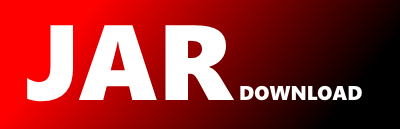
org.javafmi.proxy.FmuFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.proxy;
import org.javafmi.kernel.Files;
import org.javafmi.kernel.OS;
import org.javafmi.kernel.Unzipper;
import org.javafmi.modeldescription.FmiVersion;
import org.javafmi.modeldescription.ModelDescription;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import static java.io.File.separator;
import static java.lang.System.getProperty;
import static java.util.Arrays.asList;
import static org.javafmi.kernel.Convention.FrameworkManufacturer;
import static org.javafmi.modeldescription.ModelDescriptionDeserializer.deserialize;
import static org.javafmi.proxy.ModelDescriptionPeeker.peekVersion;
public class FmuFile {
public static final String BINARIES_FOLDER = "binaries";
public static final String MAC_OS_FOLDER = "darwin";
public static final String WINDOWS_FOLDER = "win";
public static final String LINUX_FOLDER = "linux";
public static final String ARCH_64 = "64";
public static final String ARCH_32 = "32";
public static final String MAC_OS_LIBRARY_EXTENSION = ".dylib";
public static final String WINDOWS_LIBRARY_EXTENSION = ".dll";
public static final String LINUX_LIBRARY_EXTENSION = ".so";
public static final String MISSING_SYSTEM_MESSAGE = "JavaFMI is not prepared to your system";
private static final String MODEL_DESCRIPTION_FILENAME = "modelDescription.xml";
private final File fmuFile;
private final File temporalFolder;
private Unzipper unzipper;
private final ModelDescription modelDescription;
private File libraryFile;
private File resourcesDirectory;
public FmuFile(String filePath) {
this(new File(filePath));
}
public FmuFile(File fmuFile) {
this.fmuFile = fmuFile;
this.temporalFolder = createTemporalFolder();
this.unzipper = new Unzipper(fmuFile);
this.modelDescription = getModelDescription();
this.libraryFile = unzipLibrary();
}
private File createTemporalFolder() {
File folder = new File(System.getProperty("java.io.tmpdir") + "/fmu_" + System.currentTimeMillis() + fmuFile.getName().replace(".fmu", ""));
folder.mkdirs();
return folder;
}
public void deleteTemporalFolder() {
deleteAll(temporalFolder);
}
public String[] getOperatingSystems() {
unzipper.unzipAll(temporalFolder);
return normalize(new File(getBinariesPath()).list());
}
private boolean isJavaFMU() {
return modelDescription.getGenerationTool() != null && modelDescription.getGenerationTool().startsWith(FrameworkManufacturer);
}
public String getLibraryPath() {
if (libraryFile != null) return libraryFile.getAbsolutePath();
unzipLibrary();
return libraryFile.getAbsolutePath();
}
private File unzipLibrary() {
unzipper.unzipAllFilesInDirectory(BINARIES_FOLDER + File.separator + libraryForCurrentOperatingSystem(), temporalFolder);
return new File(getBinariesPath() + libraryForCurrentOperatingSystem() + modelDescription.getModelIdentifier() + getLibraryExtension());
}
private String[] normalize(String[] folders) {
final List result = new ArrayList<>();
if (isJavaFMU()) result.add(OS.Java);
if (folders != null) for (String folder : folders) result.add(normalize(folder));
return result.toArray(new String[result.size()]);
}
private String normalize(String folder) {
return folder.replace("darwin", "Mac").replace("win", "Windows").replace("linux", "Linux");
}
private String getBinariesPath() {
return temporalFolder + separator + BINARIES_FOLDER + separator;
}
private String libraryForCurrentOperatingSystem() {
return getLibraryFolder() + getSystemArchitecture() + separator;
}
private String getLibraryFolder() {
if (systemIsMacOS(getSystemName())) return MAC_OS_FOLDER;
else if (systemIsWindows(getSystemName())) return WINDOWS_FOLDER;
else if (systemIsLinux(getSystemName())) return LINUX_FOLDER;
throw new RuntimeException(MISSING_SYSTEM_MESSAGE);
}
private String getSystemArchitecture() {
return is64bitsArchitecture(getProperty("os.arch").toLowerCase()) ? ARCH_64 : ARCH_32;
}
private String getLibraryExtension() {
if (systemIsMacOS(getSystemName())) return MAC_OS_LIBRARY_EXTENSION;
else if (systemIsWindows(getSystemName())) return WINDOWS_LIBRARY_EXTENSION;
else if (systemIsLinux(getSystemName())) return LINUX_LIBRARY_EXTENSION;
throw new RuntimeException(MISSING_SYSTEM_MESSAGE);
}
private String getSystemName() {
return getProperty("os.name").toLowerCase();
}
private boolean systemIsMacOS(String operatingSystem) {
return operatingSystem.startsWith("mac");
}
private boolean systemIsWindows(String operatingSystemName) {
return operatingSystemName.startsWith("win");
}
private boolean systemIsLinux(String operatingSystemName) {
return operatingSystemName.startsWith("linux");
}
private boolean is64bitsArchitecture(String architecture) {
return architecture.contains(ARCH_64);
}
public String getWorkingDirectoryPath() {
return temporalFolder.getAbsolutePath();
}
private void deleteAll(File file) {
if (file.isDirectory()) asList(file.listFiles()).forEach(this::deleteAll);
file.delete();
}
public ModelDescription getModelDescription() {
File modelDescriptionFile = unzipper.unzip(MODEL_DESCRIPTION_FILENAME, temporalFolder);
return isV1(modelDescriptionFile) ?
deserialize(modelDescriptionFile).asV1().build() :
deserialize(modelDescriptionFile).asV2().build();
}
private boolean isV1(File modelDescriptionFile) {
return fmuVersion(modelDescriptionFile).equals(FmiVersion.One);
}
private String fmuVersion(File modelDescriptionFile) {
return peekVersion(modelDescriptionFile);
}
public String getResourcesDirectoryPath() {
if (resourcesDirectory == null) resourcesDirectory = getResourcesDirectory(unzipper);
return resourcesDirectory.getAbsolutePath();
}
public File getResourcesDirectory(Unzipper unzipper) {
try {
return unzipper.unzipAllFilesInDirectory("resources", temporalFolder);
} catch (RuntimeException e) {
return Files.createDirectory(temporalFolder, "resources");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy