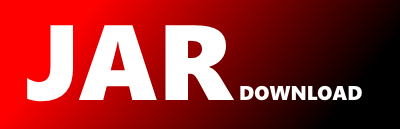
org.seppiko.commons.utils.crypto.NMPUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto;
import java.io.Serial;
import java.io.Serializable;
import java.security.NoSuchAlgorithmException;
import java.util.Objects;
import org.seppiko.commons.utils.StringUtil;
/**
* Algorithm Name/Mode/Padding utility.
*
* @author Leonard Woo
*/
public class NMPUtil implements Serializable {
@Serial
private static final long serialVersionUID = -215141540814890802L;
/** algorithm name */
private final String name;
/** algorithm mode */
private final String mode;
/** algorithm padding */
private final String padding;
/**
* Parser algorithm string.
*
* @param algorithm {@code Name[/Mode[/Padding]]}.
* @throws NoSuchAlgorithmException Algorithm String format is wrong.
* @throws NullPointerException algorithm is {@code null}.
*/
public NMPUtil(String algorithm) throws NoSuchAlgorithmException, NullPointerException {
if (StringUtil.isNullOrEmpty(algorithm)) {
throw new NullPointerException("algorithm must not be null.");
}
if (algorithm.indexOf('/') < 0) {
this.name = algorithm;
this.mode = null;
this.padding = null;
} else {
String[] strs = algorithm.split("/");
if (strs.length == 2) {
this.name = strs[0];
this.mode = strs[1];
this.padding = null;
} else if (strs.length == 3) {
this.name = strs[0];
this.mode = strs[1];
this.padding = strs[2];
} else {
throw new NoSuchAlgorithmException("Algorithm string parser failed.");
}
}
}
/**
* Initialization Name/Mode/Padding
*
* @param name Name.
* @param mode Mode.
* @param padding Padding.
* @throws NullPointerException Name is {@code null}.
*/
public NMPUtil(String name, String mode, String padding) throws NullPointerException {
if (StringUtil.isNullOrEmpty(name)) {
throw new NullPointerException("name must not be null.");
}
this.name = name;
this.mode = mode;
this.padding = padding;
}
/**
* @return Name
*/
public String getName() {
return name;
}
/**
* @return Mode
*/
public String getMode() {
return mode;
}
/**
* @return Padding
*/
public String getPadding() {
return padding;
}
/**
* Check {@link NMPUtil} instance
*
* @param o {@link NMPUtil} instance.
* @return true, if object is {@link NMPUtil} and equals.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NMPUtil that = (NMPUtil) o;
return Objects.equals(name, that.name)
&& Objects.equals(mode, that.mode)
&& Objects.equals(padding, that.padding);
}
/** NMPUtil object hashcode */
@Override
public int hashCode() {
return Objects.hash(name, mode, padding);
}
/** Re-splicing algorithm name */
@Override
public String toString() {
return StringUtil.convertJoinerString("/", name, mode, padding);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy