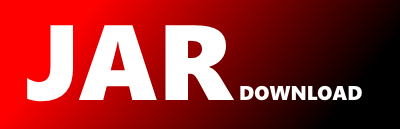
org.javafmi.kernel.Unzipper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.kernel;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import static org.javafmi.kernel.Files.createDirectory;
import static org.javafmi.kernel.Files.createFile;
import static org.javafmi.kernel.OS.*;
public class Unzipper {
private File file;
public Unzipper(File file) {
this.file = file;
if (!file.exists()) throw new RuntimeException("File " + file.getAbsolutePath() + " does not exists");
}
private ZipFile openZipForFile(File file) {
try {
return new ZipFile(file);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public File unzip(String fileName, File outFolder) {
ZipFile zip = openZipForFile(file);
createDirectory(outFolder);
File file = extractEntryToFile(zip, zip.getEntry(fileName), createFile(outFolder, fileName));
close(zip);
return file;
}
public File unzipAllFilesInDirectory(String directoryToUnZip, File workingDirectory) {
ZipFile zip = openZipForFile(file);
createDirectory(workingDirectory);
File extractedDirectory = createDirectory(workingDirectory, directoryToUnZip);
Enumeration extends ZipEntry> entries = zip.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
String entryName = entry.getName().replace("\\", "/");
if (entryName.startsWith(directoryToUnZip.replace("\\","/")) && !entryName.equals(directoryToUnZip + "/")) {
if (entry.isDirectory()) createDirectory(workingDirectory, entryName);
else extractEntryToFile(zip, entry, createFile(workingDirectory, entryName));
}
}
close(zip);
return extractedDirectory;
}
public File unzipAll(File outputDirectory) {
ZipFile zip = openZipForFile(file);
createDirectory(outputDirectory);
Enumeration extends ZipEntry> entries = zip.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
if (entry.isDirectory()) createDirectory(outputDirectory, entry.getName());
else extractEntryToFile(zip, entry, createFile(outputDirectory, entry.getName()));
}
close(zip);
return outputDirectory;
}
private File extractEntryToFile(ZipFile zip, ZipEntry entry, File outFile) {
try {
BufferedInputStream source = Files.bufferedInputStream(zip.getInputStream(entry));
BufferedOutputStream target = Files.bufferedOutputStream(Files.fileOutputStream(outFile));
Files.copyFromTo(source, target);
Files.close(source);
Files.close(target);
setExecutionPermission(outFile);
return outFile;
} catch (Exception e) {
throw new RuntimeException("Impossible to extract " + outFile.getName(), e);
}
}
private void setExecutionPermission(File outFile) throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, IllegalAccessException {
if (isLinux32() || isLinux64() || isMac()) {
Class> fspClass = Class.forName("java.util.prefs.FileSystemPreferences");
Method chmodMethod = fspClass.getDeclaredMethod("chmod", String.class, Integer.TYPE);
chmodMethod.setAccessible(true);
chmodMethod.invoke(null, outFile.getAbsolutePath(), 0777);
}
}
public void close(ZipFile zip) {
try {
zip.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy