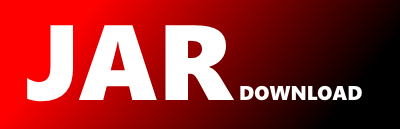
org.semanticwb.portal.resources.WBUrlContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SWBPortal Show documentation
Show all versions of SWBPortal Show documentation
SemanticWebBuilder Portal API components and utilities
The newest version!
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import java.io.*;
import java.util.*;
import java.net.*;
import java.util.Properties;
import javax.servlet.http.*;
import org.w3c.dom.*;
import org.semanticwb.Logger;
import org.semanticwb.SWBPlatform;
import org.semanticwb.SWBUtils;
import org.semanticwb.model.Resource;
import org.semanticwb.model.WebPage;
import org.semanticwb.portal.api.GenericAdmResource;
import org.semanticwb.portal.api.SWBParamRequest;
import org.semanticwb.portal.api.SWBResourceException;
import org.semanticwb.portal.admin.admresources.util.XmlBundle;
import com.arthurdo.parser.*;
import org.semanticwb.SWBPortal;
// TODO: Auto-generated Javadoc
/**
* WBUrlContent recupera el contenido de una página web externa y la incrusta como contenido local.
*
* WBUrl retrieve a external web page and embeded it like local content.
*
* @Autor: Jorge Jimenez
*/
public class WBUrlContent extends GenericAdmResource {
/** The log. */
private static Logger log = SWBUtils.getLogger(WBUrlContent.class);
/** The xml. */
String xml=null;
/** The bundle. */
XmlBundle bundle=null;
/** The recproperties. */
static Properties recproperties = null;
/** The msg2. */
String msg1 = null,msg2 = null;
/** The host. */
String host = "";
/** The file. */
String file = "";
/** The paramfrom url. */
String paramfromUrl = "";
/** The name class. */
String nameClass="WBUrlContent";
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericAdmResource#setResourceBase(org.semanticwb.model.Resource)
*/
public void setResourceBase(Resource base) throws SWBResourceException {
super.setResourceBase(base);
FileInputStream fptr = null;
recproperties = new Properties();
try {
int pos=-1;
pos=this.getClass().getName().lastIndexOf(".");
if(pos>-1){
nameClass=this.getClass().getName().substring(pos+1);
}
try {
fptr = new FileInputStream(SWBPortal.getWorkPath()+"/sites/"+base.getWebSiteId()+"/config/resources/"+nameClass+".properties");
}catch(Exception e) {
}
if(fptr==null) { //busca en raiz de work/config
try {
fptr = new FileInputStream(SWBPortal.getWorkPath()+"/work/config/resources/"+nameClass+".properties");
}catch(Exception e) {
}
}
if (fptr != null) {
recproperties.load(fptr);
msg1 = recproperties.getProperty("FuneteOriginal");
msg2 = recproperties.getProperty("InfoOriginal");
}
}catch(Exception e){
log.error("Error while loading resource properties file: "+base.getId() +"-"+ base.getTitle(), e);
}
}
/* (non-Javadoc)
* @see org.semanticwb.portal.api.GenericAdmResource#doView(javax.servlet.http.HttpServletRequest, javax.servlet.http.HttpServletResponse, org.semanticwb.portal.api.SWBParamRequest)
*/
@Override
public void doView(HttpServletRequest request, HttpServletResponse response, SWBParamRequest paramRequest) throws SWBResourceException, IOException {
Resource base=getResourceBase();
if(base.getAttribute("msg1")!=null) msg1=base.getAttribute("msg1");
if(base.getAttribute("msg2")!=null) msg2=base.getAttribute("msg2");
if(msg1==null) msg1 = paramRequest.getLocaleString("msg1") + ":";
if(msg2==null) msg2 = paramRequest.getLocaleString("msg2") + ":";
StringBuffer ret = new StringBuffer();
try{
//TODO. cambiar SWBUtils.XML.xmlToDom(base.getXml()) por el método base.getDom()
Document dom = SWBUtils.XML.xmlToDom(base.getXml());
if(dom == null) {
throw new SWBResourceException("Dom nulo");
}
ret.append("");
String param = "";
StringBuffer othersparam = new StringBuffer();
try {
if(request.getParameter("urlwb") == null) {
NodeList url = dom.getElementsByTagName("url");
if(url.getLength() > 0) {
String surl = url.item(0).getChildNodes().item(0).getNodeValue();
ret.append(CorrigeRuta(surl, paramRequest.getWebPage(), param, othersparam, request,paramRequest));
}
}else {
Enumeration en = request.getParameterNames();
boolean flag = true;
while(en.hasMoreElements()) {
String paramN = en.nextElement().toString();
if(!paramN.equals("urlwb") && !paramN.equals("param") && !paramN.equals("wbresid")) {
String[] paramval = request.getParameterValues(paramN);
for(int i = 0; i < paramval.length; i++) {
if(flag) {
othersparam.append("?" + paramN + "=" + paramval[i]);
flag = false;
}else {
othersparam.append("&" + paramN + "=" + paramval[i]);
}
}
}
}
String urlwb = "";
String wbresid = "";
if(request.getParameter("urlwb")!=null) {
urlwb = DeCodificaCadena(request.getParameter("urlwb"), paramRequest.getWebPage());
}else {
response.getWriter().print(ret.toString());
}
if(request.getParameter("param")!=null) {
param = DeCodificaCadena(request.getParameter("param"), paramRequest.getWebPage());
}
if(request.getParameter("wbresid")!=null) {
wbresid = request.getParameter("wbresid");
}else {
wbresid = base.getId();
}
if(!urlwb.equals("") && wbresid.equals(base.getId())) {
ret.append(CorrigeRuta(urlwb, paramRequest.getWebPage(), param, othersparam, request,paramRequest));
}
}
}catch (Exception f) {
ret.append("page is not in well formed..");
ret.append(" ");
log.error("Error in UrlContent resource while decoding page: "+paramRequest.getWebPage().getId(), f);
}
}catch (Exception e) {
log.error("Error in resource WBUrlContent while bringing HTML ", e);
}
PrintWriter out = response.getWriter();
out.println(ret.toString());
}
/**
* Parsea el flujo del contenido remoto parseando rutas y ligas y generando el
* resultado final del mismo.
*
* @param surl the surl
* @param topic the topic
* @param param the param
* @param othersparam the othersparam
* @param request the request
* @param paramRequest the param request
* @return the string buffer
* @throws SWBResourceException the sWB resource exception
* @return
*/
public StringBuffer CorrigeRuta(String surl, WebPage topic, String param, StringBuffer othersparam, HttpServletRequest request, SWBParamRequest paramRequest) throws SWBResourceException {
Resource base=getResourceBase();
String OriPath = surl;
String cadCod = null;
//TODO. cambiar SWBUtils.XML.xmlToDom(base.getXml()) por el método base.getDom()
Document dom = SWBUtils.XML.xmlToDom(base.getXml());
HtmlTag tag = new HtmlTag();
int pos = -1;
host = "";
file = "";
paramfromUrl = "";
String para = "";
String urlfin = "";
String surlHex = "";
String hostHex = "";
String valueHex = "";
String surlfile = "";
String surlfileHex = "";
String sruta = "";
StringBuffer ret = new StringBuffer();
NodeList tagbcawb = dom.getElementsByTagName("tagbcawb");
String stagbcawb = "bca";
if(tagbcawb.getLength() > 0)
if(!tagbcawb.item(0).getChildNodes().item(0).getNodeValue().equals(""))
stagbcawb = tagbcawb.item(0).getChildNodes().item(0).getNodeValue();
NodeList NInfoSource = dom.getElementsByTagName("InfoSource");
int iInfoSource = -1;
if(NInfoSource.getLength() > 0)
iInfoSource = Integer.parseInt(NInfoSource.item(0).getChildNodes().item(0).getNodeValue().trim());
String baserut = (String) SWBPlatform.getContextPath();
sruta = (String) SWBPlatform.getEnv("wb/distributor") + "/";
//String envia = baserut + sruta + topic.getMap().getId() + "/" + topic.getId() + "/" + base.getId();
String envia = paramRequest.getRenderUrl().toString();
if (!surl.toLowerCase().startsWith("http://") && !surl.toLowerCase().startsWith("https://"))
surl = "http://" + surl;
pos = surl.lastIndexOf("/");
if (pos != -1 && pos > 6) {
file = surl.substring(pos + 1);
surl = surl.substring(0, pos + 1);
}
pos = -1;
if (surl.startsWith("http://"))
pos = surl.indexOf("/", 7);
else if (surl.startsWith("https://"))
pos = surl.indexOf("/", 8);
if (pos != -1) {
host = surl.substring(0, pos);
} else {
surl = surl + "/";
host = surl;
}
String ssign = "";
if (!param.equals(""))
ssign = "?";
String sothersparam = "";
if (othersparam != null)
sothersparam = othersparam.toString();
sothersparam = sothersparam.replaceAll(" ", "%20");
if (file.indexOf("?") > -1 && sothersparam != null && sothersparam.length() > 0 && sothersparam.charAt(0) == '?') sothersparam = "&" + sothersparam.substring(1);
if (request.getMethod().equals("POST"))
urlfin = surl + file + sothersparam;
else
urlfin = surl + file + ssign + param + sothersparam;
pos = -1;
pos = file.indexOf("?");
if (pos > -1) {
surlfile = surl + file.substring(0, pos);
} else
surlfile = surl + file;
try {
URL pagina = new URL(urlfin);
String hostCookie = pagina.getHost();
String cookie = (String) request.getSession().getAttribute("Cookie:" + hostCookie);
String sheader = request.getHeader("user-agent");
URLConnection conex = pagina.openConnection();
if (cookie != null) conex.setRequestProperty("Cookie", cookie);
if (sheader != null) conex.setRequestProperty("user-agent", sheader);
//Paso de parametros por metodo POST
if (request.getMethod().equals("POST") && sothersparam.length() > 0) {
conex.setDoInput(true);
conex.setDoOutput(true);
conex.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
DataOutputStream dos = new DataOutputStream(conex.getOutputStream());
//String post = "param1=" + URLEncoder.encode( paramVariable1 ) + "¶m2=" + URLEncoder.encode( paramVariable2 );
if (sothersparam.charAt(0) == '?')
sothersparam = sothersparam.substring(1);
dos.writeBytes(sothersparam);
dos.flush();
dos.close();
}
// Termina paso de parametros por metodo POST
DataInputStream datos = new DataInputStream(conex.getInputStream());
HtmlStreamTokenizer tok = new HtmlStreamTokenizer(datos);
boolean framewb = false;
while (tok.nextToken() != HtmlStreamTokenizer.TT_EOF) {
int ttype = tok.getTokenType();
if (ttype == HtmlStreamTokenizer.TT_TAG || ttype == HtmlStreamTokenizer.TT_COMMENT) {
tok.parseTag(tok.getStringValue(), tag);
if (tok.getRawString().toLowerCase().startsWith("