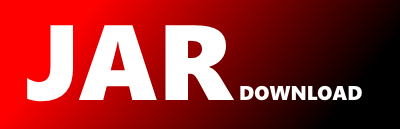
org.seppiko.commons.utils.crypto.CRCUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.util.zip.CRC32C;
import org.seppiko.commons.utils.MathUtil;
import org.seppiko.commons.utils.codec.HexUtil;
/**
* CRC Util
*
* @author Leonard Woo
*/
public class CRCUtil {
private CRCUtil() {}
/** CRC16/MAXIM */
public static class CRC16M {
private static final CRC16MAXIM crc16MAXIM;
static {
crc16MAXIM = new CRC16MAXIM();
}
/**
* encoding
*
* @param data raw data
* @return Hash code
*/
public static long encode(byte[] data) {
crc16MAXIM.update(data);
return crc16MAXIM.getValue();
}
/**
* encoding with string
*
* @param data raw data
* @return Hash HEX string
*/
public static String encodeString(byte[] data) {
long crc16 = encode(data);
return MathUtil.convertDecimalTo(crc16, HexUtil.HEXADECIMAL);
}
}
/** CRC24-C */
public static class CRC24C {
private static final CRC24 crc24;
static {
crc24 = new CRC24();
}
/**
* encoding
*
* @param data raw data
* @return Hash code
*/
public static long encode(byte[] data) {
crc24.update(data);
return crc24.getValue();
}
/**
* encoding with string
*
* @param data raw data
* @return Hash HEX string
*/
public static String encodeString(byte[] data) {
long crc24 = encode(data);
return MathUtil.convertDecimalTo(crc24, HexUtil.HEXADECIMAL);
}
}
/** CRC32-C */
public static class CRC32 {
private static final CRC32C crc32;
static {
crc32 = new CRC32C();
}
/**
* encoding
*
* @param data raw data
* @return Hash code
*/
public static long encode(byte[] data) {
crc32.update(data);
return crc32.getValue();
}
/**
* encoding with string
*
* @param data raw data
* @return Hash HEX string
*/
public static String encodeString(byte[] data) {
long crc32 = encode(data);
return MathUtil.convertDecimalTo(crc32, HexUtil.HEXADECIMAL);
}
}
/** CRC64/ECMA182 */
public static class CRC64E {
private static final CRC64ECMA182 crc64e;
static {
crc64e = new CRC64ECMA182();
}
/**
* encoding
*
* @param data raw data
* @return Hash code
*/
public static long encode(byte[] data) {
crc64e.update(data);
return crc64e.getValue();
}
/**
* encoding with string
*
* @param data raw data
* @return Hash HEX string
*/
public static String encodeString(byte[] data) {
long crc64 = encode(data);
return MathUtil.convertDecimalTo(crc64, HexUtil.HEXADECIMAL);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy