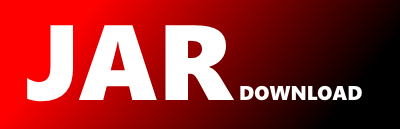
org.simple4j.wsfeeler.model.WSTestStep Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WSFeeler Show documentation
Show all versions of WSFeeler Show documentation
This is a simple end to end Web Service Testing library to test wide variety of web service.
It supports hierarchy of test cases and test steps with wiring of request response objects using templated variable replacement.
The test steps can be Web Service or DB call out of the box with capability to extend to other test steps.
The DB steps are for data setup and assertion of values from the DB.
package org.simple4j.wsfeeler.model;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.simple4j.wsclient.caller.Caller;
import org.simple4j.wsclient.caller.CallerFactory;
import org.simple4j.wsclient.exception.SystemException;
import org.simple4j.wsclient.util.CollectionsPathRetreiver;
import org.simple4j.wsfeeler.core.ConfigLoader;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.ApplicationContext;
import bsh.EvalError;
import bsh.Interpreter;
/**
* This class is a concrete implementation of test step for web service connection
*/
public class WSTestStep extends TestStep
{
private static Logger logger = LoggerFactory.getLogger(WSTestStep.class);
public WSTestStep(Map testStepInputVariables, File testStepInputFile, TestCase parent, TestSuite testSuite)
{
super(testStepInputVariables, testStepInputFile, parent, testSuite);
}
@Override
public boolean execute()
{
logger.info("Entering execute:{}", this.name);
try
{
Caller caller = null;
Object callerBeanIdObj = this.testStepVariables.get("callerBeanId");
if(callerBeanIdObj != null)
{
String callerBeanId = (String) callerBeanIdObj;
caller = getCaller(callerBeanId);
}
else
{
Object callerFactoryBeanIdObj = this.testStepVariables.get("callerFactoryBeanId");
if(callerFactoryBeanIdObj != null)
{
String callerFactoryBeanId = (String) callerFactoryBeanIdObj;
caller = getCallerFactory(callerFactoryBeanId).getCaller();
}
else
{
throw new SystemException("callerBeanId-callerFactoryBeanId-missing", "Both callerBeanId and callerFactoryBeanId is missing in the file:"+this.testStepInputFile);
}
}
logger.info("Calling service");
Map response = caller.call(this.testStepVariables);
logger.info("response from service call:"+response);
Interpreter bsh = new Interpreter();
bsh.setOut(System.out);
bsh.setErr(System.err);
logger.info("Setting step variables");
for (Entry entry : this.testStepVariables.entrySet())
{
try
{
logger.debug("Setting {} : {}",entry.getKey(), entry.getValue());
bsh.set(entry.getKey(), entry.getValue());
} catch (EvalError e)
{
logger.error("Error while setting variable for BeanShell step: {} key: {} vaue: {}", this.name, entry.getKey(), entry.getValue(), e);
}
}
logger.info("Setting step response variables");
for (Entry entry : response.entrySet())
{
this.testStepVariables.put(entry.getKey(), entry.getValue());
try
{
Class extends Object> class1 = null;
if(entry.getValue() != null)
{
class1 = entry.getValue().getClass();
}
logger.debug("Setting {} : {} : {}",entry.getKey(), entry.getValue(),
class1);
bsh.set(entry.getKey(), entry.getValue());
} catch (EvalError e)
{
logger.error("Error while setting output variable for BeanShell step: {} key: {} vaue: {}", this.name, entry.getKey(), entry.getValue(), e);
}
}
File outputPropertiesFile = new File(this.testStepInputFile.getParentFile(),this.testStepInputFile.getName().replace("input.properties", "output.properties"));
String assertionExpression = null;
Map testStepOutputVariables = null;
if(outputPropertiesFile.exists())
{
testStepOutputVariables = ConfigLoader.loadVariables(outputPropertiesFile, this.parent);
logger.info("loaded properties:{}", testStepOutputVariables);
if(testStepOutputVariables.containsKey("ASSERT"))
{
assertionExpression = ""+testStepOutputVariables.get("ASSERT");
testStepOutputVariables.remove("ASSERT");
}
}
if(testStepOutputVariables != null)
{
for (Entry entry : testStepOutputVariables.entrySet()) {
CollectionsPathRetreiver cpr = new CollectionsPathRetreiver();
logger.info("fetching property|{}| from |{}|",""+entry.getValue(), response);
List nestedProperty = cpr.getNestedProperty(response, ""+entry.getValue());
Object value = nestedProperty;
if(nestedProperty.size() == 0)
{
continue;
}
if(nestedProperty.size() == 1)
{
value = nestedProperty.get(0);
}
try
{
logger.info("setting key to intrepreter: {} value: {}", entry.getKey(), value);
bsh.set(entry.getKey(), ""+value);
} catch (EvalError e)
{
logger.error("Error while setting variable for BeanShell step: {} key: {} vaue: {}", this.name, entry.getKey(), value, e);
this.setSuccess(false);
return false;
}
this.testStepVariables.put(entry.getKey(), ""+value);
}
}
if(assertionExpression != null)
{
Object stepResult;
try
{
stepResult = bsh.eval(assertionExpression);
} catch (EvalError e)
{
logger.error("Error while evaluating ASSERT: {} in step: {}", this.name, assertionExpression, e);
this.setSuccess(false);
return false;
}
if(stepResult instanceof Boolean)
{
if(!((Boolean)stepResult))
{
logger.info("FAILURE: Teststep "+ this.name +" for assertion "+assertionExpression);
logger.info("Test step variables are "+testStepOutputVariables);
this.setSuccess(false);
return false;
}
}
else
{
logger.info("FAILURE: Assertion expression "+assertionExpression+" return non-boolean value "+ stepResult + " of type "+stepResult.getClass());
this.setSuccess(false);
return false;
}
}
this.setSuccess(true);
return true;
} catch (IOException e)
{
logger.error("Error while executing step {}", this.name, e);
this.setSuccess(false);
return false;
}
finally
{
logger.info("Exiting execute:{}", this.name);
}
}
private Caller getCaller(String callerBeanId)
{
ApplicationContext ac = this.testSuite.getConnectorsApplicationContext();
return ac.getBean(callerBeanId, Caller.class);
}
private CallerFactory getCallerFactory(String callerFactoryBeanId)
{
ApplicationContext ac = this.testSuite.getConnectorsApplicationContext();
return ac.getBean(callerFactoryBeanId, CallerFactory.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy