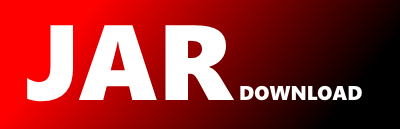
org.seppiko.commons.utils.crypto.spec.KeySpecUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto.spec;
import java.math.BigInteger;
import java.security.KeyFactory;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.security.spec.XECPublicKeySpec;
import java.util.Objects;
import javax.crypto.spec.PBEKeySpec;
import javax.crypto.spec.SecretKeySpec;
import org.seppiko.commons.utils.StringUtil;
/**
* Key Specification implement util
*
* @author Leonard Woo
*/
public class KeySpecUtil {
private KeySpecUtil() {}
/**
* SecretKeySpec object
*
* @see SecretKeyFactory
* Algorithms
* @see SecretKeySpec
* @param key the key material of the secret key
* @param algorithm the name of the secret-key algorithm to be associated with the given key
* material
* @return SecretKeySpec object
* @throws NullPointerException if key is null.
* @throws IllegalArgumentException if key is empty, or too short, i.e. key.length-offset<len.
*/
public static SecretKeySpec getSecret(byte[] key, String algorithm)
throws NullPointerException, IllegalArgumentException {
if (Objects.isNull(key)) {
throw new NullPointerException("key missing");
}
return getSecret(key, 0, key.length, algorithm);
}
/**
* SecretKeySpec object
*
* @see SecretKeyFactory
* Algorithms
* @see SecretKeySpec
* @param key the key material of the secret key
* @param off the offset in key where the key material starts
* @param len the length of the key material
* @param algorithm the name of the secret-key algorithm to be associated with the given key
* material
* @return SecretKeySpec object
* @throws NullPointerException if key is null or algorithm is null or empty.
* @throws IllegalArgumentException if key is empty, or too short, i.e. key.length-offset<len.
*/
public static SecretKeySpec getSecret(byte[] key, int off, int len, String algorithm)
throws NullPointerException, IllegalArgumentException {
if (Objects.isNull(key) || StringUtil.nonText(algorithm)) {
throw new NullPointerException("Missing argument");
}
if (key.length == 0) {
throw new IllegalArgumentException("Empty key");
}
if ((key.length - off) < len) {
throw new IllegalArgumentException("Invalid offset/length combination");
}
if (len < 0) {
throw new ArrayIndexOutOfBoundsException("len is negative");
}
return new SecretKeySpec(key, off, len, algorithm);
}
/**
* PBEKeySpec object
*
* @see PBEKeySpec
* @param password the password
* @return PBEKeySpec object
*/
public static PBEKeySpec getPBE(char[] password) {
return new PBEKeySpec(password);
}
/**
* PBEKeySpec object
*
* @see PBEKeySpec
* @param password the password
* @param salt the salt
* @param iterationCount the iteration count
* @param keyLength the to-be-derived key length
* @return PBEKeySpec object
* @throws NullPointerException if salt is null.
* @throws IllegalArgumentException if salt is empty, i.e. 0-length, iterationCount or keyLength
* is not positive.
*/
public static PBEKeySpec getPBE(char[] password, byte[] salt, int iterationCount, int keyLength)
throws NullPointerException, IllegalArgumentException {
if (Objects.isNull(password)) {
throw new NullPointerException("salt missing");
}
return new PBEKeySpec(password, salt, iterationCount, keyLength);
}
/**
* PKCS8EncodedKeySpec object
*
* @see PKCS8EncodedKeySpec
* @param key the key, which is assumed to be encoded according to the PKCS #8 standard. The
* contents of the array are copied to protect against subsequent modification.
* @return PKCS8EncodedKeySpec object
* @throws NullPointerException if key is null.
*/
public static PKCS8EncodedKeySpec getPKCS8(byte[] key) throws NullPointerException {
if (Objects.isNull(key)) {
throw new NullPointerException("key missing");
}
return new PKCS8EncodedKeySpec(key);
}
/**
* PKCS8EncodedKeySpec object
*
* @see PKCS8EncodedKeySpec
* @param key the key, which is assumed to be encoded according to the PKCS #8 standard. The
* contents of the array are copied to protect against subsequent modification.
* @param algorithm the algorithm name of the encoded private key.
* @return PKCS8EncodedKeySpec object
* @throws NullPointerException if key is null
* @throws IllegalArgumentException if algorithm is the empty string
*/
public static PKCS8EncodedKeySpec getPKCS8(byte[] key, String algorithm)
throws NullPointerException, IllegalArgumentException {
if (Objects.isNull(key)) {
throw new NullPointerException("key missing");
}
if (StringUtil.nonText(algorithm)) {
throw new IllegalArgumentException("algorithm missing");
}
return new PKCS8EncodedKeySpec(key, algorithm);
}
/**
* X509EncodedKeySpec object
*
* @see X509EncodedKeySpec
* @param key the key, which is assumed to be encoded according to the X.509 standard. The
* contents of the array are copied to protect against subsequent modification.
* @return X509EncodedKeySpec object
* @throws NullPointerException if key is null
*/
public static X509EncodedKeySpec getX509(byte[] key) throws NullPointerException {
if (Objects.isNull(key)) {
throw new NullPointerException("key missing");
}
return new X509EncodedKeySpec(key);
}
/**
* X509EncodedKeySpec object
*
* @see X509EncodedKeySpec
* @param key the key, which is assumed to be encoded according to the X.509 standard. The
* contents of the array are copied to protect against subsequent modification.
* @param algorithm the algorithm name of the encoded public key.
* @return X509EncodedKeySpec object
* @throws NullPointerException if key is null
* @throws IllegalArgumentException if algorithm is the empty string
*/
public static X509EncodedKeySpec getX509(byte[] key, String algorithm)
throws NullPointerException, IllegalArgumentException {
if (Objects.isNull(key)) {
throw new NullPointerException("key missing");
}
if (StringUtil.nonText(algorithm)) {
throw new IllegalArgumentException("algorithm missing");
}
return new X509EncodedKeySpec(key, algorithm);
}
/**
* Convert X509 to public key
*
* @param keyFactory KeyFactory object
* @param keySpec X509 object
* @return public key object
* @throws InvalidKeySpecException if the given key specification is inappropriate for this key
* factory to produce a public key.
*/
public static PublicKey genPublicKey(KeyFactory keyFactory, X509EncodedKeySpec keySpec)
throws InvalidKeySpecException {
return keyFactory.generatePublic(keySpec);
}
/**
* Convert PKC8 to private key
*
* @param keyFactory KeyFactory object
* @param keySpec PKC8 object
* @return private key object
* @throws InvalidKeySpecException if the given key specification is inappropriate for this key
* factory to produce a public key.
*/
public static PrivateKey genPrivateKey(KeyFactory keyFactory, PKCS8EncodedKeySpec keySpec)
throws InvalidKeySpecException {
return keyFactory.generatePrivate(keySpec);
}
/**
* Construct a public key spec using the supplied parameters and u coordinate.
*
* @param paramSpec the algorithm parameters
* @param u the u-coordinate of the point
* @return XECPublicKeySpec object
*/
public static XECPublicKeySpec getXECPub(AlgorithmParameterSpec paramSpec, BigInteger u) {
return new XECPublicKeySpec(paramSpec, u);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy