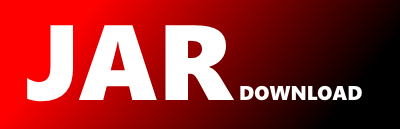
org.semanticwb.portal.resources.ImageGallery Maven / Gradle / Ivy
Show all versions of SWBPortal Show documentation
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.semanticwb.Logger;
import org.semanticwb.SWBPlatform;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBUtils;
import org.semanticwb.base.util.ImageResizer;
import org.semanticwb.model.Resource;
import org.semanticwb.portal.TemplateImp;
import org.semanticwb.portal.admin.admresources.util.WBAdmResourceUtils;
import org.semanticwb.portal.admin.resources.StyleInner;
import org.semanticwb.portal.api.*;
// TODO: Auto-generated Javadoc
/**
* ImageGallery se encarga de desplegar y administrar una colección de imágenes
* dispuestas en un carrusel.
*
* Cada imagen en el carrusel puede ser seleccionada para verse en detalle a
* tamaño real.
*
* ImageGallery is in charge to unfold and to administer image collection
* disposes in a round robin.
*
* Every image in the round robin can be selected for detail in real size.
*
* @author : Carlos Ramos Inchaustegui
* @version 1.0
*/
public class ImageGallery extends GenericResource
{
public String replaceTags(String str, HttpServletRequest request, SWBParameters paramRequest)
{
if (str == null || str.trim().length() == 0)
{
return "";
}
str = str.trim();
//TODO: codificar cualquier atributo o texto
if (str.indexOf("{") > -1)
{
Iterator it = SWBUtils.TEXT.findInterStr(str, "{request.getParameter(\"", "\")}");
while (it.hasNext())
{
String s = (String) it.next();
str = SWBUtils.TEXT.replaceAll(str, "{request.getParameter(\"" + s + "\")}", request.getParameter(replaceTags(s, request, paramRequest)));
}
it = SWBUtils.TEXT.findInterStr(str, "{session.getAttribute(\"", "\")}");
while (it.hasNext())
{
String s = (String) it.next();
str = SWBUtils.TEXT.replaceAll(str, "{session.getAttribute(\"" + s + "\")}", (String) request.getSession().getAttribute(replaceTags(s, request, paramRequest)));
}
it = SWBUtils.TEXT.findInterStr(str, "{getEnv(\"", "\")}");
while (it.hasNext())
{
String s = (String) it.next();
str = SWBUtils.TEXT.replaceAll(str, "{getEnv(\"" + s + "\")}", SWBPlatform.getEnv(replaceTags(s, request, paramRequest)));
}
str = SWBUtils.TEXT.replaceAll(str, "{user.login}", paramRequest.getUser().getLogin());
str = SWBUtils.TEXT.replaceAll(str, "{user.email}", paramRequest.getUser().getEmail());
str = SWBUtils.TEXT.replaceAll(str, "{user.language}", paramRequest.getUser().getLanguage());
str = SWBUtils.TEXT.replaceAll(str, "{user.country}", paramRequest.getUser().getCountry());
str = SWBUtils.TEXT.replaceAll(str, "{webpath}", SWBPortal.getContextPath());
str = SWBUtils.TEXT.replaceAll(str, "{distpath}", SWBPortal.getDistributorPath());
str = SWBUtils.TEXT.replaceAll(str, "{webworkpath}", SWBPortal.getWebWorkPath());
str = SWBUtils.TEXT.replaceAll(str, "{workpath}", SWBPortal.getWorkPath());
str = SWBUtils.TEXT.replaceAll(str, "{websiteid}", paramRequest.getWebPage().getWebSiteId());
str = SWBUtils.TEXT.replaceAll(str, "{topicurl}", paramRequest.getWebPage().getUrl());
str = SWBUtils.TEXT.replaceAll(str, "{topicid}", paramRequest.getWebPage().getId());
str = SWBUtils.TEXT.replaceAll(str, "{topic.title}", paramRequest.getWebPage().getDisplayTitle(paramRequest.getUser().getLanguage()));
if (str.indexOf("{templatepath}") > -1)
{
//TODO:pasar template por paramrequest
TemplateImp template = (TemplateImp) SWBPortal.getTemplateMgr().getTemplate(paramRequest.getUser(), paramRequest.getAdminTopic());
if (template != null)
{
str = SWBUtils.TEXT.replaceAll(str, "{templatepath}", template.getActualPath());
}
}
}
return str;
}
/**
* The log.
*/
private static Logger log = SWBUtils.getLogger(ImageGallery.class);
/**
* The adm res utils.
*/
private WBAdmResourceUtils admResUtils = new WBAdmResourceUtils();
/**
* The work path.
*/
private String workPath;
/**
* The web work path.
*/
private String webWorkPath;
/**
* The Constant _thumbnail.
*/
private static final String _thumbnail = "thumbn_";
/**
* The si.
*/
private StyleInner si;
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#setResourceBase(org.semanticwb.model.Resource)
*/
private void generaTbm()
{
Resource base = getResourceBase();
int width;
try
{
width = Integer.parseInt(base.getAttribute("width"));
}
catch (Exception e)
{
width = 180;
}
Iterator it = base.getAttributeNames();
while (it.hasNext())
{
String attname = it.next();
String attval = base.getAttribute(attname);
if (attname.startsWith("imggallery_") && attval != null)
{
String fn = attval.substring(attval.lastIndexOf("/") + 1);
File img = new File(workPath + fn);
File thumbnail = new File(workPath + _thumbnail + fn);
if (thumbnail.exists())
{
thumbnail.delete();
}
try
{
String s_cut = base.getAttribute("cut", "false");
boolean cut = Boolean.parseBoolean(s_cut);
if (cut)
{
try
{
int height = Integer.parseInt(base.getAttribute("height", "180"));
ImageResizer.crop(img, width, height, thumbnail, "jpeg");
}
catch (NumberFormatException e)
{
log.error(e);
}
}
else
{
ImageResizer.resizeCrop(img, width, thumbnail, "jpeg");
}
}
catch (IOException ioe)
{
log.error("Error while setting thumbnail for image: " + img + " in resource " + base.getId() + "-" + base.getTitle(), ioe);
}
}
else
{
}
}
}
@Override
public void setResourceBase(Resource base)
{
try
{
super.setResourceBase(base);
workPath = SWBPortal.getWorkPath() + base.getWorkPath() + "/";
webWorkPath = SWBPortal.getWebWorkPath() + base.getWorkPath() + "/";
generaTbm();
// Si no existen thumbnails se crean
}
catch (Exception e)
{
log.error("Error while setting resource base: " + base.getId() + "-" + base.getTitle(), e);
}
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doView(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
*/
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException
{
Resource base = getResourceBase();
List descriptions = new ArrayList();
List imgpath = new ArrayList();
Iterator it = base.getAttributeNames();
while (it.hasNext())
{
String attname = it.next();
String attval = base.getAttribute(attname);
if (attval != null && attname.startsWith("imggallery_"))
{
imgpath.add(webWorkPath + attval);
String descripiton="";
if(base.getAttribute("desc_"+attname.substring(11))!=null)
{
descripiton=base.getAttribute("desc_"+attname.substring(11));
}
descriptions.add(descripiton);
}
}
String[] ip = new String[imgpath.size()];
imgpath.toArray(ip);
List imgpath_tmb = new ArrayList();
for (String image : imgpath)
{
String img_tmb = image.substring(0, image.lastIndexOf("/")) + "/" + _thumbnail + image.substring(image.lastIndexOf("/") + 1);
imgpath_tmb.add(img_tmb);
}
if (base.getAttribute("jspPath") != null && !base.getAttribute("jspPath").trim().isEmpty())
{
try
{
String path = base.getAttribute("jspPath");
path = replaceTags(path, request, paramRequest);
request.setAttribute("paramRequest", paramRequest);
request.setAttribute("images", imgpath);
request.setAttribute("thumbnails", imgpath_tmb);
request.setAttribute("descriptions", descriptions);
//request.getRequestDispatcher(path).include(request, response);
RequestDispatcher requestDispatcher=request.getRequestDispatcher(path);
if(requestDispatcher!=null)
{
requestDispatcher.include(request, response);
}
return;
}
catch (IOException e)
{
log.error(e);
return;
}
catch (ServletException e)
{
log.error(e);
return;
}
}
response.setContentType("text/html; charset=ISO-8859-1");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Pragma", "no-cache");
PrintWriter out = response.getWriter();
String lang = paramRequest.getUser().getLanguage();
String script = getGalleryScript(base.getId(), Integer.parseInt(base.getAttribute("width", "220")), Integer.parseInt(base.getAttribute("height", "220")), Boolean.valueOf(base.getAttribute("autoplay")), Integer.parseInt(base.getAttribute("pause", "2500")), Integer.parseInt(base.getAttribute("fadetime", "500")), Boolean.parseBoolean(base.getAttribute("title")) ? (base.getTitle(lang) == null ? base.getDisplayTitle(lang) : base.getTitle(lang)) : "", ip);
out.print(script);
out.flush();
}
/**
* Gets the gallery script.
*
* @param oid the oid
* @param width the width
* @param height the height
* @param autoplay the autoplay
* @param pause the pause
* @param fadetime the fadetime
* @param title the title
* @param imagepath the imagepath
* @return the gallery script
*/
public String getGalleryScript(String oid, int width, int height, boolean autoplay, int pause, int fadetime, String title, String[] imagepath)
{
Resource base = getResourceBase();
StringBuilder out = new StringBuilder();
if (base.getAttribute("css") != null)
{
out.append("\n");
}
out.append("\n");
out.append("\n");
out.append("\n");
//out.append(" ");
//out.append(""+ title +" ");
out.append(" ");
out.append("" + title + " ");
out.append(" ");
out.append("\n");
return out.toString();
}
/**
* Gets the gallery script.
*
* @param divId the div id
* @param title the title
* @param imgpath the imgpath
* @return the gallery script
*/
public String getGalleryScript(String divId, String title, String[] imgpath)
{
return getGalleryScript(divId, 220, 170, false, 2500, 500, title, imgpath);
}
/**
* Gets the gallery script.
*
* @param imgpath the imgpath
* @return the gallery script
*/
public String getGalleryScript(String[] imgpath)
{
return getGalleryScript(Integer.toString((int) Math.random() * 100), 220, 170, false, 2500, 500, "", imgpath);
}
/**
* Gets the gallery script.
*
* @param autoplay the autoplay
* @param imgpath the imgpath
* @return the gallery script
*/
public String getGalleryScript(boolean autoplay, String[] imgpath)
{
return getGalleryScript(Integer.toString((int) Math.random() * 100), 220, 170, autoplay, 2500, 500, "", imgpath);
}
/**
* Gets the gallery script.
*
* @param width the width
* @param height the height
* @param imgpath the imgpath
* @return the gallery script
*/
public String getGalleryScript(int width, int height, String[] imgpath)
{
return getGalleryScript(Integer.toString((int) Math.random() * 100), width, height, false, 2500, 500, "", imgpath);
}
/**
* Gets the gallery script.
*
* @param width the width
* @param height the height
* @param autoplay the autoplay
* @param imgpath the imgpath
* @return the gallery script
*/
public String getGalleryScript(int width, int height, boolean autoplay, String[] imgpath)
{
return getGalleryScript(Integer.toString((int) Math.random() * 100), width, height, autoplay, 2500, 500, "", imgpath);
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doAdmin(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
*/
@Override
public void doAdmin(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException
{
response.setContentType("text/html; charset=ISO-8859-1");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Pragma", "no-cache");
PrintWriter out = response.getWriter();
Resource base = getResourceBase();
String msg = paramRequest.getLocaleString("usrmsg_ImageGallery_doAdmin_undefinedOperation");
String action = null != request.getParameter("act") && !"".equals(request.getParameter("act").trim()) ? request.getParameter("act").trim() : paramRequest.getAction();
if (action.equalsIgnoreCase("add") || action.equalsIgnoreCase("edit"))
{
out.println(getForm(request, paramRequest));
}
else if (action.equalsIgnoreCase("update"))
{
// upload settings
final int MEMORY_THRESHOLD = 1024 * 1024 * 3; // 3MB
final int MAX_FILE_SIZE = 1024 * 1024 * 40; // 40MB
final int MAX_REQUEST_SIZE = 1024 * 1024 * 50; // 50MB
//FileUpload fup = new FileUpload();
DiskFileItemFactory factory = new DiskFileItemFactory();
// sets memory threshold - beyond which files are stored in disk
factory.setSizeThreshold(MEMORY_THRESHOLD);
// sets temporary location to store files
factory.setRepository(new File(System.getProperty("java.io.tmpdir")));
ServletFileUpload upload = new ServletFileUpload(factory);
// sets maximum size of upload file
upload.setFileSizeMax(MAX_FILE_SIZE);
// sets maximum size of request (include file + form data)
upload.setSizeMax(MAX_REQUEST_SIZE);
try
{
List formItems = upload.parseRequest(request);
String jspPath = null != getValue("jspPath", formItems) && !"".equals(getValue("jspPath", formItems).trim()) ? getValue("jspPath", formItems).trim() : null;
base.setAttribute("jspPath", jspPath);
String value = null != getValue("title", formItems) && !"".equals(getValue("title", formItems).trim()) ? getValue("title", formItems).trim() : null;
base.setAttribute("title", value);
value = null != getValue("width", formItems) && !"".equals(getValue("width", formItems).trim()) ? getValue("width", formItems).trim() : "180";
base.setAttribute("width", value);
value = null != getValue("height", formItems) && !"".equals(getValue("height", formItems).trim()) ? getValue("height", formItems).trim() : null;
base.setAttribute("height", value);
value = null != getValue("autoplay", formItems) && !"".equals(getValue("autoplay", formItems).trim()) ? getValue("autoplay", formItems).trim() : null;
base.setAttribute("autoplay", value);
value = null != getValue("cut", formItems) && !"".equals(getValue("cut", formItems).trim()) ? getValue("cut", formItems).trim() : null;
base.setAttribute("cut", value);
value = null != getValue("pause", formItems) && !"".equals(getValue("pause", formItems).trim()) ? getValue("pause", formItems).trim() : null;
base.setAttribute("pause", value);
value = null != getValue("fadetime", formItems) && !"".equals(getValue("fadetime", formItems).trim()) ? getValue("fadetime", formItems).trim() : null;
base.setAttribute("fadetime", value);
value = null != getValue("titlestyle", formItems) && !"".equals(getValue("titlestyle", formItems).trim()) ? getValue("titlestyle", formItems).trim() : null;
base.setAttribute("titlestyle", value);
int width = Integer.parseInt(base.getAttribute("width"));
//String filenameAttr, removeChk;
String prefix = "imggallery_" + base.getId() + "_";
value = null != getValue("deleteall", formItems) && !"".equals(getValue("deleteall", formItems).trim()) ? getValue("deleteall", formItems).trim() : null;
if (value != null)
{
Map values = new HashMap();
Iterator keys = base.getAttributeNames();
while (keys.hasNext())
{
String key = keys.next();
String file = base.getAttribute(key, null);
if (file != null && key.startsWith(prefix))
{
values.put(key, file);
}
}
for (String key : values.keySet())
{
File file = new File(workPath + values.get(key));
file.delete();
file = new File(workPath + _thumbnail + base.getAttribute(key));
file.delete();
base.removeAttribute(key);
base.removeAttribute("desc_"+key);
}
}
else
{
for (FileItem item : formItems)
{
if (item.getFieldName().startsWith("desc_"))
{
base.setAttribute(item.getFieldName(), getValue(item.getFieldName(), formItems));
}
}
}
List imagesAddBatch = new ArrayList();
for (FileItem item : formItems)
{
if (item.getFieldName().equals("fileupload"))
{
imagesAddBatch.add(item);
}
}
for (FileItem item : imagesAddBatch)
{
value = getFileName(item.getFieldName(), imagesAddBatch);
String filenameAttr = item.getFieldName();
String id = UUID.randomUUID().toString();
String key = prefix + id;
if (value != null)
{
String filename = admResUtils.getFileName(base, value);
int intPos = filename.lastIndexOf(".");
if (intPos != -1)
{
filename = key + filename.substring(intPos);
}
if (filename != null && !filename.trim().equals(""))
{
if (!admResUtils.isFileType(filename, "bmp|jpg|jpeg|gif|png"))
{
msg = paramRequest.getLocaleString("msgErrFileType") + " bmp, jpg, jpeg, gif, png: " + filename;
}
else
{
if (uploadFile(base, filename, filenameAttr, item))
{
base.setAttribute(key, filename);
}
else
{
msg = paramRequest.getLocaleString("msgErrUploadFile") + " " + value + ".";
}
}
}
else
{
msg = paramRequest.getLocaleString("msgErrUploadFile") + " " + value + ".";
}
}
}
String prefix_removeChk = "remove_" + base.getId() + "_";
List imagesRemove = new ArrayList();
for (FileItem item : formItems)
{
if (item.getFieldName().startsWith(prefix_removeChk))
{
imagesRemove.add(item);
}
}
for (FileItem item : imagesRemove)
{
String filenameAttr = item.getFieldName();
filenameAttr = filenameAttr.replaceFirst("remove_", "imggallery_");
File file = new File(workPath + base.getAttribute(filenameAttr));
file.delete();
file = new File(workPath + _thumbnail + base.getAttribute(filenameAttr));
file.delete();
base.removeAttribute(filenameAttr);
base.removeAttribute("desc_"+filenameAttr);
}
//String prefix = "imggallery_" + base.getId() + "_";
List imagesAdd = new ArrayList();
for (FileItem item : formItems)
{
if (item.getFieldName().startsWith(prefix))
{
imagesAdd.add(item);
}
}
for (FileItem item : imagesAdd)
{
value = getFileName(item.getFieldName(), imagesAdd);
String filenameAttr = item.getFieldName();
if (value != null)
{
String filename = admResUtils.getFileName(base, value);
int intPos = filename.lastIndexOf(".");
if (intPos != -1)
{
filename = item.getFieldName() + filename.substring(intPos);
}
if (filename != null && !filename.trim().equals(""))
{
if (!admResUtils.isFileType(filename, "bmp|jpg|jpeg|gif|png"))
{
msg = paramRequest.getLocaleString("msgErrFileType") + " bmp, jpg, jpeg, gif, png: " + filename;
}
else
{
if (uploadFile(base, filename, filenameAttr, item))
{
base.setAttribute(filenameAttr, filename);
}
else
{
msg = paramRequest.getLocaleString("msgErrUploadFile") + " " + value + ".";
}
}
}
else
{
msg = paramRequest.getLocaleString("msgErrUploadFile") + " " + value + ".";
}
}
}
base.updateAttributesToDB();
generaTbm();
msg = paramRequest.getLocaleString("msgOkUpdateResource") + " " + base.getId();
out.println("");
}
catch (Exception e)
{
log.error(e);
msg = paramRequest.getLocaleString("msgErrUpdateResource") + " " + base.getId();
}
}
else if (action.equals("remove"))
{
msg = admResUtils.removeResource(base);
out.println(
"");
}
out.flush();
}
/**
* Gets the form.
*
* @param request the request
* @param paramRequest the param request
* @return the form
*/
private String getForm(javax.servlet.http.HttpServletRequest request, SWBParamRequest paramRequest) throws SWBResourceException
{
StringBuilder htm = new StringBuilder();
Resource base = getResourceBase();
// try {
SWBResourceURL url = paramRequest.getRenderUrl().setMode(SWBParamRequest.Mode_ADMIN);
url.setAction("update");
htm.append("\n");
htm.append("\n ");
htm.append("\n ");
htm.append("\n* " + paramRequest.getLocaleString("usrmsg_ImageGallery_doAdmin_required"));
htm.append("\n ");
htm.append("\n");
htm.append(getScript());
// }catch(Exception e) {
// log.error(e);
// }
return htm.toString();
}
/**
* Gets the script.
*
* @return the script
*/
private String getScript()
{
StringBuffer htm = new StringBuffer();
try
{
htm.append("\n\n");
}
catch (Exception e)
{
log.error(e);
}
return htm.toString();
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#processRequest(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
*/
@Override
public void processRequest(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramsRequest) throws SWBResourceException, IOException
{
if (paramsRequest.getMode().equalsIgnoreCase("fillStyle"))
{
doEditStyle(request, response, paramsRequest);
}
else
{
super.processRequest(request, response, paramsRequest);
}
}
/**
* Do edit style.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
public void doEditStyle(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException
{
// Resource base = getResourceBase();
// String stel = request.getParameter("stel");
// String[] tkns = stel.split("@",3);
//
// HashMap tabs = (HashMap)si.getMm(base.getId());
// if( tabs!=null && tkns[1].length()>0 ) {
// try {
// HashMap t = (HashMap)tabs.get(tkns[0]);
// if(tkns[2].equalsIgnoreCase("empty") || tkns[2].length()==0)
// t.remove(tkns[1]);
// else
// t.put(tkns[1], tkns[2]);
// StringBuilder css = new StringBuilder();
// Iterator ittabs = tabs.keySet().iterator();
// while(ittabs.hasNext()) {
// String tab = ittabs.next();
// css.append(tab);
// css.append("{");
// HashMap selectors = (HashMap)tabs.get(tab);
// Iterator its = selectors.keySet().iterator();
// while(its.hasNext()) {
// String l = its.next();
// css.append(l+":"+selectors.get(l)+";");
// }
// css.append("}");
// }
// base.setAttribute("css", css.toString());
// try{
// base.updateAttributesToDB();
// }catch(Exception e){
// log.error("Error al guardar la hoja de estilos del recurso: "+base.getId() +"-"+ base.getTitle(), e);
// }
// }catch(IndexOutOfBoundsException iobe) {
// log.error("Error al editar la hoja de estilos del recurso: "+base.getId() +"-"+ base.getTitle(), iobe);
// }
// }
}
/**
* Render gallery.
*
* @param imgpath the imgpath
* @return the string
*/
public String renderGallery(String[] imgpath)
{
return renderGallery(Integer.toString((int) Math.random() * 100), 220, 170, false, 2500, 500, 420, 370, "", "", imgpath);
}
/**
* Render gallery.
*
* @param oid the oid
* @param width the width
* @param height the height
* @param autoplay the autoplay
* @param pause the pause
* @param fadetime the fadetime
* @param fullwidth the fullwidth
* @param fullheight the fullheight
* @param title the title
* @param titlestyle the titlestyle
* @param imagepath the imagepath
* @return the string
*/
public String renderGallery(String oid, int width, int height, boolean autoplay, int pause, int fadetime, int fullwidth, int fullheight, String title, String titlestyle, String[] imagepath)
{
StringBuilder out = new StringBuilder();
out.append("\n");
out.append("");
out.append(" ");
out.append(" ");
out.append("" + title + " ");
out.append(" ");
out.append("\n");
return out.toString();
}
public String getValue(String name, List list)
{
for (FileItem fileitem : list)
{
if (fileitem.getFieldName().equals(name))
{
return fileitem.getString();
}
}
return null;
}
public String getFileName(String name, List list)
{
for (FileItem fileitem : list)
{
if (fileitem.getFieldName().equals(name))
{
return fileitem.getName();
}
}
return null;
}
public byte[] getFileData(String name, FileItem fileitem) throws IOException
{
byte[] content = new byte[(int) fileitem.getSize()];
InputStream in = fileitem.getInputStream();
in.read(content);
return content;
}
public boolean uploadFile(Resource base, String fileName, String pInForm, FileItem item)
{
//String strWorkPath = workPath;
boolean bOk = false;
try
{
if (fileName != null && !fileName.trim().equals(""))
{
//strWorkPath += base.getWorkPath() + "/";
File file = new File(workPath);
if (!file.exists())
{
file.mkdirs();
}
if (file.exists() && file.isDirectory())
{
String s3 = fileName;
int i = s3.lastIndexOf("\\");
if (i != -1)
{
s3 = s3.substring(i + 1);
}
i = s3.lastIndexOf("/");
if (i != -1)
{
s3 = s3.substring(i + 1);
}
byte[] content = getFileData(pInForm, item);
FileOutputStream fileoutputstream = new FileOutputStream(workPath + s3);
fileoutputstream.write(content, 0, content.length);
fileoutputstream.close();
return true;
}
}
}
catch (Exception e)
{
log.error(SWBUtils.TEXT.getLocaleString("locale_swb_util", "error_WBResource_uploadFile_exc01") + " " + base.getId() + SWBUtils.TEXT.getLocaleString("locale_swb_util", "error_WBResource_uploadFile_exc02") + " " + base.getResourceType() + SWBUtils.TEXT.getLocaleString("locale_swb_util", "error_WBResource_uploadFile_exc03") + " " + fileName + ".");
}
return bOk;
}
public static String escapeTags(String original)
{
if (original == null)
{
return "";
}
StringBuffer out = new StringBuffer("");
char[] chars = original.toCharArray();
for (int i = 0; i < chars.length; i++)
{
boolean found = true;
switch (chars[i])
{
case '&':
out.append("&");
break; //&
case 60:
out.append("<");
break; //<
case 62:
out.append(">");
break; //>
case 34:
out.append(""");
break; //"
default:
found = false;
break;
}
if (!found)
{
out.append(chars[i]);
}
}
return out.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy