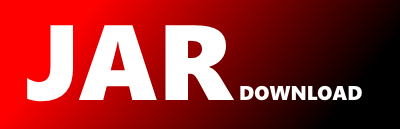
org.javafmi.proxy.FmiProxyV21 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.proxy;
import com.sun.jna.Structure;
import java.util.Arrays;
import java.util.List;
public interface FmiProxyV21 extends FmiProxyV2 {
RealsAtEvent getRealsAtEvent(double communicationPoint, int... valueReference);
IntegersAtEvent getIntegersAtEvent(double communicationPoint, int... valueReference);
BooleansAtEvent getBooleansAtEvent(double communicationPoint, int... valueReference);
StringsAtEvent getStringsAtEvent(double communicationPoint, int... valueReference);
HybridDoStepStatus hybridDoStep(double communicationPoint, double stepSize);
double getNextEventTime();
class HybridDoStepStatus {
Status status;
boolean occurredEvent;
double endTime;
public HybridDoStepStatus(Status status, boolean occurredEvent, double endTime) {
this.status = status;
this.occurredEvent = occurredEvent;
this.endTime = endTime;
}
public Status getStatus() {
return status;
}
public boolean isOccurredEvent() {
return occurredEvent;
}
public double getEndTime() {
return endTime;
}
}
Status newDiscreteStates(EventInfo info);
class EventInfo extends Structure {
public boolean newDiscreteStatesNeeded, terminateSimulation, nominalsOfContinuousStatesChanged, valuesOfContinuousStatesChanged, nextEventTimeDefined;
public double nextEventTime;
public EventInfo(boolean newDiscreteStatesNeeded, boolean terminateSimulation, boolean nominalsOfContinuousStatesChanged, boolean valuesOfContinuousStatesChanged, boolean nextEventTimeDefined, double nextEventTime) {
super();
this.newDiscreteStatesNeeded = newDiscreteStatesNeeded;
this.terminateSimulation = terminateSimulation;
this.nominalsOfContinuousStatesChanged = nominalsOfContinuousStatesChanged;
this.valuesOfContinuousStatesChanged = valuesOfContinuousStatesChanged;
this.nextEventTimeDefined = nextEventTimeDefined;
this.nextEventTime = nextEventTime;
}
public boolean isNewDiscreteStatesNeeded() {
return newDiscreteStatesNeeded;
}
public boolean isTerminateSimulation() {
return terminateSimulation;
}
public boolean isNominalsOfContinuousStatesChanged() {
return nominalsOfContinuousStatesChanged;
}
public boolean isValuesOfContinuousStatesChanged() {
return valuesOfContinuousStatesChanged;
}
public boolean isNextEventTimeDefined() {
return nextEventTimeDefined;
}
public double nextEventTime() {
return nextEventTime;
}
@Override
protected List getFieldOrder() {
return Arrays.asList("newDiscreteStatesNeeded", "terminateSimulation", "nominalsOfContinuousStatesChanged", "valuesOfContinuousStatesChanged", "nextEventTimeDefined", "nextEventTime");
}
}
class VariablesAtEvent {
private final boolean eventOccurred;
public VariablesAtEvent(boolean eventOccurred) {
this.eventOccurred = eventOccurred;
}
public boolean isEventOccurred() {
return eventOccurred;
}
}
class RealsAtEvent extends VariablesAtEvent {
private final double[] beforeEvent;
private final double[] afterEvent;
public RealsAtEvent(boolean eventOccurred, double[] beforeEvent, double[] afterEvent) {
super(eventOccurred);
this.beforeEvent = beforeEvent;
this.afterEvent = afterEvent;
}
public double[] getBeforeEvent() {
return beforeEvent;
}
public double[] getAfterEvent() {
return afterEvent;
}
}
class IntegersAtEvent extends VariablesAtEvent {
private final int[] beforeEvent;
private final int[] afterEvent;
public IntegersAtEvent(boolean eventOccurred, int[] beforeEvent, int[] afterEvent) {
super(eventOccurred);
this.beforeEvent = beforeEvent;
this.afterEvent = afterEvent;
}
public int[] getBeforeEvent() {
return beforeEvent;
}
public int[] getAfterEvent() {
return afterEvent;
}
}
class BooleansAtEvent extends VariablesAtEvent {
private final boolean[] beforeEvent;
private final boolean[] afterEvent;
public BooleansAtEvent(boolean eventOccurred, boolean[] beforeEvent, boolean[] afterEvent) {
super(eventOccurred);
this.beforeEvent = beforeEvent;
this.afterEvent = afterEvent;
}
public boolean[] getBeforeEvent() {
return beforeEvent;
}
public boolean[] getAfterEvent() {
return afterEvent;
}
}
class StringsAtEvent extends VariablesAtEvent {
private final String[] beforeEvent;
private final String[] afterEvent;
public StringsAtEvent(boolean eventOccurred, String[] beforeEvent, String[] afterEvent) {
super(eventOccurred);
this.beforeEvent = beforeEvent;
this.afterEvent = afterEvent;
}
public String[] getBeforeEvent() {
return beforeEvent;
}
public String[] getAfterEvent() {
return afterEvent;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy