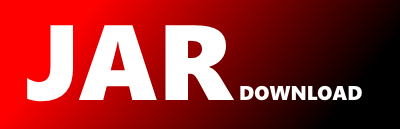
sila_java.servers.barcode_reader.BarcodeReaderServer Maven / Gradle / Ivy
package sila_java.servers.barcode_reader;
import io.grpc.stub.StreamObserver;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import sila2.org.silastandard.SiLAFramework;
import sila2.org.silastandard.core.barcodeprovider.v1.BarcodeProviderGrpc;
import sila2.org.silastandard.core.barcodeprovider.v1.BarcodeProviderOuterClass;
import sila_java.library.core.utils.SiLAErrors;
import sila_java.library.server_base.SiLAServerBase;
import sila_java.library.server_base.identification.ServerInformation;
import sila_java.library.server_base.utils.ArgumentHelper;
import javax.annotation.Nullable;
import java.io.Closeable;
import java.io.IOException;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Map;
import static sila_java.library.core.utils.FileUtils.getResourceContent;
import static sila_java.library.core.utils.SocketUtils.getAvailablePortInRange;
import static sila_java.library.core.utils.Utils.blockUntilStop;
@Slf4j
public class BarcodeReaderServer implements Closeable {
public static final String SERVER_TYPE = "BarcodeReaderMCR12";
private static final int SERVER_PORT = 50052; // Default
private static final int SERVER_PORT_RANGE = 256;
private static final int FIXED_TIMEOUT = 1; // [s] Fixed timeout for barcode reading
private static final int MAX_TIMEOUT = 20; // [s] Maximum allowed timeout for barcode reading
private final BarcodeReaderDriver barcodeReaderDriver = new BarcodeReaderDriver();
private final SiLAServerBase siLAServerBase;
public BarcodeReaderServer(@NonNull final String interfaceName,
final int serverPort,
@Nullable final Path config) {
try {
final Map fdl = new HashMap() {
{
put(
"BarcodeProvider",
getResourceContent("BarcodeProvider.xml")
);
}
};
final ServerInformation serverInfo = new ServerInformation(
SERVER_TYPE,
"A small, camera-based barcode reader with red illumination. Reads most barcode standards.",
"www.unitelabs.ch",
"v0.0"
);
if (config == null) {
this.siLAServerBase = SiLAServerBase.withoutConfig(
serverInfo,
fdl, serverPort, interfaceName,
new ReaderImpl()
);
} else {
this.siLAServerBase = SiLAServerBase.withConfig(
config,
serverInfo,
fdl, serverPort, interfaceName,
new ReaderImpl()
);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
/**
* {@inheritDoc}
*/
@Override
public void close() {
this.siLAServerBase.close();
}
public static void main(final String[] args) {
final ArgumentHelper argumentHelper = new ArgumentHelper(args, SERVER_TYPE);
final int serverPort = argumentHelper.getPort() != null ?
argumentHelper.getPort() :
getAvailablePortInRange(SERVER_PORT, SERVER_PORT + SERVER_PORT_RANGE);
// Start Server
try (final BarcodeReaderServer server = new BarcodeReaderServer(
argumentHelper.getInterface(),
serverPort,
argumentHelper.getConfigFile().orElse(null))
) {
blockUntilStop();
}
System.out.println("termination complete.");
}
class ReaderImpl extends BarcodeProviderGrpc.BarcodeProviderImplBase {
@Override
public void readCode(BarcodeProviderOuterClass.ReadCode_Parameters readCodeParameters,
StreamObserver responseObserver) {
log.info("Trying to read barcode...");
try {
final String code = BarcodeReaderServer.this.barcodeReaderDriver
.getBarcode(FIXED_TIMEOUT);
BarcodeProviderOuterClass.ReadCode_Responses response = BarcodeProviderOuterClass.ReadCode_Responses
.newBuilder()
.setBarcode(SiLAFramework.String
.newBuilder()
.setValue(code)
.build()
).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
} catch (BarcodeNotFoundException e) {
responseObserver.onError(SiLAErrors.generateExecutionError(
"BarcodeNotFound",
e.getMessage(),
"Make sure that the barcode is valid and in front of the reader"));
}
}
@Override
public void readUntil(BarcodeProviderOuterClass.ReadUntil_Parameters readUntilParameters,
StreamObserver responseObserver) {
final long timeout = readUntilParameters.getTimeout().getValue();
if (timeout < 0) {
responseObserver.onError(
SiLAErrors.generateValidationError(
"Timeout",
"Timeout can not be negative!",
"Specify a positive a value")
);
return;
} else if (timeout > MAX_TIMEOUT) {
responseObserver.onError(
SiLAErrors.generateValidationError(
"Timeout",
"Timeout can not be larger than " + MAX_TIMEOUT,
"Specify a value less or equal than " + MAX_TIMEOUT)
);
return;
}
try {
final String code = BarcodeReaderServer.this.barcodeReaderDriver
.getBarcode(timeout);
BarcodeProviderOuterClass.ReadUntil_Responses response = BarcodeProviderOuterClass.ReadUntil_Responses
.newBuilder()
.setBarcode(SiLAFramework.String
.newBuilder()
.setValue(code)
.build()
).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
} catch (BarcodeNotFoundException e) {
responseObserver.onError(SiLAErrors.generateExecutionError(
"BarcodeNotFound",
e.getMessage(),
"Make sure that the barcode is valid and in front of the reader"));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy