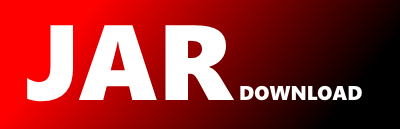
org.semanticwb.servlet.internal.MultipleFileUploader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SWBPortal Show documentation
Show all versions of SWBPortal Show documentation
SemanticWebBuilder Portal API components and utilities
The newest version!
/*
* SemanticWebBuilder es una plataforma para el desarrollo de portales y aplicaciones de integración,
* colaboración y conocimiento, que gracias al uso de tecnología semántica puede generar contextos de
* información alrededor de algún tema de interés o bien integrar información y aplicaciones de diferentes
* fuentes, donde a la información se le asigna un significado, de forma que pueda ser interpretada y
* procesada por personas y/o sistemas, es una creación original del Fondo de Información y Documentación
* para la Industria INFOTEC, cuyo registro se encuentra actualmente en trámite.
*
* INFOTEC pone a su disposición la herramienta SemanticWebBuilder a través de su licenciamiento abierto al público (‘open source’),
* en virtud del cual, usted podrá usarlo en las mismas condiciones con que INFOTEC lo ha diseñado y puesto a su disposición;
* aprender de él; distribuirlo a terceros; acceder a su código fuente y modificarlo, y combinarlo o enlazarlo con otro software,
* todo ello de conformidad con los términos y condiciones de la LICENCIA ABIERTA AL PÚBLICO que otorga INFOTEC para la utilización
* del SemanticWebBuilder 4.0.
*
* INFOTEC no otorga garantía sobre SemanticWebBuilder, de ninguna especie y naturaleza, ni implícita ni explícita,
* siendo usted completamente responsable de la utilización que le dé y asumiendo la totalidad de los riesgos que puedan derivar
* de la misma.
*
* Si usted tiene cualquier duda o comentario sobre SemanticWebBuilder, INFOTEC pone a su disposición la siguiente
* dirección electrónica:
* http://www.semanticwebbuilder.org
*/
package org.semanticwb.servlet.internal;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.Set;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSessionBindingEvent;
import javax.servlet.http.HttpSessionBindingListener;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.semanticwb.Logger;
import org.semanticwb.SWBPortal;
import org.semanticwb.SWBRuntimeException;
import org.semanticwb.SWBUtils;
import org.semanticwb.model.SWBContext;
import org.semanticwb.model.SWBModel;
import org.semanticwb.model.User;
import org.semanticwb.model.WebSite;
import org.semanticwb.util.UploadFileRequest;
import org.semanticwb.util.UploadedFile;
import org.semanticwb.util.UploaderFileCacheUtils;
// TODO: Auto-generated Javadoc
/**
* The Class MultipleFileUploader.
*
* @author serch
*/
public class MultipleFileUploader implements InternalServlet
{
public static Logger log = SWBUtils.getLogger(MultipleFileUploader.class);
{
File tmpplace = new File(org.semanticwb.SWBPortal.getWorkPath() + "/tmp/");
if (tmpplace.exists())
{
File[] childs = tmpplace.listFiles();
if (null!=childs){
for (File tmpfile : childs)
{
UploaderFileCacheUtils.delete(tmpfile);
}
} else {
log.error("MultipleFileUploader: Problem doing listFiles on "+tmpplace.getAbsolutePath());
}
}
UploaderFileCacheUtils.setHomepath(org.semanticwb.SWBPortal.getWorkPath());
}
/* (non-Javadoc)
* @see org.semanticwb.servlet.internal.InternalServlet#init(ServletContext)
*/
public void init(ServletContext config) throws ServletException
{
}
/* (non-Javadoc)
* @see org.semanticwb.servlet.internal.InternalServlet#doProcess(HttpServletRequest, HttpServletResponse, DistributorParams)
*/
public void doProcess(HttpServletRequest request, HttpServletResponse response, DistributorParams dparams) throws IOException, ServletException
{
//System.out.println("********************** MulpleFileUploades.doProcess**********************");
String uri = request.getRequestURI();
String cntx = request.getContextPath();
String path = uri.substring(cntx.length());
String iserv = "";
if (path == null || path.length() == 0)
{
path = "/";
} else
{
int j = path.indexOf('/', 1);
if (j > 0)
{
iserv = path.substring(1, j);
} else
{
iserv = path.substring(1);
}
}
String cad = path.substring(path.lastIndexOf("/") + 1);
String auri = path.substring(iserv.length() + 1);
//System.out.println("cad:"+cad+" auri:"+auri+" user:"+dparams.getUser().getLogin());
//DistributorParams dparam = null;
String smodel = auri.substring(1, auri.indexOf("/", 2));
SWBModel model=SWBContext.getSWBModel(smodel);
//System.out.println("model:"+model);
WebSite website=null;
if(!(model instanceof WebSite))
{
website=model.getParentWebSite();
}else
{
website=(WebSite)model;
}
//Agregado Jorge Jiménez para SWBSocial-12/Agosto/2013, ya que se quedaba nula la variable website y marcaba error en SWBUser
//Se utiliza este componente desde la edición de un usuario en el repositorio de Admin y al final llega Nulo el website hasta este paso.
if(website==null)
{
website=SWBContext.getAdminWebSite();
}
//Termina agregado Jorge Jimenez
User user=SWBPortal.getUserMgr().getUser(request, website);
if (!user.isSigned()){
website=SWBContext.getWebSite("SWBAdmin");
user=SWBPortal.getUserMgr().getUser(request, website);
}
// if (dparams.getUser().isSigned()) {
// dparam=dparams;
// }
// else {
// dparam=new DistributorParams(request, auri);
// if (!dparam.getUser().isSigned()){
// DistributorParams dparamt=new DistributorParams(request, "/multiuploader/SWBAdmin/home");
// if (dparamt.getUser().isSigned()){
// dparam=dparamt;
// }
// }
// }
//System.out.println("user: " + user.getFullName() + ":" + user.isSigned());
if (ServletFileUpload.isMultipartContent(request))
{
response.setContentType("application/json");
List archivos = UploaderFileCacheUtils.get(cad); //System.out.println("archivos:"+archivos);
String subcad = "f" + archivos.size() + cad.substring(4); //si no existe archivos, debemos ignorar el request, el NPE nos facilita salir
if (archivos != null)
{
File tmpplace = new File(org.semanticwb.SWBPortal.getWorkPath() + "/tmp/" + cad);
if (!tmpplace.exists())
{
tmpplace.mkdirs();
request.getSession(true).setAttribute(cad, new UploadsCleanUp(tmpplace.getCanonicalPath()));
}
DiskFileItemFactory factory = new DiskFileItemFactory(3000, tmpplace);
ServletFileUpload upload = new ServletFileUpload(factory);
if (UploaderFileCacheUtils.getRequest(cad).size()>0)
{
upload.setSizeMax(UploaderFileCacheUtils.getRequest(cad).size());
}
try
{
List items = upload.parseRequest(request);
String lastUploadedFileName = "";
for (FileItem item : items)
{
if (!item.isFormField())
{
String fieldName = item.getFieldName();
String fileName = item.getName();
if (fileName.indexOf("\\")>=0)
{
fileName = fileName.substring(fileName.lastIndexOf("\\")+1);
}
//100112 MAPS Cleaning of filename
fileName = SWBUtils.TEXT.replaceSpecialCharacters(fileName, '.', true);
//--End
String contentType = item.getContentType();
boolean isInMemory = item.isInMemory();
long sizeInBytes = item.getSize();
File uploadedFile = new File(tmpplace, subcad + "_" + fileName + "_");
item.write(uploadedFile);
archivos.add(new UploadedFile(fileName, uploadedFile.getCanonicalPath(), cad));
lastUploadedFileName = fileName;
}
}
response.getWriter().println("{\"status\":\"success\", \"detail\":\""+lastUploadedFileName+"\"}");
} catch (Exception ex)
{
response.getWriter().println("{\"status\":\"error\", \"detail\":\""+ex.getMessage()+"\"}");
throw new SWBRuntimeException("Uploading file", ex);
}
} else
{
response.getWriter().println("{\"status\":\"error\", \"detail\":\"UploadService not pre-registered\"}");
// response.sendError(500, "UploadService not pre-registered");
}
} else if (user.isSigned())
{
if (UploaderFileCacheUtils.get(cad) == null)
{
UploaderFileCacheUtils.put(cad, new java.util.LinkedList());
}
response.getWriter().print(getPage(SWBPortal.getContextPath()+request.getServletPath(),cad));
} else
{
response.getWriter().println("{\"status\":\"error\", \"detail\":\"Not enough privileges\"}");
// response.sendError(403, "Not enough privileges");
}
// // Check that we have a file upload request
// boolean isMultipart = ServletFileUpload.isMultipartContent(request);
//
// // Create a factory for disk-based file items
// DiskFileItemFactory factory = new DiskFileItemFactory();
//
//// Set factory constraints
// factory.setSizeThreshold(50000);
// factory.setRepository(new File(""));
//
//// Create a new file upload handler
// ServletFileUpload upload = new ServletFileUpload(factory);
//
//// Set overall request size constraint
// upload.setSizeMax();
//
//// Parse the request
// List /* FileItem */ items = upload.parseRequest(request);
//
}
/**
* Gets the page.
*
* @param ruta the ruta
* @param cad the cad
* @return the page
*/
private String getPage(String ruta, String cad)
{
//System.out.println("********************** MulpleFileUploades.getPage**********************");
UploadFileRequest requested = UploaderFileCacheUtils.getRequest(cad);
StringBuilder buffer = new StringBuilder();
if (null!=requested){
buffer.append("\n");
buffer.append("\n");
buffer.append(" \n");
buffer.append(" Flash HTML \n");
buffer.append(" \n");
// buffer.append(" \n");
// buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
buffer.append(" \n");
// buffer.append("\n");
// buffer.append(" \n");
buffer.append(" \n");
buffer.append("\n");
} else {
buffer.append("");
}
return buffer.toString();
}
}
class UploadsCleanUp implements HttpSessionBindingListener
{
private final String path;
public UploadsCleanUp(String path)
{
this.path = path;
}
public void valueBound(HttpSessionBindingEvent hsbe)
{
//do nothing
}
public void valueUnbound(HttpSessionBindingEvent hsbe)
{
File file = new File(path);
if (file.exists())
{
UploaderFileCacheUtils.delete(file);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy