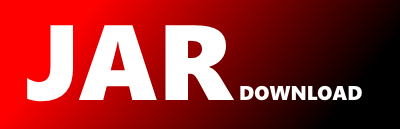
org.semanticwb.portal.resources.AdvancedSearch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SWBPortal Show documentation
Show all versions of SWBPortal Show documentation
SemanticWebBuilder Portal API components and utilities
The newest version!
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import org.semanticwb.portal.api.GenericAdmResource;
// TODO: Auto-generated Javadoc
/**
* The Class AdvancedSearch.
*
* @author Hasdai Pacheco {haxdai(at)gmail.com}
*/
public class AdvancedSearch extends GenericAdmResource {
//
// /** The log. */
// private static Logger log = SWBUtils.getLogger(AdvancedSearch.class);
//
// /** The lang. */
// private String lang = "x-x";
//
// /** The lex. */
// private SWBDictionary lex;
//
// /** The tr. */
// private SWBSparqlTranslator tr;
//
// /** The so_lat. */
// private SemanticProperty so_lat = SWBPlatform.getSemanticMgr().getVocabulary().getSemanticProperty("http://www.semanticwebbuilder.org/emexcatalog.owl#latitude");
//
// /** The so_long. */
// private SemanticProperty so_long = SWBPlatform.getSemanticMgr().getVocabulary().getSemanticProperty("http://www.semanticwebbuilder.org/emexcatalog.owl#longitude");
//
// /** The org. */
// private SemanticClass org = SWBPlatform.getSemanticMgr().getVocabulary().getSemanticClass("http://www.semanticwebbuilder.org/emexcatalog.owl#Organisation");
//
// /** The solutions. */
// private ArrayList solutions = null;
//
// /** The query string. */
// private String queryString = "";
//
// /** The dym. */
// private String dym = "";
//
// /** The index. */
// private IndexLARQ index;
//
// /** The smodel. */
// private Model smodel;
//
// /**
// * Instantiates a new advanced search.
// */
// public AdvancedSearch() {
// }
//
// /* (non-Javadoc)
// * @see org.semanticwb.portal.api.GenericAdmResource#setResourceBase(org.semanticwb.model.Resource)
// */
// @Override
// public void setResourceBase(Resource base) throws SWBResourceException {
// super.setResourceBase(base);
// try {
// smodel = SWBPlatform.getSemanticMgr().getOntology().getRDFOntModel();
// index = buildIndex();
// }catch (Exception e) {
// log.error(e);
// }
// }
//
// /* (non-Javadoc)
// * @see org.semanticwb.portal.api.GenericResource#processRequest(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
// */
// @Override
// public void processRequest(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// String mode = paramRequest.getMode();
// if (mode.equals("SUGGEST")) {
// doSuggest(request, response, paramRequest);
// } else if (mode.equals("PAGE")) {
// doShowPage(request, response, paramRequest);
// } else {
// super.processRequest(request, response, paramRequest);
// }
// }
//
// /**
// * Execute a query and gather the results. A natural language query is done
// * in first place. If there is no results a second query (using lucene) is
// * executed.
// *
// * @param query the query
// * @return the results
// */
// public ArrayList getResults(String query) {
// ArrayList res = new ArrayList();
//
// //Assert query string
// query = (query == null ? "" : query.trim());
//
// //Get google maps API key
// String mapKey = getResourceBase().getAttribute("mapKey");
// mapKey = (mapKey == null ? "" : mapKey);
//
// //Create SparQl Translator
// tr = new SWBSparqlTranslator(lex);
//
// //Translate query to SparQl
// String sparqlQuery = lex.getLexicon(lang).getPrefixString() + "\n" + tr.translateSentence(query, true);
// //dym = tr.didYouMean(query);
// //dym = (dym.equalsIgnoreCase(query) ? "" : dym);
//
// //System.out.println(sparqlQuery);
//
// //If no errors and query is not empty, show results
// if (tr.getErrCode() == 0 && !tr.isEmptyQuery()) {
//
// //Get model to query
// SemanticModel model = new SemanticModel("model", SWBPlatform.getSemanticMgr().getOntology().getRDFOntModel());
//
// //Execute select query
// QueryExecution qexec = model.sparQLQuery(sparqlQuery);
//
// //Get results of query as a result set
// ResultSet rs = qexec.execSelect();
//
// //If there are results
// if (rs != null && rs.hasNext()) {
//
// //Get next result set
// while (rs.hasNext()) {
// String mapbox = "";
// boolean requiresMap = false;
// boolean first = true;
//
// //Get next solution of the result set (var set)
// QuerySolution qs = rs.nextSolution();
// StringBuffer segment = new StringBuffer();
// segment.append("");
//
// //For each variable
// for (String vName : (List) rs.getResultVars()) {
// //Get node
// RDFNode x = qs.get(vName);
//
// //If node is not null
// if (x != null) {
// //If node is the first in the solution (there is always a subject),
// //display node in bold
// if (first) {
// //Get SemanticObject of current node
// SemanticObject so = SemanticObject.createSemanticObject(x.toString());
// if (so != null) {
// //Get url for viewing the object detail
// String dUrl = getResourceBase().getAttribute("detailUrl") + "?uri=" + URLEncoder.encode(so.getURI());
// //If object is an organization
// //TODO: compare to GeoTaggable instead of org
// if (so.instanceOf(org)) {
// //Get url for viewing maps
// String r = getResourceBase().getAttribute("mapUrl");
// if (r == null) {
// r = "#";
// }
//
// //Build map url
// String mapUrl = r.replace(" ", "%20") +
// "?lat=" + so.getProperty(so_lat) + "&long=" + so.getProperty(so_long);
//
// //Create static minimap
// requiresMap = true;
// mapbox = mapbox + "
";
//
// //Add detail link in object title
// segment.append("" + "" +
// so.getDisplayName(lang) + "(" + so.getSemanticClass().getDisplayName(lang) + ")
");
// } else { //Not an organization
// segment.append("" + "" +
// so.getDisplayName(lang) + "
");
// }
// //If only one object requested, build object abstract
// if (rs.getResultVars().size() == 1) {
// segment.append(buildAbstract(so));
// }
// } else {
// segment.append("" +
// lex.getLexicon(lang).getWord(vName, true).getLemma());
// //lex.getObjWordTag(vName).getDisplayName() + "" + "
");
// }
// first = false;
// } else { //Not a semantic object
// //If node is a literal, display a name, value pair
// if (x.isLiteral()) {
// segment.append("" +
// vName + ":" + x.asNode().getLiteral().getLexicalForm() + "" + "
");
// }
// }
// } else { //If empty node
// segment.append("" + vName + ": " + "-
");
// }
// }
// segment.append("
");
// if (requiresMap) {
// segment.append(" " + mapbox + " ");
// }
// segment.append(" ");
// res.add(segment.toString());
// }
// qexec.close();
// } else { //If no results in natural language query, try open-text search
// res = getOpenTextResults(query);
// }
// } else { //If natural language query could not be processed, try open-text search
// res = getOpenTextResults(query);
// }
// return res;
// }
//
// /* (non-Javadoc)
// * @see org.semanticwb.portal.api.GenericAdmResource#doView(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
// */
// @Override
// public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// SWBResourceURL rUrl = paramRequest.getRenderUrl();
// User user = paramRequest.getUser();
// StringBuffer sbf = new StringBuffer();
//
// //Get user language if any
// if (user != null) {
// if (!lang.equals(user.getLanguage())) {
// lang = user.getLanguage();
// SWBLocaleLexicon l = new SWBLocaleLexicon(lang, SWBDictionary.getLanguageName(lang));
// lex.addLexicon(l);
// lex.setLocale(lang);
// }
// } else {
// if (!lang.equals("es")) {
// lang = "es";
// }
// }
//
// //Create lexicon for NLP
// String pf = getResourceBase().getAttribute("prefixFilter");
// if (pf == null) {
// pf = "";
// }
//
// if (paramRequest.getCallMethod() == paramRequest.Call_STRATEGY) {
// //Set URL call method to call_DIRECT to make an AJAX call
// rUrl.setCallMethod(rUrl.Call_DIRECT);
// rUrl.setMode("SUGGEST");
//
// //Add necesary scripting
// sbf.append("\n" +
// "\n\n");
//
// /**
// * Clears the suggestions list and gives focus to the textarea.
// */
// sbf.append("");
//
// String url = "";
// Resourceable rsa = getResourceBase().getResourceable();
// if (rsa != null && rsa instanceof WebPage) {
// url = ((WebPage) rsa).getUrl();
// }
// sbf.append(" " +
// " \n");
// response.getWriter().print(sbf.toString());
// } else {
// doShowResults(request, response, paramRequest);
// }
// }
//
// /**
// * Do suggest.
// *
// * @param request the request
// * @param response the response
// * @param paramRequest the param request
// * @throws SWBResourceException the sWB resource exception
// * @throws IOException Signals that an I/O exception has occurred.
// */
// public void doSuggest(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// PrintWriter out = response.getWriter();
// StringBuffer sbf = new StringBuffer();
// SortedSet objOptions = new TreeSet();
// SortedSet proOptions = new TreeSet();
// String word = request.getParameter("word");
// boolean props = Boolean.parseBoolean(request.getParameter("props"));
// boolean lPar = false;
// boolean rPar = false;
// int idCounter = 0;
//
// word = URLDecoder.decode(word, "iso-8859-1");
//
// response.setContentType("text/html; charset=iso-8859-1");
// response.setHeader("Cache-control", "no-cache");
// response.setHeader("Pragma", "no-cache");
//
// if (word.indexOf("(") != -1) {
// lPar = true;
// word = word.replace("(", "");
// }
// if (word.indexOf(")") != -1) {
// rPar = true;
// word = word.replace(")", "");
// }
//
// word = word.replace("[", "");
// word = word.replace("]", "");
// word = word.trim();
//
// //Create prefixs list
// ArrayList preflist = null;
//
// //If asking for class name
// if (!props) {
// //Assert prefixes list
// String pf = getResourceBase().getAttribute("prefixFilter");
// if (pf == null) {
// pf = "";
// }
//
// if (!pf.trim().equals("")) {
// preflist = new ArrayList(Arrays.asList(pf.split(",")));
// }
//
// //Filter semantic classes
// Iterator cit = SWBPlatform.getSemanticMgr().getVocabulary().listSemanticClasses();
// while (cit.hasNext()) {
// SemanticClass sc = cit.next();
// String tempc = sc.getDisplayName(lang);
// if (tempc.toLowerCase().indexOf(word.toLowerCase()) != -1) {
// objOptions.add(tempc);
// }
// }
//
// //Add all properties
// Iterator sit = lex.getLexicon(lang).listPropertyWords();
// while (sit.hasNext()) {
// Word tempp = sit.next();
// if (tempp.getLexicalForm().toLowerCase().indexOf(word.toLowerCase()) != -1) {
// proOptions.add(tempp);
// }
// }
//
// //If there are suggestions
// if (proOptions.size() != 0 || objOptions.size() != 0) {
// idCounter = 0;
// int index;
// Iterator rit = objOptions.iterator();
//
// sbf.append("");
// while (rit.hasNext()) {
// String tempi = (String) rit.next();
// index = tempi.toLowerCase().indexOf(word.toLowerCase());
//
// sbf.append("- " + (lPar ? "(" : "") +
// "" + tempi + "" +
// (lPar ? ")" : "") + "
");
// idCounter++;
// }
//
// rit = proOptions.iterator();
// while (rit.hasNext()) {
// String tempi = (String) rit.next();
// index = tempi.toLowerCase().indexOf(word.toLowerCase());
//
// sbf.append("- " + (lPar ? "(" : "") +
// "" + tempi + "" +
// (lPar ? ")" : "") + "
");
// idCounter++;
// }
// sbf.append("
");
// } else {
// sbf.append("" + paramRequest.getLocaleString("msgNoSuggestions") + "");
// }
// } else {
// //System.out.println("Suggesting for " + word);
// String tag = lex.getLexicon(lang).getWord(word, true).getTags().get(0).getTagInfoParam(SWBLocaleLexicon.PARAM_ID);
// //String tag = lex.getObjWordTag(word).getObjId();
//
// if (!tag.equals("")) {
// sbf.append("");
// SemanticClass sc = SWBPlatform.getSemanticMgr().getVocabulary().getSemanticClassById(tag);
// idCounter = 0;
// Iterator sit = sc.listProperties();
// while (sit.hasNext()) {
// SemanticProperty t = sit.next();
// sbf.append("- " + (lPar ? "(" : "") +
// "" + t.getDisplayName(lang) + "" +
// (lPar ? ")" : "") + "
");
// idCounter++;
// }
// sbf.append("
");
// } else {
// tag = lex.getLexicon(lang).getWord(word, false).getSelectedTag().getTagInfoParam(SWBLocaleLexicon.PARAM_URI);
// //tag = lex.getPropWordTag(word).getRangeClassId();
// if (!tag.equals("")) {
// sbf.append("");
// SemanticClass sc = SWBPlatform.getSemanticMgr().getVocabulary().getSemanticClassById(tag);
// idCounter = 0;
// Iterator sit = sc.listProperties();
// while (sit.hasNext()) {
// SemanticProperty t = sit.next();
// sbf.append("- " + (lPar ? "(" : "") +
// "" + t.getDisplayName(lang) + "" +
// (lPar ? ")" : "") + "
");
// idCounter++;
// }
// sbf.append("
");
// } else {
// sbf.append("" + paramRequest.getLocaleString("msgNoSuggestions") + "");
// }
// }
// }
// out.println(sbf.toString());
// }
//
// /**
// * Do show page.
// *
// * @param request the request
// * @param response the response
// * @param paramRequest the param request
// * @throws SWBResourceException the sWB resource exception
// * @throws IOException Signals that an I/O exception has occurred.
// */
// public void doShowPage(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// SWBResourceURL rUrl = paramRequest.getRenderUrl().setMode("PAGE");
// PrintWriter out = response.getWriter();
// StringBuffer sbf = new StringBuffer();
//
// if (paramRequest.getCallMethod() == paramRequest.Call_CONTENT) {
// sbf.append("");
//
// sbf.append(" \n" +
// " \n" +
// " " +
// paramRequest.getLocaleString("msgResults") +
// " " + queryString + "
\n");
//
//
// if (solutions != null && solutions.size() > 0) {
// int step = 10;
// String page = request.getParameter("p");
// int _start = 0;
// int _end = 0;
//
// if (page == null) {
// page = "1";
// }
//
// _start = step * (Integer.valueOf(page) - 1);
// _end = _start + step - 1;
// if (_end > solutions.size() - 1) {
// _end = solutions.size() - 1;
// }
//
// //System.out.println("page: " + page + ", start: " + _start + ", end: " + _end);
//
// sbf.append("Mostrando resultados " + (_start + 1) + " - " + (_end + 1) + " de " + solutions.size() + "
" +
// "
\n");
//
// for (int i = _start; i <= _end; i++) {
// sbf.append(solutions.get(i));
// }
//
// sbf.append("
\n");
// double pages = Math.ceil((double) solutions.size() / (double) step);
// for (int i = 1; i <= pages; i++) {
// _start = step * (i - 1);
// if ((_start + step) - 1 > solutions.size() - 1) {
// _end = solutions.size() - 1;
// } else {
// _end = (_start + step) - 1;
// }
// if (Integer.valueOf(page) == i) {
// sbf.append("" + i + " ");
// } else {
// rUrl = paramRequest.getRenderUrl().setMode("PAGE");
// rUrl.setParameter("p", String.valueOf(i));
// sbf.append("" + i + " ");
// }
// }
//
// if (Integer.valueOf(page) < pages) {
// rUrl = paramRequest.getRenderUrl().setMode("PAGE");
// rUrl.setParameter("p", String.valueOf(Integer.valueOf(page) + 1));
// sbf.append("" + paramRequest.getLocaleString("lblNext") + "\n");
// }
// sbf.append(" ");
// } else {
// sbf.append("
\n");
// sbf.append("" +
// paramRequest.getLocaleString("msgNoResults") + "
" +
// (dym.equals("") ? "" : "" +
// paramRequest.getLocaleString("msgDidYouMean") + " " + dym + "
" +
// "
\n"));
// }
// sbf.append("
\n" +
// "
\n");
// out.println(sbf.toString());
// } else {
// doView(request, response, paramRequest);
// }
// }
//
// /**
// * Do show results.
// *
// * @param request the request
// * @param response the response
// * @param paramRequest the param request
// * @throws SWBResourceException the sWB resource exception
// * @throws IOException Signals that an I/O exception has occurred.
// */
// public void doShowResults(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// queryString = request.getParameter("q");
//
// //Assert query string
// queryString = (queryString == null ? "" : queryString.trim());
//
// if (!queryString.equals("")) {
// solutions = getResults(queryString);
// doShowPage(request, response, paramRequest);
// }
// }
//
// /**
// * Gathers information of a Semantic Object.
// *
// * @param o Semantic Object to gather information from.
// * @return the string
// */
// public String buildAbstract(SemanticObject o) {
// SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
// SimpleDateFormat timeFormat = new SimpleDateFormat("HH:mm:ss");
// StringBuffer res = new StringBuffer();
// HashMap properties = new HashMap();
// //Get list of object properties
// Iterator pit = o.listProperties();
// while (pit.hasNext()) {
// //Get next property
// SemanticProperty sp = pit.next();
// //Do not display rdf and owl properties
// if (!sp.isObjectProperty() && (!sp.getPrefix().equals("rdf") && !sp.getPrefix().equals("owl") && !sp.getPrefix().equals("rdfs"))) {
// //Get property value, if any, and display it
// if (sp != null) {
// String val = "";
//
// /*if (sp.isDate()) {
// val = dateFormat.format(SWBUtils.TEXT.iso8601DateFormat(o.getDateProperty(sp)));
// } else if (sp.isDateTime()) {
// System.out.println("......its a datetime");
// Timestamp dt = o.getDateTimeProperty(sp);
// if (dt != null) {
// val = timeFormat.format(dt);
// }
// } else {*/
// val = o.getProperty(sp);
// //}
// if (val != null && !val.equals("")) {
//
// properties.put(sp.getDisplayName(lang).toUpperCase(), o.getProperty(sp));
// }
// }
// } else if (sp.isObjectProperty()) {
// SemanticObject rg = o.getObjectProperty(sp);
// if (rg != null) {
// properties.put(sp.getDisplayName(lang).toUpperCase(), rg.getDisplayName(lang));
// }
// }
// }
// Map sorted = new TreeMap(properties);
// Set names = sorted.keySet();
// Iterator it = names.iterator();
// while (it.hasNext()) {
// String name = it.next();
// String value = (String)sorted.get(name);
// res.append("" +
// name + ": " + value + "" + "
");
// }
// return res.toString();
// }
//
// /**
// * Builds an index to perform searchs.
// *
// * @return the index larq
// */
// public IndexLARQ buildIndex()
// {
// // ---- Read and index all literal strings.
// IndexBuilderString larqBuilder = new IndexBuilderString() ;
//
// // ---- Alternatively build the index after the model has been created.
// larqBuilder.indexStatements(smodel.listStatements()) ;
//
// // ---- Finish indexing
// larqBuilder.closeWriter() ;
//
// // ---- Create the access index
// index = larqBuilder.getIndex() ;
// return index ;
// }
//
// /**
// * Gets the open text results.
// *
// * @param q the q
// * @return the open text results
// */
// public ArrayList getOpenTextResults(String q) {
// ArrayList res = new ArrayList();
//
// //Assert query string
// q = (q == null ? "" : q.trim());
//
// String mapKey = getResourceBase().getAttribute("mapKey");
// mapKey = (mapKey == null ? "" : mapKey);
//
// //Build query to return classes with literal values in their properties
// String queryString = "PREFIX xsd: \n" +
// "PREFIX pf: \n" +
// "PREFIX swb: \n" +
// "PREFIX rdf: \n" +
// "PREFIX owl: \n" +
// "SELECT ?obj ?prop ?lit {\n" +
// " ?lit pf:textMatch '" + q + "'.\n" +
// " ?obj ?prop ?lit.\n" +
// " ?obj rdf:type ?type.\n" +
// " ?type rdf:type swb:Class\n" +
// "}\n";
//
// // Make globally available
// LARQ.setDefaultIndex(index);
// Query query = QueryFactory.create(queryString);
//
// QueryExecution qExec = QueryExecutionFactory.create(query, smodel);
// ResultSet rs = qExec.execSelect();
// //ResultSetFormatter.out(System.out, rs, query);
//
// //If there are results
// if (rs != null && rs.hasNext()) {
// //Get next result set
// while (rs.hasNext()) {
// String mapbox = "";
// boolean requiresMap = false;
//
// //Get next solution of the result set (var set)
// QuerySolution qs = rs.nextSolution();
// StringBuffer segment = new StringBuffer();
// segment.append("");
//
// //Get node object from solution
// RDFNode x = qs.get("obj");
//
// //If node is not null
// if (x != null) {
// //Get SemanticObject of current node
// SemanticObject so = SemanticObject.createSemanticObject(x.toString());
// if (so != null) {
// String dUrl = getResourceBase().getAttribute("detailUrl") + "?uri=" + URLEncoder.encode(so.getURI());
// //If object is an organization
// //TODO: compare to GeoTaggable instead of org
// if (so.instanceOf(org)) {
// String r = getResourceBase().getAttribute("mapUrl");
// if (r == null) {
// r = "#";
// }
//
// String mapUrl = r.replace(" ", "%20") +
// "?lat=" + so.getProperty(so_lat) + "&long=" + so.getProperty(so_long);
//
// requiresMap = true;
// mapbox = mapbox + "
";
//
// segment.append("" + "" +
// so.getDisplayName(lang) + "(" + so.getSemanticClass().getDisplayName(lang) + ")
");
// if (rs.getResultVars().size() == 1) {
// segment.append(buildAbstract(so));
// }
// } else { //Not an organization
// segment.append("" + "" +
// so.getDisplayName(lang) + "
");
// }
// //Append object abstract
// segment.append(buildAbstract(so));
// }
// }
// segment.append("
");
// if (requiresMap) {
// segment.append(" " + mapbox + " ");
// }
// segment.append(" ");
// res.add(segment.toString());
// }
// }
// qExec.close();
// return res;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy