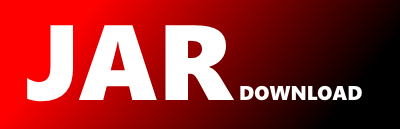
org.seppiko.commons.utils.crypto.CryptoUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.Key;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.Provider;
import java.security.Security;
import java.security.spec.AlgorithmParameterSpec;
import java.util.Objects;
import java.util.Optional;
import javax.crypto.AEADBadTagException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.Mac;
import javax.crypto.NoSuchPaddingException;
import org.seppiko.commons.utils.StringUtil;
import org.seppiko.commons.utils.crypto.spec.KeySpecUtil;
/**
* Crypto util
*
* @see
* Java Security Standard Algorithm Names
* @author Leonard Woo
*/
public class CryptoUtil {
/** Non provider */
public static final Provider NONPROVIDER = null;
private CryptoUtil() {}
/**
* Check and get provider object
*
* @param providerName the name of the provider.
* @return the provider instance.
* If provider name is empty or {@code null} return {@link CryptoUtil#NONPROVIDER}.
* If provider not found return {@link CryptoUtil#NONPROVIDER}.
*/
public static Provider getProvider(String providerName) {
if (StringUtil.isNullOrEmpty(providerName)) {
return NONPROVIDER;
}
return Security.getProvider(providerName);
}
/**
* Cipher util
*
* @see Cipher
* @see CryptoUtil#getProvider(String)
* @param algorithm cipher algorithm.
* @param provider cipher provider, if you do not need this set {@code null} or
* {@link CryptoUtil#NONPROVIDER}.
* @param opmode cipher mode.
* @param key crypto key.
* @param params Algorithm Parameter Spec, if you do not need this set {@code null}.
* @param data the input data buffer.
* @return the new data buffer with the result.
* @throws NoSuchPaddingException if transformation contains a padding scheme that is not
* available.
* @throws NoSuchAlgorithmException if transformation is null, empty, in an invalid format, or if
* no Provider supports a CipherSpi implementation for the specified algorithm.
* @throws InvalidAlgorithmParameterException if the given algorithm parameters are inappropriate
* for this cipher, or this cipher requires algorithm parameters and params is null, or the
* given algorithm parameters imply a cryptographic strength that would exceed the legal
* limits (as determined from the configured jurisdiction policy files).
* @throws InvalidKeyException if the given key is inappropriate for initializing this cipher, or
* its keysize exceeds the maximum allowable keysize (as determined from the configured
* jurisdiction policy files).
* @throws IllegalBlockSizeException if this cipher is a block cipher, no padding has been
* requested (only in encryption mode), and the total input length of the data processed by
* this cipher is not a multiple of block size; or if this encryption algorithm is unable to
* process the input data provided.
* @throws BadPaddingException if this cipher is in decryption mode, and (un)padding has been
* requested, but the decrypted data is not bounded by the appropriate padding bytes.
* @throws AEADBadTagException – if this cipher is decrypting in an AEAD mode (such as GCM/CCM),
* and the received authentication tag does not match the calculated value.
*/
public static byte[] cipher(String algorithm, Provider provider, int opmode, Key key,
AlgorithmParameterSpec params, byte[] data)
throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException,
InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher;
if (provider == NONPROVIDER) {
cipher = Cipher.getInstance(algorithm);
} else {
cipher = Cipher.getInstance(algorithm, provider);
}
if (Objects.isNull(params)) {
cipher.init(opmode, key);
} else {
cipher.init(opmode, key, params);
}
return cipher.doFinal(data);
}
/**
* Cipher util
*
* @see Cipher
* @param algorithm cipher algorithm.
* @param providerName cipher provider name, if you do not need this set {@code null} or
* {@code ""}.
* @param opmode cipher mode.
* @param key crypto key.
* @param params Algorithm Parameter Spec, if you do not need this set null.
* @param data the input data buffer.
* @return the new data buffer with the result.
* @throws NoSuchPaddingException if transformation contains a padding scheme that is not
* available.
* @throws NoSuchAlgorithmException if transformation is null, empty, in an invalid format, or if
* no Provider supports a CipherSpi implementation for the specified algorithm.
* @throws InvalidAlgorithmParameterException if the given algorithm parameters are inappropriate
* for this cipher, or this cipher requires algorithm parameters and params is null, or the
* given algorithm parameters imply a cryptographic strength that would exceed the legal
* limits (as determined from the configured jurisdiction policy files).
* @throws InvalidKeyException if the given key is inappropriate for initializing this cipher, or
* its keysize exceeds the maximum allowable keysize (as determined from the configured
* jurisdiction policy files).
* @throws IllegalBlockSizeException if this cipher is a block cipher, no padding has been
* requested (only in encryption mode), and the total input length of the data processed by
* this cipher is not a multiple of block size; or if this encryption algorithm is unable to
* process the input data provided.
* @throws BadPaddingException if this cipher is in decryption mode, and (un)padding has been
* requested, but the decrypted data is not bounded by the appropriate padding bytes.
* @throws AEADBadTagException – if this cipher is decrypting in an AEAD mode (such as GCM/CCM),
* and the received authentication tag does not match the calculated value.
*/
public static byte[] cipher(String algorithm, String providerName, int opmode, Key key,
AlgorithmParameterSpec params, byte[] data)
throws InvalidAlgorithmParameterException, NoSuchPaddingException, IllegalBlockSizeException,
NoSuchAlgorithmException, BadPaddingException, InvalidKeyException {
Provider p = getProvider(providerName);
return cipher(algorithm, p, opmode, key, params, data);
}
/**
* Message Digest util
*
* @see MessageDigest
* @param algorithm Message Digest Algorithm.
* @param provider the provider. if you do not need this set {@link CryptoUtil#NONPROVIDER}.
* @param data raw data.
* @return data hash.
* @throws NoSuchAlgorithmException if no Provider supports a MessageDigestSpi implementation for
* the specified algorithm.
* @throws NullPointerException if algorithm is null.
*/
public static byte[] md(String algorithm, Provider provider, byte[] data)
throws NoSuchAlgorithmException, NullPointerException {
MessageDigest md;
if (provider == NONPROVIDER) {
md = MessageDigest.getInstance(algorithm);
} else {
md = MessageDigest.getInstance(algorithm, provider);
}
md.update(data);
return md.digest();
}
/**
* Mac util
*
* @see Mac
* @param algorithm mac hash algorithm.
* @param provider the provider. if you do not need this set {@link CryptoUtil#NONPROVIDER}.
* @param keyAlgorithm mac hash key algorithm.
* @param data raw data.
* @param key mac hash key.
* @return data hash.
* @throws NoSuchAlgorithmException if no Provider supports a MacSpi implementation for the
* specified algorithm.
* @throws InvalidKeyException if the given key is inappropriate for initializing this MAC.
* @throws NullPointerException if algorithm is null.
*/
public static byte[] mac(
String algorithm, Provider provider, String keyAlgorithm, byte[] data, byte[] key)
throws NoSuchAlgorithmException, InvalidKeyException, NullPointerException {
Mac mac;
if (provider == NONPROVIDER) {
mac = Mac.getInstance(algorithm);
} else {
mac = Mac.getInstance(algorithm, provider);
}
mac.init(KeySpecUtil.getSecret(key, keyAlgorithm));
return mac.doFinal(data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy