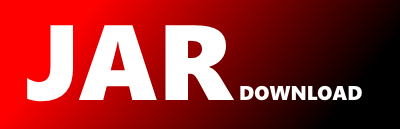
org.semanticweb.elk.owlapi.OwlChangesLoader Maven / Gradle / Ivy
/*
* #%L
* ELK OWL API Binding
*
* $Id$
* $HeadURL$
* %%
* Copyright (C) 2011 - 2012 Department of Computer Science, University of Oxford
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.semanticweb.elk.owlapi;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
import java.util.Set;
import org.apache.log4j.Logger;
import org.semanticweb.elk.loading.ChangesLoader;
import org.semanticweb.elk.loading.ElkLoadingException;
import org.semanticweb.elk.loading.Loader;
import org.semanticweb.elk.owl.interfaces.ElkAxiom;
import org.semanticweb.elk.owl.visitors.ElkAxiomProcessor;
import org.semanticweb.elk.owlapi.wrapper.OwlConverter;
import org.semanticweb.elk.reasoner.ProgressMonitor;
import org.semanticweb.owlapi.model.AddAxiom;
import org.semanticweb.owlapi.model.OWLAxiom;
import org.semanticweb.owlapi.model.OWLOntologyChange;
import org.semanticweb.owlapi.model.RemoveAxiom;
/**
* An {@link ChangesLoader} that accumulates the {@link OWLOntologyChange} and
* provides them by converting through {@link OwlConverter}
*
* @author "Yevgeny Kazakov"
*
*/
public class OwlChangesLoader implements ChangesLoader {
// logger for this class
private static final Logger LOGGER_ = Logger
.getLogger(OwlChangesLoader.class);
private static final OwlConverter OWL_CONVERTER_ = OwlConverter
.getInstance();
private final ProgressMonitor progressMonitor;
/** list to accumulate the unprocessed changes to the ontology */
protected final LinkedList pendingChanges;
OwlChangesLoader(ProgressMonitor progressMonitor) {
this.progressMonitor = progressMonitor;
this.pendingChanges = new LinkedList();
}
@Override
public Loader getLoader(final ElkAxiomProcessor axiomInserter,
final ElkAxiomProcessor axiomDeleter) {
return new Loader() {
@Override
public void load() throws ElkLoadingException {
if (!pendingChanges.isEmpty()) {
String status = "Loading of Changes";
progressMonitor.start(status);
int changesCount = pendingChanges.size();
if (LOGGER_.isTraceEnabled())
LOGGER_.trace(status + ": " + changesCount);
int currentAxiom = 0;
for (;;) {
if (Thread.currentThread().isInterrupted())
break;
OWLOntologyChange change = ((Queue) pendingChanges)
.poll();
if (change == null)
break;
if (!change.isAxiomChange()) {
ElkLoadingException exception = new ElkLoadingException(
"Cannot apply non-axiom change!");
LOGGER_.error(exception);
throw exception;
}
OWLAxiom owlAxiom = change.getAxiom();
ElkAxiom elkAxiom = OWL_CONVERTER_.convert(owlAxiom);
if (change instanceof AddAxiom) {
axiomInserter.visit(elkAxiom);
if (LOGGER_.isTraceEnabled())
LOGGER_.trace("adding " + owlAxiom);
} else if (change instanceof RemoveAxiom) {
axiomDeleter.visit(elkAxiom);
if (LOGGER_.isTraceEnabled())
LOGGER_.trace("removing " + owlAxiom);
} else
throw new ElkLoadingException(
"Change type is not supported!");
currentAxiom++;
progressMonitor.report(currentAxiom, changesCount);
}
progressMonitor.finish();
}
}
@Override
public void dispose() {
// nothing was allocated by this loader
}
};
}
void registerChange(OWLOntologyChange change) {
pendingChanges.add(change);
}
Set getPendingAxiomAdditions() {
Set added = new HashSet();
for (OWLOntologyChange change : pendingChanges) {
if (change instanceof AddAxiom) {
added.add(change.getAxiom());
}
}
return added;
}
Set getPendingAxiomRemovals() {
Set removed = new HashSet();
for (OWLOntologyChange change : pendingChanges) {
if (change instanceof RemoveAxiom) {
removed.add(change.getAxiom());
}
}
return removed;
}
List getPendingChanges() {
return pendingChanges;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy