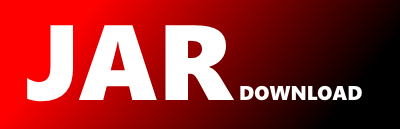
org.seppiko.commons.utils.StreamUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.StringReader;
import java.net.URL;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* File and Stream Util
*
* @author Leonard Woo
*/
public class StreamUtil {
private StreamUtil() {}
/**
* Find and load file from pathname
*
* @param clazz classloader loading point.
* @param pathname relative path.
* @return file input stream if file not found is {@code null}.
*/
public static InputStream findFileInputStream(Class> clazz, String pathname) {
try {
return new FileInputStream(findFile(clazz, pathname));
} catch (IOException | NullPointerException ignored) {
}
return null;
}
/**
* Find and load file from pathname
*
* @param clazz classloader loading point.
* @param pathname relative path.
* @return file instance.
* @throws NullPointerException pathname is {@code null}.
*/
public static File findFile(Class> clazz, String pathname) throws NullPointerException {
Objects.requireNonNull(pathname);
String _pathname = getClassLoaderPath(clazz.getClassLoader(), pathname);
_pathname = (_pathname == null ? pathname : _pathname);
return new File(_pathname);
}
private static String getClassLoaderPath(ClassLoader cl, String path) throws NullPointerException {
URL url = cl.getResource(path);
return Objects.isNull(url) ? null : url.getPath();
}
/**
* Load the target file or create it. if it fails.
*
* @param path file pathname.
* @return target file.
* @throws IllegalArgumentException File could not be loaded.
* @throws NullPointerException path is {@code null}.
* @throws IOException The path is a folder address or failed to create a sub-folder.
*/
public static File loadFile(String path) throws IllegalArgumentException, NullPointerException, IOException {
File file = new File(path);
if (file.isDirectory()) {
throw new IllegalArgumentException("Target path is not a valid file.");
}
if (file.exists()) {
return file;
}
String absolutePath = file.getAbsolutePath();
int _lastFolder = absolutePath.lastIndexOf('/');
if (_lastFolder < 0) {
_lastFolder = absolutePath.lastIndexOf('\\');
}
String targetPath = absolutePath.substring(0, _lastFolder);
File folder = new File(targetPath);
if (!folder.mkdirs()) {
throw new IOException("Failed to create destination folder.");
}
if (!file.createNewFile()) {
throw new IllegalArgumentException("Unable to load target file.");
}
return file;
}
/**
* Find and get file steam
*
* @param clazz classloader loading point.
* @param pathname relative path.
* @return File input stream if not found is {@code null}.
*/
public static InputStream getStream(Class> clazz, String pathname) {
return getStream(findFile(clazz, pathname));
}
/**
* Get file stream
*
* @param filepath File path.
* @return File input stream if file not found is {@code null}.
*/
public static InputStream getStream(String filepath) {
return getStream(new File(filepath));
}
/**
* Get file stream
*
* @param file File instance.
* @return File input stream if file not found is {@code null}.
*/
public static InputStream getStream(File file) {
try {
Objects.requireNonNull(file);
return new BufferedInputStream(new FileInputStream(file));
} catch (FileNotFoundException | NullPointerException ignored) {
}
return null;
}
/**
* Load string to reader
*
* @param str String.
* @return Reader instance.
*/
public static BufferedReader loadReader(String str) {
return new BufferedReader(new StringReader(str));
}
/**
* Load reader from InputStream.
*
* @param is Input stream.
* @return Reader instance.
*/
public static BufferedReader loadReader(InputStream is) {
return new BufferedReader(new InputStreamReader(is));
}
/**
* Load input stream to reader with charset
*
* @param is Input stream.
* @param charset Charset see {@link StandardCharsets} and {@link ExtendedCharsets}.
* @return Reader instance.
*/
public static BufferedReader loadReader(InputStream is, Charset charset) {
return new BufferedReader(new InputStreamReader(is, charset));
}
/**
* export byte array to output stream
*
* @param bytes byte array.
* @param os output stream.
* @throws IOException byte array write failed.
*/
public static void exportOutputStream(byte[] bytes, OutputStream os) throws IOException {
try(BufferedOutputStream bos = new BufferedOutputStream(os)) {
bos.write(bytes);
bos.flush();
}
}
/**
* Convert reader to String and close
*
* @param reader reader object.
* @param lineStrip if true remote every line begin and end whitespace, false otherwise.
* @param ignoreNewLine if true read all content without new line, false otherwise.
* @return reader content without newline chars.
* @throws IOException read close exception.
*/
public static String readerTo(BufferedReader reader, boolean lineStrip, boolean ignoreNewLine)
throws IOException {
try (reader) {
return readerTo(reader, lineStrip, ignoreNewLine ? null : Environment.NEW_LINE);
}
}
/**
* Convert reader to string
*
* @param reader reader object.
* @param lineStrip if true strip every line, false otherwise.
* @param newLine newline chars.
* @return reader content.
* @throws IOException read close exception.
*/
public static String readerTo(BufferedReader reader, boolean lineStrip, String newLine)
throws IOException {
StringBuilder sb = new StringBuilder();
ArrayList lines = reader.lines().collect(Collectors.toCollection(ArrayList::new));
for (String line : lines) {
if (lineStrip) {
line = line.strip();
}
sb.append(line);
if (newLine != null) {
sb.append(newLine);
}
}
reader.close();
return sb.toString();
}
/**
* Convert reader all to String
*
* @param reader reader object.
* @return reader content.
* @throws IOException read exception.
*/
public static String readerToString(BufferedReader reader) throws IOException {
StringBuilder sb = new StringBuilder();
while (reader.ready()) {
sb.append((char) reader.read());
}
reader.close();
return sb.toString();
}
/**
* Write byte array to file If not exist create it
*
* @param b bytes.
* @param file File.
* @throws IOException I/O error occurs.
*/
public static void writeFile(byte[] b, File file) throws IOException {
writeFile(b, file, false);
}
/**
* Write byte array to file If not exist create it
*
* @param b bytes.
* @param file File.
* @param append file append.
* @throws IOException I/O error occurs.
*/
public static void writeFile(byte[] b, File file, boolean append) throws IOException {
if (!file.exists()) {
if (!file.createNewFile()) {
throw new IOException("Can not create file");
}
}
if (!file.canWrite()) {
throw new IOException("Can not write file");
}
try (OutputStream os = new FileOutputStream(file, append)) {
exportOutputStream(b, os);
os.flush();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy