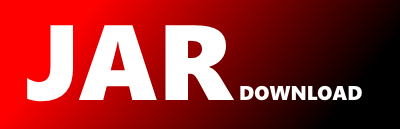
org.seppiko.commons.utils.crypto.CRC64ECMA182 Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.util.zip.Checksum;
/**
* A class that can be used to compute the CRC64 of a data stream with ECMA182.
*
* @see
* Cyclic redundancy check(CRC)
* @see ECMA-182
* @author Leonard Woo
*/
public class CRC64ECMA182 implements Checksum {
private static final long ECMA182INIT = 0;
private static final long ECMA182POLY = 0xC96C5795D7870F42L;
private static final int TABLE_LENGTH = 0x100;
private static final long[] table;
static {
// Generator table
table = new long[TABLE_LENGTH];
for (int n = 0; n < TABLE_LENGTH; n++) {
long crc = n;
for (int k = 0; k < 8; k++) {
crc = ((crc & 1) == 1) ? ((crc >>> 1) ^ ECMA182POLY) : (crc >>> 1);
}
table[n] = crc;
}
}
private long crc;
/** Create CRC64 object and initialization. */
public CRC64ECMA182() {
crc = ECMA182INIT;
}
/**
* Updates the CRC64 checksum with the specified byte.
*
* @param b number.
*/
@Override
public void update(int b) {
updateByte((byte) (b & 0xFF));
}
/**
* Updates the CRC64 checksum with the specified array of bytes.
*
* @param b data byte array.
* @param off data init offset.
* @param len data length.
* @throws NullPointerException data is {@code null}.
* @throws ArrayIndexOutOfBoundsException if off is negative, or len is negative, or off+len is *
* negative or greater than the length of the array b.
*/
@Override
public void update(byte[] b, int off, int len)
throws NullPointerException, ArrayIndexOutOfBoundsException {
updateCheck(b, off, len);
for (int i = off; len > 0; i++, len--) {
updateByte(b[i]);
}
}
// calc exec
private void updateByte(final byte b) {
crc = ~crc;
crc = (crc >>> 8) ^ table[((int) (crc ^ b)) & 0xFF];
crc = ~crc;
}
private void updateCheck(byte[] b, int off, int len)
throws NullPointerException, ArrayIndexOutOfBoundsException {
if (b == null) {
throw new NullPointerException();
}
if (off < 0 || len < 0 || off > (b.length - len)) {
throw new ArrayIndexOutOfBoundsException();
}
}
/**
* Returns CRC64 value.
*
* @return CRC64 value.
*/
@Override
public long getValue() {
return crc;
}
/** Resets CRC64 to initial value. */
@Override
public void reset() {
crc = ECMA182INIT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy