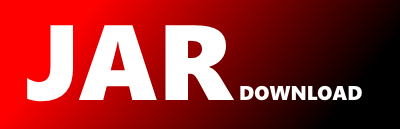
org.simplejavamail.internal.moduleloader.ModuleLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-java-mail Show documentation
Show all versions of simple-java-mail Show documentation
Simple API, Complex Emails. A light weight wrapper for the JavaMail SMTP API
/*
* Copyright © 2009 Benny Bottema ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.simplejavamail.internal.moduleloader;
import org.simplejavamail.internal.modules.AuthenticatedSocksModule;
import org.simplejavamail.internal.modules.BatchModule;
import org.simplejavamail.internal.modules.DKIMModule;
import org.simplejavamail.internal.modules.OutlookModule;
import org.simplejavamail.internal.modules.SMIMEModule;
import org.simplejavamail.internal.util.MiscUtil;
import java.util.HashSet;
import java.util.Set;
import java.util.HashMap;
import java.util.Map;
import static java.lang.String.format;
public class ModuleLoader {
private static final boolean BATCH_SUPPORT_CLASS_AVAILABLE = MiscUtil.classAvailable("org.simplejavamail.internal.batchsupport.BatchSupport");
private static final boolean SMIME_SUPPORT_CLASS_AVAILABLE = MiscUtil.classAvailable("org.simplejavamail.internal.smimesupport.SMIMESupport");
private static final boolean DKIM_SUPPORT_CLASS_AVAILABLE = MiscUtil.classAvailable("org.simplejavamail.internal.dkimsupport.DKIMSigner");
private static final Map LOADED_MODULES = new HashMap<>();
// used from junit tests
private static final Set FORCED_DISABLED_MODULES = new HashSet<>();
private static final Set FORCED_RECHECK_MODULES = new HashSet<>();
public static AuthenticatedSocksModule loadAuthenticatedSocksModule() {
if (!LOADED_MODULES.containsKey(AuthenticatedSocksModule.class)) {
LOADED_MODULES.put(AuthenticatedSocksModule.class, loadModule(
AuthenticatedSocksModule.class,
"Authenticated Socks",
"org.simplejavamail.internal.authenticatedsockssupport.AuthenticatedSocksHelper",
"https://github.com/bbottema/simple-java-mail/tree/develop/modules/authenticated-socks-module"));
}
return (AuthenticatedSocksModule) LOADED_MODULES.get(AuthenticatedSocksModule.class);
}
public static DKIMModule loadDKIMModule() {
if (!LOADED_MODULES.containsKey(DKIMModule.class)) {
LOADED_MODULES.put(DKIMModule.class, loadModule(
DKIMModule.class,
"DKIM",
"org.simplejavamail.internal.dkimsupport.DKIMSigner",
"https://github.com/bbottema/simple-java-mail/tree/develop/modules/dkim-module"));
}
return (DKIMModule) LOADED_MODULES.get(DKIMModule.class);
}
public static OutlookModule loadOutlookModule() {
if (!LOADED_MODULES.containsKey(OutlookModule.class)) {
LOADED_MODULES.put(OutlookModule.class, loadModule(
OutlookModule.class,
"Outlook",
"org.simplejavamail.internal.outlooksupport.converter.OutlookEmailConverter",
"https://github.com/bbottema/simple-java-mail/tree/develop/modules/outlook-module"
));
}
return (OutlookModule) LOADED_MODULES.get(OutlookModule.class);
}
public static SMIMEModule loadSmimeModule() {
if (!LOADED_MODULES.containsKey(SMIMEModule.class)) {
LOADED_MODULES.put(SMIMEModule.class, loadModule(
SMIMEModule.class,
"S/MIME",
"org.simplejavamail.internal.smimesupport.SMIMESupport",
"https://github.com/bbottema/simple-java-mail/tree/develop/modules/smime-module"
));
}
return (SMIMEModule) LOADED_MODULES.get(SMIMEModule.class);
}
public static BatchModule loadBatchModule() {
if (FORCED_DISABLED_MODULES.contains(BatchModule.class)) {
throw new IllegalStateException("BatchModule forcefully disabled");
}
if (!LOADED_MODULES.containsKey(BatchModule.class)) {
LOADED_MODULES.put(BatchModule.class, loadModule(
BatchModule.class,
"Batch",
"org.simplejavamail.internal.batchsupport.BatchSupport",
"https://github.com/bbottema/simple-java-mail/tree/develop/modules/batch-module"
));
}
return (BatchModule) LOADED_MODULES.get(BatchModule.class);
}
public static boolean batchModuleAvailable() {
return !FORCED_DISABLED_MODULES.contains(BatchModule.class) &&
((FORCED_RECHECK_MODULES.contains(BatchModule.class) &&
MiscUtil.classAvailable("org.simplejavamail.internal.batchsupport.BatchSupport")) ||
BATCH_SUPPORT_CLASS_AVAILABLE);
}
public static boolean smimeModuleAvailable() {
return !FORCED_DISABLED_MODULES.contains(SMIMEModule.class) &&
((FORCED_RECHECK_MODULES.contains(SMIMEModule.class) &&
MiscUtil.classAvailable("org.simplejavamail.internal.smimesupport.SMIMESupport")) ||
SMIME_SUPPORT_CLASS_AVAILABLE);
}
public static boolean dkimModuleAvailable() {
return !FORCED_DISABLED_MODULES.contains(DKIMModule.class) &&
((FORCED_RECHECK_MODULES.contains(DKIMModule.class) &&
MiscUtil.classAvailable("org.simplejavamail.internal.dkimsupport.DKIMSigner")) ||
DKIM_SUPPORT_CLASS_AVAILABLE);
}
@SuppressWarnings("unchecked")
private static T loadModule(Class moduleClass,String moduleName, String moduleImplClassName, String moduleHome) {
try {
if (FORCED_DISABLED_MODULES.contains(moduleClass)) {
throw new IllegalAccessException("Module is forcefully disabled");
}
if (!MiscUtil.classAvailable(moduleImplClassName)) {
throw new ModuleLoaderException(format(ModuleLoaderException.ERROR_MODULE_MISSING, moduleName, moduleHome));
}
return (T) Class.forName(moduleImplClassName).newInstance();
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException e) {
throw new ModuleLoaderException(format(ModuleLoaderException.ERROR_LOADING_MODULE, moduleName), e);
}
}
// used from junit tests (using reflection, because it's invisible in the core-module)
@SuppressWarnings("unused")
public static void _forceDisableBatchModule() {
FORCED_DISABLED_MODULES.add(BatchModule.class);
}
// used from junit tests (using reflection, because it's invisible in the core-module)
@SuppressWarnings("unused")
public static void _forceRecheckModule() {
FORCED_RECHECK_MODULES.add(BatchModule.class);
FORCED_RECHECK_MODULES.add(SMIMEModule.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy