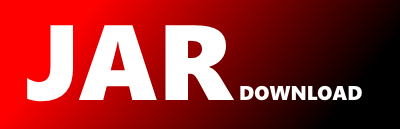
org.javafmi.proxy.v21.CallbackFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.proxy.v21;
import com.sun.jna.Native;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
public class CallbackFunctions extends Structure {
public Callbacks.Logger logger;
public Callbacks.MemoryAllocator memoryAllocator;
public Callbacks.MemoryLiberator memoryLiberator;
public Callbacks.StepFinished stepFinished;
public Pointer componentEnvironment;
public CallbackFunctions() {
super();
this.logger = new Logger();
MemoryHandler memoryHandler = new MemoryHandler();
this.memoryAllocator = memoryHandler::allocateMemory;
this.memoryLiberator = memoryHandler::freeMemory;
this.stepFinished = new StepFinished();
this.componentEnvironment = null;
}
@Override
protected List getFieldOrder() {
return Arrays.asList("logger", "memoryAllocator", "memoryLiberator", "stepFinished", "componentEnvironment");
}
public static class StepFinished implements Callbacks.StepFinished {
@Override
public void stepFinished(Pointer componentEnvironment, int status) {
}
}
public static class MemoryHandler {
private List pointers = new LinkedList<>();
public Pointer allocateMemory(int numberOfObjects, int size) {
pointers.add(0, createPointer((numberOfObjects * size)));
return pointers.get(0);
}
public void freeMemory(Pointer pointer) {
if (pointers.remove(pointer))
freePointer(pointer);
}
private Pointer createPointer(int size) {
size++;
long peer = Native.malloc(size);
Pointer pointer = new Pointer(peer);
pointer.setMemory(0, size, (byte) 0);
return pointer;
}
public void terminate() {
pointers.forEach(this::freePointer);
pointers.clear();
}
private void freePointer(Pointer pointer) {
Native.free(Pointer.nativeValue(pointer));
}
}
public static class Logger implements Callbacks.Logger {
@Override
public void logMessage(Pointer componentEnvironment, String instanceName, int status, String category, String message, Pointer args) {
System.out.println("[" + instanceName + getCategory(category) + "] " + message);
}
private String getCategory(String category) {
return category.isEmpty() ? "" : ":" + category;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy