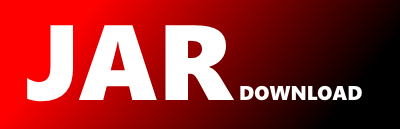
org.javafmi.wrapper.variables.VariableWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.wrapper.variables;
import org.javafmi.modeldescription.ModelDescription;
import org.javafmi.modeldescription.ScalarVariable;
import org.javafmi.proxy.FmiProxy;
import org.javafmi.proxy.Status;
import org.javafmi.wrapper.Variable;
public class VariableWriter {
private final ModelDescription modelDescription;
private FmiProxy fmiProxy;
public VariableWriter(FmiProxy fmiProxy, ModelDescription modelDescription) {
this.fmiProxy = fmiProxy;
this.modelDescription = modelDescription;
}
public Status write(String name, Object value) {
ScalarVariable modelVariable = modelDescription.getModelVariable(name);
int valueReference = modelVariable.getValueReference();
switch (modelVariable.getTypeName()) {
case "Real":
return fmiProxy.setReal(new int[]{valueReference}, new double[]{getDoubleValue(value)});
case "Integer":
return fmiProxy.setInteger(new int[]{valueReference}, new int[]{getIntValue(value)});
case "Boolean":
return fmiProxy.setBoolean(new int[]{valueReference}, new boolean[]{getBooleanValue(value)});
case "String":
return fmiProxy.setString(new int[]{valueReference}, new String[]{getStringValue(value)});
default:
break;
}
return Status.ERROR;
}
public void write(Variable... variablesToWrite) {
double[] values = extractValues(variablesToWrite);
int[] valueReferences = modelDescription.getValueReferences(extractNames(variablesToWrite));
fmiProxy.setReal(valueReferences, values);
}
private String[] extractNames(Variable[] variablesToWrite) {
String[] names = new String[variablesToWrite.length];
for (int i = 0; i < names.length; i++) {
names[i] = variablesToWrite[i].getName();
}
return names;
}
private double[] extractValues(Variable[] variablesToWrite) {
double[] values = new double[variablesToWrite.length];
for (int i = 0; i < values.length; i++) {
values[i] = (Double) variablesToWrite[i].getValue();
}
return values;
}
private double getDoubleValue(Object value) {
return Double.parseDouble(getStringValue(value));
}
private Integer getIntValue(Object value) {
return Integer.parseInt(getStringValue(value));
}
private Boolean getBooleanValue(Object value) {
return Boolean.parseBoolean(getStringValue(value));
}
private String getStringValue(Object value) {
return String.valueOf(value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy