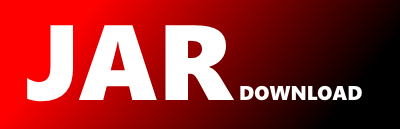
org.seppiko.commons.utils.SerializationUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.NotSerializableException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.Objects;
/**
* Serialization Utility
*
*
* JDK object serialization and deserialization
*
* @see ByteArrayInputStream
* @see ByteArrayOutputStream
* @see ObjectInputStream
* @see ObjectOutputStream
* @author Leonard Woo
*/
public class SerializationUtil {
private SerializationUtil() {}
/**
* Serialization object.
*
* @param object an object.
* @return serialize byte array.
* @param the object type. If object is {@code null} return an empty bytes.
* @throws IOException Any exception thrown by the underlying {@link OutputStream}.
* @throws SecurityException if the stream header is incorrect.
*/
public static byte[] serialize(T object)
throws IOException, SecurityException {
if (Objects.isNull(object)) {
return Environment.EMPTY_BYTE_ARRAY;
}
byte[] result;
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
try (ObjectOutputStream oos = new ObjectOutputStream(baos)) {
oos.writeObject(object);
oos.flush();
}
result = baos.toByteArray();
}
return result;
}
/**
* Deserialization object.
*
* @param bytes object byte array.
* @param clazz the object type class.
* @return the object.
* @param the object type. If serialized object not found return {@code null}.
* @throws IOException Any exception thrown by the underlying {@link OutputStream}.
* Or something is wrong with a class used by deserialization.
* @throws ClassNotFoundException Class of a serialized object cannot be found.
* @throws SecurityException if the stream header is incorrect.
* @throws NullPointerException if bytes is {@code null} or clazz is {@code null}.
*/
public static T deserialize(byte[] bytes, Class clazz)
throws IOException, ClassNotFoundException, SecurityException {
if (bytes == null || bytes.length == 0) {
throw new NullPointerException("bytes must not be blank.");
}
Objects.requireNonNull(clazz, "clazz must not be null.");
T result;
try (ByteArrayInputStream bais = new ByteArrayInputStream(bytes)) {
try (ObjectInputStream ois = new ObjectInputStream(bais)) {
result = clazz.cast(ois.readObject());
}
}
return result;
}
}