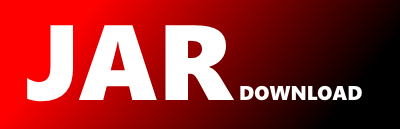
org.javafmi.proxy.v1.Callbacks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.proxy.v1;
import com.sun.jna.*;
import java.util.HashSet;
import java.util.Set;
interface Callbacks extends Library {
interface FMIStatus {
int fmiOK = 0;
int fmiWarning = 1;
int fmiDiscard = 2;
int fmiError = 3;
int fmiFatal = 4;
int fmiPending = 5;
}
interface FMIStatusKind {
int fmiDoStepStatus = 0;
int fmiPendingStatus = 1;
int fmiLastSuccessfulTime = 2;
}
interface CallbackLogger extends Callback {
void record(Pointer fmiComponent, String instanceName, int status, String category, String message, Pointer parameters);
}
interface CallbackAllocateMemory extends Callback {
Pointer allocate(Callbacks.Size_T numberOfObjects, Callbacks.Size_T size);
}
interface CallbackFreeMemory extends Callback {
void free(Pointer object);
}
interface CallbackStepFinished extends Callback {
void apply(Pointer fmiComponent, int status);
}
class Logger implements CallbackLogger {
@Override
public void record(Pointer fmiComponent, String logSource, int status, String category, String message, Pointer parameters) {
System.out.print(logSource + " reports: " + category);
System.out.format(" with status %d \n", status);
if (parameters != null)
System.out.println("There was a problem inside the fmu, but some information cannot be retrieved");
System.out.println(message);
}
}
class AllocateMemory implements CallbackAllocateMemory {
public static Set onMemoryAllocatedObjects = new HashSet<>();
private int MEMORY_OFFSET = 4;
@Override
public Pointer allocate(Size_T numberOfObjects, Size_T objectSize) {
Memory memoryToShare = new Memory(fixNumberOfObjects(numberOfObjects.intValue()) * objectSize.intValue());
memoryToShare.align(MEMORY_OFFSET);
memoryToShare.clear();
onMemoryAllocatedObjects.add(memoryToShare);
return memoryToShare;
}
private int fixNumberOfObjects(int numberOfObjects) {
return (numberOfObjects <= 0) ? 1 : numberOfObjects;
}
}
class FreeMemory implements CallbackFreeMemory {
@Override
public void free(Pointer object) {
AllocateMemory.onMemoryAllocatedObjects.remove(object);
System.gc();
}
}
class StepFinished implements CallbackStepFinished {
@Override
public void apply(Pointer fmiComponent, int status) {
}
}
class Size_T extends IntegerType {
public Size_T() {
this(0);
}
public Size_T(long size) {
super(com.sun.jna.Native.SIZE_T_SIZE, size);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy