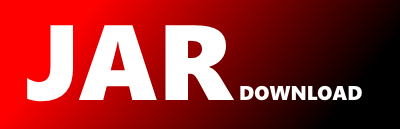
org.sfm.poi.SheetMapperFactory Maven / Gradle / Ivy
package org.sfm.poi;
import org.apache.poi.ss.usermodel.Row;
import org.sfm.csv.CsvColumnKey;
import org.sfm.map.mapper.AbstractMapperFactory;
import org.sfm.map.GetterFactory;
import org.sfm.map.column.FieldMapperColumnDefinition;
import org.sfm.map.mapper.FieldMapperColumnDefinitionProviderImpl;
import org.sfm.map.MapperConfig;
import org.sfm.poi.impl.DynamicSheetMapper;
import org.sfm.poi.impl.RowGetterFactory;
import org.sfm.reflect.TypeReference;
import org.sfm.reflect.meta.ClassMeta;
import java.lang.reflect.Type;
public class SheetMapperFactory extends AbstractMapperFactory, SheetMapperFactory> {
private GetterFactory getterFactory = new RowGetterFactory();
/**
*
* @return new instance of factory
*/
public static SheetMapperFactory newInstance() {
return new SheetMapperFactory();
}
private SheetMapperFactory() {
super(new FieldMapperColumnDefinitionProviderImpl(), FieldMapperColumnDefinition.identity());
}
/**
* set a new getterFactory.
* @param getterFactory the getterFactory
* @return the instance
*/
public SheetMapperFactory getterFactory(GetterFactory getterFactory) {
this.getterFactory = getterFactory;
return this;
}
/**
*
* @param type the type to map
* @param the type to map
* @return a builder on the specified type
*/
public SheetMapperBuilder newBuilder(Class type) {
return newBuilder((Type)type);
}
/**
*
* @param type the type to map
* @param the type to map
* @return a builder on the specified type
*/
public SheetMapperBuilder newBuilder(TypeReference type) {
return newBuilder(type.getType());
}
/**
*
* @param type the type to map
* @param the type to map
* @return a builder on the specified type
*/
public SheetMapperBuilder newBuilder(Type type) {
MapperConfig> mapperConfig = mapperConfig();
ClassMeta classMeta = getClassMeta(type);
return new SheetMapperBuilder(classMeta, mapperConfig, getterFactory);
}
/**
*
* @param type the type to map
* @param the type to map
* @return a dynamic mapper on the specified type
*/
public SheetMapper newMapper(Class type) {
return newMapper((Type)type);
}
/**
*
* @param type the type to map
* @param the type to map
* @return a dynamic mapper on the specified type
*/
public SheetMapper newMapper(TypeReference type) {
return newMapper(type.getType());
}
/**
*
* @param type the type to map
* @param the type to map
* @return a dynamic mapper on the specified type
*/
public SheetMapper newMapper(Type type) {
ClassMeta classMeta = getClassMeta(type);
MapperConfig> mapperConfig = mapperConfig();
return new DynamicSheetMapper(classMeta, mapperConfig, getterFactory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy