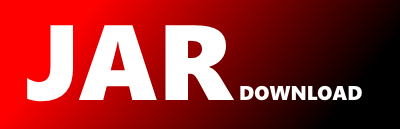
org.semanticweb.elk.reasoner.saturation.classes.ContextElClassSaturation Maven / Gradle / Ivy
/*
* #%L
* ELK Reasoner
*
* $Id$
* $HeadURL$
* %%
* Copyright (C) 2011 - 2012 Department of Computer Science, University of Oxford
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.semanticweb.elk.reasoner.saturation.classes;
import java.util.Set;
import org.semanticweb.elk.reasoner.indexing.hierarchy.IndexedDisjointnessAxiom;
import org.semanticweb.elk.reasoner.indexing.hierarchy.IndexedClassExpression;
import org.semanticweb.elk.reasoner.indexing.hierarchy.IndexedPropertyChain;
import org.semanticweb.elk.reasoner.saturation.rulesystem.AbstractContext;
import org.semanticweb.elk.reasoner.saturation.rulesystem.Queueable;
import org.semanticweb.elk.util.collections.ArrayHashSet;
import org.semanticweb.elk.util.collections.HashSetMultimap;
import org.semanticweb.elk.util.collections.Multimap;
/**
* Context implementation that is used for EL reasoning. It provides data
* structures for storing and retrieving various types of derived expressions,
* including computed subsumptions between class expressions.
*
* @author Markus Kroetzsch
* @author Frantisek Simancik
*
*/
public class ContextElClassSaturation extends AbstractContext implements
ContextClassSaturation {
final Set superClassExpressions;
Multimap backwardLinksByObjectProperty;
Multimap forwardLinksByObjectProperty;
Multimap> propagationsByObjectProperty;
Set disjointnessAxioms;
/**
* A context is saturated if all superclass expressions of the root
* expression have been computed.
*/
protected volatile boolean isSaturated = false;
/**
* False if owl:Nothing is stored as a superclass in this context.
*/
protected volatile boolean isSatisfiable = true;
/**
* If set to true, then composition rules will be applied to derive all
* incoming links. This is usually needed only when at least one propagation
* has been derived at this object.
*/
protected boolean composeBackwardLinks = false;
/**
* If set to true, then propagations will be derived in this context. This
* is needed only when there is at least one backward link.
*/
protected boolean derivePropagations = false;
public ContextElClassSaturation(IndexedClassExpression root) {
super(root);
this.superClassExpressions = new ArrayHashSet(
13);
}
@Override
public Set getSuperClassExpressions() {
return superClassExpressions;
}
@Override
public boolean isSatisfiable() {
return isSatisfiable;
}
@Override
public void setSatisfiable(boolean satisfiable) {
isSatisfiable = satisfiable;
}
/**
* Determines if composition rules should be applied to derive all incoming
* links.
*
* @return {@code true} if composition rules should be applied to derive all
* incoming link.
*/
public boolean getDeriveBackwardLinks() {
return composeBackwardLinks;
}
public void setDeriveBackwardLinks(boolean deriveBackwardLinks) {
this.composeBackwardLinks = deriveBackwardLinks;
}
public Multimap getBackwardLinksByObjectProperty() {
return backwardLinksByObjectProperty;
}
/**
* Initialize the set of propagations with the empty set
*/
public void initBackwardLinksByProperty() {
backwardLinksByObjectProperty = new HashSetMultimap();
}
public Multimap getForwardLinksByObjectProperty() {
return forwardLinksByObjectProperty;
}
public void initForwardLinksByProperty() {
forwardLinksByObjectProperty = new HashSetMultimap();
}
/**
* If set to true, then propagations will be derived in this context. This
* is needed only when there is at least one backward link.
*/
/**
* Determines if propagations should be derived in this context.
*
* @return {@code true} if propagations should be derived in this context
*/
public boolean getDerivePropagations() {
return derivePropagations;
}
public void setDerivePropagations(boolean derivePropagations) {
this.derivePropagations = derivePropagations;
}
public Multimap> getPropagationsByObjectProperty() {
return propagationsByObjectProperty;
}
/**
* Initialize the set of propagations with the empty set
*/
public void initPropagationsByProperty() {
propagationsByObjectProperty = new HashSetMultimap>();
}
public boolean addDisjointessAxiom(
IndexedDisjointnessAxiom disjointnessAxiom) {
if (disjointnessAxioms == null)
disjointnessAxioms = new ArrayHashSet();
return disjointnessAxioms.add(disjointnessAxiom);
}
/**
* Mark context as saturated. A context is saturated if all superclass
* expressions of the root expression have been computed.
*/
@Override
public void setSaturated() {
isSaturated = true;
}
/**
* Return if context is saturated. A context is saturated if all superclass
* expressions of the root expression have been computed. This needs to be
* set explicitly by some processor.
*
* @return {@code true} if this context is saturated and {@code false}
* otherwise
*/
@Override
public boolean isSaturated() {
return isSaturated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy