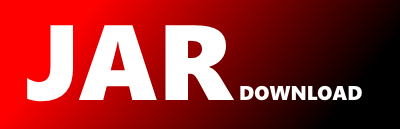
org.signal.libsignal.net.CdsiLookupRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libsignal-client Show documentation
Show all versions of libsignal-client Show documentation
Signal Protocol cryptography library for Java
//
// Copyright 2023 Signal Messenger, LLC.
// SPDX-License-Identifier: AGPL-3.0-only
//
package org.signal.libsignal.net;
import static org.signal.libsignal.internal.FilterExceptions.filterExceptions;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import org.signal.libsignal.internal.Native;
import org.signal.libsignal.protocol.ServiceId;
import org.signal.libsignal.zkgroup.profiles.ProfileKey;
public class CdsiLookupRequest {
final Set previousE164s;
final Set newE164s;
final Set removedE164s;
final Map serviceIds;
final boolean requireAcis;
final byte[] token;
public CdsiLookupRequest(
Set previousE164s,
Set newE164s,
Map serviceIds,
boolean requireAcis,
Optional token) {
if (previousE164s.size() > 0 && !token.isPresent()) {
throw new IllegalArgumentException("You must have a token if you have previousE164s!");
}
this.previousE164s = previousE164s;
this.newE164s = newE164s;
this.removedE164s = Collections.emptySet();
this.serviceIds = serviceIds;
this.requireAcis = requireAcis;
this.token = token.orElse(null);
}
NativeRequest makeNative() {
return new NativeRequest(
this.previousE164s,
this.newE164s,
this.removedE164s,
this.serviceIds,
this.requireAcis,
this.token);
}
class NativeRequest {
private NativeRequest(
Set previousE164s,
Set newE164s,
Set removedE164s,
Map serviceIds,
boolean requireAcis,
byte[] token) {
this.nativeHandle = Native.LookupRequest_new();
for (String e164 : previousE164s) {
Native.LookupRequest_addPreviousE164(this.nativeHandle, e164);
}
for (String e164 : newE164s) {
Native.LookupRequest_addE164(this.nativeHandle, e164);
}
filterExceptions(
() -> {
for (Map.Entry entry : serviceIds.entrySet()) {
Native.LookupRequest_addAciAndAccessKey(
this.nativeHandle,
entry.getKey().toServiceIdFixedWidthBinary(),
entry.getValue().deriveAccessKey());
}
});
Native.LookupRequest_setReturnAcisWithoutUaks(this.nativeHandle, requireAcis);
if (token != null) {
Native.LookupRequest_setToken(this.nativeHandle, token);
}
}
long getHandle() {
return this.nativeHandle;
}
@Override
@SuppressWarnings("deprecation")
protected void finalize() {
Native.LookupRequest_Destroy(this.nativeHandle);
}
private long nativeHandle;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy