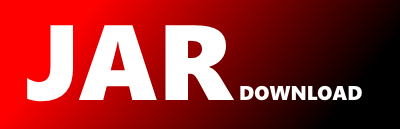
org.javafmi.wrapper.v21.Access Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fmu-wrapper Show documentation
Show all versions of fmu-wrapper Show documentation
javaFMI is a Java Library for the functional mock-up interface (or FMI). FMI defines a standardized interface to be used in computer simulations. The FMI Standard has beed developed by a large number of software companies and research centers that have worked in a cooperation project under the name of MODELISAR. This library addresses the connection of a java application with a FMU (functional mock-up unit).
/*
* Copyright 2013-2016 SIANI - ULPGC
*
* This File is part of JavaFMI Project
*
* JavaFMI Project is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* JavaFMI Project is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JavaFMI. If not, see .
*/
package org.javafmi.wrapper.v21;
import org.javafmi.modeldescription.v2.Capabilities;
import org.javafmi.modeldescription.v2.ModelDescription;
import org.javafmi.modeldescription.v2.ScalarVariable;
import org.javafmi.proxy.FmiProxyV21;
import org.javafmi.proxy.FmuFile;
import org.javafmi.proxy.Status;
import org.javafmi.proxy.v2.State;
import org.javafmi.wrapper.Simulation;
import static java.util.Arrays.fill;
public class Access implements FmuView {
private FmiProxyV21 proxy;
private ModelDescription modelDescription;
private FmuFile fmuFile;
public Access(String fmuPath) {
this(new Simulation(fmuPath));
}
public Access(Simulation simulation) {
this(new ProxyRetriever(simulation).proxy, (ModelDescription) simulation.getModelDescription(), simulation.getFmuFile());
}
Access(FmiProxyV21 proxy, ModelDescription modelDescription, FmuFile fmuFile) {
this.proxy = proxy;
this.modelDescription = modelDescription;
this.fmuFile = fmuFile;
}
public String getVersion() {
return proxy.getVersion();
}
@Deprecated
public double[][] getDirectionalDerivatives(String[] unknownVariables, String[] knownVariables) {
double[][] directionalMatrix = new double[unknownVariables.length][knownVariables.length];
for (int i = 0; i < unknownVariables.length; i++)
for (int j = 0; j < knownVariables.length; j++)
directionalMatrix[i][j] = getDirectionalDerivative(unknownVariables[j], knownVariables[i])[0];
return directionalMatrix;
}
@Deprecated
public double[] getDirectionalDerivative(String knownVariable, String... unknownVariables) {
int unknownVariablesValueReferences[] = modelDescription.getValueReferences(unknownVariables);
int knownVariablesValueReferences[] = modelDescription.getValueReferences(knownVariable);
return proxy.getDirectionalDerivative(unknownVariablesValueReferences, knownVariablesValueReferences, new double[]{1.0});
}
public String getModelName() {
return modelDescription.getModelName();
}
public Status doStep(double simulationTime, double stepSize) {
return proxy.doStep(simulationTime, stepSize);
}
public FmiProxyV21.HybridDoStepStatus hybridDoStep(double simulationTime, double stepSize) {
return proxy.hybridDoStep(simulationTime, stepSize);
}
public Status cancelStep() {
return proxy.cancelStep();
}
public Status terminate() {
return proxy.terminate();
}
public void freeInstance() {
proxy.freeInstance();
fmuFile.deleteTemporalFolder();
}
public Status reset() {
return proxy.reset();
}
public double[] getReal(int... valueReference) {
return proxy.getReal(valueReference);
}
public FmiProxyV21.RealsAtEvent getRealAtEvent(double simulationTime, int... valueReference) {
return proxy.getRealsAtEvent(simulationTime, valueReference);
}
public int[] getInteger(int... valueReference) {
return proxy.getInteger(valueReference);
}
public FmiProxyV21.IntegersAtEvent getIntegerAtEvent(double simulationTime, int... valueReference) {
return proxy.getIntegersAtEvent(simulationTime, valueReference);
}
public boolean[] getBoolean(int... valueReference) {
return proxy.getBoolean(valueReference);
}
public FmiProxyV21.BooleansAtEvent getBooleanAtEvent(double simulationTime, int... valueReference) {
return proxy.getBooleansAtEvent(simulationTime, valueReference);
}
public String[] getString(int... valueReference) {
return proxy.getString(valueReference);
}
public FmiProxyV21.StringsAtEvent getStringAtEvent(double simulationTime, int... valueReference) {
return proxy.getStringsAtEvent(simulationTime, valueReference);
}
public Object getEnumeration(ScalarVariable modelVariable) {
return proxy.getEnumeration(modelVariable);
}
public Status setReal(int[] valueReference, double[] doubleValue) {
return proxy.setReal(valueReference, doubleValue);
}
public Status setInteger(int[] valueReference, int[] intValue) {
return proxy.setInteger(valueReference, intValue);
}
public Status setBoolean(int[] valueReference, boolean[] booleanValue) {
return proxy.setBoolean(valueReference, booleanValue);
}
public Status setString(int[] valueReference, String[] stringValue) {
return proxy.setString(valueReference, stringValue);
}
public double getNextEventTime() {
return proxy.getNextEventTime();
}
public Status newDiscreteStates(FmiProxyV21.EventInfo info) {
return proxy.newDiscreteStates(info);
}
public Status enterInitializationMode() {
return proxy.enterInitializationMode();
}
public Status exitInitializationMode() {
return proxy.exitInitializationMode();
}
public Status setUpExperiment(boolean toleranceDefined, double tolerance, double startTime, boolean stopTimeDefined, double stopTime) {
return proxy.setUpExperiment(toleranceDefined, tolerance, startTime, stopTimeDefined, stopTime);
}
public Status setDebugLogging(boolean loggingOn, int numberOfCategories, String[] categories) {
return proxy.setDebugLogging(loggingOn, categories);
}
public State getState(State state) {
return proxy.getState(state);
}
public Status setState(State state) {
return proxy.setState(state);
}
public Status freeState(State state) {
return proxy.freeState(state);
}
public int getStateSize(State state) {
return proxy.getStateSize(state);
}
public byte[] serializeState(State state) {
return proxy.serializeState(state);
}
public State deserializeState(byte[] serializedState) {
return proxy.deserializeState(serializedState);
}
public void instantiate(double timeout) {
proxy.instantiate(fmuFile.getResourcesDirectoryPath());
}
public Status setRealInputDerivatives(String[] realInputNames, double[] derivatives) {
return proxy.setRealInputDerivatives(modelDescription.getValueReferences(realInputNames), getDefaultOrders(derivatives.length), derivatives);
}
public double[] getRealOutputDerivatives(String[] realInputNames) {
return proxy.getRealOutputDerivatives(modelDescription.getValueReferences(realInputNames), getDefaultOrders(realInputNames.length));
}
private int[] getDefaultOrders(int length) {
int[] orders = new int[length];
fill(orders, 1);
return orders;
}
public int[] getValueReferences(String[] variables) {
return modelDescription.getValueReferences(variables);
}
public int getValueReference(String variable) {
return modelDescription.getValueReference(variable);
}
public double[] getDirectionalDerivative(int[] unknownValueReferences, int[] knownValueReferences, double[] knownDerivative) {
return proxy.getDirectionalDerivative(unknownValueReferences, knownValueReferences, knownDerivative);
}
@Override
public ModelDescription getModelDescription() {
return modelDescription;
}
@Override
public boolean check(Capabilities.Capability capability) {
return modelDescription.check(capability);
}
@Override
public ScalarVariable[] getModelVariables() {
return modelDescription.getModelVariables();
}
@Override
public ScalarVariable getModelVariable(String varName) {
return modelDescription.getModelVariable(varName);
}
private static class ProxyRetriever extends Simulation {
private final FmiProxyV21 proxy;
public ProxyRetriever(Simulation simulation) {
checkVersion(simulation);
this.proxy = (FmiProxyV21) getProxy(simulation);
}
private void checkVersion(Simulation simulation) {
if (getProxy(simulation) instanceof FmiProxyV21) return;
throw new RuntimeException("Access to FMU does not match with Simulation version");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy