("swb/db/nameconn", getEnv("wb/db/nameconn", "swb")));
Connection con = SWBUtils.DB.getDefaultConnection();
if (con != null) {
log.info("-->Database: " + SWBUtils.DB.getDatabaseName());
if (SWBUtils.DB.getDatabaseName().startsWith("HSQL")) {
checkHSQLHack(con); //MAPS74
}
haveDB = true;
haveDBTables = true;
con.close();
}
if (!haveDB()) {
log.warn("-->Default SemanticWebBuilder database not found...");
return;
}
if (!haveDBTables()) {
log.warn("-->Default SemanticWebBuilder database tables not found...");
return;
}
try {
System.setProperty("sun.net.client.defaultConnectTimeout", getEnv("swb/defaultConnectTimeout", "5000"));
System.setProperty("sun.net.client.defaultReadTimeout", getEnv("swb/defaultReadTimeout", "30000"));
} catch (Exception e) {
log.error("Error seting defaultConnectTimeout, defaultReadTimeout");
}
//TODO: agregar modelos
//semanticMgr.init(this);
// Timestamp initime = null;
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_InstanceConfiguration") + util.getEnv("wb/clientServer"), true);
// cpus = getNumProcessors();
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_ProcessorNumber") + cpus, true);
// util.log("IP:\t\t\t"+java.net.InetAddress.getLocalHost(),true);
//
// try
// {
// UpdateVersion updater = new UpdateVersion(version);
// updater.update();
// } catch (Exception e)
// {
// AFUtils.log(e, com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "error_WBLoader_Init_versioneerror"), true);
// }
//
if (!"ignore".equals(getEnv("swb/security.auth.login.config", "ignore"))) {
System.setProperty("java.security.auth.login.config", SWBUtils.getApplicationPath() + "/WEB-INF/classes" + getEnv("swb/security.auth.login.config", "/wb_jaas.config"));
log.info("-->Login Config:" + System.getProperty("java.security.auth.login.config"));
}
// String dbsync = util.getEnv("wb/DBSync","false");
// if (wbutil.isClient() || dbsync.trim().equalsIgnoreCase("true"))
// {
// initime = DBDbSync.getInstance().getLastUpdate();
// objs.add(DBDbSync.getInstance());
// }
//
// //if admin
// if (!wbutil.isClient())
// {
// objs.add(DBResHits.getInstance());
// objs.add(DBAdmLog.getInstance());
// objs.add(WBAccessLog.getInstance());
// objs.add(WBIndexMgr.getInstance());
// //objs.add(WBFileReplicatorSrv.getInstance());
// } else
// {
// util.log("remoteWorkPath --> " + wbutil.getRemoteWorkPath(), true);
// }
//
// if (wbutil.isClient() || dbsync.trim().equalsIgnoreCase("true"))
// {
// timer = WBDBSyncTimer.getInstance();
// timer.init();
// objs.add(timer);
// }
//
//
// Runtime.getRuntime().gc();
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_totalMemory") + Runtime.getRuntime().totalMemory(), true);
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_FreeMemory") + "\t\t" + Runtime.getRuntime().freeMemory(), true);
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_MemoryUsed") + "\t\t" + (Runtime.getRuntime().totalMemory() - Runtime.getRuntime().freeMemory()), true);
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_MemoryUsedbyWb") + "\t" + (Runtime.getRuntime().totalMemory() - Runtime.getRuntime().freeMemory() - inimem), true);
//
// util.log(com.infotec.appfw.util.AFUtils.getLocaleString("locale_core", "log_WBLoader_Init_WbInicializing"), true);
} catch (Throwable e) {
log.error("Error loading SemanticWebBuilder Instance...", e);
}
loadSemanticModels();
loadAdminFiles();
resmgr = new SWBResourceMgr();
try {
WebSite site = SWBContext.getAdminWebSite();
if (site == null) {
log.event("Creating Admin WebSite...");
SWBPlatform.getSemanticMgr().createModel(SWBContext.WEBSITE_ADMIN, "http://www.semanticwb.org/SWBAdmin#");
//MAPS74
SWBPlatform.getSemanticMgr().createKeyPair();
}
// site = SWBContext.getOntEditor();
// if (site == null)
// {
// log.event("Creating Ontology Editor WebSite...");
// SWBPlatform.getSemanticMgr().createModel(SWBContext.WEBSITE_ONTEDITOR, "http://www.semanticwb.org/SWBOntEdit#");
// }
UserRepository urep = SWBContext.getAdminRepository();
if (urep == null) {
File nt=new File(SWBUtils.getApplicationPath()+"/swbadmin/rdf/uradm.nt");
if(nt.exists())
{
log.event("Importing Admin User Repository...");
SemanticModel model=SWBPlatform.getSemanticMgr().createDBModelByRDF(SWBContext.USERREPOSITORY_ADMIN, "http://www.semanticwb.org/uradm#", new FileInputStream(nt), "N-TRIPLE");
urep = SWBContext.getAdminRepository();
}else
{
log.event("Creating Admin User Repository...");
urep = SWBContext.createUserRepository(SWBContext.USERREPOSITORY_ADMIN, "http://www.semanticwb.org/uradm#");
urep.setTitle("Admin User Repository");
urep.setDescription("Admin User Repository");
//MAPS74 - Cambiado a semantic prop
urep.setAuthMethod("FORM");
urep.setLoginContext("swb4TripleStoreModule");
urep.setCallBackHandlerClassName("org.semanticwb.security.auth.SWB4CallbackHandlerLoginPasswordImp");
urep.setUndeleteable(true);
//Create User
User user = urep.createUser();
user.setLogin("admin");
user.setPassword("webbuilder");
user.setEmail("[email protected]");
user.setFirstName("Admin");
user.setLanguage("es");
user.setCreated(new Date());
user.setUpdated(new Date());
user.setActive(true);
UserGroup grp1 = urep.createUserGroup("admin");
grp1.setTitle("Administrator", "en");
grp1.setTitle("Administrador", "es");
grp1.setCreated(new Date());
grp1.setUpdated(new Date());
grp1.setCreator(user);
grp1.setModifiedBy(user);
grp1.setUndeleteable(true);
UserGroup grp2 = urep.createUserGroup("su");
grp2.setTitle("Super User", "en");
grp2.setTitle("Super Usuario", "es");
grp2.setCreated(new Date());
grp2.setUpdated(new Date());
grp2.setCreator(user);
grp2.setModifiedBy(user);
grp2.setUndeleteable(true);
grp2.setParent(grp1);
user.addUserGroup(grp1);
}
try {
site = SWBContext.getWebSite("basico");
if (site == null) {
File file = new File(getWorkPath() + "/sitetemplates/basico.zip");
if (file.exists()) {
log.event("Creating Demo WebSite...");
UTIL.InstallZip(file);
}
}
} catch (Exception e) {
log.error(e);
}
}
//Check for GlobalWebSite
site = SWBContext.getGlobalWebSite();
if (site == null) {
File file = new File(getWorkPath() + "/sitetemplates/"+SWBContext.WEBSITE_GLOBAL+".zip");
if (file.exists()) {
log.event("Importing Global WebSite...");
UTIL.InstallZip(file);
site = SWBContext.getGlobalWebSite();
}else
{
log.event("Creating Global WebSite...");
site = SWBContext.createWebSite(SWBContext.WEBSITE_GLOBAL, "http://www.semanticwb.org/wsglobal#");
site.setTitle("Global");
site.setDescription("Global WebSite");
site.setActive(true);
site.setUndeleteable(true);
//Crear lenguajes por defecto
Language lang = site.createLanguage("es");
lang.setTitle("Español", "es");
lang.setTitle("Spanish", "en");
lang = site.createLanguage("en");
lang.setTitle("Ingles", "es");
lang.setTitle("English", "en");
//Create HomePage
// WebPage home = site.createWebPage("home");
// site.setHomePage(home);
// home.setActive(true);
//Create DNS
// Dns dns = site.createDns("localhost");
// dns.setTitle("localhost");
// dns.setDescription("DNS por default", "es");
// dns.setDescription("Default DNS", "en");
// dns.setDefault(true);
// dns.setWebPage(home);
}
}
urep = SWBContext.getDefaultRepository();
if (urep == null) {
log.event("Creating Default User Repository...");
urep = SWBContext.createUserRepository(SWBContext.USERREPOSITORY_DEFAULT, "http://www.semanticwb.org/urswb#");
urep.setTitle("Default UserRepository");
urep.setDescription("Default UserRpository");
//MAPS74 - Cambiado a semantic prop
urep.setAuthMethod("FORM");
urep.setLoginContext("swb4TripleStoreModule");
urep.setCallBackHandlerClassName("org.semanticwb.security.auth.SWB4CallbackHandlerLoginPasswordImp");
site.setUserRepository(urep);
}
} catch (Exception e) {
e.printStackTrace();
}
//Crear tablas LOGS
try {
Connection con = SWBUtils.DB.getDefaultConnection();
try {
ResultSet rs = con.getMetaData().getTables(null, null, "%", null);
boolean hasTable = false;
while (rs.next()) {
if (rs.getString(3).toLowerCase().indexOf("swb_admlog") > -1) {
hasTable = true;
}
}
if (!hasTable) {
log.event("Creating Logs Tables... " + SWBUtils.DB.getDatabaseName());
GenericDB db = new GenericDB();
String xml = SWBUtils.IO.getFileFromPath(SWBUtils.getApplicationPath() + "/WEB-INF/xml/swb_logs.xml");
db.executeSQLScript(xml, SWBUtils.DB.getDatabaseName(), null);
}
rs.close();
} catch (SQLException e) {
log.error(e);
}
con.close();
} catch (SQLException e) {
log.error(e);
}
usrMgr = new SWBUserMgr();
usrMgr.init();
monitor = new SWBMonitor();
monitor.init();
resmgr.init();
pflowMgr = new PFlowManager();
pflowMgr.init();
templatemgr = new SWBTemplateMgr();
templatemgr.init();
admlog = new SWBDBAdmLog();
admlog.init();
msgcenter = new SWBMessageCenter();
msgcenter.init();
platform.setMessageCenter(msgcenter);
procesor = new SWBMessageProcesor(msgcenter);
procesor.init();
acclog = new SWBAccessLog();
acclog.init();
accInc = new SWBAccessIncrement();
accInc.init();
adminfiltermgr = new SWBAdminFilterMgr();
adminfiltermgr.init();
servicemgr = new SWBServiceMgr();
servicemgr.init();
indmgr = new SWBIndexMgr();
indmgr.init();
log.event("Loading Friendly Urls...");
FriendlyURL.refresh();
//Inicializa el RuleMgr
Rule.getRuleMgr();
if (!"ignore".equals(getEnv("swb/cloudImplementation", "ignore"))) {
String cloudClass = getEnv("swb/cloudImplementation");
try {
Class clazz = Class.forName(cloudClass);
cloudServices = (SWBCloud) clazz.newInstance();
new Thread() {
public void run() {
cloudServices.launch();
}
}.start();
log.event("CloudServices enabled...");
} catch (Exception roe) { //catching Reflection and Thread Exceptions
log.event("Can't instantiate cloud services", roe);
assert false;
}
}
try {
//cargando clases de startup.properties
SWBStartup startup = new SWBStartup(new ArrayList()); //objs);
startup.load("startup.properties");
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Load admin files.
*/
public void loadAdminFiles() {
log.event("Loading Admin Files...");
admFiles = new ConcurrentHashMap();
try {
log.debug("Loading admin Files from: /WEB-INF/lib/SWBAdmin.jar");
String zipPath = SWBUtils.getApplicationPath() + "/WEB-INF/lib/SWBAdmin.jar";
ZipFile zf = new ZipFile(zipPath);
Enumeration e = zf.entries();
while (e.hasMoreElements()) {
ZipEntry ze = (ZipEntry) e.nextElement();
log.debug("/" + ze.getName() + ", " + ze.getSize() + ", " + ze.getTime());
admFiles.put("/" + ze.getName(), new JarFile(ze, zf));
}
zf.close();
//log.event("-->Admin Files in Memory:\t" + admFiles.size());
} catch (Exception e) {
log.warn("Error loading files for Webbuilder Administration:" + SWBUtils.getApplicationPath() + "/WEB-INF/lib/SWBAdmin.jar", e);
}
try {
log.debug("Loading admin Files from: /WEB-INF/lib/dojo.zip");
String zipPath = SWBUtils.getApplicationPath() + "/WEB-INF/lib/dojo.zip";
ZipFile zf = new ZipFile(zipPath);
Enumeration e = zf.entries();
while (e.hasMoreElements()) {
ZipEntry ze = (ZipEntry) e.nextElement();
log.debug("/" + ze.getName() + ", " + ze.getSize() + ", " + ze.getTime());
admFiles.put("/" + ze.getName(), new JarFile(ze, zf));
}
zf.close();
log.event("-->Admin Files in Memory:\t" + admFiles.size());
} catch (Exception e) {
log.warn("Error loading files for Webbuilder Administration:" + SWBUtils.getApplicationPath() + "/WEB-INF/lib/dojo.zip", e);
}
}
/**
* Loads the model discribed by each onthology indicated in
* {@code swb/ontologyFiles} environment variable.
*
* Carga el modelo descrito para cada ontología indicada en
* {@code swb/ontologyFiles}.
*/
public void loadSemanticModels() {
log.event("Loading SemanticModels...");
String owlPath = getEnv("swb/ontologyFiles", "/WEB-INF/owl/swb.owl");
SWBPlatform.getSemanticMgr().initializeDB();
//Load Ontology from file
StringTokenizer st = new StringTokenizer(owlPath, ",;");
while (st.hasMoreTokens()) {
String file = st.nextToken();
String swbowl = "file:" + SWBUtils.getApplicationPath() + file;
log.info("Loading OWL:" + swbowl);
SemanticModel model = SWBPlatform.getSemanticMgr().addBaseOntology(swbowl);
}
SWBPlatform.getSemanticMgr().loadBaseVocabulary();
//System.out.println("End Vocabulary");
// ontoModel.loadImports();
// ontoModel.getDocumentManager().addAltEntry(source,"file:"+SWBUtils.getApplicationPath()+"/WEB-INF/owl/swb.owl" );
// ontoModel.read(source);
// Reasoner reasoner = ReasonerRegistry.getOWLReasoner();
// reasoner = reasoner.bindSchema(swbSquema);
// InfModel infmodel = ModelFactory.createInfModel(reasoner, adminModel);
//adminModel.createResource("http://www.sep.gob.mx/site/WBAdmin",ontoModel.getResource("http://www.semanticwebbuilder.org/swb4/ontology#WebSite"));
//adminModel.createResource(ontoModel.getResource("http://www.semanticwebbuilder.org/swb4/ontology#WebPage"));
//debugClasses(ontoModel);
//debugModel(ontoModel);
//load SWBSystem Model
// log.debug("Loading DBModel:"+"SWBSystem");
// m_system=new SemanticModel("SWBSystem",loadRDFDBModel("SWBSystem"));
//// debugModel(m_system);
// if(SWBPlatform.isUseDB())m_ontology.addSubModel(m_system,false);
SWBPlatform.getSemanticMgr().loadDBModels();
if (getEnv("swb/addModel_DBPedia", "false").equals("true")) {
SWBPlatform.getSemanticMgr().loadRemoteModel("DBPedia", "http://dbpedia.org/sparql", "http://dbpedia.org/resource/", false);
}
// if(SWBPlatform.getEnv("swb/addOnt_DBPedia","false").equals("true"))
// {
// loadRemoteModel("Books", "http://www.sparql.org/books", "http://example.org/book/",false);
// }
//TODO agregar RDFa
SWBPlatform.getSemanticMgr().rebind();
}
/**
* Gets the context path for this resource's instance. For example:
* {@literal /swb}, or {@literal /} if the context is the root context.
*
* Obtiene la ruta del contexto para esta instancia de portal.
Por
* ejemplo:
*
* @return the string representing the context path for this resource's
* instance. {@literal /swb}, o {@literal /} si es el contexto
* raíz
*/
public static String getContextPath() {
if (instance != null) {
return instance.contextPath;
}
return "";
}
/**
* Sets the value for this portal instance's context path.
*
* Fija el valor de la ruta del contexto de esta instancia de portal
*
* @param contextPath the string representing the new path for the portal
* context. If the string ends with a {@literal /}, the value stored won't
* contain it. This is:
value received {@literal /swb/} -> value stored
* {@literal /swb}
*/
public void setContextPath(String contextPath) {
log.event("ContextPath:" + contextPath);
this.contextPath = contextPath;
try {
if (getEnv("swb/webWorkPath", "/work").startsWith("/")) {
if (contextPath.endsWith("/")) {
webWorkPath = contextPath + getEnv("swb/webWorkPath", "/work").substring(1);
} else {
webWorkPath = contextPath + getEnv("swb/webWorkPath", "/work");
}
} else {
webWorkPath = getEnv("swb/webWorkPath", "/work");
}
} catch (Exception e) {
log.error("Can't read the context path...", e);
webWorkPath = "";
}
SWBPlatform.createInstance().setContextPath(contextPath);
}
/**
* Gets this portal work directory's physical path. For
* example:
/opt/swb/work
*
* Obtiene la ruta física del directorio de trabajo del portal
* Ejemplo: /opt/swb/work
*
* @return a string representing the work directory's physical path
*/
public static String getWorkPath() {
return workPath;
}
/**
* Gets the work path type.
*
* @return the work path type
*/
public static String getWorkPathType() {
return workPathType;
}
/**
* Gets the web path for this portal work directory.
*
For example: /[context]/work
*
* Obtiene ruta web del directorio de trabajo del portal Ejemplo:
* /[context]/work
*
* @return a string representing the work directory's web path
*/
public static String getWebWorkPath() {
return webWorkPath;
}
/**
* Retrieves the value of the environment variable whose name matches
* {@code name}. The variable to look for might be declared into either
* web.xml or web.properties files.
*
* Obtiene el valor de una variable de ambiente cuyo nombre coincida con el
* valor contenido en {@code name}. La variable a buscar puede estar
* declarada en los archivos web.xml o web.properties.
*
* @param name a string representing the environment variable's name to find
* @return a string representing the environment variable's value. In case
* the indicated variable is not found, {@code null} will be returned.
*/
public static String getEnv(String name) {
return getEnv(name, null);
}
/**
* Retrieves the environment variable's value whose name matches the value
* of.
*
* @param name un string que indica el nombre de la variable a buscar
* @param defect un string con el valor a devolder por defecto, en caso de
* no encontrar la variable.
* @return un string con el valor de la variable indicada por {@code name},
* si existe, en caso contrario devuelve el valor de {@code defect}.
* {@code name}, or returns the value of {@code defect}. The searched
* variable might be declared in web.xml or web.properties files.
*
* Obtiene el valor de la variable de ambiente cuyo nombre coincida con el
* valor contenido en {@code name}, o devuelve el valor de {@code defect}.
* La variable a buscar puede estar declarada en los archivos web.xml o
* web.properties.
*/
public static String getEnv(String name, String defect) {
String obj = null;
obj = System.getProperty("swb.web." + name);
if (obj != null) {
return obj;
}
if (props != null) {
obj = props.getProperty(name);
}
if (obj == null) {
return defect;
}
return obj;
}
/**
* Retrieves the security environment variable's value whose name matches
* the value of.
*
* @param name un string que indica el nombre de la variable a buscar
* @param defect un string con el valor a devolder por defecto, en caso de
* no encontrar la variable.
* @return un string con el valor de la variable indicada por {@code name},
* si existe, en caso contrario devuelve el valor de {@code defect}.
* {@code name}, or returns the value of {@code defect}. The searched
* variable might be declared in security.properties files.
*
* Obtiene el valor de la variable de ambiente cuyo nombre coincida con el
* valor contenido en {@code name}, o devuelve el valor de {@code defect}.
* La variable a buscar puede estar declarada en el archivo
* security.properties.
*/
public static String getSecEnv(String name, String defect) {
String obj = null;
if (secProps != null) {
obj = secProps.getProperty(name);
}
if (obj == null) {
return defect;
}
return obj;
}
/**
* Gets a reference to the servletContext associated to this object
*
* Obtiene el servletContext asociado a este recurso.
*
* @return the servletContext used in this object's creation
*/
public static ServletContext getServletContext() {
return servletContext;
}
/**
* Gets a reference to the filter associated to this resource.
*
* Obtiene el filter asociado a este recurso.
*
* @return the filter used in this object creation, {@code null} if no
* filter was specified.
*/
public static Filter getVirtualHostFilter() {
return virtualHostFilter;
}
/**
* Gets a reference to the object holding the properties declared in
* {@literal web.properties} file.
*
* Obtiene la referencia a todas las propiedades declaradas en el archivo
* web.properties.
*
* @return the properties object wich stores the configured properties in
* {@literal web.properties} file.
*/
public static Properties getWebProperties() {
return props;
}
/**
* Indicates if this instance of portal has a database related to it.
*
* Indica si esta instancia de portal tiene relacionada una base de
* datos.
*
* @return the boolean indicating if a database existis for this instance of
* portal.
*/
public static boolean haveDB() {
return haveDB;
}
/**
* Indicates if this instance of portal has database tables to work with.
*
* Indica si esta instancia de portal tiene las tablas de base de datos
* necesarias para trabajar.
*
* @return the boolean indicating if this instance of portal has database
* tables to work with.
*/
public static boolean haveDBTables() {
return haveDBTables;
}
/**
* Indicates if this instance of portal is working with a database.
*
* Indica si esta instancia de portal está trabajanso con una base de
* datos.
*
* @return the boolean indicating if this instance of portal is working with
* a database.
*/
public static boolean isUseDB() {
return useDB;
}
/**
* Modifies the indicator of this instance of portal to work with a
* database.
*
* Modifica el indicador de esta instancia de portal para trabajar con una
* base de datos.
*
* @param useDB a boolean that indicates to this instance of portal if it
* has to use a database.
*/
public static void setUseDB(boolean useDB) {
SWBPortal.useDB = useDB;
}
/**
* Deletes a file whose representing path is within the work directory of
* this portal's instance.
*
* Elimina un archivo cuya ruta se encuentra dentro del directorio de
* trabajo de esta instancia de portal.
*
* @param path the string representing the file to delete, which has to be
* within the work directory.
*/
public static void removeFileFromWorkPath(String path) {
//TOTO:Impementar Replicacion de archivos
File file = new File(getWorkPath() + path);
file.delete();
}
/**
* Reads a file whose path is located within this portal's work directory.
*
* Lee un archivo cuya ruta está ubicada dentro del directorio de
* trabajo de este portal.
*
* @param path the string representing the file's path to read, which has to
* be within the work directory.
* @return an inputStream with the indicated file's content
* @throws SWBException if the file path indicated cannot be located within
* the work directory or is equal to {@code null}
*/
public static InputStream getFileFromWorkPath(String path) throws SWBException {
InputStream ret = null;
//TOTO:Impementar Replicacion de archivos
try {
// String confCS = (String) getEnv("swb/clientServer");
//
// if(confCS.equalsIgnoreCase("ClientFR")) {
// try {
// ret = new FileInputStream(getWorkPath() + path);
// }catch(FileNotFoundException fnfe) {
// //ret=getFileFromAdminWorkPath(path);
// DownloadDirectory downdir=new DownloadDirectory(AFUtils.getEnv("wb/serverURL"),getWorkPath(),"workpath");
// downdir.download(path);
// ret = new FileInputStream(getWorkPath() + path);
// }
// }else if(confCS.equalsIgnoreCase("Client")) {
// ret=getFileFromAdminWorkPath(path);
// } else {
ret = new FileInputStream(getWorkPath() + path);
// }
} catch (Exception e) {
throw new SWBException(e.getMessage(), e);
}
return ret;
}
//TOTO:Impementar Replicacion de archivos
// public InputStream getFileFromAdminWorkPath(String path) throws MalformedURLException, IOException {
// InputStream ret = null;
// //String str = getRemoteWorkPath() + path;
// String servlet=DownloadDirectory.SERVDWN;
// String str = getEnv("swb/serverURL")+servlet+ "?workpath=" + path;
//
// str = str.substring(0, str.lastIndexOf("/") + 1) + com.infotec.appfw.util.URLEncoder.encode(str.substring(str.lastIndexOf("/") + 1));
// ret = new java.net.URL(str).openStream();
// return ret;
// }
/**
* Creates a file to the path and with the content indicated.
*
* Crea un archivo en la ruta y con el contenido indicados.
*
* @param path a string representing the path within the work directory to
* create the file
* @param in an input stream with the new file's content
* @param user the user
* @throws org.semanticwb.SWBException if the path specified cannot be
* created or is {@code null}
* @throws SWBException the sWB exception
*/
public static void writeFileToWorkPath(String path, InputStream in, User user) throws SWBException {
//TOTO:Impementar Replicacion de archivos
try {
// String confCS = (String) AFUtils.getInstance().getEnv("wb/clientServer");
//
// //System.out.println("clientServer:"+confCS);
// if(confCS.equalsIgnoreCase("ClientFR")||confCS.equalsIgnoreCase("Client")) {
// String str = AFUtils.getEnv("wb/serverURL")+DownloadDirectory.SERVUP;
// URL url=new URL(str);
// //System.out.println("url:"+url);
// URLConnection urlconn=url.openConnection();
// //if(jsess!=null)urlconn.setRequestProperty("Cookie", "JSESSIONID="+jsess);
// urlconn.setRequestProperty("type","workpath");
// urlconn.setRequestProperty("path",path);
// urlconn.setRequestProperty("user",userid);
// urlconn.setDoOutput(true);
// AFUtils.copyStream(in,urlconn.getOutputStream());
// //System.out.println("copyStream");
// String ret=AFUtils.getInstance().readInputStream(urlconn.getInputStream());
// //System.out.println("ret:"+ret);
// }else {
File file = new File(getWorkPath() + path);
file.getParentFile().mkdirs();
FileOutputStream out = new FileOutputStream(file);
SWBUtils.IO.copyStream(in, out);
// }
} catch (Exception e) {
throw new SWBException(e.getMessage(), e);
}
}
/**
* Reads a file whose path exists within the work directory and puts its
* content into a string.
*
* Lee un archivo cuya ruta existe dentro del directorio de trabajo
* ({@literal work}) y coloca su contenido en una string.
*
* @param path the string representing the file's path within the work
* directory
* @return a stream with the indicated file's content
* @throws IOException if the file specified cannot be read.
* @throws SWBException the sWB exception
*/
public static String readFileFromWorkPath(String path) throws IOException, SWBException {
return SWBUtils.IO.readInputStream(getFileFromWorkPath(path));
}
/**
* Reads a file whose path is located within this portal's work directory
* using a specified character code. It uses the method
* {@code getFileFromWorkPath(String)}'s services.
*
* Lee un archivo cuya ruta se ubica dentro del directorio de trabajo
* ({@code work}) de este portal utilizando el código de caracteres
* especificado. Utiliza los servicios del método
* {@code getFileFromWorkPath(String)}.
*
* @param path a string representing the file's path to read, which has to
* be within the work directory.
* @param encode a string representing the character code to use while
* reading the file's content.
* @return a stream with the indicated file's content
* @throws org.semanticwb.SWBException if there's a problem while reading
* the file's content.
* @throws SWBException the sWB exception
*/
public static InputStreamReader getFileFromWorkPath(String path, String encode) throws SWBException {
InputStreamReader ret = null;
try {
ret = new InputStreamReader(getFileFromWorkPath(path), encode);
} catch (Exception e) {
throw new SWBException(e.getMessage(), e);
}
return ret;
}
/**
* Reads a file whose path exists within the work directory and puts the
* file's content into a string.
*
* Lee un archivo cuya ruta existe dentro del directorio {@code work} y
* coloca el contenido del archivo en una string.
*
* @param path the string representing the file's path within the work
* directory
* @param encode a string representing the character code to use while
* reading the file's content.
* @return a string representing the specified file's content
* @throws SWBException if there's a problem while reading the file's
* content.
*/
public static String readFileFromWorkPath(String path, String encode) throws SWBException {
StringBuilder ret = new StringBuilder(IO.getBufferSize());
try {
InputStreamReader file = getFileFromWorkPath(path, encode);
char[] bfile = new char[SWBUtils.IO.getBufferSize()];
int x;
while ((x = file.read(bfile, 0, SWBUtils.IO.getBufferSize())) > -1) {
ret.append(bfile, 0, x);
}
file.close();
} catch (Exception e) {
throw new SWBException(e.getMessage(), e);
}
return ret.toString();
}
//TODO:validar client
/**
* Indicates if the SemanticWebBuilder instance is configured as a client.
*
* Indica si esta instancia de SemanticWebBuilder (SWB) está
* configurada como cliente.
*
* @return a boolean that indicates if the SWB instance is configured as a
* client.
*/
public static boolean isClient() {
return client;
}
//TODO:
/**
* Indicates if the SemanticWebBuilder instance is configured as standalone.
*
* Indica si esta instancia de SemanticWebBuilder (SWB) está
* configurada como una sola maquina.
*
* @return a boolean that indicates if the SWB instance is configured as a
* stand alone.
*/
public static boolean isStandAlone() {
return standalone;
}
/**
* Gets the distributor path in this instance of SWB.
*
* Obtiene la ruta del distribuidor de esta instancia de SWB
*
* @return a string representing the distributor path within the context of
* this instance of SWB.
*/
public static String getDistributorPath() {
return getContextPath() + "/" + getEnv("swb/distributor", "swb");
}
/**
* Gets a reference to the user manager for this instance of SWB.
*
* Obtiene una referencia al administrador de usuarios de esta instancia de
* SWB
*
* @return a SWBUserMgr object for this instance of SWB
*/
public static SWBUserMgr getUserMgr() {
return usrMgr;
}
/**
* Gets a reference to the monitor for this instance of SWB.
*
* Obtiene una referencia al monitor de esta instancia de SWB
*
* @return a SWBMonitor object for this instance of SWB.
*/
public static SWBMonitor getMonitor() {
return monitor;
}
/**
* Gets a reference to the resource manager for this instance of SWB.
*
* Obtiene una referencia al administrador de recursos de esta instancia de
* SWB
*
* @return a SWBResourceMgr object for this instance of SWB.
*/
public static SWBResourceMgr getResourceMgr() {
return resmgr;
}
/**
* Gets a reference to the template manager for this instance of SWB.
*
* Obtiene una referencia al administrador de plantillas de esta instancia
* de SWB
*
* @return a SWBTemplateMgr object for this instance of SWB.
*/
public static SWBTemplateMgr getTemplateMgr() {
return templatemgr;
}
/**
* Gets a reference to the service manager for this instance of SWB.
*
* Obtiene una referencia al administrador de servicios de esta instancia de
* SWB
*
* @return a SWBServiceMgr object for this instance of SWB.
*/
public static SWBServiceMgr getServiceMgr() {
return servicemgr;
}
/**
* Gets a reference to the service manager for this instance of SWB.
*
* Obtiene una referencia al administrador de servicios de esta instancia de
* SWB
*
* @return a SWBServiceMgr object for this instance of SWB.
*/
public static SWBAdminFilterMgr getAdminFilterMgr() {
return adminfiltermgr;
}
/**
* Gets a reference to the database administration log for this instance of
* SWB.
*
* Obtiene una referencia a la bitácora de administración de
* base de datos de esta instancia de SWB
*
* @return a SWBDBAdmLog object for this instance of SWB.
*/
public static SWBDBAdmLog getDBAdmLog() {
return admlog;
}
/**
* Gets a reference to the message center for this instance of SWB.
*
* Obtiene una referencia al centro de mensajes de esta instancia de SWB
*
* @return a SWBMessageCenter object for this instance of SWB.
*/
public static SWBMessageCenter getMessageCenter() {
return msgcenter;
}
/**
* Gets a reference to the access log for this instance of SWB.
*
* Obtiene una referencia a la bitácora de accesos de esta instancia
* de SWB
*
* @return a SWBAccessLog object for this instance of SWB.
*/
public static SWBAccessLog getAccessLog() {
return acclog;
}
/**
* Gets a reference to the publication flow manager for this instance of
* SWB.
*
* Obtiene una referencia al administrador de flujos de publicación
* de esta instancia de SWB
*
* @return a PFlowManager object for this instance of SWB.
*/
public static PFlowManager getPFlowManager() {
return pflowMgr;
}
/**
* Gets a reference to the access incrementor for this instance of SWB.
*
* Obtiene una referencia al incrementador de accesos de esta instancia de
* SWB
*
* @return a SWBAccessIncrement object for this instance of SWB.
*/
public static SWBAccessIncrement getAccessIncrement() {
return accInc;
}
/**
* Gets a reference to the index manager for this instance of SWB.
*
* Obtiene una referencia al administrador de índices de esta
* instancia de SWB.
*
* @return a SWBIndexMgr object for this instance of SWB.
*/
public static SWBIndexMgr getIndexMgr() {
return indmgr;
}
/**
* Gets a file from the .jar files loaded at initialization of SWB. The
* requested file's path has to be within the structure directory of
* SWBAdmin.jar or dojo.jar.
*
* Extrae un archivo de los archivos .jar cargados en la
* inicialización de SWB. La ruta de archivo solicitada debe existir
* en la estructura de directorios de SWBAdmin.jar o dojo.jar.
*
* @param path a string representing an existing path within SWBAdmin.jar or
* dojo.jar files.
* @return a jarFile object with the specified file's content.
*/
public static JarFile getAdminFile(String path) {
JarFile f = (JarFile) admFiles.get(path);
if (f != null) {
JarFile aux = null;
if (f.isReplaced()) {
aux = new JarFile(path);
} else {
aux = f;
}
if (f.isExpired()) {
JarFile aux2 = new JarFile(path);
if (aux2.exists()) {
f.setReplaced(true);
aux = aux2;
} else {
if (f.isReplaced()) {
f.setReplaced(false);
}
}
f.touch();
}
f = aux;
} else {
f = new JarFile(path);
}
return f;
}
/**
* Gets a file from the .jar files loaded at initialization of SWB. The
* requested file's path has to be within the structure directory of
* SWBAdmin.jar or dojo.jar.
*
* Extrae un archivo de los archivo .jar cargados al inicializar SWB. La
* ruta de archivo solicitada debe existir en la estructura de directorios
* de SWBAdmin.jar o dojo.jar.
*
* @param path a string representing an existing path within SWBAdmin.jar or
* dojo.jar files.
* @return an input stream with the specified file's content.
*/
public static InputStream getAdminFileStream(String path) {
JarFile f = (JarFile) admFiles.get(path);
if (f == null) {
f = new JarFile(path);
}
if (!f.exists()) {
return null;
}
return f.getInputStream();
}
/**
* Retrieves all languages from all sites in this instance of SWB.
*
* Obtiene todos los lenguajes de todos los sitios en esta instancia de
* SWB
*
* @return an arrayList with all languages available in all sites
*/
public static ArrayList getAppLanguages() {
ArrayList languages = new ArrayList();
Iterator it = SWBContext.listWebSites();
while (it.hasNext()) {
WebSite site = it.next();
Iterator itLang = site.listLanguages();
while (itLang.hasNext()) {
Language lang = itLang.next();
if (!languages.contains(lang.getId())) {
languages.add(lang.getId());
}
}
}
return languages;
}
/**
* Gets the cloud services interface
*
* @return
*/
public final static SWBCloud getCloud() {
return cloudServices;
}
/**
* Combines general usefull operations for the portal platform, such as:
* relative path replacement within HTML code, search of anchors in HTML
* code, captcha like image generation, HTML in-code referenced file
* listing.
*
* Conjunta operaciones útiles a nivel general para la plataforma de
* portal, como: sustitución de rutas locales en código HTML,
* búsqueda de anclas en HTML, generación de imágenes
* tipo captcha, listado de archivos referenciados por código
* HTML.
*/
public static class UTIL {
/**
* a string that contains all characters in the english alphabet and the
* 10 digits.
*
* una string que contiene todos los caracteres del alfabeto
* inglés y los 10 dígitos del sistema decimal.
* Used to generate strings with characters selected randomly.
*/
private final static String ALPHABETH = "ABCDEFGHiJKLMNPQRSTUVWXYZ123456789+=?";
/**
* Looks for relative paths in the attributes of HTML tags and replaces
* their values with {@code ruta}, the content will be displayed in one
* single page. The HTML tags and elements evaluated are the same as for
* the {@code parseHTML(String, String, int)} method.
*
* Busca rutas relativas en los atributos de tags de HTML y remplaza sus
* valores con {@code ruta}, el contenido se desplegará en una
* sola página. Los tags y atributos de HTML a evaluar son los
* mismos que para el método
* {@code parseHTML(String, String, int)}.
*
* @param datos the string with the HTML code to parse.
* @param ruta the string with the new path to write to {@code datos}.
* @return a string with the HTML code with relative paths modified.
*/
public static String parseHTML(String datos, String ruta) {
return parseHTML(datos, ruta, 0);
}
/**
* Looks for relative paths in the attributes of HTML tags and replaces
* their values with {@code ruta}, indicating the number of
* {@code pages} into which the content is divided. The HTML tags it
* evaluates are: {@code img}, {@code applet}, {@code script},
* {@code table},
*
* @param datos the string with the HTML code to parse.
* @param ruta the string with the new path to write to {@code datos}.
* @param pages the number of pages which the content is divided into.
* @return a string with the HTML code with relative paths modified.
* {@code tr}, {@code td}, {@code body}, {@code form}, {@code input},
* {@code a}, {@code area} {@code meta}, {@code bca}, {@code link},
* {@code param}, {@code embed}, {@code iframe} and {@code frame} and
* the attributes it evaluates are: {@code src}, {@code href},
* {@code background}, {@code codebase}, {@code value},
* {@code onmouseover}, {@code onload}, {@code onmouseout} and
* {@code onclick}.
*
* Busca rutas de archivo relativas en los atributos de tags de HTML y
* remplaza sus valores con la recibida en {@code ruta}, indicando el
* número de páginas (con {@code pages}) en que se divide
* el contenido. Los tags de HTML a evaluar son: {@code img},
* {@code applet}, {@code script}, {@code table}, {@code tr},
* {@code td}, {@code body}, {@code form}, {@code input}, {@code a},
* {@code area}, {@code meta}, {@code bca}, {@code link}, {@code param},
* {@code embed}, {@code iframe} y {@code frame}; los atributos que
* evalúa son: {@code src}, {@code href}, {@code background},
* {@code codebase}, {@code value}, {@code onmouseover}, {@code onload},
* {@code onmouseout} y {@code onclick}
* @author Jorge Jiménez
*/
public static String parseHTML(String datos, String ruta, int pages) {
hAnchors = new HashMap();
//detección de si el contenido es de word
boolean iswordcontent = false;
int posword = -1;
posword = datos.toLowerCase().indexOf("name=generator content=\"microsoft word");
if (posword > -1) {
iswordcontent = true;
} else {
posword = datos.toLowerCase().indexOf("name=\"generator\" content=\"microsoft word");
if (posword > -1) {
iswordcontent = true;
}
}
//termina detección de si es contenido de word
HtmlTag tag = new HtmlTag();
int pos = -1;
//int pos1 = -1; // no usada
StringBuilder ret = new StringBuilder();
try {
HtmlStreamTokenizer tok = new HtmlStreamTokenizer(new ByteArrayInputStream(datos.getBytes()));
while (tok.nextToken() != HtmlStreamTokenizer.TT_EOF) {
int ttype = tok.getTokenType();
if (ttype == HtmlStreamTokenizer.TT_TAG || ttype == HtmlStreamTokenizer.TT_COMMENT) {
if (ttype == HtmlStreamTokenizer.TT_COMMENT && tok.getRawString().equals("")) {
continue;
}
tok.parseTag(tok.getStringValue(), tag);
if (tok.getRawString().toLowerCase().startsWith("\r\n");
ttype = tok.nextToken();
while (!(ttype == HtmlStreamTokenizer.TT_TAG || ttype == HtmlStreamTokenizer.TT_COMMENT)) {
ttype = tok.nextToken();
}
if (ttype == HtmlStreamTokenizer.TT_TAG || ttype == HtmlStreamTokenizer.TT_COMMENT) {
if (ttype == HtmlStreamTokenizer.TT_COMMENT && tok.getRawString().equals("")) {
continue;
}
}
tok.parseTag(tok.getStringValue(), tag);
}
}
if ((tag.getTagString().toLowerCase().equals("img") || tag.getTagString().toLowerCase().equals("applet") || tag.getTagString().toLowerCase().equals("script") || tag.getTagString().toLowerCase().equals("tr") || tag.getTagString().toLowerCase().equals("td") || tag.getTagString().toLowerCase().equals("table") || tag.getTagString().toLowerCase().equals("body") || tag.getTagString().toLowerCase().equals("input") || tag.getTagString().toLowerCase().equals("a") || tag.getTagString().toLowerCase().equals("form") || tag.getTagString().toLowerCase().equals("area") || tag.getTagString().toLowerCase().equals("meta") || tag.getTagString().toLowerCase().equals("bca") || tag.getTagString().toLowerCase().equals("link") || tag.getTagString().toLowerCase().equals("param") || tag.getTagString().toLowerCase().equals("embed") || tag.getTagString().toLowerCase().equals("iframe") || tag.getTagString().toLowerCase().equals("frame")) && !tok.getRawString().startsWith("")) {
continue;
}
tok.parseTag(tok.getStringValue(), tag);
if (tok.getRawString().toLowerCase().startsWith("