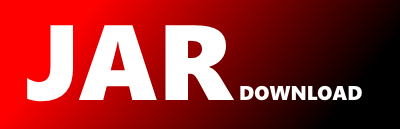
org.semanticwb.portal.resources.Comment Maven / Gradle / Ivy
Show all versions of SWBPortal Show documentation
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import java.io.File;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.StringTokenizer;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Date;
import java.util.Enumeration;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.semanticwb.Logger;
import org.semanticwb.SWBUtils;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBPlatform;
import org.semanticwb.model.User;
import org.semanticwb.model.WebPage;
import org.semanticwb.model.Resource;
import org.semanticwb.model.Resourceable;
import org.semanticwb.portal.util.FileUpload;
import org.semanticwb.portal.api.SWBParamRequest;
import org.semanticwb.portal.api.GenericResource;
import org.semanticwb.portal.api.SWBResourceURL;
import org.semanticwb.portal.api.SWBResourceException;
import org.semanticwb.portal.admin.admresources.util.WBAdmResourceUtils;
import javax.mail.internet.InternetAddress;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.transform.TransformerException;
import org.semanticwb.portal.api.SWBActionResponse;
// TODO: Auto-generated Javadoc
/**
* Presenta la interfaz para el envío de comentarios al sitio en la categoría
* seleccionada por el usuario. Este recurso cuenta con facilidades de administración
* que permiten indicar categorías de comentarios y destinatarios a cada categoría,
* así como el estilo del mecanismo que presenta la interfaz de captura del comentario.
*
* Displays the interface needed to send comments of a selected category. This resource's
* administration screen allows the creation of comment categories and a recipient for each
* category; likewise it allows the selection of characteristics to present the link to the
* capture interface
*
* @author : Vanessa Arredondo Núñez
* @version 1.0
*/
public class Comment extends GenericResource {
/** The log. */
private static Logger log = SWBUtils.getLogger(Comment.class);
/** The web work path. */
private static String webWorkPath;
/** The path. */
private static String path;
/** The Constant _FAIL. */
private static final String _FAIL = "failure";
/**
* Contiene la definición del layout para la interfaz de captura de comentarios.
* Contains the layout definition for the commentaries capture interface.
*/
private javax.xml.transform.Templates tpl = null;
/**
* Creates a new instance of Comment.
*/
public Comment() {
}
/**
* Fija la plantilla del layout para la interfaz de captura de comentarios y
* la mantiene en memoria.Sets this resource layout template for the commentaries
* capture interface and keeps it in memory.
* @param base el objeto base para la creación de este recurso.
* the base object for this resource's creation.
*/
@Override
public void setResourceBase(Resource base) {
String name = getClass().getName().substring(getClass().getName().lastIndexOf(".") + 1);
try {
super.setResourceBase(base);
webWorkPath = SWBPortal.getWebWorkPath()+base.getWorkPath() + "/";
path = SWBPlatform.getContextPath() + "/swbadmin/xsl/" + name + "/";
} catch (Exception e) {
log.error("Error while setting resource base: " + base.getId() + "-" + base.getTitle(), e);
}
if( !"".equals(base.getAttribute("template", "").trim()) ) {
try {
tpl = SWBUtils.XML.loadTemplateXSLT( SWBPortal.getFileFromWorkPath(base.getWorkPath()+"/"+base.getAttribute("template").trim()) );
path = webWorkPath + "/";
} catch (Exception e) {
log.error("Error while loading resource template: " + base.getId(), e);
}
}
if( tpl==null ) {
try {
tpl = SWBUtils.XML.loadTemplateXSLT( SWBPortal.getAdminFileStream("/swbadmin/xsl/" + name + "/" + name + ".xsl"));
} catch (Exception e) {
log.error("Error while loading default resource template: " + base.getId(), e);
}
}
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#processAction(HttpServletRequest, SWBActionResponse)
*/
@Override
public void processAction(HttpServletRequest request, SWBActionResponse response) throws SWBResourceException {
Resource base = getResourceBase();
String action = response.getAction();
if(SWBActionResponse.Action_ADD.equals(action)){
boolean hasCaptcha = Boolean.parseBoolean(base.getAttribute("captcha"));
String securCodeSent = request.getParameter("cmnt_seccode");
String securCodeCreated = (String)request.getSession(true).getAttribute("cs");
if( (hasCaptcha && securCodeCreated!=null && securCodeCreated.equalsIgnoreCase(securCodeSent)) || !hasCaptcha ) {
try {
processEmails(request, response);
try {
feedCommentLog(request, response);
}catch (IOException ioe) {
log.error("Error in resource Comment, while trying to log the action. ", ioe);
}
response.setMode(SWBActionResponse.Mode_HELP);
}catch(TransformerException te) {
log.error("Error in resource Comment, while trying to send the email. ", te);
response.setRenderParameter(_FAIL, te.getMessage());
}catch(SWBResourceException re) {
log.error("Error in resource Comment, while trying to send the email. ", re);
response.setRenderParameter(_FAIL, re.getMessage());
}catch(Exception e) {
log.error("Error in resource Comment, while trying to send the email. ", e);
response.setRenderParameter(_FAIL, e.getMessage());
}
}else {
Enumeration e = request.getParameterNames();
while(e.hasMoreElements()){
String key = (String)e.nextElement();
response.setRenderParameter(key, request.getParameter(key));
}
}
}
}
/**
* Obtiene los datos de la configuración del recurso, sin tomar en
* cuenta aquellos para el envío del correo y genera un objeto
* Document
con ellos. Gets this resource's configuration data (whithout
* those to send e-mails) and generates a Document
object with it
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param paramRequest the param request
* @return estructura de la interfaz de captura de comentarios.
* @throws SWBResourceException si no existe el archivo de mensajes del idioma utilizado.
* if there is no file message of the corresponding language.
* @throws IOException Signals that an I/O exception has occurred.
*/
public Document getDom(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
// String action = ((null != request.getParameter("com_act")) && (!"".equals(request.getParameter("com_act").trim()))
// ? request.getParameter("com_act").trim()
// : "com_step2");
Resource base = getResourceBase();
try {
Document dom = SWBUtils.XML.getNewDocument();
// if ("com_step3".equals(action)) {
// dom = getDomEmail(request, response, paramRequest); // Envia correo
// }else {
// Nueva ventana con formulario
User user = paramRequest.getUser();
// SWBResourceURLImp url = new SWBResourceURLImp(request, base,
// paramRequest.getWebPage(), SWBResourceURL.UrlType_RENDER);
// url.setResourceBase(base);
// url.setMode(SWBResourceURLImp.Mode_VIEW);
// /*url.setWindowState(SWBResourceURLImp.WinState_MAXIMIZED);*/
// url.setParameter("com_act", "com_step3");
// url.setTopic(paramRequest.getWebPage());
// /*url.setCallMethod(reqParams.Call_DIRECT);*/
SWBResourceURL url = paramRequest.getActionUrl().setAction(paramRequest.Action_ADD);
Element root = dom.createElement("form");
root.setAttribute("path", SWBPlatform.getContextPath() + "/swbadmin/css/");
root.setAttribute("accion", url.toString());
root.setAttribute("styleClass", base.getAttribute("styleClass", "").equals("") ? "" : "");
root.setAttribute("styleClassClose", "");
dom.appendChild(root);
Element emn = null;
emn = dom.createElement("msgComments");
emn.appendChild(dom.createTextNode(paramRequest.getLocaleString("msgComments")));
root.appendChild(emn);
if (!"".equals(base.getAttribute("comentario", "").trim())) {
emn = dom.createElement("fselect");
emn.setAttribute("tag",
paramRequest.getLocaleString("msgViewTypeComment"));
emn.setAttribute("inname", "selTipo");
if (base.getDom() != null) {
NodeList node = base.getDom().getElementsByTagName(
"comentario");
String comentario = "";
for (int i = 0; i < node.getLength(); i++) {
comentario = node.item(i).getChildNodes().item(
0).getNodeValue().trim();
if (!"".equals(comentario)) {
Element child = dom.createElement("foption");
child.setAttribute("invalue", comentario);
emn.appendChild(child);
}
}
}
root.appendChild(emn);
}
if (user.isSigned()) {
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgFirstName"));
emn.setAttribute("inname", "txtFromName");
String strFromName = ("1".equals(
base.getAttribute("firstname", "0").trim())
&& (null != user.getFirstName()
&& !"".equals(user.getFirstName().trim()))
? user.getFirstName().trim()
: "");
strFromName += ("1".equals(
base.getAttribute("lastname", "0").trim())
&& (null != user.getLastName()
&& !"".equals(user.getLastName().trim()))
? " " + user.getLastName().trim()
: "");
strFromName += ("1".equals(
base.getAttribute("middlename", "0").trim())
&& (null != user.getSecondLastName()
&& !"".equals(user.getSecondLastName().trim()))
? " " + user.getSecondLastName().trim()
: "");
emn.setAttribute("invalue", strFromName);
root.appendChild(emn);
}else {
if("1".equals(base.getAttribute("firstname", "0"))) {
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgFirstName"));
emn.setAttribute("inname", "txtFromName");
root.appendChild(emn);
}
if("1".equals(base.getAttribute("lastname", "0"))) {
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgLastName"));
emn.setAttribute("inname", "txtFromLName");
root.appendChild(emn);
}
if("1".equals(base.getAttribute("middlename", "0"))) {
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgMiddleName"));
emn.setAttribute("inname", "txtFromSLName");
root.appendChild(emn);
}
}
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgViewEmail"));
emn.setAttribute("inname", "txtFromEmail");
if (user.isSigned()) {
String strFromEmail = (null != user.getEmail()
&& !"".equals(user.getEmail().trim())
? user.getEmail().trim()
: "");
emn.setAttribute("invalue", strFromEmail);
}
root.appendChild(emn);
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgPhone"));
emn.setAttribute("inname", "txtPhone");
root.appendChild(emn);
emn = dom.createElement("ftext");
emn.setAttribute("tag", paramRequest.getLocaleString("msgFax"));
emn.setAttribute("inname", "txtFax");
root.appendChild(emn);
emn = dom.createElement("ftextarea");
emn.setAttribute("tag", paramRequest.getLocaleString("msgMessage"));
emn.setAttribute("inname", "tarMsg");
root.appendChild(emn);
emn = dom.createElement("fsubmit");
if (!"".equals(base.getAttribute("imgenviar", "").trim())) {
emn.setAttribute("img", "1");
emn.setAttribute("src", webWorkPath + base.getAttribute("imgenviar").trim());
if (!"".equals(base.getAttribute("altenviar", "").trim()))
emn.setAttribute("alt",
base.getAttribute("altenviar").trim());
else emn.setAttribute("alt",
paramRequest.getLocaleString("msgViewSubmitAlt"));
} else {
emn.setAttribute("img", "0");
if (!"".equals(base.getAttribute("btnenviar", "").trim())) {
emn.setAttribute("tag",
base.getAttribute("btnenviar").trim());
} else {
emn.setAttribute("tag",
paramRequest.getLocaleString("msgViewSubmitButton"));
}
}
root.appendChild(emn);
emn = dom.createElement("freset");
if (!"".equals(base.getAttribute("imglimpiar", "").trim())) {
emn.setAttribute("img", "1");
emn.setAttribute("src", webWorkPath + base.getAttribute("imglimpiar").trim());
if (!"".equals(base.getAttribute("altlimpiar", "").trim())) {
emn.setAttribute("alt",
base.getAttribute("altlimpiar").trim());
} else {
emn.setAttribute("alt",
paramRequest.getLocaleString("msgViewResetAlt"));
}
} else {
emn.setAttribute("img", "0");
if (!"".equals(base.getAttribute("btnlimpiar", "").trim())) {
emn.setAttribute("tag",
base.getAttribute("btnlimpiar").trim());
} else {
emn.setAttribute("tag",
paramRequest.getLocaleString("msgViewResetButton"));
}
}
root.appendChild(emn);
// }
return dom;
} catch (SWBResourceException swbre) {
throw swbre;
} catch (org.semanticwb.SWBException e) {
log.error("Error while generating the comments form in resource "+base.getResourceType().getResourceClassName()+" with identifier " + base.getId()+" - "+base.getTitle(), e);
}
return null;
}
/**
* Obtiene todos los datos de la configuración del recurso y genera
* un objeto Document con ellos.Gets all the
* configuration data for this resource and generates a Document object
* with it.
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @return información de un comentario para su envío.
* @throws SWBResourceException si el nombre del remitente, su correo y mensaje
* son nulos. if sender's name, e-mail account and message is null.
*/
public Document getDomEmail(HttpServletRequest request, SWBActionResponse response) throws SWBResourceException {
Resource base = getResourceBase();
try {
String strFromName = (null != request.getParameter("txtFromName")
&& !"".equals(request.getParameter("txtFromName").trim())
? request.getParameter("txtFromName").trim()
: null);
String strFromEmail = (null != request.getParameter("txtFromEmail")
&& !"".equals(request.getParameter("txtFromEmail").trim())
? request.getParameter("txtFromEmail").trim()
: null);
String strTarMsg = (null != request.getParameter("tarMsg")
&& !"".equals(request.getParameter("tarMsg").trim())
? request.getParameter("tarMsg").trim()
: null);
if (strFromName != null && strFromEmail != null && strTarMsg != null) {
Document dom = SWBUtils.XML.getNewDocument();
String strSubject = response.getLocaleString("emlSubject");
String commentType = (null != request.getParameter("selTipo")
&& !"".equals(request.getParameter("selTipo").trim())
? request.getParameter("selTipo").trim()
: strSubject);
if (!"".equals(base.getAttribute("subject", "").trim())) {
strSubject = base.getAttribute("subject").trim();
} else if (commentType != null ) {
strSubject = commentType + "...";
}
WebPage topic = response.getWebPage();
String lang = response.getUser().getLanguage();
Element emn = dom.createElement("form");
emn.setAttribute("path", "http://" + request.getServerName()
+ (request.getServerPort() != 80
? ":" + request.getServerPort()
: "") + SWBPlatform.getContextPath() + "/swbadmin/css/");
emn.setAttribute("email", "1");
emn.setAttribute("styleClass",
base.getAttribute("styleClass", "").equals("")
? ""
: " class=\"" + base.getAttribute("styleClass", "") + "\"");
emn.setAttribute("styleClassClose", "");
dom.appendChild(emn);
addElem(dom, emn, "site", topic.getWebSiteId());
addElem(dom, emn, "topic",
topic.getTitle(lang) != null
? topic.getTitle(lang) : "Sin título");
addElem(dom, emn, "fromname", strFromName);
addElem(dom, emn, "fromemail", strFromEmail);
addElem(dom, emn, "subject", strSubject);
addElem(dom, emn, "message", strTarMsg);
addElem(dom, emn, "msgTo", response.getLocaleString("msgTo"));
addElem(dom, emn, "msgName", response.getLocaleString("msgName"));
addElem(dom, emn, "msgViewEmail",
response.getLocaleString("msgViewEmail"));
addElem(dom, emn, "msgPhone", response.getLocaleString("msgPhone"));
addElem(dom, emn, "msgFax", response.getLocaleString("msgFax"));
addElem(dom, emn, "msgMessage",
response.getLocaleString("msgMessage"));
String value = "";
String area = "";
String responsable = "";
String toemail = "";
if (commentType != null ) {
addElem(dom, emn, "commenttype", commentType);
Document dom2 = base.getDom();
NodeList node = dom2.getElementsByTagName("comentario");
for (int i = 0; i < node.getLength(); i++) {
area = "";
responsable = "";
toemail = "";
value = node.item(i).getFirstChild().getNodeValue().trim();
if (value.equals(commentType)) {
NodeList child = node.item(i).getChildNodes();
for (int j = 0; j < child.getLength(); j++) {
if (child.item(j) != null) {
if ("area".equals(child.item(j).getNodeName())) {
area = child.item(j).getFirstChild(
).getNodeValue().trim();
continue;
} else if ("responsable".equals(child.item(
j).getNodeName())) {
responsable = child.item(j).getFirstChild(
).getNodeValue().trim();
continue;
} else if ("email".equals(child.item(
j).getNodeName())) {
toemail = child.item(j).getFirstChild(
).getNodeValue().trim();
continue;
}
}
}
break;
}
}
if (!"".equals(area)) {
addElem(dom, emn, "area", area);
}
if (!"".equals(responsable)) {
addElem(dom, emn, "responsable", responsable);
}
if (!"".equals(toemail)) {
addElem(dom, emn, "toemail", toemail);
}
}
if ("".equals(toemail)) {
addElem(dom, emn, "area", base.getAttribute("area","").trim());
addElem(dom, emn, "responsable",
base.getAttribute("responsable", "").trim());
addElem(dom, emn, "toemail",
base.getAttribute("email", "").trim());
}
StringBuilder strHeadermsg = new StringBuilder(800);
strHeadermsg.append("
\n");
strHeadermsg.append("----------------------------------------------------------------------
\n");
strHeadermsg.append(response.getLocaleString("emlSite"));
strHeadermsg.append(" " + topic.getWebSiteId() + ".");
strHeadermsg.append((topic.getTitle(lang) != null
? topic.getTitle(lang) : "Sin título") + " \n
");
strHeadermsg.append(response.getLocaleString("emlCommentType"));
strHeadermsg.append(" \n");
if (commentType != null ) {
strHeadermsg.append(commentType);
} else {
strHeadermsg.append(response.getLocaleString(
"emlHeaderMessage1"));
}
strHeadermsg.append("
\n");
strHeadermsg.append("----------------------------------------------------------------------
\n");
if (!"".equals(base.getAttribute("headermsg", "").trim())) {
addElem(dom, emn, "headermsg",
base.getAttribute("headermsg").trim());
strHeadermsg.append("
"
+ base.getAttribute("headermsg").trim()
+ "
\n");
}
strHeadermsg.append("
\n");
strHeadermsg.append(" \n");
strHeadermsg.append(" \n");
strHeadermsg.append(base.getAttribute("responsable", "").trim());
strHeadermsg.append("
" + base.getAttribute("area", "").trim());
strHeadermsg.append("
\n");
strHeadermsg.append(" \n");
strHeadermsg.append(response.getLocaleString("emlName"));
strHeadermsg.append(" \n");
strHeadermsg.append(strFromName + " \n");
strHeadermsg.append("");
strHeadermsg.append(response.getLocaleString("emlEmail"));
strHeadermsg.append(" \n");
strHeadermsg.append("");
strHeadermsg.append(strFromEmail + " \n");
if (request.getParameter("txtPhone") != null
&& !"".equals(request.getParameter("txtPhone").trim())) {
addElem(dom, emn, "phone",
request.getParameter("txtPhone").trim());
strHeadermsg.append("");
strHeadermsg.append(response.getLocaleString("emlPhone"));
strHeadermsg.append(" \n");
strHeadermsg.append("");
strHeadermsg.append(request.getParameter("txtPhone").trim());
strHeadermsg.append(" \n");
}
if (request.getParameter("txtFax") != null
&& !"".equals(request.getParameter("txtFax").trim())) {
addElem(dom, emn, "fax", request.getParameter("txtFax").trim());
strHeadermsg.append("");
strHeadermsg.append(response.getLocaleString("emlFax"));
strHeadermsg.append(" \n");
strHeadermsg.append("");
strHeadermsg.append(request.getParameter("txtFax").trim());
strHeadermsg.append(" \n");
}
strTarMsg = " \n";
strTarMsg += ("
"
+ response.getLocaleString("emlHeaderMessage2")
+ "
" + request.getParameter("tarMsg").trim()
+" \n");
strTarMsg += " \n";
String strFootermsg = "
\n";
if (!"".equals(base.getAttribute("footermsg", "").trim())) {
addElem(dom, emn, "footermsg",
base.getAttribute("footermsg").trim());
strFootermsg += "
" + base.getAttribute("footermsg").trim();
}
strFootermsg += "
\n";
strHeadermsg.append(strTarMsg);
strHeadermsg.append(strFootermsg);
addElem(dom, emn, "emailbody", strHeadermsg.toString());
return dom;
} else {
throw new SWBResourceException("Error Missing Data. The following data fields are required: "
+ "\n\t sender's name: " + strFromName
+ "\n\t email account of the sender: " + strFromEmail
+ "\n\t email message: " + strTarMsg
+ "\n in getDomEmail()");
}
} catch (Exception e) {
log.error("Error while generating email message in resource " + base.getResourceType().getResourceClassName() + " with identifier " + base.getId() + " - " + base.getTitle(), e);
}
return null;
}
/**
* Muestra la estructura de datos generada por getDom()
.
* Shows the data structure generated by getDom()
.
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param paramRequest the param request
* @throws IOException al obtener el Writer
del response
correspondiente.
* when getting the corresponding response
's Writer
.
* @throws SWBResourceException si se produce en getDom
.
* if getDom
propagates this exception
.
*/
@Override
public void doXML(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/xml; charset=ISO-8859-1");
response.setHeader("Cache-Control","no-cache");
response.setHeader("Pragma","no-cache");
Resource base=getResourceBase();
PrintWriter out = response.getWriter();
try {
Document dom = getDom(request, response, paramRequest);
out.println(SWBUtils.XML.domToXml(dom));
}catch(Exception e) {
log.error("Error in doXML method while rendering the XML script: "+base.getId()+"-"+base.getTitle(), e);
out.println("Error in doXML method while rendering the XML script");
}
}
/**
* Muestra la liga o la pantalla de captura de comentarios en base al valor
* del parámetro com_act
en el request
del usuario.
* Shows the commentaries' capture link or screen depending on the
* com_act
parameter's value through the
* user's request.
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param paramRequest the param request
* @throws IOException al obtener el Writer
del response
correspondiente.
* when getting the corresponding response
's Writer
.
* @throws SWBResourceException si no existe el archivo de mensajes del idioma utilizado.
* if there is no file message of the corresponding language.
*/
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws IOException, SWBResourceException {
response.setContentType("text/html; charset=ISO-8859-1");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Pragma", "no-cache");
PrintWriter out = response.getWriter();
Resource base = getResourceBase();
if( paramRequest.getCallMethod()==SWBParamRequest.Call_STRATEGY ) {
String surl = paramRequest.getWebPage().getUrl();
Iterator res = base.listResourceables();
while(res.hasNext()) {
Resourceable re = res.next();
if( re instanceof WebPage ) {
surl = ((WebPage)re).getUrl();
break;
}
}
if( base.getAttribute("link")!=null ) {
out.println(""+base.getAttribute("link")+"");
}else if( base.getAttribute("label")!=null ) {
out.println("");
}else if( base.getAttribute("img")!=null ) {
out.println("");
out.println("
");
out.println("");
}else {
out.println("");
out.println(""+base.getAttribute("lnktexto",paramRequest.getLocaleString("msgComments"))+"");
out.println("");
}
}else {
if( request.getParameter(_FAIL)!=null ) {
out.println("");
}else {
boolean hasCaptcha = Boolean.parseBoolean(base.getAttribute("captcha"));
Document dom = getDom(request, response, paramRequest);
String html;
try {
html = SWBUtils.XML.transformDom(tpl, dom);
if(hasCaptcha) {
String captcha = (getCaptchaScript(paramRequest));
html = html.replaceFirst("captcha", captcha);
html = html + getScriptCapcha();
}else
html = html.replaceFirst("captcha", " ");
}catch(TransformerException te) {
html = te.getMessage();
log.error(te.getMessage());
}
out.println(html);
}
}
out.flush();
out.close();
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doHelp(HttpServletRequest, HttpServletResponse, SWBParamRequest)
*/
@Override
public void doHelp(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
if( paramRequest.getCallMethod()==paramRequest.Call_CONTENT ) {
response.setContentType("text/html; charset=ISO-8859-1");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Pragma", "no-cache");
PrintWriter out = response.getWriter();
out.println("");
out.println(paramRequest.getLocaleString("msgSendEmail"));
out.println("
");
out.println(""+paramRequest.getLocaleString("msgDoViewAnotherMsg")+"");
out.println("
");
out.flush();
out.close();
}else {
doView(request, response, paramRequest);
}
}
/**
* Process emails.
*
* @param request the request
* @param response the response
* @throws TransformerException the transformer exception
* @throws SWBResourceException the sWB resource exception
* @throws Exception the exception
*/
private void processEmails(HttpServletRequest request, SWBActionResponse response) throws TransformerException, SWBResourceException, Exception {
Document dom = getDomEmail(request, response);
String from =SWBUtils.EMAIL.getAdminEmail();
// String from2 = dom.getElementsByTagName("fromemail").item(0).getFirstChild().getNodeValue();
// if( from2==null )
// throw new Exception(response.getLocaleString("msgErrCustomerEmailRequired"));
String fromname = dom.getElementsByTagName("fromname").item(0).getFirstChild().getNodeValue();
if( fromname==null )
throw new Exception(response.getLocaleString("msgErrCustomerNameRequired"));
String to = dom.getElementsByTagName("toemail").item(0).getFirstChild().getNodeValue();
if( to==null )
throw new Exception(response.getLocaleString("msgErrManagerEmailRequired"));
String subject = dom.getElementsByTagName("subject").item(0).getFirstChild().getNodeValue();
if( subject==null )
throw new Exception(response.getLocaleString("msgErrSubjectRequired"));
String message = dom.getElementsByTagName("message").item(0).getFirstChild().getNodeValue();
if( message==null )
throw new Exception(response.getLocaleString("msgErrMessageRequired"));
String html = SWBUtils.XML.transformDom(tpl, dom);
InternetAddress iaddress = new InternetAddress();
iaddress.setAddress(to);
ArrayList addresses = new ArrayList();
addresses.add(iaddress);
if( SWBUtils.EMAIL.sendMail(from, fromname, addresses, null, null, subject, "HTML", html, null, null, null)==null )
throw new Exception(response.getLocaleString("msgErrSending"));
}
/**
* Muestra la vista para los datos de administración de este recurso
* y realiza las operaciones de almacenamiento de información necesarias.
* Shows the data administration screen for this resource and performs the
* data store operations needed.
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param paramsRequest el objeto generado por SWB y asociado a la petición
* del usuario.the object gnerated by SWB and asociated to the user's request
* @throws IOException al obtener el Writer
del response
correspondiente.
* when getting the corresponding response
's Writer
.
* @throws SWBResourceException si no existe el archivo de mensajes del idioma utilizado.
* if there is no file message of the corresponding language.
*/
@Override
public void doAdmin(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramsRequest) throws IOException, SWBResourceException {
response.setContentType("text/html; charset=ISO-8859-1");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Pragma", "no-cache");
StringBuilder ret = new StringBuilder();
Resource base = getResourceBase();
String msg = paramsRequest.getLocaleString("msgUndefinedOperation");
String action = ((null != request.getParameter("act"))
&& (!"".equals(request.getParameter("act").trim()))
? request.getParameter("act").trim()
: paramsRequest.getAction());
WBAdmResourceUtils admResUtils = new WBAdmResourceUtils();
if (action.equals("add") || action.equals("edit")) {
ret.append(getForm(request, paramsRequest));
} else if (action.equals("update")) {
FileUpload fup = new FileUpload();
try {
fup.getFiles(request, response);
String applet = null;
String value = (null != fup.getValue("notmp")
&& !"".equals(fup.getValue("notmp").trim())
? fup.getValue("notmp").trim()
: "0");
if ("1".equals(value)
&& !"".equals(base.getAttribute("img", "").trim())) {
SWBUtils.IO.removeDirectory(SWBPortal.getWorkPath()
+ base.getWorkPath() + "/"
+ base.getAttribute("template").trim());
base.removeAttribute("template");
}else {
value = (null != fup.getFileName("template")
&& !"".equals(fup.getFileName("template").trim())
? fup.getFileName("template").trim()
: null);
if (value != null) {
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals("")) {
if (!admResUtils.isFileType(file, "xsl|xslt")) {
msg = paramsRequest.getLocaleString("msgErrFileType")
+" xsl, xslt: " + file;
} else {
applet = admResUtils.uploadFileParsed(base, fup,
"template", request.getSession().getId());
if (applet != null && !applet.trim().equals("")) {
base.setAttribute("template", file);
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile")
+ " " + value + ".";
}
}
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile")
+ " " + value + ".";
}
}
}
value = (null != fup.getValue("noimg")
&& !"".equals(fup.getValue("noimg").trim())
? fup.getValue("noimg").trim()
: "0");
if ("1".equals(value)
&& !"".equals(base.getAttribute("img", "").trim())) {
SWBUtils.IO.removeDirectory(SWBPortal.getWorkPath()
+ base.getWorkPath() + "/"
+ base.getAttribute("img").trim());
base.removeAttribute("img");
} else {
value = (null != fup.getFileName("img")
&& !"".equals(fup.getFileName("img").trim())
? fup.getFileName("img").trim()
: null);
if (value != null) {
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals("")) {
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")) {
msg = paramsRequest.getLocaleString("msgErrFileType")
+ " jpg, jpeg, gif, png: " + file;
} else {
if (admResUtils.uploadFile(base, fup, "img")) {
base.setAttribute("img", file);
} else {
msg = paramsRequest.getLocaleString(
"msgErrUploadFile")
+ " " + value + ".";
}
}
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile")
+ " " + value + ".";
}
}
}
value = (null != fup.getValue("noimgenviar")
&& !"".equals(fup.getValue("noimgenviar").trim())
? fup.getValue("noimgenviar").trim()
: "0");
if ("1".equals(value)
&& !"".equals(base.getAttribute("imgenviar", "").trim())) {
SWBUtils.IO.removeDirectory(SWBPortal.getWorkPath()
+ base.getWorkPath() + "/"
+ base.getAttribute("imgenviar").trim());
base.removeAttribute("imgenviar");
} else {
value = (null != fup.getFileName("imgenviar")
&& !"".equals(fup.getFileName("imgenviar").trim())
? fup.getFileName("imgenviar").trim()
: null);
if (value != null) {
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals("")) {
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")) {
msg = paramsRequest.getLocaleString("msgErrFileType") + " jpg, jpeg, gif, png: " + file;
}else {
if (admResUtils.uploadFile(base, fup, "imgenviar")) {
base.setAttribute("imgenviar", file);
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile") + " " + value + ".";
}
}
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile")
+ " " + value + ".";
}
}
}
value = (null != fup.getValue("noimglimpiar")
&& !"".equals(fup.getValue("noimglimpiar").trim())
? fup.getValue("noimglimpiar").trim()
: "0");
if ("1".equals(value)
&& !"".equals(base.getAttribute("imglimpiar", "").trim())) {
SWBUtils.IO.removeDirectory(SWBPortal.getWorkPath()
+ base.getWorkPath() + "/"
+ base.getAttribute("imglimpiar").trim());
base.removeAttribute("imglimpiar");
} else {
value = (null != fup.getFileName("imglimpiar")
&& !"".equals(fup.getFileName("imglimpiar").trim())
? fup.getFileName("imglimpiar").trim()
: null);
if (value != null) {
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals("")) {
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")) {
msg = paramsRequest.getLocaleString("msgErrFileType")
+ " jpg, jpeg, gif, png: " + file;
} else {
if (admResUtils.uploadFile(base, fup, "imglimpiar")) {
base.setAttribute("imglimpiar", file);
} else {
msg = paramsRequest.getLocaleString(
"msgErrUploadFile")
+ " " + value + ".";
}
}
} else {
msg = paramsRequest.getLocaleString("msgErrUploadFile")
+ " " + value + ".";
}
}
}
setAttribute(base, fup, "alt");
setAttribute(base, fup, "btntexto");
setAttribute(base, fup, "lnktexto");
// setAttribute(base, fup, "blnstyle");
setAttribute(base, fup, "altenviar");
setAttribute(base, fup, "btnenviar");
setAttribute(base, fup, "altlimpiar");
setAttribute(base, fup, "btnlimpiar");
setAttribute(base, fup, "firstname", "1");
setAttribute(base, fup, "lastname", "1");
setAttribute(base, fup, "middlename", "1");
// setAttribute(base, fup, "styleClass");
setAttribute(base, fup, "captcha");
// setAttribute(base, fup, "menubar", "yes");
// setAttribute(base, fup, "toolbar", "yes");
// setAttribute(base, fup, "status", "yes");
// setAttribute(base, fup, "location", "yes");
// setAttribute(base, fup, "directories", "yes");
// setAttribute(base, fup, "scrollbars", "yes");
// setAttribute(base, fup, "resizable", "yes");
// setAttribute(base, fup, "width");
// setAttribute(base, fup, "height");
// setAttribute(base, fup, "top");
// setAttribute(base, fup, "left");
setAttribute(base, fup, "subject");
setAttribute(base, fup, "headermsg");
setAttribute(base, fup, "footermsg");
setAttribute(base, fup, "generatelog");
base.updateAttributesToDB();
Document dom=base.getDom();
if (dom != null) {
removeAllNodes(dom, Node.ELEMENT_NODE, "comentario");
} else {
dom = SWBUtils.XML.getNewDocument();
Element root = dom.createElement("resource");
dom.appendChild(root);
}
value = (null != fup.getValue("comentarios")
&& !"".equals(fup.getValue("comentarios").trim())
? fup.getValue("comentarios").trim()
: null);
String type = (null != fup.getValue("type")
&& !"".equals(fup.getValue("type").trim())
? fup.getValue("type").trim()
: "");
String actype = (null != fup.getValue("actype")
&& !"".equals(fup.getValue("actype").trim())
? fup.getValue("actype").trim()
: "edit");
if (value != null) {
StringTokenizer stk = new StringTokenizer(value, "|");
for (int i = 1; stk.hasMoreTokens(); i++) {
if ("remove".equals(actype)
&& type.equals(String.valueOf(i))) {
stk.nextToken();
continue;
}
Element comment = dom.createElement("comentario");
StringTokenizer stk2 = new StringTokenizer(
stk.nextToken(), ";");
for (int j = 0; stk2.hasMoreTokens(); j++) {
value = stk2.nextToken();
String att = "comentario";
if (j == 1) {
att = "area";
} else if (j == 2) {
att = "responsable";
} else if (j == 3) {
att = "email";
}
if ("edit".equals(actype) && type.equals(String.valueOf(i))) {
value = (null != fup.getValue(att) && !"".equals(fup.getValue(att).trim()) ? fup.getValue(att).trim() : "");
}
if (j < 1) {
comment.appendChild(dom.createTextNode(value));
} else {
Element data = dom.createElement(att);
data.appendChild(dom.createTextNode(value));
comment.appendChild(data);
}
}
dom.getFirstChild().appendChild(comment);
}
}
base.setXml(SWBUtils.XML.domToXml(dom));
msg = paramsRequest.getLocaleString("msgOkUpdateResource")
+ " " + base.getId();
if (applet != null && !"".equals(applet.trim())) {
ret.append(applet);
} else {
ret.append("\n");
}
} catch (Exception e) {
log.error(e);
msg = paramsRequest.getLocaleString("msgErrUpdateResource")
+ " " + base.getId();
}
ret.append("\n");
} else if (action.equals("remove")) {
msg = admResUtils.removeResource(base);
ret.append("\n");
}
response.getWriter().print(ret.toString());
}
/**
* Fija un atributo en el objeto base con el nombre indicado.
* Si el atributo no existe en el objeto fup
o su valor es null
,
* el atributo att
se elimina de base
.
* Sets a property in this object's resource base with the specified name.
* If the indicated attribute does not exist in fup
, or its value
* is null
, the attribute is eliminated.
* @param base Resource
en el que se fijará el atributo.
* Resource
in which the attribute is going to be set.
* @param fup objeto del cual se obtiene el valor del atributo.
* object from which the attribute's value is gotten.
* @param att contiene el nombre del atributo a fijar en base
.
* contains the attribute's name.
*/
protected void setAttribute(Resource base, FileUpload fup, String att) {
try {
if (null != fup.getValue(att)
&& !"".equals(fup.getValue(att).trim())) {
base.setAttribute(att, fup.getValue(att).trim());
} else {
base.removeAttribute(att);
}
} catch (Exception e) {
log.error("Error while setting resource attribute: " + att + ", "
+ base.getId() + "-" + base.getTitle(), e);
}
}
/**
* Fija un atributo en el objeto base con el nombre y el valor indicados.
* Si el atributo no existe en el objeto fup o tiene otro valor al indicado,
* el atributo att se elimina de base.
* Sets a property in base
with the indicated name and value.
* If the indicated attribute does not exist in fup
or has another
* value than the specified as an argument, the attribute att
is
* eliminated from base
.
* @param base Resource
en el que se fijará el atributo.
* Resource
in which the attribute is going to be set.
* @param fup objeto del cual se verifica el valor del atributo.
* contains the value of the attribute to be set.
* @param att contiene el nombre del atributo a fijar en base.
* contains the name of the attribute to be set.
* @param value contiene el valor del atributo a fijar en base.
* value to set in the attribute, validated against the value for the
* attribute in fup
.
*/
protected void setAttribute(Resource base, FileUpload fup, String att, String value) {
try {
if (null != fup.getValue(att)
&& value.equals(fup.getValue(att).trim())) {
base.setAttribute(att, fup.getValue(att).trim());
} else {
base.removeAttribute(att);
}
} catch (Exception e) {
log.error("Error while setting resource attribute: " + att + ", "
+ base.getId() + "-" + base.getTitle(), e);
}
}
/**
* Elimina del dom recibido todos los elementos correspondientes al nodeType
* y name especificados. Removes from dom
all the elements whose
* name matches the value of name
.
* @param dom Document
del que se eliminarán los nodos.
* Document
from which the nodes are going to be removed.
* @param nodeType tipo de nodos a eliminar. node type to eliminate.
* @param name nombre de los nodos a eliminar. node name to search for elimination.
*/
protected void removeAllNodes(Document dom, short nodeType, String name) {
NodeList list = dom.getElementsByTagName(name);
for (int i = 0; i < list.getLength(); i++) {
Node node = list.item(i);
if (node.getNodeType() == nodeType) {
node.getParentNode().removeChild(node);
if (node.hasChildNodes()) {
removeAllNodes(dom, nodeType, name);
}
}
}
}
/**
* Arma una cadena que contiene el código HTML para mostrar la forma de los
* datos presentados por la pantalla de administración del recurso.
* Gets the HTML code for this resource's administration screen.
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param paramsRequest el objeto generado por SWB y asociado a la petición
* del usuario.the object gnerated by SWB and asociated to the user's request
* @return el código HTML a mostrar.
*
*/
private String getForm(HttpServletRequest request, SWBParamRequest paramsRequest) {
String name = getClass().getName().substring(getClass().getName().lastIndexOf(".") + 1);
WBAdmResourceUtils admResUtils = new WBAdmResourceUtils();
StringBuilder ret = new StringBuilder();
Resource base = getResourceBase();
try {
SWBResourceURL url = paramsRequest.getRenderUrl().setAction("update");
ret.append("");
ret.append(" \n");
ret.append(" \n");
ret.append(getScript(paramsRequest));
} catch (Exception e) {
log.error(e);
}
return ret.toString();
}
/**
* Agrupa los datos del área, responsable y cuenta de correo por cada
* comentario contenido en dom
.
* Parses dom
looking for the following data: area, recipient
* and e-mail account; of each commentary.
*
* @param dom Document
con los datos a extraer data to parse
* @return que
* almacenan los datos del área, responsable y cuenta de correo
* objects which contain area, recipient
* and e-mail account information.
*/
private ArrayList getTypesComment(Document dom) {
ArrayList list = new ArrayList();
if (dom != null) {
TypeComment tc = new TypeComment();
String comentario = "";
String area = "";
String responsable = "";
String email = "";
NodeList node = dom.getElementsByTagName("comentario");
for (int i = 0; i < node.getLength(); i++) {
area = "";
responsable = "";
email = "";
comentario = node.item(i).getFirstChild().getNodeValue().trim();
if (!"".equals(comentario)) {
NodeList child = node.item(i).getChildNodes();
for (int j = 0; j < child.getLength(); j++) {
if (child.item(j) != null) {
if ("area".equals(child.item(j).getNodeName())) {
area = child.item(j).getFirstChild(
).getNodeValue().trim();
} else if ("responsable".equals(
child.item(j).getNodeName())) {
responsable = child.item(j).getFirstChild(
).getNodeValue().trim();
} else if ("email".equals(
child.item(j).getNodeName())) {
email = child.item(j).getFirstChild(
).getNodeValue().trim();
}
}
}
if ("".equals(email)) {
child = dom.getFirstChild().getChildNodes();
for (int j = 0; j < child.getLength(); j++) {
if (child.item(j) != null) {
if ("area".equals(child.item(j).getNodeName())) {
area = child.item(j).getFirstChild(
).getNodeValue().trim();
} else if ("responsable".equals(
child.item(j).getNodeName())) {
responsable = child.item(j).getFirstChild(
).getNodeValue().trim();
} else if ("email".equals(
child.item(j).getNodeName())) {
email = child.item(j).getFirstChild(
).getNodeValue().trim();
}
}
}
}
tc = new TypeComment(comentario, area, responsable, email);
list.add(tc);
}
}
}
return list;
}
private String getScriptCapcha() {
StringBuilder ret = new StringBuilder();
ret.append("\n");
ret.append(" \n");
return ret.toString();
}
/**
* Obtiene el código de JavaScript necesario para validar los datos
* capturados en la forma presentada por getForm()
. Gets the
* JavaScript code which performs the validations asociated with the code
* generated by getForm()
.
*
* @param paramsRequest el objeto generado por SWB y asociado a la petición
* del usuario.the object gnerated by SWB and asociated to the user's request
* @return contiene el código de JavaScript a ejecutar
*/
private String getScript(SWBParamRequest paramsRequest) {
StringBuilder ret = new StringBuilder();
WBAdmResourceUtils admResUtils = new WBAdmResourceUtils();
try {
ret.append(" \n");
}catch(SWBResourceException e) {
}finally {
ret.append("\n--> \n");
ret.append(" \n");
}
return ret.toString();
}
/**
* Gets the captcha script.
*
* @param paramRequest the param request
* @return the captcha script
* @throws SWBResourceException the sWB resource exception
*/
private String getCaptchaScript(SWBParamRequest paramRequest) throws SWBResourceException {
StringBuilder html = new StringBuilder();
html.append(" \n");
html.append(" +"/swbadmin/jsp/securecode.jsp\")
\n");
html.append(" \n");
html.append(" \n");
html.append(" \n");
html.append(" \n");
html.append(" \n");
html.append(" \n");
return html.toString();
}
/**
* Agrega un nodo al parent
especificado en el doc
recibido.
* Inserts a node to the specified parent
in doc
* @param doc Document al que se desea agregar el nodo. Document
* to which the new node is going to be added.
* @param parent elemento que será el padre del nuevo nodo. new node's
* parent element
* @param elemName nombre del elemento a agregar. new element's name
* @param elemValue valor del elemento a agregar. new element's value
*/
private void addElem(Document doc, Element parent, String elemName, String elemValue) {
Element elem = doc.createElement(elemName);
elem.appendChild(doc.createTextNode(elemValue));
parent.appendChild(elem);
}
/**
* Agrega la información enviada por correo, al archivo log de este
* recurso. Adds to this resource's log the commentaries' data set by mail.
*
* @param request the request
* @param response the response
* @throws IOException Signals that an I/O exception has occurred.
*/
protected void feedCommentLog(HttpServletRequest request, SWBActionResponse response) throws IOException {
Resource base = getResourceBase();
String logPath = SWBPortal.getWorkPath() + base.getWorkPath() + "/Comment.log";
StringBuilder toLog = new StringBuilder();
Date now = new Date();
NodeList nl = null;
toLog.append(SWBUtils.TEXT.iso8601DateFormat(now));
User user = response.getUser();
if( user.isSigned() ) {
toLog.append("\n User:");
toLog.append((null != user.getFirstName() && !"".equals(user.getFirstName().trim())) ? user.getFirstName().trim() : "");
toLog.append((null != user.getLastName() && !"".equals(user.getLastName().trim())) ? user.getLastName().trim() : "");
toLog.append((null != user.getSecondLastName() && !"".equals(user.getSecondLastName().trim())) ? user.getSecondLastName().trim() : "");
}
try {
Document dom = getDomEmail(request, response);
nl = dom.getElementsByTagName("fromname");
toLog.append("\n From:" + (nl != null && nl.getLength() > 0
? nl.item(0).getFirstChild().getNodeValue() : ""));
nl = dom.getElementsByTagName("fromemail");
if (nl != null && nl.getLength() > 0) {
toLog.append("<" + nl.item(0).getFirstChild().getNodeValue() + ">");
}
/* toLog.append("\n To:" + dom.getElementsByTagName("responsable").item(
0).getFirstChild().getNodeValue());
toLog.append("<" + dom.getElementsByTagName("toemail").item(
0).getFirstChild().getNodeValue() + ">"); */
nl = dom.getElementsByTagName("subject");
toLog.append("\n Subject:" + (nl != null && nl.getLength() > 0
? nl.item(0).getFirstChild().getNodeValue() : ""));
/* toLog.append("\n Area:" + dom.getElementsByTagName("area").item(
0).getFirstChild().getNodeValue()); */
nl = dom.getElementsByTagName("phone");
toLog.append("\n Phone:" + (nl != null && nl.getLength() > 0
? nl.item(0).getFirstChild().getNodeValue() : ""));
nl = dom.getElementsByTagName("fax");
toLog.append("\n Fax:" + (nl != null && nl.getLength() > 0
? nl.item(0).getFirstChild().getNodeValue() : ""));
nl = dom.getElementsByTagName("message");
toLog.append("\n Message:" + (nl != null && nl.getLength() > 0
? nl.item(0).getFirstChild().getNodeValue() : ""));
toLog.append("\n");
}catch(SWBResourceException te) {
log.error(te);
}
File file = new File(SWBPortal.getWorkPath() + base.getWorkPath());
if (!file.exists()) {
file.mkdirs();
}
SWBUtils.IO.log2File(logPath, toLog.toString());
}
}
/**
* Objeto: Tipos de comentario en memoria.
*
* Object: Comment types in memory.
*
* @author : Vanessa Arredondo Núñez
* @version 1.0
*/
class TypeComment {
private String comentario;
private String area;
private String responsable;
private String email;
/**
* Creates a new instance of TypeComment
*/
public TypeComment() {
}
/**
* Creates a new instance of TypeComment
*
* @param comentario cuerpo del comentario. commentary's text
* @param area área a la que pertenece el destinatario. recipient's area
* @param responsable destinatario. recipient
* @param email cuenta de correo del destinatario. recipient's e-mail account
*/
public TypeComment(String comentario, String area, String responsable, String email) {
this.comentario = comentario;
this.area = area;
this.responsable = responsable;
this.email = email;
}
/**
* Obtiene el texto del comentario. Gets the commentary's text.
* @return String
el texto del comentario. commentary's text.
*/
public String getComentario() {
return this.comentario;
}
/**
* Fija el texto del comentario. Sets the commentary's text.
* @param comentario texto a enviar como comentario. text to send as a
* comment.
*/
public void setComentario(String comentario) {
this.comentario = comentario;
}
/**
* Obtiene el nombre del área del destinatario. Gets the recipient's area.
* @return String
nombre del área del destinatario. recipient's
* area's name.
*/
public String getArea() {
return this.area;
}
/**
* Fija una nueva área del destinatario. Sets the recipient's area.
* @param area nuevo nombre del área del destinatario. new recipient's
* area's name.
*/
public void setArea(String area) {
this.area = area;
}
/**
* Obtiene el nombre del destinatario del comentario. Gets the recipient's name
* @return String
nombre del destinatario del comentario. recipient's name.
*/
public String getResponsable() {
return this.responsable;
}
/**
* Fija un nuevo nombre del destinatario. Sets the recipient's name.
* @param responsable nuevo nombre del destinatario. new recipient's name.
*/
public void setResponsable(String responsable) {
this.responsable = responsable;
}
/**
* Obtiene cuenta de correo del destinatario. Gets the recipient's e-mail account.
* @return String
cuenta de correo del destinatario. recipient's
* e-mail account.
*/
public String getEmail() {
return this.email;
}
/**
* Fija una nueva cuenta de correo del destinatario. Sets a new recipient's e-mail account.
* @param email nueva cuenta de correo del destinatario. new recipient's
* e-mail account.
*/
public void setEmail(String email) {
this.email = email;
}
}