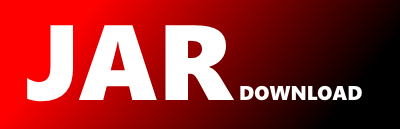
org.simpleflatmapper.jdbi3.SfmRowMapperFactory Maven / Gradle / Ivy
package org.simpleflatmapper.jdbi3;
import org.jdbi.v3.core.config.ConfigRegistry;
import org.jdbi.v3.core.mapper.RowMapper;
import org.jdbi.v3.core.mapper.RowMapperFactory;
import org.simpleflatmapper.jdbc.DynamicJdbcMapper;
import org.simpleflatmapper.jdbc.JdbcMapperFactory;
import org.simpleflatmapper.map.ContextualSourceMapper;
import org.simpleflatmapper.map.SourceMapper;
import org.simpleflatmapper.util.BiPredicate;
import org.simpleflatmapper.util.UnaryFactory;
import java.lang.reflect.Type;
import java.sql.ResultSet;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class SfmRowMapperFactory implements RowMapperFactory {
private static final UnaryFactory> DEFAULT_FACTORY = new UnaryFactory>() {
@Override
public ContextualSourceMapper newInstance(Type type) {
return JdbcMapperFactory.newInstance().newMapper(type);
}
};
private static final BiPredicate DEFAULT_ACCEPT_PREDICATE = new BiPredicate() {
@Override
public boolean test(Type type, ConfigRegistry configRegistry) {
return true;
}
};
private final UnaryFactory> mapperFactory;
private final ConcurrentMap> cache = new ConcurrentHashMap>();
private final BiPredicate acceptsPredicate;
public SfmRowMapperFactory() {
this(DEFAULT_FACTORY);
}
public SfmRowMapperFactory(UnaryFactory> mapperFactory) {
this(DEFAULT_ACCEPT_PREDICATE, mapperFactory);
}
public SfmRowMapperFactory(BiPredicate acceptsPredicate, UnaryFactory> mapperFactory) {
this.mapperFactory = mapperFactory;
this.acceptsPredicate = acceptsPredicate;
}
private RowMapper toRowMapper(ContextualSourceMapper resultSetMapper) {
RowMapper mapper;
if (resultSetMapper instanceof DynamicJdbcMapper) {
mapper = new DynamicRowMapper((DynamicJdbcMapper) resultSetMapper);
} else {
mapper = new StaticRowMapper(resultSetMapper);
}
return mapper;
}
@Override
public Optional> build(Type type, ConfigRegistry configRegistry) {
if (acceptsPredicate.test(type, configRegistry)) {
RowMapper> rowMapper = cache.get(type);
if (rowMapper == null) {
ContextualSourceMapper resultSetMapper = mapperFactory.newInstance(type);
rowMapper = toRowMapper(resultSetMapper);
RowMapper> cachedMapper = cache.putIfAbsent(type, rowMapper);
if (cachedMapper != null) {
rowMapper = cachedMapper;
}
}
return Optional.of(rowMapper);
}
return Optional.empty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy