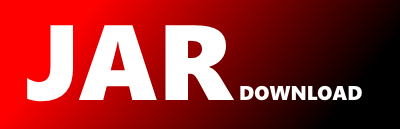
org.seppiko.commons.utils.crypto.Cryptography Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.Provider;
import java.security.spec.AlgorithmParameterSpec;
import javax.crypto.BadPaddingException;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
/**
* Cryptography interface.
*
* @author Leonard Woo
*/
public interface Cryptography {
/**
* Set provider.
*
* @param providerName the provider name.
*/
default void setProvider(String providerName) {
setProvider(CryptoUtil.getProvider(providerName));
}
/**
* Set provider.
*
* @param provider the provider.
*/
void setProvider(Provider provider);
/**
* Set {@link AlgorithmParameterSpec}.
*
* @param params {@link AlgorithmParameterSpec} subclass.
*/
void setParameterSpec(AlgorithmParameterSpec params);
/**
* Encrypt data.
*
* @param data plaintext data buffer.
* @return ciphertext data buffer.
* @throws InvalidAlgorithmParameterException if the given algorithm parameters are inappropriate
* for this cipher, or this cipher requires algorithm parameters and params is null, or the
* given algorithm parameters imply a cryptographic strength that would exceed the legal
* limits (as determined from the configured jurisdiction policy files).
* @throws NoSuchPaddingException if transformation contains a padding scheme that is not
* available.
* @throws IllegalBlockSizeException if this cipher is a block cipher, no padding has been
* requested (only in encryption mode), and the total input length of the data processed by
* this cipher is not a multiple of block size; or if this encryption algorithm is unable to
* process the input data provided.
* @throws NoSuchAlgorithmException if transformation is null, empty, in an invalid format, or if
* no Provider supports a CipherSpi implementation for the specified algorithm.
* @throws BadPaddingException if this cipher is in decryption mode, and (un)padding has been
* requested, but the decrypted data is not bounded by the appropriate padding bytes.
* @throws InvalidKeyException if the given key is inappropriate for initializing this cipher, or
* its key-size exceeds the maximum allowable key-size (as determined from the configured
* jurisdiction policy files).
* @throws NullPointerException key is {@code null}.
*/
byte[] encrypt(byte[] data)
throws InvalidAlgorithmParameterException, NoSuchPaddingException, IllegalBlockSizeException,
NoSuchAlgorithmException, BadPaddingException, InvalidKeyException, NullPointerException;
/**
* Decrypt data.
*
* @param data ciphertext data buffer.
* @return plaintext data buffer.
* @throws InvalidAlgorithmParameterException if the given algorithm parameters are inappropriate
* for this cipher, or this cipher requires algorithm parameters and params is null, or the
* given algorithm parameters imply a cryptographic strength that would exceed the legal
* limits (as determined from the configured jurisdiction policy files).
* @throws NoSuchPaddingException if transformation contains a padding scheme that is not
* available.
* @throws IllegalBlockSizeException if this cipher is a block cipher, no padding has been
* requested (only in encryption mode), and the total input length of the data processed by
* this cipher is not a multiple of block size; or if this encryption algorithm is unable to
* process the input data provided.
* @throws NoSuchAlgorithmException if transformation is null, empty, in an invalid format, or if
* no Provider supports a CipherSpi implementation for the specified algorithm.
* @throws BadPaddingException if this cipher is in decryption mode, and (un)padding has been
* requested, but the decrypted data is not bounded by the appropriate padding bytes.
* @throws InvalidKeyException if the given key is inappropriate for initializing this cipher, or
* its key-size exceeds the maximum allowable key-size (as determined from the configured
* jurisdiction policy files).
* @throws NullPointerException key is {@code null}.
*/
byte[] decrypt(byte[] data)
throws InvalidAlgorithmParameterException, NoSuchPaddingException, IllegalBlockSizeException,
NoSuchAlgorithmException, BadPaddingException, InvalidKeyException, NullPointerException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy