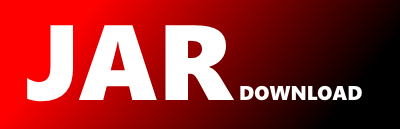
org.seppiko.commons.utils.jdbc.ConnectionUtil Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.seppiko.commons.utils.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Objects;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
import org.seppiko.commons.utils.StringUtil;
/**
* JDBC Connection util
*
* @author Leonard Woo
*/
public class ConnectionUtil {
private ConnectionUtil() {}
/**
* Create Connection
*
* @param driverClazzName driver class name.
* @param url JDBC URL.
* jdbc:subprotocol:subname
* @param username Database username.
* @param password Database password.
* @return a connection to the URL.
* @throws SQLException if a database access error occurs.
* @throws ClassNotFoundException if the driver class cannot be located.
* @throws IllegalArgumentException if the driver class is null.
* @throws NullPointerException JDBC URL is null.
*/
public static Connection getConnection(String driverClazzName, String url, String username, String password)
throws SQLException, ClassNotFoundException, IllegalArgumentException, NullPointerException {
if (StringUtil.isNullOrEmpty(driverClazzName)) {
throw new IllegalArgumentException("Driver class name must be not null");
}
Class.forName(driverClazzName);
return getConnection(url, username, password);
}
/**
* Create Connection
*
* @param url JDBC URL.
* jdbc:subprotocol:subname
.
* @param username Database username.
* @param password Database password.
* @return a connection to the URL.
* @throws SQLException if a database access error occurs.
* @throws NullPointerException JDBC URL is null.
*/
public static Connection getConnection(String url, String username, String password)
throws SQLException, NullPointerException {
Objects.requireNonNull(url, "JDBC url must be not null.");
return DriverManager.getConnection(url, username, password);
}
/**
* Get DataSource with JNDI name
*
* @param jndiName JNDI name.
* @return a datasource to the jndi name.
* @throws NamingException if a naming exception is encountered or in a naming exception is
* encountered.
*/
public static DataSource getDataSourceWithJNDI(String jndiName)
throws NamingException {
return (DataSource) new InitialContext().lookup(jndiName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy