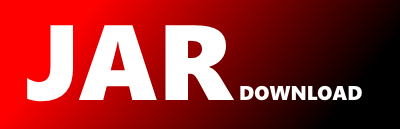
org.seppiko.commons.utils.crypto.GeneratorUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.security.AlgorithmParameterGenerator;
import java.security.InvalidAlgorithmParameterException;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
import java.security.Provider;
import java.security.SecureRandom;
import java.security.SecureRandomParameters;
import java.security.spec.AlgorithmParameterSpec;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import org.seppiko.commons.utils.StringUtil;
/**
* Key and KeyPair Generator object util And SecureRandom object util
*
* @see Java
* Security Standard Algorithm Names
* @author Leonard Woo
*/
public class GeneratorUtil {
private GeneratorUtil() {}
/**
* Returns a KeyGenerator object that generates secret keys for the specified algorithm.
*
* @param algorithm the standard name of the requested key algorithm
* @return new KeyGenerator instance
* @throws NoSuchAlgorithmException if no Provider supports a KeyGeneratorSpi implementation for
* the specified algorithm
* @throws NullPointerException if algorithm is null
*/
public static KeyGenerator keyGenerator(String algorithm)
throws NoSuchAlgorithmException, NullPointerException {
return KeyGenerator.getInstance(algorithm);
}
/**
* Returns a KeyGenerator object that generates secret keys for the specified algorithm.
*
* @param algorithm the standard name of the requested key algorithm
* @param provider the provider
* @return new KeyGenerator instance
* @throws NoSuchAlgorithmException if a KeyGeneratorSpi implementation for the specified
* algorithm is not available from the specified Provider object
* @throws NullPointerException if algorithm is null
* @throws IllegalArgumentException if the provider is null
*/
public static KeyGenerator keyGenerator(String algorithm, Provider provider)
throws NoSuchAlgorithmException, NullPointerException, IllegalArgumentException {
return KeyGenerator.getInstance(algorithm, provider);
}
private static KeyGenerator keyGenerator0(String algorithm, Provider provider)
throws NoSuchAlgorithmException, NullPointerException, IllegalArgumentException {
if (provider == null) {
return keyGenerator(algorithm);
} else {
return keyGenerator(algorithm, provider);
}
}
/**
* Returns a KeyPairGenerator object that generates public/private key pairs for the specified
* algorithm.
*
* @param algorithm the standard string name of the algorithm
* @return the new KeyPairGenerator instance
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm
* @throws NullPointerException if algorithm is null
*/
public static KeyPairGenerator keyPairGenerator(String algorithm)
throws NoSuchAlgorithmException, NullPointerException {
return KeyPairGenerator.getInstance(algorithm);
}
/**
* Returns a KeyPairGenerator object that generates public/private key pairs for the specified *
* algorithm.
*
* @param algorithm the standard string name of the algorithm
* @param provider the provider
* @return the new KeyPairGenerator instance
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm
* @throws IllegalArgumentException if the specified provider is null
* @throws NullPointerException if algorithm is null
*/
public static KeyPairGenerator keyPairGenerator(String algorithm, Provider provider)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
return KeyPairGenerator.getInstance(algorithm, provider);
}
private static KeyPairGenerator keyPairGenerator0(String algorithm, Provider provider)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
if (provider == null) {
return keyPairGenerator(algorithm);
} else {
return keyPairGenerator(algorithm, provider);
}
}
/**
* Returns an AlgorithmParameterGenerator object for generating a set of parameters to be used
* with the specified algorithm.
*
* @param algorithm the name of the algorithm this parameter generator is associated with
* @return the new AlgorithmParameterGenerator instance
* @throws NoSuchAlgorithmException if an AlgorithmParameterGeneratorSpi implementation for the
* specified algorithm is not available from the specified Provider object
* @throws NullPointerException if algorithm is null
*/
public static AlgorithmParameterGenerator algorithmParameterGenerator(String algorithm)
throws NoSuchAlgorithmException, NullPointerException {
return AlgorithmParameterGenerator.getInstance(algorithm);
}
/**
* Returns an AlgorithmParameterGenerator object for generating a set of parameters to be used
* with the specified algorithm.
*
* @param algorithm the name of the algorithm this parameter generator is associated with
* @param provider the Provider
* @return the new AlgorithmParameterGenerator instance
* @throws NoSuchAlgorithmException if an AlgorithmParameterGeneratorSpi implementation for the
* specified algorithm is not available from the specified Provider object
* @throws IllegalArgumentException if the specified provider is null
* @throws NullPointerException if algorithm is null
*/
public static AlgorithmParameterGenerator algorithmParameterGenerator(
String algorithm, Provider provider)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
return AlgorithmParameterGenerator.getInstance(algorithm, provider);
}
/**
* Cryptographic key generator
*
* @param algorithm the standard name of the requested key algorithm
* @param provider the provider, if use default provider is null
* @param keySize key size
* @return secret key byte array
* @throws NoSuchAlgorithmException if a KeyGeneratorSpi implementation for the specified
* algorithm is not available from the specified Provider object
* @throws NullPointerException if algorithm is null
* @throws IllegalArgumentException if the provider is null
*/
public static SecretKey keyGenerator(String algorithm, Provider provider, int keySize)
throws NoSuchAlgorithmException, NullPointerException, IllegalArgumentException {
KeyGenerator keyGenerator = keyGenerator0(algorithm, provider);
keyGenerator.init(keySize);
return keyGenerator.generateKey();
}
/**
* Signature cryptographic key pair generator
*
* @see KeyPairGenerator
* @param algorithm the standard string name of the algorithm.
* @param provider the provider, if use default provider is null.
* @return keypair instance.
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm.
* @throws IllegalArgumentException if the provider is null.
* @throws NullPointerException if algorithm is null.
*/
public static KeyPair keyPairGeneratorWithoutInitialize(String algorithm, Provider provider)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
KeyPairGenerator keyPairGenerator = keyPairGenerator0(algorithm, provider);
return keyPairGenerator.generateKeyPair();
}
/**
* Signature cryptographic key pair generator
*
* @see KeyPairGenerator
* @param algorithm the standard string name of the algorithm.
* @return keypair instance.
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm.
* @throws IllegalArgumentException if the provider is null.
* @throws NullPointerException if algorithm is null.
*/
public static KeyPair keyPairGeneratorWithoutInitialize(String algorithm)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
return keyPairGeneratorWithoutInitialize(algorithm, null);
}
/**
* Asymmetric cryptographic key pair generator
*
* Every implementation of the Java platform is required to support the
* following standard {@link KeyPairGenerator} algorithms and keysizes in
* parentheses:
*
* - {@code DiffieHellman} (1024, 2048, 4096)
* - {@code DSA} (1024, 2048)
* - {@code RSA} (1024, 2048, 4096)
* - {@code EdDSA} OR {@code Ed25519} (255)
* - {@code Ed448} (448)
* - {@code XDH} OR {@code X25519} (255)
* - {@code X448} (448)
*
*
* @see KeyPairGenerator
* @param algorithm the standard string name of the algorithm.
* @param provider the provider, if use default provider is null.
* @param keySize the keysize. This is an algorithm-specific metric, such as modulus length,
* specified in number of bits.
* @return keypair instance.
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm.
* @throws IllegalArgumentException if the provider is null.
* @throws NullPointerException if algorithm is null.
*/
public static KeyPair keyPairGenerator(String algorithm, Provider provider, int keySize)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException {
KeyPairGenerator keyPairGenerator = keyPairGenerator0(algorithm, provider);
keyPairGenerator.initialize(keySize);
return keyPairGenerator.generateKeyPair();
}
/**
* Asymmetric cryptographic key pair generator
*
* @see KeyPairGenerator
* @param algorithm the standard string name of the algorithm.
* @param provider the provider, if use default provider is null.
* @param params the parameter set used to generate the keys.
* @return keypair instance.
* @throws NoSuchAlgorithmException if no Provider supports a KeyPairGeneratorSpi implementation
* for the specified algorithm.
* @throws IllegalArgumentException if the provider is null.
* @throws NullPointerException if algorithm is null.
* @throws InvalidAlgorithmParameterException if the given parameters are inappropriate for this
* key pair generator.
*/
public static KeyPair keyPairGenerator(
String algorithm, Provider provider, AlgorithmParameterSpec params)
throws NoSuchAlgorithmException, IllegalArgumentException, NullPointerException,
InvalidAlgorithmParameterException {
KeyPairGenerator keyPairGenerator = keyPairGenerator0(algorithm, provider);
keyPairGenerator.initialize(params);
return keyPairGenerator.generateKeyPair();
}
/**
* Returns a SecureRandom object that implements the specified Random Number Generator (RNG)
* algorithm.
*
* @return the new SecureRandom instance.
*/
public static SecureRandom secureRandom() {
return new SecureRandom();
}
/**
* Returns a SecureRandom object that implements the specified Random Number Generator (RNG)
* algorithm.
*
* @param algorithm the name of the RNG algorithm.
* @return the new SecureRandom instance.
*/
public static SecureRandom secureRandom(String algorithm) {
return secureRandom(algorithm, CryptoUtil.NONPROVIDER);
}
/**
* Returns a SecureRandom object that implements the specified Random Number Generator (RNG)
* algorithm.
*
* @param algorithm the name of the RNG algorithm.
* @param provider the provider.
* @return the new SecureRandom instance.
*/
public static SecureRandom secureRandom(String algorithm, Provider provider) {
return secureRandom(algorithm, provider, null);
}
/**
* Returns a SecureRandom object that implements the specified Random Number Generator (RNG)
* algorithm.
*
* @param algorithm the name of the RNG algorithm.
* @param provider the provider.
* @param params the SecureRandomParameters the newly created SecureRandom object must support.
* @return the new SecureRandom instance.
*/
public static SecureRandom secureRandom(String algorithm, Provider provider,
SecureRandomParameters params) {
return secureRandom0(algorithm, provider, params);
}
/**
* Get secure random bytes
*
* @param algorithm the name of the RNG algorithm.
* @param provider the provider.
* @param params the SecureRandomParameters the newly created SecureRandom object must support.
* @param seedSize the number of seed bytes to generate.
* @return the seed bytes.
*/
public static byte[] secureRandom(String algorithm, Provider provider,
SecureRandomParameters params, int seedSize) {
return secureRandom(algorithm, provider, params).generateSeed(seedSize);
}
private static SecureRandom secureRandom0(String algorithm, Provider provider,
SecureRandomParameters params) {
if (!StringUtil.isNullOrEmpty(algorithm)) {
try {
if (provider != CryptoUtil.NONPROVIDER && params != null) {
return SecureRandom.getInstance(algorithm, params, provider);
} else if (provider != CryptoUtil.NONPROVIDER) {
return SecureRandom.getInstance(algorithm, provider);
} else {
return SecureRandom.getInstance(algorithm);
}
} catch (NoSuchAlgorithmException ignored) {
}
}
return secureRandom();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy