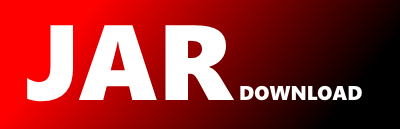
org.semanticwb.portal.resources.Poll Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SWBPortal Show documentation
Show all versions of SWBPortal Show documentation
SemanticWebBuilder Portal API components and utilities
The newest version!
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Timestamp;
import java.util.Date;
import java.util.HashMap;
import java.util.Locale;
import java.util.StringTokenizer;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.transform.Templates;
import javax.xml.transform.TransformerException;
import org.semanticwb.Logger;
import org.semanticwb.SWBPlatform;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBUtils;
import org.semanticwb.model.Resource;
import org.semanticwb.portal.admin.admresources.util.WBAdmResourceUtils;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import org.w3c.dom.Node;
import org.semanticwb.portal.util.SWBCookieMgr;
import org.semanticwb.portal.api.*;
import org.semanticwb.portal.util.FileUpload;
/**
* Poll se encarga de desplegar y administrar una encuesta de opinion bajo
* ciertos criterios(configuraci?n de recurso).
*
* Poll is in charge to unfold and to administer a survey of opinion under
* certain criteria (resource configuration).
*
*/
public class Poll extends GenericResource {
/** The log. */
private static Logger log = SWBUtils.getLogger(Poll.class);
/** The Constant PREF. */
private static final String PREF = "poll_";
/** The hash prim. */
HashMap hashPrim=new HashMap();
/** The tpl. */
private Templates tpl;
/** The work path. */
private String workPath;
/** The web work path. */
private String webWorkPath;
/** The path. */
private String path = SWBPlatform.getContextPath() +"/swbadmin/xsl/Poll/";
/** The restype. */
private static String restype;
/** The Mng cookie. */
private SWBCookieMgr MngCookie;
/** The adm res utils. */
private WBAdmResourceUtils admResUtils = new WBAdmResourceUtils();
/**
* The Enum Display.
*/
public enum Display {
/** The SLIDE. */
SLIDE,
/** The POPUP. */
POPUP,
/** The SIMPLE. */
SIMPLE;
/* (non-Javadoc)
* @see java.lang.Enum#toString()
*/
@Override
public String toString() {
return Integer.toString(this.ordinal());
}
}
/**
* The Enum VMode.
*/
public enum VMode {
/** The IP. */
IP,
/** The COOKIE. */
COOKIE;
/* (non-Javadoc)
* @see java.lang.Enum#toString()
*/
@Override
public String toString() {
return Integer.toString(this.ordinal());
}
}
/**
* The Enum LocLnks.
*/
public enum LocLnks {
/** The INPOLL. */
INPOLL,
/** The INRESULTS. */
INRESULTS,
/** The INBOTH. */
INBOTH;
/* (non-Javadoc)
* @see java.lang.Enum#toString()
*/
@Override
public String toString() {
return Integer.toString(this.ordinal());
}
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#setResourceBase(Resource)
*/
@Override
public void setResourceBase(Resource base) {
try {
super.setResourceBase(base);
workPath = SWBPortal.getWorkPath()+base.getWorkPath()+"/";
//webWorkPath = SWBPortal.getWebWorkPath()+base.getWorkPath()+"/";
webWorkPath = SWBPortal.getWebWorkPath();
restype= base.getResourceType().getResourceClassName();
}catch(Exception e) {
log.error("Error while setting resource base: "+base.getId() +"-"+ base.getTitle(), e);
}
if(!"".equals(base.getAttribute("template","").trim())) {
try {
tpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getFileFromWorkPath(base.getWorkPath() +"/"+ base.getAttribute("template").trim()));
path = webWorkPath;
}catch(Exception e) {
log.error("Error while loading resource template: "+base.getId(), e);
}
}
if( tpl==null ) {
try {
tpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getAdminFileStream("/swbadmin/xsl/Poll/Poll.xsl"));
}catch(Exception e) {
log.error("Error while loading default resource template: "+base.getId(), e);
}
}
}
/**
* Process request.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
@Override
public void processRequest(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
if(paramRequest.getMode().equals("showResults"))
doShowPollResults(request,response,paramRequest);
else if(paramRequest.getMode().equals("accesible"))
doAccesible(request, response, paramRequest);
else
super.processRequest(request, response, paramRequest);
}
/**
* Gets the dom.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @return the dom
* @throws SWBResourceException the sWB resource exception
*/
public Document getDom(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException {
Resource base=paramRequest.getResourceBase();
String title = base.getAttribute("header");
String imgTitle = base.getAttribute("imgencuesta");
String question = base.getAttribute("question");
String imgVote = base.getAttribute("button");
Display display;
try
{
display = Display.valueOf(base.getAttribute("display", Display.SLIDE.name()));
}
catch(Exception noe)
{
display = Display.POPUP;
}
SWBResourceURL url = paramRequest.getRenderUrl().setCallMethod(SWBParamRequest.Call_DIRECT);
url.setParameter("NombreCookie", "VotosEncuesta" + base.getId());
if(display==Display.SIMPLE) {
url.setMode("accesible");
}else {
url.setMode("showResults");
//url.setCallMethod(SWBParamRequest.Call_DIRECT);
}
Document dom = SWBUtils.XML.getNewDocument();
Element root = dom.createElement("poll");
root.setAttribute("action", url.toString());
root.setAttribute("id", "poll_"+base.getId());
dom.appendChild(root);
Element e;
if( title!=null ) {
e = dom.createElement("title");
e.appendChild(dom.createTextNode(title));
root.appendChild(e);
}
if( imgTitle!=null && !imgTitle.equals("null") ) {
e = dom.createElement("imgTitle");
e.setAttribute("path", path);
e.setAttribute("src", webWorkPath+base.getWorkPath()+"/"+imgTitle);
e.setAttribute("alt", base.getAttribute("header",question));
root.appendChild(e);
}
e = dom.createElement("question");
e.appendChild(dom.createTextNode(question));
root.appendChild(e);
Element options = dom.createElement("options");
root.appendChild(options);
Document domResource = SWBUtils.XML.xmlToDom(base.getXml());
NodeList nodes = domResource.getElementsByTagName("option");
for (int i = 0; i < nodes.getLength(); i++) {
e = dom.createElement("option");
e.setAttribute("value", "enc_votos"+(i+1));
e.setAttribute("id", "opc_"+base.getId()+"_"+i);
e.setAttribute("name","radiobutton");
e.appendChild(dom.createTextNode(nodes.item(i).getChildNodes().item(0).getNodeValue()));
options.appendChild(e);
}
e = dom.createElement("vote");
e.appendChild(dom.createTextNode(base.getAttribute("msg_tovote", paramRequest.getLocaleString("msg_tovote"))));
e.setAttribute("path", path);
if( imgVote!=null ) {
e.setAttribute("src", webWorkPath+base.getWorkPath()+"/"+imgVote);
e.setAttribute("alt", base.getAttribute("msg_tovote", paramRequest.getLocaleString("msg_tovote")));
}
e.setAttribute("label", base.getAttribute("msg_tovote",paramRequest.getLocaleString("msg_tovote")));
if( !Display.SIMPLE.toString().equals(display) ) {
boolean isCLIValidable = Boolean.parseBoolean(base.getAttribute("oncevote")) && VMode.COOKIE.ordinal()==Integer.parseInt(base.getAttribute("vmode", "0"));
e.setAttribute("action", "buscaCookie('VotosEncuesta"+base.getId()+"','radiobutton',"+isCLIValidable+",'"+url+"')");
}
root.appendChild(e);
e = dom.createElement("results");
e.setAttribute("title", base.getAttribute("msg_viewresults", paramRequest.getLocaleString("lblAdmin_msgResults").replaceAll("\"", "")));
if(display==Display.POPUP)
{
e.setAttribute("action", "abreResultados('"+url.toString(true)+"')");
}
else if(display==Display.SLIDE)
{
e.setAttribute("action", "postHtml('"+url.toString(true)+"','"+PREF+base.getId()+"'); expande();");
}
else
{
//e.setAttribute("action", "window.location.href='"+url.toString(true)+"'");
e.setAttribute("action", "postHtml('"+url.toString(true)+"','"+PREF+base.getId()+"')");
}
e.setAttribute("path", path);
e.setAttribute("title", paramRequest.getLocaleString("msgResults_title"));
e.appendChild(dom.createTextNode(base.getAttribute("msg_viewresults", paramRequest.getLocaleString("msg_viewresults"))));
root.appendChild(e);
if( LocLnks.INPOLL.toString().equals(base.getAttribute("wherelinks", "")) || LocLnks.INBOTH.toString().equals(base.getAttribute("wherelinks", "").trim()) ) {
Element links = dom.createElement("links");
root.appendChild(links);
nodes = domResource.getElementsByTagName("link");
if( nodes!=null ) {
for(int i=0; i-1 ) {
content = value.substring(idx + 1);
value = value.substring(0, idx);
}
e = dom.createElement("link");
e.setAttribute("url", value);
e.setAttribute("title", content);
e.appendChild(dom.createTextNode(content));
links.appendChild(e);
}
}
}
}
return dom;
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doXML(HttpServletRequest, HttpServletResponse, SWBParamRequest)
*/
@Override
public void doXML(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
Document dom=getDom(request, response, paramRequest);
if( dom!=null )
response.getWriter().println(SWBUtils.XML.domToXml(dom));
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doView(HttpServletRequest, HttpServletResponse, SWBParamRequest)
*/
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
Resource base = paramRequest.getResourceBase();
if(base.getAttribute("jspfile")!=null) {
String jspFile = base.getAttribute("jspfile");
request.setAttribute("paramRequest", paramRequest);
RequestDispatcher rd = request.getRequestDispatcher(jspFile);
try {
rd.include(request, response);
}catch(ServletException se) {
log.error(se);
}
}else {
response.setContentType("text/html; charset=iso-8859-1");
response.setHeader("Cache-Control","no-cache"); //HTTP 1.1
response.setHeader("Pragma","no-cache"); //HTTP 1.0
response.setDateHeader ("Expires", 0); //prevents caching at the proxy server
try {
Document dom = getDom(request, response, paramRequest);
Templates curtpl = tpl;
if(!"".equals(base.getAttribute("template","").trim())) {
try {
curtpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getFileFromWorkPath(base.getWorkPath() +"/"+ base.getAttribute("template").trim()));
path = webWorkPath;
}catch(Exception e) {
curtpl = tpl;
}
}
StringBuilder html = new StringBuilder(SWBUtils.XML.transformDom(curtpl, dom));
//html.append(getRenderScript(paramRequest));
Display display;
try {
display = Display.valueOf(base.getAttribute("display", Display.SLIDE.name()));
}catch(Exception noe) {
display = Display.POPUP;
}
//if(display==Display.SLIDE) {
if(display!=Display.POPUP) {
html.append(" ");
}
html.append(getRenderScript(paramRequest));
response.getWriter().println(html.toString());
}catch(TransformerException te) {
log.error(te);
}catch(Exception e) {
response.getWriter().println(paramRequest.getLocaleString("msgErrResource"));
}
}
}
/**
* Do accesible.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
public void doAccesible(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/html; charset=iso-8859-1");
response.setHeader("Cache-Control","no-cache"); //HTTP 1.1
response.setHeader("Pragma","no-cache"); //HTTP 1.0
StringBuilder html = new StringBuilder();
// try {
Document dom = getDom(request, response, paramRequest);
// html.append( SWBUtils.XML.transformDom(tpl,dom) );
vote(request, response, paramRequest);
dom = SWBUtils.XML.xmlToDom( getResourceBase().getData() );
html.append(getPollResults(request, paramRequest, dom));
// }catch(TransformerException te) {
// log.error("Error in a resource Poll while transforms the document. ", te);
// }
response.getWriter().println(html.toString());
}
/**
* Muestra el html al usuario final.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws IOException Signals that an I/O exception has occurred.
* @throws SWBResourceException the sWB resource exception
*/
/*
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/html; charset=iso-8859-1");
response.setHeader("Cache-Control","no-cache"); //HTTP 1.1
response.setHeader("Pragma","no-cache"); //HTTP 1.0
response.setDateHeader ("Expires", 0); //prevents caching at the proxy server
Resource base=getResourceBase();
//StringBuffer ret = new StringBuffer("");
PrintWriter out = response.getWriter();
//String action = null != request.getParameter("enc_act") && !"".equals(request.getParameter("enc_act").trim()) ? request.getParameter("enc_act").trim() : "enc_step1";
try
{
Document dom=SWBUtils.XML.xmlToDom(base.getXml());
if(dom!=null) {
NodeList node = dom.getElementsByTagName("option");
if (!"".equals(base.getAttribute("question", "").trim()) && node.getLength() > 1) {
if( base.getAttribute("cssClass")!=null && !base.getAttribute("cssClass").trim().equals("") ) {
out.println("");
}else {
out.println("");
}
if( base.getAttribute("header")!=null ) {
String style = "";
if( base.getAttribute("headerStyle")!=null ) {
style = "style=\""+base.getAttribute("headerStyle")+"\"";
}
out.println(""+base.getAttribute("header")+"
");
}
out.println("");
out.println("");
StringBuilder win = new StringBuilder();
win.append("menubar="+ base.getAttribute("menubar", "no"));
win.append(",toolbar="+ base.getAttribute("toolbar", "no"));
win.append(",status="+ base.getAttribute("status", "no"));
win.append(",location="+ base.getAttribute("location", "no"));
win.append(",directories="+ base.getAttribute("directories", "no"));
win.append(",scrollbars="+ base.getAttribute("scrollbars", "no"));
win.append(",resizable="+ base.getAttribute("resizable", "no"));
win.append(",width="+ base.getAttribute("width", "360"));
win.append(",height="+ base.getAttribute("height", "260"));
win.append(",top="+ base.getAttribute("top", "125"));
win.append(",left="+ base.getAttribute("left", "220"));
out.println("");
}
}
}catch (Exception e) {
log.error(paramRequest.getLocaleString("msgView_resource") +" "+ restype +" "+ paramRequest.getLocaleString("msgView_method"), e);
}
out.flush();
}*/
/**
* Muestra los resultados de la encuesta en especifico
* @param request
* @param response
* @param reqParams
* @throws AFException
* @throws IOException
*/
public void doShowPollResults(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/html; charset=iso-8859-1");
response.setHeader("Cache-Control","no-cache"); //HTTP 1.1
response.setHeader("Pragma","no-cache"); //HTTP 1.0
//synchronized(request){
//System.out.println("vote");
vote(request, response, paramRequest);
//}
Document dom = SWBUtils.XML.xmlToDom( getResourceBase().getData() );
response.getWriter().println( getPollResults(request, paramRequest, dom) );
}
/**
* Metodo que valida si se encuentra la cookie de la encuensta registrada en la maquina del usuario.
*
* @param request the request
* @return true, if successful
*/
private boolean validateCookie(javax.servlet.http.HttpServletRequest request) {
for(int i=0;i 0)
{
if(hashPrim.containsKey(actualIP)){
Timestamp ipdate=(Timestamp)hashPrim.get(actualIP);
if(ipdate.after(fctual)) {
return true; //No puede votar
}else{ //Despues de pasado el tiempo se elimina la ip, para que pueda votar y vuelva a pasar el tiempo definido para volver a podet votar
hashPrim.remove(actualIP);
}
}else {
hashPrim.put(request.getRemoteAddr(), Tfctualmoretime);
}
}else if (hashPrim.size() == 0)
{
hashPrim = new HashMap();
hashPrim.put(request.getRemoteAddr(), Tfctualmoretime);
}
return flag;
}
/**
* Vote.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
private void vote(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
Resource base = null;
String data = null;
Document dom = null;
if(request.getParameter("radiobutton")==null)
return;
base = getResourceBase();
synchronized(this) {
data = base.getData();
}
dom = SWBUtils.XML.xmlToDom(data);
boolean cliVoted = false;
String validateMode = base.getAttribute("vmode", VMode.IP.toString());
if( validateMode.equals(VMode.IP.toString()) ) {
cliVoted = validateIPAddress(request);
}else {
cliVoted = validateCookie(request);
//Pone cookie
MngCookie = new SWBCookieMgr();
//String value = (String) MngCookie.SearchCookie("VotosEncuesta"+ base.getId(), request);
MngCookie.AddCookie("VotosEncuesta"+ base.getId(), "SI", true, true, request, response);
}
boolean voteOnce = Boolean.parseBoolean(base.getAttribute("oncevote", "true"));
if( !voteOnce || !cliVoted) { // Es un usuario que paso la prueba de las IPs
int valor = 0;
try {
valor = Integer.parseInt(request.getParameter("radiobutton").substring(9));
}catch(Exception e) {
valor=0;
log.error("Respuesta de encuesta en cero. ", e);
}
if(valor > 0) {
String varia = "enc_votos";
if (data == null) {
try {
dom = SWBUtils.XML.getNewDocument();
Element root = dom.createElement("resource");
dom.appendChild(root);
Element option = dom.createElement(varia + valor);
option.appendChild(dom.createTextNode("1"));
root.appendChild(option);
synchronized(this) {
base.setData(SWBUtils.XML.domToXml(dom));
}
}catch (Exception e) {
log.error(paramRequest.getLocaleString("msgView_setData") +" "+ restype +" " + paramRequest.getLocaleString("msgView_id") +" "+ base.getId() +" - "+ base.getTitle(), e);
}
}else {
try {
NodeList nlist = dom.getElementsByTagName(varia + valor);
boolean exist = false;
for (int i = 0; i < nlist.getLength(); i++) {
exist = true;
try {
int votosOption = Integer.parseInt(nlist.item(i).getChildNodes().item(0).getNodeValue());
votosOption = votosOption + 1;
nlist.item(i).getChildNodes().item(0).setNodeValue(String.valueOf(votosOption));
}catch(NumberFormatException nfe) {
log.error("La opción está guardando un valor no numérico.\n" + nfe);
}
}
if(!exist) {
Node nres = dom.getFirstChild();
Element option = dom.createElement(varia + valor);
option.appendChild(dom.createTextNode("1"));
nres.appendChild(option);
}
synchronized(this) {
base.setData(SWBUtils.XML.domToXml(dom));
}
}
catch (Exception e) {
log.error(paramRequest.getLocaleString("msgView_setData") +" "+ restype +" " + paramRequest.getLocaleString("msgView_id") +" "+ base.getId() +" - "+ base.getTitle(), e);
}finally {
base.addHit(request, paramRequest.getUser(), paramRequest.getWebPage());
}
}
}
}
}
/**
* Muestra los resultados de la encuesta.
*
* @param request the request
* @param paramRequest the param request
* @param data the data
* @return the poll results
* @throws IOException Signals that an I/O exception has occurred.
* @throws SWBResourceException the sWB resource exception
*/
private String getPollResults(HttpServletRequest request, SWBParamRequest paramRequest, Document data) throws SWBResourceException, IOException {
StringBuilder ret = new StringBuilder();
Resource base = getResourceBase();
Display display;
try
{
display = Display.valueOf(base.getAttribute("display", Display.SLIDE.name()));
}catch(Exception noe)
{
display = Display.POPUP;
}
Document dom = SWBUtils.XML.xmlToDom(base.getXml());
if(dom==null) {
return ret.toString();
}
String backimgres;
NodeList node = dom.getElementsByTagName("backimgres");
if(node!=null && node.getLength()>0)
backimgres = "style=\"background-image:url("+webWorkPath+base.getWorkPath()+"/"+node.item(0).getChildNodes().item(0).getNodeValue()+");\"";
else
backimgres = "";
if(display==Display.POPUP) {
ret.append("");
ret.append("");
ret.append("");
ret.append("" + paramRequest.getLocaleString("msgResults_title") + " ");
ret.append("");
ret.append("");
ret.append("");
}
ret.append("\n");
ret.append(""+paramRequest.getLocaleString("msgResults_title")+"
\n");
ret.append("\n");
node = dom.getElementsByTagName("option");
if( !"".equals(base.getAttribute("question", "").trim()) && node.getLength()>0 )
{
ret.append("" + base.getAttribute("question").trim() + " \n");
ret.append(" \n");
if (data != null)
{
//Suma el total de votos para calcular el porcentaje
long intTotalVotos = 0;
long intAjuste = 0;
Node nodoFC = data.getFirstChild();
NodeList nlOption = nodoFC.getChildNodes();
for (int j = 0; j < nlOption.getLength(); j++)
{
if (nlOption.item(j).getChildNodes().getLength() > 0)
{
intTotalVotos = intTotalVotos + Integer.parseInt(nlOption.item(j).getChildNodes().item(0).getNodeValue());
Integer votos = new Integer(nlOption.item(j).getChildNodes().item(0).getNodeValue());
intAjuste = intAjuste + ((votos.longValue() * 100) / intTotalVotos);
}
}
if (intAjuste > 0) {
intAjuste = 100 - intAjuste;
}
long intVotos = 0;
float intPorcentaje = 0;
float largo = 0;
boolean porcent = Boolean.valueOf(base.getAttribute("porcent","true")).booleanValue();
boolean totvote = Boolean.valueOf(base.getAttribute("totvote","true")).booleanValue();
for (int i = 0; i < node.getLength(); i++) {
int num = i + 1;
ret.append("\n");
ret.append(" "+node.item(i).getChildNodes().item(0).getNodeValue()+" \n");
String varia = "enc_votos";
NodeList nlist = data.getElementsByTagName(varia + num);
for (int j = 0; j < nlist.getLength(); j++)
{
String key = nlist.item(j).getNodeName();
String nume = key.substring(9);
Integer votos = new Integer(nlist.item(j).getChildNodes().item(0).getNodeValue());
intVotos = votos.intValue();
intPorcentaje = ((float) votos.intValue() * 100) / (float) intTotalVotos;
intPorcentaje = (intPorcentaje * 10);
intPorcentaje += .5;
intPorcentaje = (int) intPorcentaje;
intPorcentaje = intPorcentaje / 10;
if (Integer.parseInt(nume) == num) {
largo = intPorcentaje;
ret.append(" \n");
ret.append(" \n");
if (porcent) {
ret.append(""+largo+"% \n");
}
if (porcent && totvote) {
ret.append(" : ");
}
if (totvote) {
ret.append(""+intVotos+" "+base.getAttribute("msg_vote",paramRequest.getLocaleString("msg_vote"))+"(s) \n");
}
ret.append(" \n");
ret.append(" \n");
}
}
}
ret.append(" \n");
intAjuste = 0;
if (totvote) {
ret.append(" \n");
ret.append(""+ base.getAttribute("msg_totvotes",paramRequest.getLocaleString("msg_totvotes")) + ": " + intTotalVotos + " \n");
ret.append(" \n");
}
}else {
ret.append("" + paramRequest.getLocaleString("msgResults_noVotes") +" \n");
}
ret.append("
\n");
ret.append(" \n");
ret.append(" \n");
if( LocLnks.INRESULTS.toString().equals(base.getAttribute("wherelinks")) || LocLnks.INBOTH.toString().equals(base.getAttribute("wherelinks")) )
ret.append(getLinks(dom.getElementsByTagName("link"), paramRequest.getLocaleString("usrmsg_Encuesta_doView_relatedLink"))+" \n");
if(display==Display.POPUP)
ret.append("" + base.getAttribute("msg_closewin",paramRequest.getLocaleString("msg_closewin")) + "
\n");
else if( display == Display.SIMPLE )
ret.append("" + base.getAttribute("msg_closewin",paramRequest.getLocaleString("msg_closewin")) + "
\n");
else
ret.append("" + base.getAttribute("msg_closewin",paramRequest.getLocaleString("msg_closewin")) + "
\n");
ret.append(" \n");
}
if(display==Display.POPUP) {
ret.append(" \n");
ret.append(" \n");
}
return ret.toString();
}
/**
* Gets the links.
*
* @param links the links
* @param genDesc the gen desc
* @return the links
*/
private String getLinks(NodeList links, final String genDesc) {
StringBuilder ret = new StringBuilder("");
if( links==null )
return ret.toString();
String ownDesc = genDesc;
for (int i = 0; i < links.getLength(); i++) {
String value = links.item(i).getChildNodes().item(0).getNodeValue().trim();
if( !"".equals(value) ) {
//int idx = value.indexOf("/wblink/");
int idx = value.indexOf(",");
if( idx>-1 ) {
ownDesc = value.substring(idx + 1);
value = value.substring(0, idx);
}
ret.append(" ");
ownDesc=genDesc;
}
}
return ret.toString();
}
/**
* Metodo que despliega la administraci?n del recurso.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @throws IOException Signals that an I/O exception has occurred.
* @throws SWBResourceException the sWB resource exception
*/
@Override
public void doAdmin(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
PrintWriter out = response.getWriter();
Resource base=getResourceBase();
String msg=paramRequest.getLocaleString("lblAdmin_undefinedOperation");
String action = null != request.getParameter("act") && !"".equals(request.getParameter("act").trim()) ? request.getParameter("act").trim() : paramRequest.getAction();
if(action.equals("add") || action.equals("edit")) {
out.println(getForm(request, paramRequest));
}else if(action.equals("update")) {
FileUpload fup = new FileUpload();
try
{
fup.getFiles(request, response);
String value = null != fup.getValue("question") && !"".equals(fup.getValue("question").trim()) ? fup.getValue("question").trim() : null;
String option = null != fup.getValue("option") && !"".equals(fup.getValue("option").trim()) ? fup.getValue("option").trim() : null;
if (value!=null && option!=null)
{
base.setAttribute("question", value);
value = null != fup.getValue("noimgencuesta") && !"".equals(fup.getValue("noimgencuesta").trim()) ? fup.getValue("noimgencuesta").trim() : "0";
if ("1".equals(value) && !"".equals(base.getAttribute("imgencuesta", "").trim()))
{
SWBUtils.IO.removeDirectory(workPath+base.getAttribute("imgencuesta").trim());
base.removeAttribute("imgencuesta");
}
else
{
value = null != fup.getFileName("imgencuesta") && !"".equals(fup.getFileName("imgencuesta").trim()) ? fup.getFileName("imgencuesta").trim() : null;
if (value!=null)
{
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals(""))
{
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")){
msg=paramRequest.getLocaleString("msgErrFileType") +" jpg, jpeg, gif, png: " + file;
}
else
{
if (admResUtils.uploadFile(base, fup, "imgencuesta")){
base.setAttribute("imgencuesta", file);
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
value = null != fup.getValue("jspfile") && !"".equals(fup.getValue("jspfile").trim()) ? fup.getValue("jspfile").trim() : null;
if(value!=null)
base.setAttribute("jspfile",value);
else
base.removeAttribute("jspfile");
value = null != fup.getValue("notemplate") && !"".equals(fup.getValue("notemplate").trim()) ? fup.getValue("notemplate").trim() : "0";
if ("1".equals(value) && !"".equals(base.getAttribute("template", "").trim())) {
SWBUtils.IO.removeDirectory(workPath+base.getAttribute("template").trim());
base.removeAttribute("template");
}else {
value = null != fup.getFileName("template") && !"".equals(fup.getFileName("template").trim()) ? fup.getFileName("template").trim() : null;
if (value!=null)
{
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals(""))
{
if (!admResUtils.isFileType(file, "xsl|xslt")){
msg=paramRequest.getLocaleString("msgErrFileType") +" xsl, xslt: " + file;
}else {
if (admResUtils.uploadFile(base, fup, "template")){
base.setAttribute("template", file);
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
value = null != fup.getValue("noimg_button") && !"".equals(fup.getValue("noimg_button").trim()) ? fup.getValue("noimg_button").trim() : "0";
if ("1".equals(value) && !"".equals(base.getAttribute("button", "").trim()))
{
SWBUtils.IO.removeDirectory(workPath+base.getAttribute("button").trim());
base.removeAttribute("button");
}
else
{
value = null != fup.getFileName("button") && !"".equals(fup.getFileName("button").trim()) ? fup.getFileName("button").trim() : null;
if (value!=null)
{
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals(""))
{
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")) {
msg=paramRequest.getLocaleString("msgErrFileType") +" jpg, jpeg, gif, png: " + file;
}
else
{
if (admResUtils.uploadFile(base, fup, "button")) {
base.setAttribute("button", file);
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
value = null != fup.getValue("nobackimgres") && !"".equals(fup.getValue("nobackimgres").trim()) ? fup.getValue("nobackimgres").trim() : "0";
if ("1".equals(value) && !"".equals(base.getAttribute("backimgres", "").trim()))
{
SWBUtils.IO.removeDirectory(workPath+base.getAttribute("backimgres").trim());
base.removeAttribute("backimgres");
}
else
{
value = null != fup.getFileName("backimgres") && !"".equals(fup.getFileName("backimgres").trim()) ? fup.getFileName("backimgres").trim() : null;
if (value!=null)
{
String file = admResUtils.getFileName(base, value);
if (file != null && !file.trim().equals(""))
{
if (!admResUtils.isFileType(file, "jpg|jpeg|gif|png")) {
msg=paramRequest.getLocaleString("msgErrFileType") +" jpg, jpeg, gif, png: " + file;
}
else
{
if (admResUtils.uploadFile(base, fup, "backimgres")){
base.setAttribute("backimgres", file);
}
else {
msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
}
else msg=paramRequest.getLocaleString("msgErrUploadFile") +" " + value + ".";
}
}
value = null != fup.getValue("time") && !"".equals(fup.getValue("time").trim()) ? fup.getValue("time").trim() : "20";
base.setAttribute("time", value);
setAttribute(base, fup, "wherelinks");
setAttribute(base, fup, "oncevote");
setAttribute(base, fup, "vmode");
setAttribute(base, fup, "display");
setAttribute(base, fup, "porcent");
setAttribute(base, fup, "totvote");
setAttribute(base, fup, "menubar", "yes");
setAttribute(base, fup, "toolbar", "yes");
setAttribute(base, fup, "status", "yes");
setAttribute(base, fup, "location", "yes");
setAttribute(base, fup, "directories", "yes");
setAttribute(base, fup, "scrollbars", "yes");
setAttribute(base, fup, "resizable", "yes");
setAttribute(base, fup, "width");
setAttribute(base, fup, "height");
setAttribute(base, fup, "top");
setAttribute(base, fup, "left");
setAttribute(base, fup, "msg_viewresults");
setAttribute(base, fup, "msg_vote");
setAttribute(base, fup, "msg_closewin");
setAttribute(base, fup, "msg_totvotes");
setAttribute(base, fup, "msg_tovote");
setAttribute(base, fup, "cssClass");
setAttribute(base, fup, "header");
base.updateAttributesToDB();
Document dom=SWBUtils.XML.xmlToDom(base.getXml());
if(dom!=null) {
removeAllNodes(dom, Node.ELEMENT_NODE, "option");
}else {
dom = SWBUtils.XML.getNewDocument();
Element root = dom.createElement("resource");
dom.appendChild(root);
}
value = null != fup.getValue("option") && !"".equals(fup.getValue("option").trim()) ? fup.getValue("option").trim() : null;
if(value!=null)
{
StringTokenizer stk = new StringTokenizer(value, "|");
while (stk.hasMoreTokens())
{
value = stk.nextToken();
Element emn = dom.createElement("option");
emn.appendChild(dom.createTextNode(value));
dom.getFirstChild().appendChild(emn);
}
}
removeAllNodes(dom, Node.ELEMENT_NODE, "link");
value = null != fup.getValue("link") && !"".equals(fup.getValue("link").trim()) ? fup.getValue("link").trim() : null;
if(value!=null)
{
StringTokenizer stk = new StringTokenizer(value, "|");
while (stk.hasMoreTokens())
{
value = stk.nextToken();
Element emn = dom.createElement("link");
emn.appendChild(dom.createTextNode(value));
dom.getFirstChild().appendChild(emn);
}
}
base.setXml(SWBUtils.XML.domToXml(dom));
msg=paramRequest.getLocaleString("msgOkUpdateResource") +" "+ base.getId();
}else {
msg=paramRequest.getLocaleString("msgDataRequired");
}
}catch(Exception e) {
log.error(e);
msg=paramRequest.getLocaleString("msgErrUpdateResource") +" "+ base.getId();
}finally {
out.println("");
out.println("");
}
}
else if(action.equals("remove"))
{
msg=admResUtils.removeResource(base);
out.println("");
}
out.flush();
}
/**
* Metodo que muestra la forma de la encuesta de opini?n en html.
*
* @param request the request
* @param paramRequest the param request
* @return the form
*/
private String getForm(javax.servlet.http.HttpServletRequest request, SWBParamRequest paramRequest) {
StringBuilder ret=new StringBuilder();
Resource base=getResourceBase();
try
{
SWBResourceURL url = paramRequest.getRenderUrl().setMode(paramRequest.Mode_ADMIN);
url.setAction("update");
ret.append("");
ret.append(" ");
ret.append("");
ret.append(getAdminScript(paramRequest));
}
catch(Exception e) {
log.error(e);
}
return ret.toString();
}
/**
* Sets the attribute.
*
* @param base the base
* @param fup the fup
* @param att the att
*/
protected void setAttribute(Resource base, FileUpload fup, String att) {
try
{
if(null != fup.getValue(att) && !"".equals(fup.getValue(att).trim())) {
base.setAttribute(att, fup.getValue(att).trim());
}
else {
base.removeAttribute(att);
}
}
catch(Exception e) { log.error("Error while setting resource attribute: "+att + ", "+base.getId() +"-"+ base.getTitle(), e); }
}
/**
* Sets the attribute.
*
* @param base the base
* @param fup the fup
* @param att the att
* @param value the value
*/
protected void setAttribute(Resource base, FileUpload fup, String att, String value) {
try
{
if(null != fup.getValue(att) && value.equals(fup.getValue(att).trim())) {
base.setAttribute(att, fup.getValue(att).trim());
}
else {
base.removeAttribute(att);
}
}
catch(Exception e) { log.error("Error while setting resource attribute: "+att + ", "+base.getId() +"-"+ base.getTitle(), e); }
}
/**
* Removes the all nodes.
*
* @param dom the dom
* @param nodeType the node type
* @param name the name
*/
private void removeAllNodes(Document dom, short nodeType, String name) {
NodeList list = dom.getElementsByTagName(name);
for (int i = 0; i < list.getLength(); i++)
{
Node node=list.item(i);
if (node.getNodeType() == nodeType)
{
node.getParentNode().removeChild(node);
if(node.hasChildNodes()) {
removeAllNodes(dom, nodeType, name);
}
}
}
}
/**
* Metodo de validaci?n en javascript para la encuesta.
*
* @param paramRequest the param request
* @return the admin script
* @throws SWBResourceException the sWB resource exception
*/
private String getAdminScript(SWBParamRequest paramRequest) throws SWBResourceException {
Locale locale = new Locale(paramRequest.getUser().getLanguage());
StringBuilder script = new StringBuilder();
script.append("\n");
return script.toString();
}
/**
* Gets the render script.
*
* @param paramRequest the param request
* @return the render script
* @throws SWBResourceException the sWB resource exception
*/
private String getRenderScript(SWBParamRequest paramRequest) throws SWBResourceException {
Resource base = paramRequest.getResourceBase();
StringBuilder script = new StringBuilder();
StringBuilder win = new StringBuilder();
win.append("menubar=" + base.getAttribute("menubar", "no"));
win.append(",toolbar=" + base.getAttribute("toolbar", "no"));
win.append(",status=" + base.getAttribute("status", "no"));
win.append(",location=" + base.getAttribute("location", "no"));
win.append(",directories=" + base.getAttribute("directories", "no"));
win.append(",scrollbars=" + base.getAttribute("scrollbars", "no"));
win.append(",resizable=" + base.getAttribute("resizable", "no"));
win.append(",width=" + base.getAttribute("width", "360"));
win.append(",height=" + base.getAttribute("height", "260"));
win.append(",top=" + base.getAttribute("top", "125"));
win.append(",left=" + base.getAttribute("left", "220"));
script.append("\n");
return script.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy