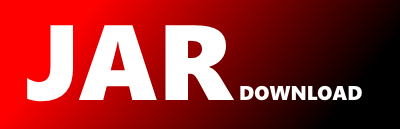
org.simplejavamail.converter.EmailConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-java-mail Show documentation
Show all versions of simple-java-mail Show documentation
Simple API, Complex Emails. A light weight wrapper for the JavaMail SMTP API
/*
* Copyright © 2009 Benny Bottema ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.simplejavamail.converter;
import jakarta.mail.MessagingException;
import jakarta.mail.Session;
import jakarta.mail.internet.InternetAddress;
import jakarta.mail.internet.MimeMessage;
import lombok.val;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.simplejavamail.api.email.CalendarMethod;
import org.simplejavamail.api.email.ContentTransferEncoding;
import org.simplejavamail.api.email.Email;
import org.simplejavamail.api.email.EmailPopulatingBuilder;
import org.simplejavamail.api.email.OriginalSmimeDetails;
import org.simplejavamail.api.email.OriginalSmimeDetails.SmimeMode;
import org.simplejavamail.api.internal.general.HeadersToIgnoreWhenParsingExternalEmails;
import org.simplejavamail.api.internal.outlooksupport.model.EmailFromOutlookMessage;
import org.simplejavamail.api.internal.outlooksupport.model.OutlookMessage;
import org.simplejavamail.api.internal.smimesupport.builder.SmimeParseResult;
import org.simplejavamail.api.mailer.config.EmailGovernance;
import org.simplejavamail.api.mailer.config.Pkcs12Config;
import org.simplejavamail.converter.internal.InternalEmailConverterImpl;
import org.simplejavamail.converter.internal.mimemessage.MimeDataSource;
import org.simplejavamail.converter.internal.mimemessage.MimeMessageParser;
import org.simplejavamail.converter.internal.mimemessage.MimeMessageParser.ParsedMimeMessageComponents;
import org.simplejavamail.converter.internal.mimemessage.MimeMessageProducerHelper;
import org.simplejavamail.email.EmailBuilder;
import org.simplejavamail.email.internal.EmailPopulatingBuilderFactoryImpl;
import org.simplejavamail.email.internal.EmailStartingBuilderImpl;
import org.simplejavamail.email.internal.InternalEmailPopulatingBuilder;
import org.simplejavamail.internal.moduleloader.ModuleLoader;
import org.simplejavamail.internal.smimesupport.model.OriginalSmimeDetailsImpl;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.util.Map;
import java.util.Properties;
import static java.lang.String.format;
import static java.nio.charset.StandardCharsets.UTF_8;
import static org.simplejavamail.api.email.OriginalSmimeDetails.SmimeMode.PLAIN;
import static org.simplejavamail.internal.moduleloader.ModuleLoader.loadSmimeModule;
import static org.simplejavamail.internal.util.MiscUtil.extractCID;
import static org.simplejavamail.internal.util.MiscUtil.readInputStreamToString;
import static org.simplejavamail.internal.util.MiscUtil.valueNullOrEmpty;
import static org.simplejavamail.internal.util.Preconditions.checkNonEmptyArgument;
import static org.simplejavamail.internal.util.Preconditions.verifyNonnullOrEmpty;
import static org.simplejavamail.mailer.internal.EmailGovernanceImpl.NO_GOVERNANCE;
/**
* Utility to help convert {@link org.simplejavamail.api.email.Email} instances to other formats (MimeMessage, EML etc.) and vice versa.
*
* If you use the Outlook parsing API, make sure you load the following dependency: org.simplejavamail::outlook-message-parser
*/
@SuppressWarnings("WeakerAccess")
public final class EmailConverter {
private EmailConverter() {
// util / helper class
}
/*
To Email instance
*/
/**
* Delegates to {@link #mimeMessageToEmail(MimeMessage, Pkcs12Config)}.
*/
@NotNull
public static Email mimeMessageToEmail(@NotNull final MimeMessage mimeMessage) {
return mimeMessageToEmail(mimeMessage, null);
}
/**
* Delegates to {@link #mimeMessageToEmailBuilder(MimeMessage, Pkcs12Config)}.
*/
@NotNull
public static Email mimeMessageToEmail(@NotNull final MimeMessage mimeMessage, @Nullable final Pkcs12Config pkcs12Config) {
return mimeMessageToEmailBuilder(mimeMessage, pkcs12Config).buildEmail();
}
/**
* Delegates to {@link #mimeMessageToEmailBuilder(MimeMessage, Pkcs12Config, boolean)}.
*/
@NotNull
public static Email mimeMessageToEmail(@NotNull final MimeMessage mimeMessage, @Nullable final Pkcs12Config pkcs12Config, final boolean fetchAttachmentData) {
return mimeMessageToEmailBuilder(mimeMessage, pkcs12Config, fetchAttachmentData).buildEmail();
}
/**
* Delegates to {@link #mimeMessageToEmailBuilder(MimeMessage, Pkcs12Config)}.
*/
@NotNull
public static EmailPopulatingBuilder mimeMessageToEmailBuilder(@NotNull final MimeMessage mimeMessage) {
return mimeMessageToEmailBuilder(mimeMessage, null);
}
/**
* Delegates to {@link #mimeMessageToEmailBuilder(MimeMessage, Pkcs12Config, boolean)}.
*/
@NotNull
public static EmailPopulatingBuilder mimeMessageToEmailBuilder(@NotNull final MimeMessage mimeMessage, @Nullable final Pkcs12Config pkcs12Config) {
return mimeMessageToEmailBuilder(mimeMessage, pkcs12Config, true);
}
/**
* @param mimeMessage The MimeMessage from which to create the {@link Email}.
* @param pkcs12Config Private key store for decrypting S/MIME encrypted attachments
* (only needed when the message is encrypted rather than just signed).
* @param fetchAttachmentData When false only the names of the attachments are retrieved but no data
*/
@NotNull
public static EmailPopulatingBuilder mimeMessageToEmailBuilder(@NotNull final MimeMessage mimeMessage, @Nullable final Pkcs12Config pkcs12Config, final boolean fetchAttachmentData) {
checkNonEmptyArgument(mimeMessage, "mimeMessage");
val builder = EmailBuilder.startingBlank();
val parsed = MimeMessageParser.parseMimeMessage(mimeMessage, fetchAttachmentData);
val emailBuilder = buildEmailFromMimeMessage(builder, parsed);
return decryptAttachments(emailBuilder, mimeMessage, pkcs12Config);
}
/**
* Delegates to {@link #outlookMsgToEmail(String, Pkcs12Config)}.
*
* @param msgFileName The file name of an Outlook (.msg) message from which to create the {@link Email}.
*/
@SuppressWarnings("unused")
@NotNull
public static Email outlookMsgToEmail(@NotNull final String msgFileName) {
return outlookMsgToEmail(msgFileName, null);
}
/**
* @param msgFileName The file name of an Outlook (.msg) message from which to create the {@link Email}.
* @param pkcs12Config Private key store for decrypting S/MIME encrypted attachments
* (only needed when the message is encrypted rather than just signed).
*/
@SuppressWarnings("deprecation")
@NotNull
public static Email outlookMsgToEmail(@NotNull final String msgFileName, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(msgFileName, "msgFile");
EmailFromOutlookMessage result = ModuleLoader.loadOutlookModule()
.outlookMsgToEmailBuilder(msgFileName, new EmailStartingBuilderImpl(), new EmailPopulatingBuilderFactoryImpl(), InternalEmailConverterImpl.INSTANCE);
return decryptAttachments(result.getEmailBuilder(), result.getOutlookMessage(), pkcs12Config)
.buildEmail();
}
/**
* Delegates to {@link #outlookMsgToEmailBuilder(File)} and then builds and returns the email.
*
* @param msgFile The content of an Outlook (.msg) message from which to create the {@link Email}.
*/
@NotNull
public static Email outlookMsgToEmail(@NotNull final File msgFile) {
return outlookMsgToEmailBuilder(msgFile).buildEmail();
}
/**
* Delegates to {@link #outlookMsgToEmailBuilder(File, Pkcs12Config)} and then builds and returns the email.
*
* @param msgFile The content of an Outlook (.msg) message from which to create the {@link Email}.
*/
@SuppressWarnings("unused")
@NotNull
public static Email outlookMsgToEmail(@NotNull final File msgFile, @Nullable final Pkcs12Config pkcs12Config) {
return outlookMsgToEmailBuilder(msgFile, pkcs12Config).buildEmail();
}
/**
* Delegates to {@link #outlookMsgToEmailBuilder(File, Pkcs12Config)}.
*
* @param msgFile The content of an Outlook (.msg) message from which to create the {@link Email}.
*/
@NotNull
public static EmailPopulatingBuilder outlookMsgToEmailBuilder(@NotNull final File msgFile) {
return outlookMsgToEmailBuilder(msgFile, null);
}
/**
* @param msgFile The content of an Outlook (.msg) message from which to create the {@link Email}.
* @param pkcs12Config Private key store for decrypting S/MIME encrypted attachments
* (only needed when the message is encrypted rather than just signed).
*/
@SuppressWarnings({ "deprecation" })
@NotNull
public static EmailPopulatingBuilder outlookMsgToEmailBuilder(@NotNull final File msgFile, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(msgFile, "msgFile");
EmailFromOutlookMessage result = ModuleLoader.loadOutlookModule()
.outlookMsgToEmailBuilder(msgFile, new EmailStartingBuilderImpl(), new EmailPopulatingBuilderFactoryImpl(), InternalEmailConverterImpl.INSTANCE);
return decryptAttachments(result.getEmailBuilder(), result.getOutlookMessage(), pkcs12Config);
}
/**
* Delegates to {@link #outlookMsgToEmail(InputStream, Pkcs12Config)}.
*/
@SuppressWarnings("unused")
@NotNull
public static Email outlookMsgToEmail(@NotNull final InputStream msgInputStream) {
return outlookMsgToEmail(msgInputStream, null);
}
/**
* Delegates to {@link #outlookMsgToEmailBuilder(InputStream, Pkcs12Config)}.
*/
@NotNull
public static Email outlookMsgToEmail(@NotNull final InputStream msgInputStream, @Nullable final Pkcs12Config pkcs12Config) {
return outlookMsgToEmailBuilder(msgInputStream, pkcs12Config).getEmailBuilder().buildEmail();
}
/**
* Delegates to {@link #outlookMsgToEmailBuilder(InputStream, Pkcs12Config)}.
*/
@NotNull
public static EmailFromOutlookMessage outlookMsgToEmailBuilder(@NotNull final InputStream msgInputStream) {
return outlookMsgToEmailBuilder(msgInputStream, null);
}
/**
* Note: the email builder wrapper by {@link EmailFromOutlookMessage} is set to ignore defaults as to stay as close as possible to the original MimeMessage.
* If you wish to use the result to send an email, you might want to first call {@link EmailPopulatingBuilder#ignoringDefaults(boolean)} to set the builder
* to use defaults again.
*
* @param msgInputStream The content of an Outlook (.msg) message from which to create the {@link Email}.
*/
@SuppressWarnings("deprecation")
@NotNull
public static EmailFromOutlookMessage outlookMsgToEmailBuilder(@NotNull final InputStream msgInputStream, @Nullable final Pkcs12Config pkcs12Config) {
EmailFromOutlookMessage fromMsgBuilder = ModuleLoader.loadOutlookModule()
.outlookMsgToEmailBuilder(msgInputStream, new EmailStartingBuilderImpl(), new EmailPopulatingBuilderFactoryImpl(), InternalEmailConverterImpl.INSTANCE);
decryptAttachments(fromMsgBuilder.getEmailBuilder(), fromMsgBuilder.getOutlookMessage(), pkcs12Config);
return fromMsgBuilder;
}
private static EmailPopulatingBuilder decryptAttachments(final EmailPopulatingBuilder emailBuilder, final OutlookMessage outlookMessage, @Nullable final Pkcs12Config pkcs12Config) {
if (ModuleLoader.smimeModuleAvailable()) {
SmimeParseResult smimeParseResult = loadSmimeModule().decryptAttachments(emailBuilder.getAttachments(), outlookMessage, pkcs12Config);
handleSmimeParseResult((InternalEmailPopulatingBuilder) emailBuilder, smimeParseResult);
updateEmailIfBothSignedAndEncrypted(emailBuilder, smimeParseResult);
}
return emailBuilder;
}
@NotNull
private static EmailPopulatingBuilder decryptAttachments(final EmailPopulatingBuilder emailBuilder, final MimeMessage mimeMessage, @Nullable final Pkcs12Config pkcs12Config) {
if (ModuleLoader.smimeModuleAvailable()) {
SmimeParseResult smimeParseResult = loadSmimeModule().decryptAttachments(emailBuilder.getAttachments(), mimeMessage, pkcs12Config);
handleSmimeParseResult((InternalEmailPopulatingBuilder) emailBuilder, smimeParseResult);
updateEmailIfBothSignedAndEncrypted(emailBuilder, smimeParseResult);
}
return emailBuilder;
}
/**
* if we have both an encrypted and signed part in the email, have the
* top-level email reflect this as {@link SmimeMode#SIGNED_ENCRYPTED}.
*/
private static void updateEmailIfBothSignedAndEncrypted(final EmailPopulatingBuilder emailBuilder, final SmimeParseResult smimeParseResult) {
if (emailBuilder.getSmimeSignedEmail() != null) {
OriginalSmimeDetails nestedSmime = emailBuilder.getSmimeSignedEmail().getOriginalSmimeDetails();
OriginalSmimeDetailsImpl originalSmimeDetails = (OriginalSmimeDetailsImpl) emailBuilder.getOriginalSmimeDetails();
if (nestedSmime.getSmimeMode() != PLAIN && nestedSmime.getSmimeMode() != originalSmimeDetails.getSmimeMode()) {
originalSmimeDetails.completeWithSmimeMode(SmimeMode.SIGNED_ENCRYPTED);
} else if (smimeParseResult.getDecryptedAttachmentResults().size() == 1) {
final SmimeMode smimeMode = smimeParseResult.getDecryptedAttachmentResults().get(0).getSmimeMode();
originalSmimeDetails.completeWithSmimeMode(smimeMode);
}
}
}
private static void handleSmimeParseResult(final InternalEmailPopulatingBuilder emailBuilder, final SmimeParseResult smimeParseResult) {
emailBuilder.withDecryptedAttachments(smimeParseResult.getDecryptedAttachments());
emailBuilder.withOriginalSmimeDetails(smimeParseResult.getOriginalSmimeDetails());
if (smimeParseResult.getSmimeSignedOrEncryptedEmail() != null) {
emailBuilder.withSmimeSignedEmail(emlToEmail(smimeParseResult.getSmimeSignedOrEncryptedEmail().getDataSourceInputStream()));
}
}
/**
* Delegates to {@link #emlToEmail(InputStream, Pkcs12Config)}.
*/
@NotNull
public static Email emlToEmail(@NotNull final InputStream emlInputStream) {
return emlToEmail(emlInputStream, null);
}
/**
* Delegates to {@link #emlToEmail(InputStream, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static Email emlToEmail(@NotNull final InputStream emlInputStream, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmail(emlInputStream, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(InputStream, Pkcs12Config)} with the full string value read from the given InputStream
.
*/
@NotNull
public static Email emlToEmail(@NotNull final InputStream emlInputStream, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
return emlToEmailBuilder(emlInputStream, pkcs12Config, session).buildEmail();
}
/**
* Delegates to {@link #emlToEmail(String, Pkcs12Config)}.
*/
@NotNull
public static Email emlToEmail(@NotNull final String eml) {
return emlToEmail(eml, null);
}
/**
* Delegates to {@link #emlToEmail(String, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static Email emlToEmail(@NotNull final String eml, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmail(eml, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(String, Pkcs12Config, Session)}.
*/
@NotNull
public static Email emlToEmail(@NotNull final String eml, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
return emlToEmailBuilder(eml, pkcs12Config, session).buildEmail();
}
/**
* Delegates to {@link #emlToEmail(File, Pkcs12Config)}.
*/
@NotNull
public static Email emlToEmail(@NotNull final File emlFile) {
return emlToEmail(emlFile, null);
}
/**
* Delegates to {@link #emlToEmail(File, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static Email emlToEmail(@NotNull final File emlFile, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmail(emlFile, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(File, Pkcs12Config, Session)}.
*/
@NotNull
public static Email emlToEmail(@NotNull final File emlFile, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
return emlToEmailBuilder(emlFile, pkcs12Config, session).buildEmail();
}
/**
* Delegates to {@link #emlToEmailBuilder(File, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final File emlFile) {
return emlToEmailBuilder(emlFile, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(File, Pkcs12Config, Session)}.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final File emlFile, @NotNull final Session session) {
return emlToEmailBuilder(emlFile, null, session);
}
/**
* Delegates to {@link #emlToEmailBuilder(File, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final File emlFile, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmailBuilder(emlFile, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(InputStream, Pkcs12Config, Session)}.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final File emlFile, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
try {
return emlToEmailBuilder(new FileInputStream(checkNonEmptyArgument(emlFile, "emlFile")), pkcs12Config, session);
} catch (final FileNotFoundException e) {
throw new EmailConverterException(format(EmailConverterException.PARSE_ERROR_EML_FROM_FILE, e.getMessage()), e);
}
}
/**
* Delegates to {@link #emlToEmailBuilder(InputStream, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final InputStream emlInputStream) {
return emlToEmailBuilder(emlInputStream, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(InputStream, Pkcs12Config, Session)}.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final InputStream emlInputStream, @NotNull final Session session) {
return emlToEmailBuilder(emlInputStream, null, session);
}
/**
* Delegates to {@link #emlToEmailBuilder(InputStream, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final InputStream emlInputStream, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmailBuilder(emlInputStream, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(String, Pkcs12Config, Session)} with the full string value read from the given InputStream
.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final InputStream emlInputStream, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
try {
String emlStr = readInputStreamToString(checkNonEmptyArgument(emlInputStream, "emlInputStream"), UTF_8);
return emlToEmailBuilder(emlStr, pkcs12Config, session);
} catch (IOException e) {
throw new EmailConverterException(EmailConverterException.ERROR_READING_EML_INPUTSTREAM, e);
}
}
/**
* Delegates to {@link #emlToEmailBuilder(String, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final String eml) {
return emlToEmailBuilder(eml, createDummySession());
}
/**
* Delegates to {@link #emlToEmailBuilder(String, Pkcs12Config, Session)}.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final String eml, @NotNull final Session session) {
return emlToEmailBuilder(eml, null, session);
}
/**
* Delegates to {@link #emlToEmailBuilder(String, Pkcs12Config, Session)} using a dummy {@link Session} instance.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final String eml, @Nullable final Pkcs12Config pkcs12Config) {
return emlToEmailBuilder(eml, pkcs12Config, createDummySession());
}
/**
* Delegates to {@link #emlToMimeMessage(String, Session)} using a dummy {@link Session} instance and passes the result to {@link
* #mimeMessageToEmailBuilder(MimeMessage, Pkcs12Config)}.
*/
@NotNull
public static EmailPopulatingBuilder emlToEmailBuilder(@NotNull final String eml, @Nullable final Pkcs12Config pkcs12Config, @NotNull final Session session) {
final MimeMessage mimeMessage = emlToMimeMessage(checkNonEmptyArgument(eml, "eml"), session);
return mimeMessageToEmailBuilder(mimeMessage, pkcs12Config);
}
/*
To MimeMessage instance
*/
/**
* Delegates to {@link #outlookMsgToMimeMessage(String, Pkcs12Config)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final String msgFile) {
return outlookMsgToMimeMessage(msgFile, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(String, Pkcs12Config)} and {@link #emailToMimeMessage(Email)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final String msgData, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(msgData, "outlookMsgData");
return emailToMimeMessage(outlookMsgToEmail(msgData, pkcs12Config));
}
/**
* Delegates to {@link #outlookMsgToMimeMessage(File, Pkcs12Config)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final File outlookMsgFile) {
return outlookMsgToMimeMessage(outlookMsgFile, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(File, Pkcs12Config)} and {@link #emailToMimeMessage(Email)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final File outlookMsgFile, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(outlookMsgFile, "outlookMsgFile");
return emailToMimeMessage(outlookMsgToEmail(outlookMsgFile, pkcs12Config));
}
/**
* Delegates to {@link #outlookMsgToMimeMessage(InputStream, Pkcs12Config)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final InputStream outlookMsgInputStream) {
return outlookMsgToMimeMessage(outlookMsgInputStream, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(InputStream, Pkcs12Config)} and {@link #emailToMimeMessage(Email)}.
*/
@NotNull
public static MimeMessage outlookMsgToMimeMessage(@NotNull final InputStream outlookMsgInputStream, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(outlookMsgInputStream, "outlookMsgInputStream");
return emailToMimeMessage(outlookMsgToEmail(outlookMsgInputStream, pkcs12Config));
}
/**
* Delegates to {@link #emailToMimeMessage(Email, Session, EmailGovernance)}, using a new empty {@link Session} instance,
* and without email governance - but defaults from (system) prorties (files) are still applied, if provided..
*/
public static MimeMessage emailToMimeMessage(@NotNull final Email email) {
return emailToMimeMessage(checkNonEmptyArgument(email, "email"), createDummySession(), NO_GOVERNANCE());
}
/**
* Delegates to {@link #emailToMimeMessage(Email, Session, EmailGovernance)}, using a new empty {@link Session} instance.
*/
public static MimeMessage emailToMimeMessage(@NotNull final Email email, final EmailGovernance emailGovernance) {
return emailToMimeMessage(checkNonEmptyArgument(email, "email"), createDummySession(), emailGovernance);
}
/**
* Delegates to {@link #emailToMimeMessage(Email, Session, EmailGovernance)} with no email governance -
* but defaults from (system) prorties (files) are still applied, if provided.
*/
public static MimeMessage emailToMimeMessage(@NotNull final Email email, @NotNull final Session session) {
return emailToMimeMessage(email, session, NO_GOVERNANCE());
}
/**
* Delegates to {@link MimeMessageProducerHelper#produceMimeMessage(Email, Session)}.
*/
public static MimeMessage emailToMimeMessage(@NotNull final Email email, @NotNull final Session session, @NotNull final EmailGovernance emailGovernance) {
try {
return MimeMessageProducerHelper.produceMimeMessage(
emailGovernance.produceEmailApplyingDefaultsAndOverrides(email),
checkNonEmptyArgument(session, "session"));
} catch (UnsupportedEncodingException | MessagingException e) {
// this should never happen, so we don't acknowledge this exception (and simply bubble up)
throw new IllegalStateException(e.getMessage(), e);
}
}
/**
* Delegates to {@link #emlToMimeMessage(File, Session)}, using {@link #createDummySession()}.
*/
@NotNull
public static MimeMessage emlToMimeMessage(@NotNull final File emlFile) {
return emlToMimeMessage(emlFile, createDummySession());
}
/**
* Delegates to {@link #emlToMimeMessage(InputStream, Session)}.
*/
public static MimeMessage emlToMimeMessage(@NotNull final File emlFile, @NotNull final Session session) {
try {
return emlToMimeMessage(new FileInputStream(checkNonEmptyArgument(emlFile, "emlFile")), session);
} catch (final FileNotFoundException e) {
throw new EmailConverterException(format(EmailConverterException.PARSE_ERROR_EML_FROM_FILE, e.getMessage()), e);
}
}
/**
* Delegates to {@link #emlToMimeMessage(InputStream, Session)} using {@link #createDummySession()}.
*/
@NotNull
public static MimeMessage emlToMimeMessage(@NotNull final InputStream inputStream) {
return emlToMimeMessage(inputStream, createDummySession());
}
/**
* Relies on JavaMail's native parser of EML data, {@link MimeMessage#MimeMessage(Session, InputStream)}.
*
* @see MimeMessage#MimeMessage(Session, InputStream)
*/
@NotNull
public static MimeMessage emlToMimeMessage(@NotNull final InputStream inputStream, @NotNull final Session session) {
try {
return new MimeMessage(session, inputStream);
} catch (final MessagingException e) {
throw new EmailConverterException(format(EmailConverterException.PARSE_ERROR_EML_FROM_STREAM, e.getMessage()), e);
}
}
/**
* Delegates to {@link #emlToMimeMessage(String, Session)} with an empty {@link Session} instance.
*/
public static MimeMessage emlToMimeMessage(@NotNull final String eml) {
return emlToMimeMessage(checkNonEmptyArgument(eml, "eml"), createDummySession());
}
/**
* Relies on JavaMail's native parser of EML data, {@link MimeMessage#MimeMessage(Session, InputStream)}.
*/
public static MimeMessage emlToMimeMessage(@NotNull final String eml, @NotNull final Session session) {
checkNonEmptyArgument(session, "session");
checkNonEmptyArgument(eml, "eml");
try {
return new MimeMessage(session, new ByteArrayInputStream(eml.getBytes(UTF_8)));
} catch (final MessagingException e) {
throw new EmailConverterException(format(EmailConverterException.PARSE_ERROR_EML_FROM_STREAM, e.getMessage()), e);
}
}
/*
To EML String
*/
/**
* @return The result of {@link MimeMessage#writeTo(OutputStream)} which should be in the standard EML format.
*/
public static byte[] mimeMessageToEMLByteArray(@NotNull final MimeMessage mimeMessage) {
final ByteArrayOutputStream os = new ByteArrayOutputStream();
try {
checkNonEmptyArgument(mimeMessage, "mimeMessage").writeTo(os);
return os.toByteArray();
} catch (IOException | MessagingException e) {
// this should never happen, so we don't acknowledge this exception (and simply bubble up)
throw new IllegalStateException("This should never happen", e);
}
}
/**
* @return The result of {@link MimeMessage#writeTo(OutputStream)} with which should be in the standard EML format, to UTF8 string.
*/
public static String mimeMessageToEML(@NotNull final MimeMessage mimeMessage) {
final ByteArrayOutputStream os = new ByteArrayOutputStream();
try {
checkNonEmptyArgument(mimeMessage, "mimeMessage").writeTo(os);
return os.toString(UTF_8.name());
} catch (IOException | MessagingException e) {
// this should never happen, so we don't acknowledge this exception (and simply bubble up)
throw new IllegalStateException("This should never happen", e);
}
}
/**
* Delegates to {@link #emailToMimeMessage(Email)} and passes the result to {@link #mimeMessageToEML(MimeMessage)}.
*
* @see #emailToMimeMessage(Email, Session)
*/
public static String emailToEML(@NotNull final Email email) {
return mimeMessageToEML(emailToMimeMessage(checkNonEmptyArgument(email, "email")));
}
/**
* Delegates to {@link #outlookMsgToEML(String, Pkcs12Config)}.
*/
@NotNull
public static String outlookMsgToEML(@NotNull final String msgFile) {
return outlookMsgToEML(msgFile, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(String, Pkcs12Config)} and {@link #emailToEML(Email)}
*/
@NotNull
public static String outlookMsgToEML(@NotNull final String msgData, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(msgData, "outlookMsgData");
return emailToEML(outlookMsgToEmail(msgData, pkcs12Config));
}
/**
* Delegates to {@link #outlookMsgToEML(File, Pkcs12Config)}.
*/
@NotNull
public static String outlookMsgToEML(@NotNull final File outlookMsgFile) {
return outlookMsgToEML(outlookMsgFile, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(File, Pkcs12Config)} and {@link #emailToEML(Email)}
*/
@NotNull
public static String outlookMsgToEML(@NotNull final File outlookMsgFile, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(outlookMsgFile, "outlookMsgFile");
return emailToEML(outlookMsgToEmail(outlookMsgFile, pkcs12Config));
}
/**
* Delegates to {@link #outlookMsgToEML(InputStream, Pkcs12Config)}.
*/
@NotNull
public static String outlookMsgToEML(@NotNull final InputStream outlookMsgInputStream) {
return outlookMsgToEML(outlookMsgInputStream, null);
}
/**
* @return Result of {@link #outlookMsgToEmail(InputStream, Pkcs12Config)} and {@link #emailToEML(Email)}
*/
@NotNull
public static String outlookMsgToEML(@NotNull final InputStream outlookMsgInputStream, @Nullable final Pkcs12Config pkcs12Config) {
checkNonEmptyArgument(outlookMsgInputStream, "outlookMsgInputStream");
return emailToEML(outlookMsgToEmail(outlookMsgInputStream, pkcs12Config));
}
/*
Helpers
*/
private static EmailPopulatingBuilder buildEmailFromMimeMessage(@NotNull final EmailPopulatingBuilder builder, @NotNull final ParsedMimeMessageComponents parsed) {
checkNonEmptyArgument(builder, "emailBuilder");
checkNonEmptyArgument(parsed, "parsedMimeMessageComponents");
if (parsed.getSentDate() != null) {
builder.fixingSentDate(parsed.getSentDate());
}
if (parsed.getFromAddress() != null) {
builder.from(parsed.getFromAddress().getPersonal(), parsed.getFromAddress().getAddress());
}
if (parsed.getReplyToAddresses() != null) {
builder.withReplyTo(parsed.getReplyToAddresses().getPersonal(), parsed.getReplyToAddresses().getAddress());
}
for (val headerEntry : parsed.getHeaders().entrySet()) {
if (!HeadersToIgnoreWhenParsingExternalEmails.shouldIgnoreHeader(headerEntry.getKey())) {
for (Object headerValue : headerEntry.getValue()) {
builder.withHeader(headerEntry.getKey(), headerValue);
}
}
}
if (parsed.getDispositionNotificationTo() != null) {
builder.withDispositionNotificationTo(parsed.getDispositionNotificationTo());
}
if (parsed.getReturnReceiptTo() != null) {
builder.withReturnReceiptTo(parsed.getReturnReceiptTo());
}
if (parsed.getBounceToAddress() != null) {
builder.withBounceTo(parsed.getBounceToAddress());
}
if (parsed.getContentTransferEncoding() != null) {
builder.withContentTransferEncoding(ContentTransferEncoding.byEncoder(parsed.getContentTransferEncoding()));
}
builder.fixingMessageId(parsed.getMessageId());
for (final InternetAddress to : parsed.getToAddresses()) {
builder.to(to);
}
//noinspection QuestionableName
for (final InternetAddress cc : parsed.getCcAddresses()) {
builder.cc(cc);
}
for (final InternetAddress bcc : parsed.getBccAddresses()) {
builder.bcc(bcc);
}
builder.withSubject(parsed.getSubject() != null ? parsed.getSubject() : "");
builder.withPlainText(parsed.getPlainContent());
builder.withHTMLText(parsed.getHtmlContent());
if (parsed.getCalendarMethod() != null) {
builder.withCalendarText(CalendarMethod.valueOf(parsed.getCalendarMethod()), verifyNonnullOrEmpty(parsed.getCalendarContent()));
}
for (final Map.Entry cid : parsed.getCidMap().entrySet()) {
final String cidName = checkNonEmptyArgument(cid.getKey(), "cid.key");
builder.withEmbeddedImage(extractCID(cidName), cid.getValue().getDataSource());
}
for (final MimeDataSource attachment : parsed.getAttachmentList()) {
final ContentTransferEncoding encoding = !valueNullOrEmpty(attachment.getContentTransferEncoding())
? ContentTransferEncoding.byEncoder(attachment.getContentTransferEncoding()) : null;
builder.withAttachment(extractCID(attachment.getName()), attachment.getDataSource(), attachment.getContentDescription(), encoding);
}
return builder;
}
private static Session createDummySession() {
return Session.getInstance(new Properties());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy