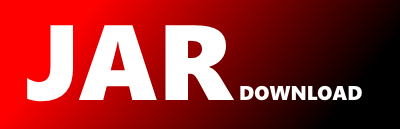
org.seppiko.commons.utils.RegexUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils;
import java.util.Objects;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
/**
* Regular expression util
*
* @see Pattern
* @see Matcher
* @author Leonard Woo
*/
public class RegexUtil {
private RegexUtil() {}
/**
* Compiles the given regular expression and attempts to match the given input against it.
*
* @param regex The expression to be compiled.
* @param input The character sequence to be matched.
* @return Whether the regular expression matches on the input.
*/
public static boolean matches(String regex, CharSequence input) {
try {
return Objects.requireNonNull(getMatcher(regex, input)).matches();
} catch (NullPointerException ignored) {
}
return false;
}
/**
* Returns the input subsequence captured by the given index during match operation.
*
* @param regex The expression to be compiled.
* @param input The character sequence to be matched.
* @return The (possibly empty) subsequence captured by the index during match,
* or empty if the group failed to match part of the input.
*/
public static String find(String regex, CharSequence input) {
try {
Matcher matcher = Objects.requireNonNull(getMatcher(regex, input));
if (matcher.find()) {
return matcher.group();
}
} catch (NullPointerException ignored) {
}
return "";
}
/**
*
* @param regex The delimiting regular expression.
* @param input The character sequence to be split.
* @return The array of strings computed by splitting the input around matches of this pattern.
* If the expression's syntax is invalid return an empty string array.
*/
public static String[] split(String regex, CharSequence input) {
try {
Pattern pattern = getPattern(regex);
return pattern.split(input);
} catch (PatternSyntaxException | NullPointerException ignored) {
}
return new String[0];
}
/**
* A matcher that will match the given input against this pattern.
*
* @param regex The expression to be compiled.
* @param input The character sequence to be matched.
* @return {@link Matcher} instance.
* @throws NullPointerException If regex or input is {@code null}.
* @throws IllegalArgumentException if the expression's syntax is invalid.
*/
public static Matcher getMatcher(String regex, CharSequence input) throws NullPointerException, IllegalArgumentException {
try {
Pattern pattern = getPattern(regex);
return pattern.matcher(Objects.requireNonNull(input));
} catch (PatternSyntaxException ex) {
throw new IllegalArgumentException(ex);
}
}
/**
* Compiles the given regular expression the given input against it.
*
* @param regex The expression to be compiled.
* @return {@link Pattern} The given regular expression compiled into a pattern.
* @throws PatternSyntaxException If the expression's syntax is invalid.
* @throws NullPointerException If regex is {@code null}.
*/
public static Pattern getPattern(String regex) throws PatternSyntaxException, NullPointerException {
return Pattern.compile(Objects.requireNonNull(regex));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy