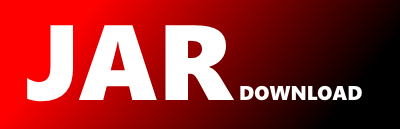
org.seppiko.commons.utils.NumberUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.text.DecimalFormat;
/**
* Number Util
*
* @author Leonard Woo
*/
public class NumberUtil {
private NumberUtil() {}
/** ISO-LATIN-1(ASCII) digits ({@code '0'} through {@code '9'}) */
public static final int LATIN_DIGITS = 1; // 0x30 to 0x39
/** Fullwidth digits ({@code '0'} through {@code '9'}) */
public static final int FULLWIDTH_DIGITS = 4; // 0xFF10 to 0xFF19
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(byte num, byte min, byte max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(short num, short min, short max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(int num, int min, int max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(long num, long min, long max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(float num, float min, float max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(double num, double min, double max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(char num, char min, char max) {
return num >= min && num <= max;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(BigDecimal num, BigDecimal min, BigDecimal max) {
return num.compareTo(min) >= 0 && num.compareTo(max) <= 0;
}
/**
* test num between min and max
*
* @param num number
* @param min minimum number
* @param max maximum number
* @return true number is between the maximum and minimum
*/
public static boolean between(BigInteger num, BigInteger min, BigInteger max) {
return num.compareTo(min) >= 0 && num.compareTo(max) <= 0;
}
/**
* Double format
*
* @param precisionPattern precision pattern, e.g. 0.00
* @param number double number
* @return formatted string
*/
public static String format(String precisionPattern, double number) {
return new DecimalFormat(precisionPattern).format(number);
}
/**
* byte array to unsigned int array
*
* @param src byte array
* @return unsigned int array
*/
public static int[] toUnsignedIntArray(byte[] src) {
int[] dst = new int[src.length];
for (int i = 0; i < src.length; i++) {
dst[i] = Byte.toUnsignedInt(src[i]);
}
return dst;
}
/**
* short array to unsigned int array
*
* @param src short array
* @return unsigned int array
*/
public static int[] toUnsignedShortArray(short[] src) {
int[] dst = new int[src.length];
for (int i = 0; i < src.length; i++) {
dst[i] = Short.toUnsignedInt(src[i]);
}
return dst;
}
/**
* int array to unsigned long array
*
* @param src int array
* @return unsigned long array
*/
public static long[] toUnsignedLongArray(int[] src) {
long[] dst = new long[src.length];
for (int i = 0; i < src.length; i++) {
dst[i] = Integer.toUnsignedLong(src[i]);
}
return dst;
}
/**
* Convert between {@link #LATIN_DIGITS} and {@link #FULLWIDTH_DIGITS} and support simple
* calculation result.
*
* @param input digit character sequence
* @param outType output digit type {@link #LATIN_DIGITS} or {@link #FULLWIDTH_DIGITS}
* @return decimal string
* @throws IllegalArgumentException input is not digit or outType is wrong
*/
public static String convert(CharSequence input, int outType) throws IllegalArgumentException {
if (!StringUtil.isNumeric(input)) {
throw new IllegalArgumentException("input is not number");
}
BigDecimal digit = new BigDecimal(toHalfWidthWithoutNumeric(input));
if (outType == LATIN_DIGITS) {
return digit.toString();
} else if (outType == FULLWIDTH_DIGITS) {
return StringUtil.toFullWidth(digit.toString());
}
throw new IllegalArgumentException("outType is wrong");
}
/**
* To half-width without numeric
*
* @param input full-width numeric.
* @return Half-Width without numeric.
*/
protected static String toHalfWidthWithoutNumeric(CharSequence input) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < input.length(); i++) {
char c = input.charAt(i);
if (between(c, CharUtil.FULLWIDTH_DIGIT_ZERO, CharUtil.FULLWIDTH_DIGIT_NINE)) {
sb.append(c);
} else {
sb.append(StringUtil.toHalfWidth(String.valueOf(c)));
}
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy