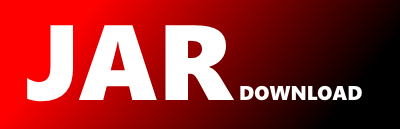
org.semanticwb.portal.resources.Promo Maven / Gradle / Ivy
Show all versions of SWBPortal Show documentation
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Iterator;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.transform.Templates;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.semanticwb.Logger;
import org.semanticwb.SWBPlatform;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBUtils;
import org.semanticwb.model.Resource;
import org.semanticwb.portal.api.GenericResource;
import org.semanticwb.portal.api.SWBActionResponse;
import org.semanticwb.portal.api.SWBParamRequest;
import org.semanticwb.portal.api.SWBResourceException;
import org.semanticwb.portal.api.SWBResourceURL;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
// TODO: Auto-generated Javadoc
/**
* Promo se encarga de desplegar y administrar un texto promocional con una
* imagen opcional bajo ciertos criterios(configuraci?n de recurso). Es un recurso
* que viene de la versi?n 2 de WebBuilder y puede ser instalado como recurso de
* estrategia o recurso de contenido.
*
* Promo displays and administrate a promocional text with an optional image
* under criteria like configuration. This resource comes from WebBuilder 2 and can
* be installed as content resource or a strategy resource.
*
* @Autor:Jorge Jiménez,Carlos Ramos
*/
public class Promo extends GenericResource {
/** The log. */
private static Logger log = SWBUtils.getLogger(Promo.class);
/** The tpl. */
private Templates tpl;
/** The work path. */
private String workPath;
/** The web work path. */
private String webWorkPath;
/** The path. */
private String path = SWBPlatform.getContextPath() +"/swbadmin/xsl/Promo/";
/** The restype. */
private static String restype;
private static final String Action_UPDATE = "update";
/**
* Sets the resource base.
*
* @param base the new resource base
*/
@Override
public void setResourceBase(Resource base) {
try {
super.setResourceBase(base);
workPath = SWBPortal.getWorkPath()+base.getWorkPath()+"/";
webWorkPath = SWBPortal.getWebWorkPath()+base.getWorkPath()+"/";
restype = base.getResourceType().getResourceClassName();
}catch(Exception e) {
log.error("Error while setting resource base: "+base.getId() +"-"+ base.getTitle(), e);
}
// if(!"".equals(base.getAttribute("template","").trim())) {
// try {
// tpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getFileFromWorkPath(base.getWorkPath() +"/"+ base.getAttribute("template").trim()));
// path = webWorkPath;
// }catch(Exception e) {
// log.error("Error while loading resource template: "+base.getId(), e);
// }
// }
// if( tpl==null || Boolean.parseBoolean(base.getAttribute("deftmp"))) {
try {
tpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getAdminFileStream("/swbadmin/xsl/Promo/PromoRightAligned.xsl"));
}catch(Exception e) {
log.error("Error while loading default resource template: "+base.getId(), e);
}
// }
}
/**
* Gets the dom.
*
* @param request the request
* @param response the response
* @param paramRequest the param request
* @return the dom
* @throws SWBResourceException the sWB resource exception
*/
public Document getDom(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException {
Resource base=paramRequest.getResourceBase();
if(base.getAttribute("imgfile")==null)
throw new SWBResourceException(paramRequest.getLocaleString("msgErrResource"));
Document dom = SWBUtils.XML.getNewDocument();
Element root = dom.createElement("promo");
root.setAttribute("id", "promo_"+base.getId());
root.setAttribute("width", base.getAttribute("width",""));
root.setAttribute("height", base.getAttribute("height",""));
dom.appendChild(root);
Element e;
e = dom.createElement("title");
e.appendChild(dom.createTextNode( base.getAttribute("title","") ));
root.appendChild(e);
e = dom.createElement("subtitle");
e.appendChild(dom.createTextNode( base.getAttribute("subtitle","") ));
root.appendChild(e);
Element img = dom.createElement("image");
img.setAttribute("width", base.getAttribute("imgWidth","90"));
img.setAttribute("height", base.getAttribute("imgHeight","140"));
img.setAttribute("src", webWorkPath+base.getAttribute("imgfile"));
img.setAttribute("alt", base.getAttribute("caption",base.getAttribute("title","image promo")));
img.setAttribute("url", base.getAttribute("url",""));
root.appendChild(img);
e = dom.createElement("imageFoot");
e.appendChild(dom.createTextNode( base.getAttribute("caption","") ));
img.appendChild(e);
e = dom.createElement("content");
e.setAttribute("url", base.getAttribute("url",""));
e.appendChild(dom.createTextNode(base.getAttribute("text","")));
root.appendChild(e);
e = dom.createElement("more");
e.setAttribute("url", base.getAttribute("url",""));
e.setAttribute("target", base.getAttribute("target","true"));
e.appendChild(dom.createTextNode(base.getAttribute("more","")));
root.appendChild(e);
return dom;
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericResource#doXML(HttpServletRequest, HttpServletResponse, SWBParamRequest)
*/
@Override
public void doXML(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/xml; charset=ISO-8859-1");
response.setHeader("Cache-Control","no-cache");
response.setHeader("Pragma","no-cache");
Resource base=getResourceBase();
PrintWriter out = response.getWriter();
try {
Document dom = getDom(request, response, paramRequest);
out.println(SWBUtils.XML.domToXml(dom));
}catch(Exception e) {
log.error("Error in doXML method while rendering the XML script: "+base.getId()+"-"+base.getTitle(), e);
out.println("Error in doXML method while rendering the XML script");
}
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericAdmResource#doView(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
*/
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/html; charset=ISO-8859-1");
Resource base = paramRequest.getResourceBase();
PrintWriter out = response.getWriter();
if(base.getAttribute("template")!=null || Boolean.parseBoolean(base.getAttribute("deftmp"))) {
Templates curtpl;
try {
curtpl = SWBUtils.XML.loadTemplateXSLT(SWBPortal.getFileFromWorkPath(base.getWorkPath() +"/"+ base.getAttribute("template").trim()));
path = webWorkPath;
}catch(Exception e) {
curtpl = tpl;
}
try {
Document dom = getDom(request, response, paramRequest);
String html = SWBUtils.XML.transformDom(curtpl, dom);
out.println(html);
}catch(SWBResourceException swbe) {
out.println(swbe.getMessage());
}catch(Exception e) {
log.error("Error in doView method while rendering the resource base: "+base.getId() +"-"+ base.getTitle(), e);
}
}else {
try {
if(base.getAttribute("cssClass")==null) {
out.println(renderWithStyle(paramRequest));
}else {
out.println(render(paramRequest));
}
}catch(SWBResourceException swbe) {
out.println(swbe.getMessage());
}
}
}
/**
* Render with style.
*
* @return the string
*/
private String renderWithStyle(SWBParamRequest paramRequest) throws SWBResourceException {
StringBuilder out = new StringBuilder();
Resource base=getResourceBase();
int width;
try {
width = Integer.parseInt(base.getAttribute("width","0"));
}catch(NumberFormatException nfe) {
width = 0;
}
int height;
try {
height = Integer.parseInt(base.getAttribute("height","0"));
}catch(NumberFormatException nfe) {
height = 0;
}
String textcolor = base.getAttribute("textcolor");
String title = base.getAttribute("title");
String titleStyle = base.getAttribute("titleStyle");
String subtitle = base.getAttribute("subtitle");
String subtitleStyle = base.getAttribute("subtitleStyle");
if(base.getAttribute("imgfile")==null)
throw new SWBResourceException(paramRequest.getLocaleString("msgErrResource"));
String imgfile = base.getAttribute("imgfile");
String caption = base.getAttribute("caption");
String captionStyle = base.getAttribute("captionStyle");
String imgWidth="";
if(base.getAttribute("imgWidth")!=null){
imgWidth = "width=\"" + base.getAttribute("imgWidth")+"\"";
}
String imgHeight="";
if(base.getAttribute("imgHeight")!=null){
imgHeight = "height=\"" + base.getAttribute("imgHeight")+"\"";
}
String text = base.getAttribute("text","");
String textStyle = base.getAttribute("textStyle");
String more = base.getAttribute("more","");
String moreStyle = base.getAttribute("moreStyle");
String url = base.getAttribute("url");
String uline = base.getAttribute("uline", "0");
boolean target = Boolean.parseBoolean(base.getAttribute("target"));
int imgPos;
try {
imgPos = Integer.parseInt(base.getAttribute("imgPos","1"));
}catch(NumberFormatException nfe) {
imgPos = 1;
}
try {
//marco
out.append("0 || height>0) {
out.append(" style=\""+(textcolor==null?"":"color:"+textcolor+";")+(width>0?"width:"+width+"px;":"")+(height>0?"height:"+height+"px;":"")+"\"");
}
out.append(">");
//title
if(title != null) {
out.append(" \n");
out.append(title);
out.append("
\n");
}
boolean hasLink = base.getAttribute("more")!=null && url!=null;
//image
String margin = "";
StringBuilder img = new StringBuilder("");
if(imgfile != null) {
if(imgPos == 3) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append("\n");
margin = "margin-right:"+(imgWidth+20)+"px;";
out.append(img);
}else if(imgPos == 4) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append("\n");
margin = "margin-left:"+(imgWidth+20)+"px;";
out.append(img);
}else if(imgPos == 2) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append("\n");
out.append(img);
}else if(imgPos == 1) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append("\n");
out.append(img);
}else if(imgPos == 5) {
img.append(" \n");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append(" \n");
out.append(img);
}else {
imgPos = 6;
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink) {
img.append("");
}
img.append("\n");
if(caption != null) {
img.append(""+caption+"
\n");
}
img.append("\n");
}
}
//subtitle
if(subtitle != null) {
out.append(""+subtitle+"
\n");
}
if(hasLink) {
out.append("");
out.append("");
out.append(text);
out.append("");
out.append("
\n");
out.append("- ");
out.append("");
out.append(more);
out.append("");
out.append("
\n");
}else {
out.append("");
if(url != null) {
out.append("");
out.append(text);
out.append("\n");
}else {
out.append("");
out.append(text);
out.append("\n");
}
out.append("
\n");
}
if( imgfile!=null && imgPos==6 ) {
out.append(img);
}
out.append("");
}catch (Exception e) {
log.error("Error while setting resource base: "+base.getId() +"-"+ base.getTitle(), e);
}
return out.toString();
}
/**
* Render.
*
* @return the string
*/
private String render(SWBParamRequest paramRequest) throws SWBResourceException {
StringBuilder out = new StringBuilder();
Resource base=getResourceBase();
int width;
try {
width = Integer.parseInt(base.getAttribute("width","0"));
}catch(NumberFormatException nfe) {
width = 0;
}
int height;
try {
height = Integer.parseInt(base.getAttribute("height","0"));
}catch(NumberFormatException nfe) {
height = 0;
}
String title = base.getAttribute("title");
String subtitle = base.getAttribute("subtitle");
if(base.getAttribute("imgfile")==null)
throw new SWBResourceException(paramRequest.getLocaleString("msgErrResource"));
String imgfile = base.getAttribute("imgfile");
String caption = base.getAttribute("caption");
String imgWidth="";
if(base.getAttribute("imgWidth")!=null){
imgWidth = "width=\"" + base.getAttribute("imgWidth")+"\"";
}
String imgHeight="";
if(base.getAttribute("imgHeight")!=null){
imgHeight = "height=\"" + base.getAttribute("imgHeight")+"\"";
}
String text = base.getAttribute("text","");
String more = base.getAttribute("more","");
String url = base.getAttribute("url");
String uline = base.getAttribute("uline", "0");
boolean target = Boolean.parseBoolean(base.getAttribute("target"));
String cssClass = base.getAttribute("cssClass","");
int imgPos;
try {
imgPos = Integer.parseInt(base.getAttribute("imgPos","1"));
}catch(NumberFormatException nfe) {
imgPos = 1;
}
try {
//marco
out.append("");
//title
if(title != null) {
out.append(" \n");
out.append(title);
out.append("
\n");
}
//image
String margin = "";
StringBuilder img = new StringBuilder("");
if(imgfile != null) {
boolean hasLink = base.getAttribute("more")==null && url!=null;
if(imgPos == 3) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append("\n");
margin = "margin-right:"+(imgWidth+20)+"px;";
out.append(img);
}else if(imgPos == 4) {
img.append("");
img.append("");
if(hasLink){
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append("\n");
margin = "margin-left:"+(imgWidth+20)+"px;";
out.append(img);
}else if(imgPos == 2) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append("\n");
out.append(img);
}else if(imgPos == 1) {
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append("\n");
out.append(img);
}else if(imgPos == 5) {
img.append(" \n");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append(" \n");
out.append(img);
}else {
imgPos = 6;
img.append("");
img.append("");
if(hasLink) {
img.append("");
}
img.append("
");
if(hasLink)
img.append("");
img.append("\n");
if(caption != null)
img.append(""+caption+"
\n");
img.append("\n");
}
}
//subtitle
if(subtitle != null) {
out.append(""+subtitle+"
\n");
}
if( base.getAttribute("more")==null ) {
//texto
out.append("");
if(url != null) {
out.append("");
out.append(text);
out.append("\n");
}else {
out.append("");
out.append(text);
out.append("\n");
}
out.append("
\n");
}else {
out.append("");
out.append("");
out.append(text);
out.append("");
out.append("
\n");
//más...
if(url!=null) {
out.append("- ");
out.append("");
out.append(more);
out.append("");
out.append("
\n");
}
}
if( imgfile!=null && imgPos==6 ) {
out.append(img);
}
//marco
out.append("");
}catch (Exception e) {
log.error("Error while setting resource base: "+base.getId() +"-"+ base.getTitle(), e);
}
return out.toString();
}
/**
* Obtiene las ligas de redireccionamiento del promocional.
*
* @param paramRequest the param request
* @param base the base
* @return the url html
*/
// private String getUrlHtml(SWBParamRequest paramRequest, Resource base) {
// StringBuffer ret = new StringBuffer("");
// SWBResourceURL wburl = paramRequest.getActionUrl();
//
// ret.append(" \n");
// return ret.toString();
// }
/**
* Obtiene la imagen del promocional asi como su posicionamiento (en caso de
* existir).
*
* @param reqParams the req params
* @param base the base
* @return the img promo
*/
// private String getImgPromo(SWBParamRequest reqParams, Resource base) {
// StringBuilder ret = new StringBuilder("");
// String position = base.getAttribute("pos", "3").trim();
// String img=base.getAttribute("img", "").trim();
// String url=base.getAttribute("url", "").trim();
//
// if("1".equals(position)) {
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"left\" vspace=\"1\" hspace=\"5\" /> \n");
// }
// ret.append(getTextHtml(base));
// }else if("2".equals(position)) {
// ret.append(getTextHtml(base));
// if(!"".equals(url)) {
// ret.append(" \n");
// }
// ret.append(" \n");
// ret.append(" \n");
// if(!"".equals(url)) {
// ret.append(getUrlHtml(reqParams, base));
// }
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"left\" vspace=\"1\" hspace=\"5\" /> \n");
// }
// }else if("3".equals(position)) {
// ret.append(" \n");
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"left\" /> \n");
// }
// ret.append(" \n");
// if(!"".equals(url)) {
// ret.append(" \n");
// }
// ret.append(" \n");
// ret.append(" \n");
// ret.append(" \n");
// ret.append(" \n");
// if(!"".equals(url)) {
// ret.append(getUrlHtml(reqParams, base));
// }
// ret.append(getTextHtml(base));
// }else if ("4".equals(position)) {
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"right\" vspace=\"1\" hspace=\"10\" /> \n");
// }
// ret.append(getTextHtml(base));
// }else if("5".equals(position)) {
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" /> \n");
// }
// if(!"".equals(url)) {
// ret.append(" \n");
// }
// ret.append(" \n");
// ret.append(" \n");
// if(!"".equals(url)) {
// ret.append(getUrlHtml(reqParams, base));
// }
// ret.append(getTextHtml(base));
// }else if("6".equals(position)) {
// ret.append(getTextHtml(base));
// if(!"".equals(url)) {
// ret.append(" \n");
// }
// ret.append(" \n");
// ret.append(" \n");
// ret.append(" \n");
// ret.append(" \n");
// if(!"".equals(url)) {
// ret.append(getUrlHtml(reqParams, base));
// }
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" /> \n");
// }
// }else if("7".equals(position)) {
// ret.append(getTextHtml(base));
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"left\" vspace=\"1\" hspace=\"5\" /> \n");
// }
// }else if("8".equals(position)) {
// ret.append(getTextHtml(base));
// ret.append(getImgHtml(reqParams, base));
// if(!img.endsWith(".swf")) {
// ret.append(" align=\"right\" vspace=\"1\" hspace=\"10\" /> \n");
// }
// }
// return ret.toString();
// }
/**
* Obtiene el html de la imagen.
*
* @param paramRequest the param request
* @param base the base
* @return the img html
*/
// private String getImgHtml(SWBParamRequest paramRequest, Resource base) {
// StringBuilder ret = new StringBuilder("");
// String width=base.getAttribute("width", "");
// String height=base.getAttribute("height", "");
//
// if(base.getAttribute("img", "").trim().endsWith(".swf")) {
// ret.append(" \n");
// }else {
// String border = (String)paramRequest.getArguments().get("border");
// ////ret.append("");
// ret.append("
\n");
// }
// ////ret.append("" + base.getAttribute("text").trim() + "
");
// ret.append(base.getAttribute("text")+"\n");
// if(!"".equals(base.getAttribute("textcolor", ""))) {
// ret.append(" \n");
// }
// }
// return ret.toString();
// }
/**
* Process action.
*
* @param request the request
* @param response the response
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
@Override
public void processAction(HttpServletRequest request, SWBActionResponse response) throws SWBResourceException, IOException {
Resource base=getResourceBase();
String action = response.getAction();
if(SWBActionResponse.Action_ADD.equals(action)) {
getResourceBase().addHit(request, response.getUser(), response.getWebPage());
String url = base.getAttribute("url");
if( url!=null ) {
response.sendRedirect(url);
}
}else if(SWBActionResponse.Action_EDIT.equals(action)) {
/*try {
edit(request, response);
if( Boolean.parseBoolean(base.getAttribute("wbNoFile_imgfile")) ) {
File file = new File(SWBPortal.getWorkPath()+base.getWorkPath()+"/"+base.getAttribute("imgfile"));
if(file.exists() && file.delete()) {
base.removeAttribute("imgfile");
base.removeAttribute("wbNoFile_imgfile");
}
}
base.updateAttributesToDB();
response.setAction(Action_UPDATE);
}catch(FileNotFoundException fne) {
log.info(fne.getMessage());
}catch(Exception e) {
log.error(e);
}*/
}
}
@Override
public void doAdmin(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
response.setContentType("text/html; charset=utf-8");//ISO-8859-1
response.setHeader("Cache-Control","no-cache");
response.setHeader("Pragma","no-cache");
Resource base=getResourceBase();
PrintWriter out = response.getWriter();
String action = null != request.getParameter("act") && !"".equals(request.getParameter("act").trim()) ? request.getParameter("act").trim() : paramRequest.getAction();
if(SWBParamRequest.Action_ADD.equals(action) || SWBParamRequest.Action_EDIT.equals(action)) {
out.println(getForm(request, paramRequest));
}else if(Action_UPDATE.equals(action)) {
try {
edit(request);
if( Boolean.parseBoolean(base.getAttribute("wbNoFile_imgfile")) ) {
File file = new File(SWBPortal.getWorkPath()+base.getWorkPath()+"/"+base.getAttribute("imgfile"));
if(file.exists() && file.delete()) {
base.removeAttribute("imgfile");
base.removeAttribute("wbNoFile_imgfile");
}
}
base.updateAttributesToDB();
}catch(FileNotFoundException fne) {
log.info(fne.getMessage());
}catch(Exception e) {
log.error(e);
}
out.println("");
}
}
@Override
public void doAdminHlp(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
Resource base=getResourceBase();
PrintWriter out = response.getWriter();
String action = null != request.getParameter("act") && !"".equals(request.getParameter("act").trim()) ? request.getParameter("act").trim() : paramRequest.getAction();
if(SWBParamRequest.Action_ADD.equals(action) || SWBParamRequest.Action_EDIT.equals(action)) {
out.println(getForm(request, paramRequest));
}else if(Action_UPDATE.equals(action)) {
out.println("");
}
}
private String getForm(javax.servlet.http.HttpServletRequest request, SWBParamRequest paramRequest) throws SWBResourceException {
Resource base=getResourceBase();
StringBuilder htm = new StringBuilder();
final String path = SWBPortal.getWebWorkPath()+base.getWorkPath()+"/";
//final SWBResourceURL url = paramRequest.getActionUrl().setAction(SWBParamRequest.Action_EDIT);
final SWBResourceURL url = paramRequest.getRenderUrl().setAction("update");
htm.append("\n").append("\n");
htm.append("\n");
htm.append("\n");
htm.append("\n");
return htm.toString();
}
private void edit(HttpServletRequest request) throws FileNotFoundException, Exception {
Resource base = getResourceBase();
boolean isMultipart = ServletFileUpload.isMultipartContent(request);
if (isMultipart) {
File file = new File(SWBPortal.getWorkPath()+base.getWorkPath()+"/");
if(!file.exists()) {
file.mkdirs();
}
base.setAttribute("deftmp", "false");
Iterator iter = SWBUtils.IO.fileUpload(request, null);
while(iter.hasNext()) {
FileItem item = iter.next();
if(item.isFormField()) {
String name = item.getFieldName();
String value = item.getString().trim();
base.setAttribute(name, value);
}else {
String filename = item.getName().replaceAll(" ", "_").trim();
filename = filename.substring(filename.lastIndexOf('\\')+1);
if(item.getFieldName().equals("imgfile") && filename.equals("") && base.getAttribute("imgfile")==null)
{
throw new FileNotFoundException(item.getFieldName()+" es requerido");
}
else if(!filename.equals("")) {
file = new File(SWBPortal.getWorkPath()+base.getWorkPath()+"/"+filename);
item.write(file);
base.setAttribute(item.getFieldName(), filename);
base.setAttribute("wbNoFile_"+item.getFieldName(), "false");
}
else
{
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy