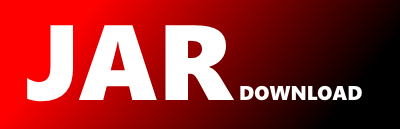
org.sitoolkit.tester.DebugSupport Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Monocrea Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sitoolkit.tester;
import java.util.Scanner;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
/**
*
* @author yuichi.kuwahara
*/
@Component
public class DebugSupport {
private static Logger LOG = LoggerFactory.getLogger(DebugSupport.class);
private static final String CMD_PAUSE = "s";
private static final String CMD_STEP_FORWARD = "n";
private static final String CMD_CURRENT = "c";
private static final String CMD_STEP_BACK = "p";
private static final String CMD_EXEC_SCRIPT = "!";
private static final String CMD_UPD_NUMBER = "#";
@Autowired
TestContext current;
/**
* 一時停止する間隔
*/
private int pauseSpan = 800;
String input;
String scriptNo;
ExecutorService executor;
private boolean paused = false;
public boolean next() {
int currentIndex = current.getCurrentIndex();
TestScriptCatalog catalog = current.getCatalog();
if (currentIndex < catalog.getTestScriptCount()) {
if (isDebug()) {
if (!StringUtils.isEmpty(catalog.getTestScript(currentIndex).getBreakPoint())) {
setPaused(true);
}
currentIndex = getNextIndex(currentIndex);
}
TestScript ts = catalog.getTestScript(currentIndex);
current.setTestScript(ts);
current.setCurrentIndex(++currentIndex);
return true;
} else {
return false;
}
}
public boolean isDebug() {
return "true".equalsIgnoreCase(System.getProperty("tester.debug", "false"));
}
protected int getNextIndex(int i) {
if (isPaused()) {
LOG.info("テストスクリプトの実行を一時停止中です");
}
while (isPaused()) {
try {
Thread.sleep(getPauseSpan());
} catch (InterruptedException e) {
LOG.warn("スレッドの待機に失敗しました", e);
}
if (current.getCatalog().isScriptFileChanged()) {
LOG.info("テストスクリプトが変更されています。再読込します。");
current.getCatalog().reload();
}
if (input == null) {
continue;
}
boolean shouldBreak = false;
switch (input.toLowerCase()) {
case CMD_STEP_BACK:
if (i > 0) {
LOG.info("スクリプト番号を1つ戻します");
i--;
}
break;
case CMD_CURRENT:
LOG.info("現在のテストスクリプトを実行します");
i--;
shouldBreak = true;
break;
case CMD_STEP_FORWARD:
LOG.info("次のテストスクリプトを実行します");
shouldBreak = true;
break;
case CMD_EXEC_SCRIPT:
LOG.info("No.{}のテストスクリプトを実行します", scriptNo);
i = current.getCatalog().getIndexByScriptNo(scriptNo);
shouldBreak = true;
break;
case CMD_UPD_NUMBER:
LOG.info("テストスクリプトの実行をNo.{}から開始するように設定します", scriptNo);
i = current.getCatalog().getIndexByScriptNo(scriptNo);
break;
case CMD_PAUSE:
break;
default:
usage();
}
if (shouldBreak) break;
input = null;
}
input = null;
return i;
}
public void pause() {
setPaused(true);
}
@PostConstruct
public void init() {
if (isDebug()) {
LOG.info("デバッグモードでテストを実行します。"
+ "実行を一時停止するには\"s\"を入力しEnterキーをタイプしてください。");
executor = Executors.newSingleThreadExecutor();
executor.submit(new Runnable() {
@Override
public void run() {
Scanner scan = new Scanner(System.in);
try {
while ((input = scan.nextLine()) != null) {
if (CMD_PAUSE.equalsIgnoreCase(input)) {
changeStatus();
}
if (StringUtils.startsWith(input, CMD_UPD_NUMBER)) {
scriptNo = StringUtils.substringAfter(input, CMD_UPD_NUMBER);
input = CMD_UPD_NUMBER;
}
if (StringUtils.startsWith(input, CMD_EXEC_SCRIPT)) {
scriptNo = StringUtils.substringAfter(input, CMD_EXEC_SCRIPT);
input = CMD_EXEC_SCRIPT;
}
}
} finally {
scan.close();
}
}
});
}
}
@PreDestroy
public void destroy(){
if (isDebug()) {
executor.shutdownNow();
}
}
private void changeStatus(){
paused = !paused;
if (paused) {
LOG.info("テスト実行を一時停止します");
} else {
LOG.info("テスト実行を再開します");
}
}
public int getPauseSpan() {
return pauseSpan;
}
public void setPauseSpan(int pauseSpan) {
this.pauseSpan = pauseSpan;
}
public boolean isPaused(){
return paused;
}
public void setPaused(boolean paused){
this.paused = paused;
}
private void usage(){
LOG.info("デバッグ操作方法:\n"
+ "s : テストスクリプト実行を一時停止/再開します\n"
+ "c : 現在のテストスクリプトを実行します\n"
+ "n : 次のテストスクリプトを実行します\n"
+ "p : スクリプト番号を1つ戻します\n"
+ "!{0} : No.{0}のテストスクリプトを実行します\n"
+ "#{0} : テストスクリプトの実行をNo.{0}から開始するように設定します\n");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy