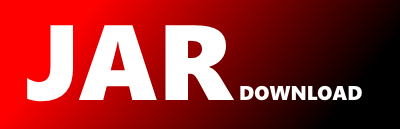
org.seppiko.commons.utils.crypto.spec.ParameterSpacUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.crypto.spec;
import java.math.BigInteger;
import java.security.InvalidParameterException;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.ECField;
import java.security.spec.ECParameterSpec;
import java.security.spec.ECPoint;
import java.security.spec.EdDSAParameterSpec;
import java.security.spec.EllipticCurve;
import javax.crypto.spec.ChaCha20ParameterSpec;
import javax.crypto.spec.DHParameterSpec;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.OAEPParameterSpec;
import javax.crypto.spec.PBEParameterSpec;
import javax.crypto.spec.PSource;
import javax.crypto.spec.PSource.PSpecified;
/**
* Parameter Specification implement util
*
* @see Java Security Standard Algorithm Names
* @author Leonard Woo
*/
public class ParameterSpacUtil {
private ParameterSpacUtil() {}
/**
* GCMParameterSpec instance
*
* @see GCMParameterSpec
* @param tLen GCM authentication tag length
* @param src the IV source buffer
* @return GCMParameterSpec instance
* @throws NullPointerException src is null
* @throws IllegalArgumentException if tLen is negative, src is null, len or offset is negative,
* or the sum of offset and len is greater than the length of the src byte array.
*/
public static GCMParameterSpec getGCM(int tLen, byte[] src)
throws NullPointerException, IllegalArgumentException {
if (src == null) {
throw new NullPointerException("IV source buffer missing");
}
return getGCM(tLen, src, 0, src.length);
}
/**
* GCMParameterSpec instance
*
* @see GCMParameterSpec
* @param tLen GCM authentication tag length
* @param src the IV source buffer
* @param off the offset in src where the IV starts
* @param len the number of IV bytes
* @return GCMParameterSpec instance
* @throws IllegalArgumentException if tLen is negative, src is null, len or offset is negative,
* or the sum of offset and len is greater than the length of the src byte array.
*/
public static GCMParameterSpec getGCM(int tLen, byte[] src, int off, int len)
throws IllegalArgumentException {
return new GCMParameterSpec(tLen, src, off, len);
}
/**
* IvParameterSpec instance
*
* @see IvParameterSpec
* @param iv the buffer with the IV
* @return IvParameterSpec instance
* @throws NullPointerException if iv is null
* @throws IllegalArgumentException if iv is null or (iv.length - offset < len)
* @throws ArrayIndexOutOfBoundsException is thrown if offset or len index bytes outside the iv.
*/
public static IvParameterSpec getIV(byte[] iv) throws NullPointerException {
if (iv == null) {
throw new NullPointerException("IV missing");
}
return getIV(iv, 0, iv.length);
}
/**
* IvParameterSpec instance
*
* @see IvParameterSpec
* @param iv the buffer with the IV
* @param off the offset in iv where the IV starts
* @param len the number of IV bytes
* @return IvParameterSpec instance
* @throws IllegalArgumentException if iv is null or (iv.length - offset < len)
* @throws ArrayIndexOutOfBoundsException is thrown if offset or len index bytes outside the iv.
*/
public static IvParameterSpec getIV(byte[] iv, int off, int len) {
return new IvParameterSpec(iv, off, len);
}
/**
* PBEParameterSpec instance
*
* @see PBEParameterSpec
* @param salt the salt. The contents of salt are copied to protect against subsequent
* modification.
* @param iterationCount the iteration count.
* @return PBEParameterSpec instance
* @throws NullPointerException if salt is null
*/
public static PBEParameterSpec getPBE(byte[] salt, int iterationCount)
throws NullPointerException {
return getPBE(salt, iterationCount, null);
}
/**
* PBEParameterSpec object
*
* @see PBEParameterSpec
* @param salt the salt. The contents of salt are copied to protect against subsequent
* modification.
* @param iterationCount the iteration count.
* @param paramSpec the cipher algorithm parameter specification
* @return PBEParameterSpec instance
* @throws NullPointerException if salt is null
*/
public static PBEParameterSpec getPBE(
byte[] salt, int iterationCount, AlgorithmParameterSpec paramSpec)
throws NullPointerException {
if (salt == null) {
throw new NullPointerException("Salt missing");
}
return new PBEParameterSpec(salt, iterationCount, paramSpec);
}
/**
* DHParameterSpec instance
*
* @see DHParameterSpec
* @param p the prime modulus
* @param g the base generator
* @return DHParameterSpec instance
*/
public static DHParameterSpec getDH(BigInteger p, BigInteger g) {
return getDH(p, g, 0);
}
/**
* DHParameterSpec instance
*
* @see DHParameterSpec
* @param p the prime modulus
* @param g the base generator
* @param l the size in bits of the random exponent (private value)
* @return DHParameterSpec instance
*/
public static DHParameterSpec getDH(BigInteger p, BigInteger g, int l) {
return new DHParameterSpec(p, g, l);
}
/**
* ECParameterSpec instance
*
* @see ECParameterSpec
* @param field the finite field that this elliptic curve is over.
* @param fristCoefficient the first coefficient of this elliptic curve.
* @param secondfficient the second coefficient of this elliptic curve.
* @param affineX the affine x-coordinate.
* @param affineY the affine y-coordinate.
* @param generator the order of the generator.
* @param cofactor the cofactor.
* @return ECParameterSpec instance
* @throws NullPointerException if same parameter is null
* @throws IllegalArgumentException if {@code generator} or {@code cofactor} is not positive or
* {@code affineX} or {@code affineY} is not null and not in {@code field}.
*/
public static ECParameterSpec getEC(
ECField field,
BigInteger fristCoefficient,
BigInteger secondfficient,
BigInteger affineX,
BigInteger affineY,
BigInteger generator,
int cofactor)
throws NullPointerException, IllegalArgumentException {
EllipticCurve curve = new EllipticCurve(field, fristCoefficient, secondfficient);
ECPoint point = new ECPoint(affineX, affineY);
return new ECParameterSpec(curve, point, generator, cofactor);
}
/**
* ChaCha20ParameterSpec instance
*
* @see ChaCha20ParameterSpec
* @param nonce a 12-byte nonce value
* @param counter the initial counter value
* @return ChaCha20ParameterSpec instance.
* @throws NullPointerException {@code nonce} must be not {@code null}.
* @throws IllegalArgumentException {@code nonce} must be 12 bytes in length.
*/
public static ChaCha20ParameterSpec getChacha20(byte[] nonce, int counter)
throws NullPointerException, IllegalArgumentException {
if (nonce == null) {
throw new NullPointerException("Nonce must be not null");
}
if (nonce.length != 12) {
throw new IllegalArgumentException("Nonce must be 12-bytes in length");
}
return new ChaCha20ParameterSpec(nonce, counter);
}
/**
* Constructs a parameter set for OAEP padding as defined in
* the PKCS #1 standard using the specified message digest
* algorithm mdName
, mask generation function
* algorithm mgfName
, parameters for the mask
* generation function mgfSpec
, and source of
* the encoding input P pSrc
.
*
* @see OAEPParameterSpec
* @param mdName the algorithm name for the message digest.
* @param mgfName the algorithm name for the mask generation
* function.
* @param mgfSpec the parameters for the mask generation function.
* If null is specified, null will be returned by getMGFParameters().
* @param pSrc the source of the encoding input P.
* @return OAEPParameterSpec instance.
* @exception NullPointerException if mdName
,
* mgfName
, or pSrc
is null.
*/
public static OAEPParameterSpec getOAEP(String mdName, String mgfName,
AlgorithmParameterSpec mgfSpec, PSource pSrc) throws NullPointerException {
if (pSrc == null) {
pSrc = PSpecified.DEFAULT;
}
return new OAEPParameterSpec(mdName, mgfName, mgfSpec, pSrc);
}
/**
* Construct an {@code EdDSAParameterSpec} by specifying whether the prehash mode
* is used. No context is provided so this constructor specifies a mode
* in which the context is null. Note that this mode may be different than the mode
* in which an empty array is used as the context.
*
* @param prehash whether the prehash mode is specified.
* @param context the context is copied and bound to the signature.
* @return EdDSAParameterSpec instance.
* @throws InvalidParameterException if context length is greater than 255.
*/
public static EdDSAParameterSpec getEdDSA(boolean prehash, byte[] context)
throws InvalidParameterException {
if (context == null) {
return new EdDSAParameterSpec(prehash);
}
return new EdDSAParameterSpec(prehash, context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy