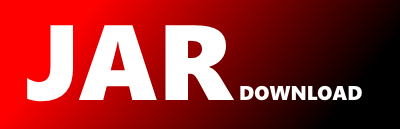
org.seppiko.commons.utils.crypto.HashUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.Provider;
import java.security.spec.InvalidKeySpecException;
import org.seppiko.commons.utils.codec.HexUtil;
import org.seppiko.commons.utils.crypto.spec.KeySpecUtil;
/**
* Hash Util
*
* @author Leonard Woo
*/
public class HashUtil {
private HashUtil() {}
/**
* Simplifies common {@link java.security.MessageDigest} tasks.
*
* @param algorithm Message Digest Algorithm
* @param provider Hash provider, null is use default provider
* @param data data to hash
* @return complete hash value
* @throws IllegalArgumentException when a {@link NoSuchAlgorithmException} is caught.
*/
public static byte[] mdHash(MessageDigestAlgorithms algorithm, Provider provider, byte[] data)
throws IllegalArgumentException {
try {
return CryptoUtil.md(algorithm.getName(), provider, data);
} catch (NoSuchAlgorithmException ex) {
throw new IllegalArgumentException(ex);
}
}
/**
* Simplifies common {@link java.security.MessageDigest} tasks.
*
* @param algorithm Message Digest Algorithm
* @param provider Hash provider, null is use default provider
* @param data data to hash
* @return complete hash string
* @throws IllegalArgumentException when a {@link NoSuchAlgorithmException} is caught.
*/
public static String mdHashString(
MessageDigestAlgorithms algorithm, Provider provider, byte[] data)
throws IllegalArgumentException {
return new String(HexUtil.encode(mdHash(algorithm, provider, data)));
}
/**
* Simplifies common {@link javax.crypto.Mac} tasks.
*
* @param algorithm Hmac Algorithm
* @param provider Hash provider, null is use default provider
* @param data data to hash with key
* @param key the keyed digest
* @return complete hash value including key
* @throws InvalidKeyException if the given key is {@code null} or does not match the allowed
* pattern.
* @throws IllegalArgumentException when a {@link NoSuchAlgorithmException} is caught.
*/
public static byte[] hmacHash(
HmacAlgorithms algorithm, Provider provider, byte[] data, byte[] key)
throws InvalidKeyException, IllegalArgumentException {
try {
return CryptoUtil.mac(algorithm.getName(), provider, algorithm.getName(), data, key);
} catch (NoSuchAlgorithmException ignored) {
}
throw new IllegalArgumentException();
}
/**
* Simplifies common {@link javax.crypto.Mac} tasks.
*
* @param algorithm Hmac Algorithm
* @param provider Hash provider, null is use default provider
* @param data data to hash with key
* @param key the keyed digest
* @return complete hash string including key
* @throws InvalidKeyException if the given key is {@code null} or does not match the allowed
* pattern.
* @throws IllegalArgumentException when a {@link NoSuchAlgorithmException} is caught.
*/
public static String hmacHashString(
HmacAlgorithms algorithm, Provider provider, byte[] data, byte[] key)
throws InvalidKeyException, IllegalArgumentException {
return new String(HexUtil.encode(hmacHash(algorithm, provider, data, key)));
}
/**
* Simplifies common PBKDF2 tasks
*
* @param algorithm PBKDF2With<Hmac Algorithm>
* @param provider Hash provider, null is use default provider
* @param password raw password
* @param salt password salt
* @param iterations iteration
* @param keySize key size
* @return PBKDF2With<Hmac Algorithm> password
* @throws IllegalArgumentException password or salt is null, number is wrong or could not execute
* task.
*/
public static byte[] pbkdf2Hash(
HmacAlgorithms algorithm,
Provider provider,
char[] password,
byte[] salt,
int iterations,
int keySize)
throws IllegalArgumentException {
try {
if (algorithm.getName().contains("PBE")) {
throw new NoSuchAlgorithmException("Algorithm must be exclude PBE.");
}
return KeyUtil.secretKeyFactory("PBKDF2With" + algorithm.getName(), provider,
KeySpecUtil.getPBE(password, salt, iterations, keySize));
} catch (NoSuchAlgorithmException
| InvalidKeySpecException
| IllegalArgumentException
| NullPointerException ex) {
throw new IllegalArgumentException(ex);
}
}
/**
* Simplifies common PBKDF2 tasks
*
* @param algorithm PBKDF2With<Hmac Algorithm>
* @param provider Hash provider, null is use default provider
* @param password raw password
* @param salt password salt
* @param iterations iteration
* @param keySize key size
* @return PBKDF2With<Hmac Algorithm> password string
* @throws IllegalArgumentException password or salt is null, number is wrong or could not execute
* task.
*/
public static String pbkdf2HashString(
HmacAlgorithms algorithm,
Provider provider,
char[] password,
byte[] salt,
int iterations,
int keySize)
throws IllegalArgumentException {
return new String(
HexUtil.encode(
pbkdf2Hash(algorithm, provider, password, salt, iterations, keySize)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy