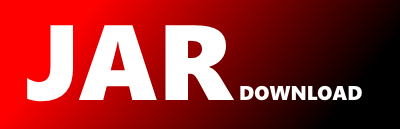
org.semanticwb.portal.resources.Window Maven / Gradle / Ivy
Show all versions of SWBPortal Show documentation
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.portal.resources;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.semanticwb.portal.api.GenericAdmResource;
import org.semanticwb.Logger;
import java.io.IOException;
import java.io.PrintWriter;
import org.semanticwb.SWBPlatform;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBUtils;
import org.semanticwb.model.Resource;
import org.semanticwb.portal.api.SWBActionResponse;
import org.semanticwb.portal.api.SWBParamRequest;
import org.semanticwb.portal.api.SWBResourceException;
import org.semanticwb.portal.api.SWBResourceURL;
import org.semanticwb.portal.api.SWBResourceURLImp;
// TODO: Auto-generated Javadoc
/** Despliega y administra una ventana de tipo pop-up bajo ciertos
* criterios de configuración.
*
* Displays and manages a pop-up window under some configuration criteria.
* @author : Jorge Alberto Jiménez Sandoval
* @since : 2.0
*/
public class Window extends GenericAdmResource {
/**
* contiene la ruta física de la carpeta de trabajo para este recurso
* contains this resource's phisical work directory path.
*/
String workPath = "";
/**
* contiene la ruta en Web de la carpeta de trabajo para este recurso
* contains this resource's Web work directory path.
*/
String webWorkPath = "/work";
/**
* objeto encargado de crear mensajes en los archivos log de SemanticWebBuilder (SWB).
* object that creates messages in SWB's log file.
*/
private static Logger log = SWBUtils.getLogger(Window.class);
/**
* Crea una nueva instancia de esta clase.
*/
public Window() {
}
/**
* Inicializa este recurso configurando los valores de {@code workPath} y {@code webWorkPath}
* de acuerdo con la instalación de SemanticWebBuilder
*
Initializes this resource confiugring the values of {@code workPath} and {@code webWorkPath}
* accordingly to SemanticWebBuilder instalation.
* @param base el objeto base para la configuración de este recurso
* the base object for this resource's configuration
*/
@Override
public void setResourceBase(Resource base) {
try {
super.setResourceBase(base);
workPath = SWBPortal.getWorkPath() + base.getWorkPath();
webWorkPath = SWBPortal.getWebWorkPath() + base.getWorkPath();
} catch (Exception e) {
log.error("Error while setting resource base: " + base.getId()
+ "-" + base.getTitle(), e);
}
}
/**
* Genera el código HTML para abrir una ventana, de acuerdo a la
* configuración seleccionada en la administración del recurso.
* Generates the HTML code needed to open a browser window, according to
* the configuration selected in this resource's administration view
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param reqParams el objeto generado por SWB y asociado a la petición
* del usuario.the object generated by SWB and asociated to the user's request
* @throws SWBResourceException si la llamada al método {@code showWindow}
* o la obtención del Writer
del response
* correspondiente la propagan.
* if it is propagated by calling method {@code showWindow}
* or getting the corresponding response
's Writer
.
* @throws IOException si la llamada al método {@code showWindow} la propaga.
* if it is propagated by the call to method {@code showWindow}.
*/
@Override
public void doView(HttpServletRequest request,
HttpServletResponse response,
SWBParamRequest reqParams)
throws SWBResourceException, java.io.IOException {
String action = (null != request.getParameter("ven_act")
&& !"".equals(request.getParameter("ven_act").trim()))
? request.getParameter("ven_act").trim()
: "ven_step1";
if ("ven_step2".equals(action)) {
showWindow(request, response, reqParams); // Nueva ventana pop-up
} else { // Se invoca la nueva ventana
Resource base = getResourceBase();
if ("yes".equals(base.getAttribute("bysession", ""))) {
if (request.getSession().getAttribute("wbwindow") != null
&& String.valueOf(base.getId() + "_"
+ base.getWebSiteId()).equals(request.getSession(
).getAttribute("wbwindow"))) {
return;
} else {
request.getSession().setAttribute("wbwindow",
base.getId() + "_" + base.getWebSiteId());
}
}
StringBuilder props = new StringBuilder(250);
props.append("menubar=");
props.append(base.getAttribute("menubar", "no").trim());
props.append(",toolbar=");
props.append(base.getAttribute("toolbar", "no").trim());
props.append(",status=");
props.append(base.getAttribute("status", "no").trim());
props.append(",location=");
props.append(base.getAttribute("location", "no").trim());
props.append(",directories=");
props.append(base.getAttribute("directories", "no").trim());
props.append(",scrollbars=");
props.append(base.getAttribute("scrollbars", "no").trim());
props.append(",resizable=");
props.append(base.getAttribute("resizable", "no").trim());
props.append(",width=");
props.append(base.getAttribute("width", "300").trim());
props.append(",height=");
props.append(base.getAttribute("height", "200").trim());
props.append(",top=");
props.append(base.getAttribute("top", "10").trim());
props.append(",left=");
props.append(base.getAttribute("left", "10").trim());
SWBResourceURLImp url = new SWBResourceURLImp(request, base,
reqParams.getWebPage(), SWBResourceURL.UrlType_RENDER);
url.setResourceBase(base);
url.setMode(SWBResourceURL.Mode_VIEW);
url.setWindowState(SWBResourceURL.WinState_MAXIMIZED);
url.setParameter("ven_act", "ven_step2");
url.setTopic(reqParams.getWebPage());
url.setCallMethod(reqParams.Call_DIRECT);
//response.sendRedirect(url.toString());
String ret = null;
ret = "";
PrintWriter out = response.getWriter();
if (ret != null) {
out.println(ret);
}
}
}
/**
* Crea el código del documento HTML a mostrar en la ventana indicada,
* según la configuración seleccionada en la administración
* de este recurso.
* Creates the HTML code needed to show a browser window with the
* characteristics indicated in this resource's adminitration view.
*
* @param request la petición HTTP generada por el usuario. the user's HTTP request
* @param response la respuesta hacia el usuario.the response to the user
* @param reqParams el objeto generado por SWB y asociado a la petición
* del usuario.the object generated by SWB and asociated to the user's request
* @throws SWBResourceException the sWB resource exception
* @throws IOException al obtener el Writer
del response
* correspondiente. when getting the corresponding response
's
* Writer
.
*/
public void showWindow(HttpServletRequest request,
HttpServletResponse response,
SWBParamRequest reqParams)
throws SWBResourceException, java.io.IOException {
StringBuffer ret = new StringBuffer("");
Resource base = getResourceBase();
ret.append(" \n");
ret.append(" \n");
if (!"".equals(base.getAttribute("title", "").trim())) {
ret.append("" + base.getAttribute("title").trim() + " ");
}
ret.append(" \n");
ret.append(" \n");
ret.append(" \n");
if (!"".equals(base.getAttribute("title", "").trim())) {
ret.append(base.getAttribute("title"));
ret.append("
");
}
SWBResourceURL wburl = reqParams.getActionUrl();
String url = base.getAttribute("url", "").trim();
if (!"".equals(url)) {
/*
0 - en la misma ventana
1 - en el window.opener y cerrar ventana
2 - en el window.opener y sin cerrar ventana
3 - nueva ventana y cerrar la anterior
4 - nueva ventana y sin cerrar la anterior (default)
*/
String target = base.getAttribute("target", "4").trim();
if ("0".equals(target)) {
ret.append(" \n");
} else if ("1".equals(target) || "2".equals(target)) {
ret.append(" \n");
ret.append(" \n");
} else if ("3".equals(target) || "4".equals(target)) {
ret.append(" \n");
}
}
String img = base.getAttribute("img", "").trim();
if (!"".equals(img)) {
if (img.endsWith(".swf")) {
ret.append("\n");
} else {
ret.append("
");
}
}
if (!"".equals(base.getAttribute("text", "").trim())) {
ret.append(base.getAttribute("text"));
}
if (!"".equals(url)) {
ret.append(" \n");
}
ret.append(" \n");
ret.append(" \n");
ret.append(" \n");
response.getWriter().print(ret.toString());
}
/**
* Registra el hit realizado en el contenido de este recurso, cuando se
* realiza un clic en la liga mostrada por el texto o imagen desplegados para
* posteriormente redirigir el flujo al destino de la liga.
* Registers the hit executed by the user on this resource's content, when
* clicking the link showed to open the window.
*
* @param request la petición HTTP generada por el usuario. the
* user's HTTP request
* @param response la respuesta a la acción solicitada por el usuario
* the response to the action requested by the user.
* @throws SWBResourceException the sWB resource exception
* @throws IOException Signals that an I/O exception has occurred.
*/
@Override
public void processAction(HttpServletRequest request,
SWBActionResponse response)
throws SWBResourceException, java.io.IOException {
Resource base = getResourceBase();
base.addHit(request, response.getUser(), response.getWebPage());
String url = base.getAttribute("url", "").trim();
if (!url.equals("")) {
response.sendRedirect(url);
}
}
}