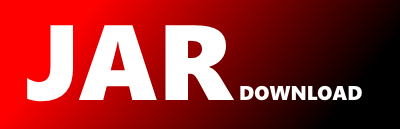
org.simpleflatmapper.map.impl.ExtendPropertyFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfm-map Show documentation
Show all versions of sfm-map Show documentation
Java library to map flat record - ResultSet, csv - to java object with minimum configuration and low footprint.
package org.simpleflatmapper.map.impl;
import org.simpleflatmapper.reflect.Getter;
import org.simpleflatmapper.reflect.InstantiatorDefinition;
import org.simpleflatmapper.reflect.ReflectionService;
import org.simpleflatmapper.reflect.Setter;
import org.simpleflatmapper.reflect.meta.PropertyFinder;
import org.simpleflatmapper.reflect.meta.PropertyMatchingScore;
import org.simpleflatmapper.reflect.meta.PropertyMeta;
import org.simpleflatmapper.reflect.meta.PropertyNameMatcher;
import org.simpleflatmapper.reflect.meta.SubPropertyMeta;
import org.simpleflatmapper.util.Function;
import org.simpleflatmapper.util.Predicate;
import org.simpleflatmapper.util.TypeHelper;
import java.lang.reflect.Type;
import java.util.List;
public class ExtendPropertyFinder extends PropertyFinder {
private static final Runnable EMPTY_CALLBACK = new Runnable() { @Override public void run() { } };
private static final Function IDENTITY =
new Function() {
@Override
public PropertyFinderTransformer apply(PropertyFinderTransformer propertyFinderTransformer) {
return propertyFinderTransformer;
}
};
private final PropertyFinder delegate;
private final List> customProperties;
private final Function transformerFunction;
public ExtendPropertyFinder(PropertyFinder delegate,
List> customProperties) {
this(delegate, customProperties, IDENTITY);
}
public ExtendPropertyFinder(PropertyFinder delegate,
List> customProperties,
Function transformerFunction) {
super(delegate.selfScoreFullName());
this.delegate = delegate;
this.customProperties = customProperties;
this.transformerFunction = transformerFunction;
}
@Override
public void lookForProperties(final PropertyNameMatcher propertyNameMatcher, Object[] properties, final FoundProperty matchingProperties, final PropertyMatchingScore score, final boolean allowSelfReference, final PropertyFinderTransformer propertyFinderTransformer, TypeAffinityScorer typeAffinityScorer, Predicate> propertyFilter) {
for (CustomProperty, ?> property : customProperties) {
if (property.isApplicable(delegate.getOwnerType()) && propertyNameMatcher.matches(property.getName())) {
matchingProperties.found((CustomProperty) property, EMPTY_CALLBACK, score.matches(propertyNameMatcher), typeAffinityScorer);
}
}
PropertyFinderTransformer newTransformer = transformerFunction.apply(propertyFinderTransformer);
delegate
.lookForProperties(
propertyNameMatcher,
properties, matchingProperties,
score.tupleIndex(1),
allowSelfReference,
newTransformer, typeAffinityScorer, propertyFilter);
}
@Override
public List getEligibleInstantiatorDefinitions() {
return delegate.getEligibleInstantiatorDefinitions();
}
@SuppressWarnings("unchecked")
@Override
public PropertyFinder> getSubPropertyFinder(PropertyMeta, ?> owner) {
return delegate.getSubPropertyFinder(owner);
}
@Override
public PropertyFinder> getOrCreateSubPropertyFinder(SubPropertyMeta, ?, ?> subPropertyMeta) {
return delegate.getOrCreateSubPropertyFinder(subPropertyMeta);
}
@Override
public Type getOwnerType() {
return delegate.getOwnerType();
}
public static class CustomProperty extends PropertyMeta {
private final Type type;
private final Setter super T, ? super P> setter;
private final Getter super T, ? extends P> getter;
public CustomProperty(
Type ownerType,
ReflectionService reflectService,
String name,
Type type,
Setter super T, ? super P> setter,
Getter super T, ? extends P> getter) {
super(name, ownerType, reflectService);
this.type = type;
this.setter = setter;
this.getter = getter;
}
@Override
public Setter super T, ? super P> getSetter() {
return setter;
}
@Override
public Getter super T, ? extends P> getGetter() {
return getter;
}
@Override
public Type getPropertyType() {
return type;
}
@Override
public String getPath() {
return getName();
}
public boolean isApplicable(Type ownerType) {
return TypeHelper.isAssignable(getOwnerType(), ownerType);
}
@Override
public boolean isConstructorProperty() {
return false;
}
@Override
public boolean isSubProperty() {
return false;
}
@Override
public boolean isSelf() {
return false;
}
@Override
public boolean isValid() {
return true;
}
@Override
public PropertyMeta withReflectionService(ReflectionService reflectionService) {
return new CustomProperty(getOwnerType(), reflectionService, getName(), type, setter, getter);
}
}
public static class ExtendPropertyFinderTransformer implements PropertyFinder.PropertyFinderTransformer {
private final PropertyFinder.PropertyFinderTransformer propertyFinderTransformer;
private final List> customProperties;
public ExtendPropertyFinderTransformer(PropertyFinder.PropertyFinderTransformer propertyFinderTransformer, List> customProperties) {
this.propertyFinderTransformer = propertyFinderTransformer;
this.customProperties = customProperties;
}
@Override
public PropertyFinder apply(PropertyFinder propertyFinder) {
if (propertyFinder instanceof ExtendPropertyFinder) {
throw new IllegalStateException();
}
return new ExtendPropertyFinder(propertyFinderTransformer.apply(propertyFinder), customProperties);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy