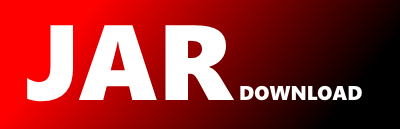
org.simpleflatmapper.map.context.KeyDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfm-map Show documentation
Show all versions of sfm-map Show documentation
Java library to map flat record - ResultSet, csv - to java object with minimum configuration and low footprint.
package org.simpleflatmapper.map.context;
import org.simpleflatmapper.map.context.impl.MultiValueKey;
import org.simpleflatmapper.map.context.impl.SingleValueKey;
import org.simpleflatmapper.util.ErrorHelper;
public class KeyDefinition {
public static final Key NOT_EQUALS = new Key() {
@Override
public int hashCode() {
return super.hashCode();
}
@Override
public boolean equals(Object obj) {
return false;
}
};
private final KeySourceGetter keySourceGetter;
private final KeyAndPredicate[] keyAndPredicates;
private final KeyAndPredicate singleKeyAndPredicate;
private final boolean empty;
private final int index;
public KeyDefinition(KeyAndPredicate[] keyAndPredicates, KeySourceGetter keySourceGetter, int index) {
this.singleKeyAndPredicate = getSingle(keyAndPredicates);
if (singleKeyAndPredicate == null) {
this.keyAndPredicates = keyAndPredicates;
} else {
this.keyAndPredicates = null;
}
this.keySourceGetter = keySourceGetter;
this.empty = keyAndPredicates == null || keyAndPredicates.length == 0;
this.index = index;
}
private static K getSingle(K[] array) {
//IFJAVA8_START
if (array != null && array.length == 1) return array[0];
//IFJAVA8_END
return null;
}
public boolean isEmpty() {
return empty;
}
public Key getValues(S source) {
if (empty) throw new IllegalStateException("cannot get value on empty keys");
try {
if (singleKeyAndPredicate != null) {
return singleValueKeys(source);
} else {
return multiValueKeys(source);
}
} catch (Exception e) {
return ErrorHelper.rethrow(e);
}
}
private Key singleValueKeys(S source) throws Exception {
Object value;
if (singleKeyAndPredicate.test(source)) {
value = keySourceGetter.getValue(singleKeyAndPredicate.key, source);
} else {
return NOT_EQUALS;
}
return new SingleValueKey(value);
}
private Key multiValueKeys(S source) throws Exception {
Object[] values = new Object[keyAndPredicates.length];
boolean empty = true;
for (int i = 0; i < values.length; i++) {
Object value = null;
KeyAndPredicate keyAndPredicate = keyAndPredicates[i];
if (keyAndPredicate.test(source)) {
empty = false;
value = keySourceGetter.getValue(keyAndPredicate.key, source);
}
values[i] = value;
}
if (empty) return NOT_EQUALS;
return new MultiValueKey(values);
}
public int getIndex() {
return index;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy