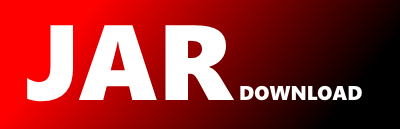
org.sitoolkit.tester.PageSpecConverter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Monocrea Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sitoolkit.tester;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.filefilter.DirectoryFileFilter;
import org.apache.commons.io.filefilter.PrefixFileFilter;
import org.apache.commons.lang.StringUtils;
import org.sitoolkit.core.infra.repository.DocumentMapper;
import org.sitoolkit.core.infra.repository.DocumentRepository;
import org.sitoolkit.core.infra.repository.RowData;
import org.sitoolkit.core.infra.repository.TableDataCatalog;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Scope;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.springframework.stereotype.Component;
/**
*
* @author kaori.ogawa
*/
@Component
@Scope("prototype")
public class PageSpecConverter {
protected final Logger log = LoggerFactory.getLogger(getClass());
@Autowired
ApplicationContext appCtx;
@Autowired
OperationConverter operationConverter;
@Autowired
DocumentRepository repo;
@Autowired
TestScriptConvertUtils testScriptConvertUtils;
@Resource(name = "pageSpecConverterMap")
Map pageSpecConverterMap;
private static final String SYSPROP_TEST_SCRIPT_DIR = "testScriptDir";
private static final String TEMPLATE_PATH = "src/main/resources/TestScriptTemplate.xlsx";
private static final String CASE_NO = "001";
private static final String ITEM_DEF_SHEET_NAME = "項目定義";
public static void main(String[] args) {
ApplicationContext appCtx = new ClassPathXmlApplicationContext("classpath:sit-tester-conf.xml");
PageSpecConverter converter = appCtx.getBean(PageSpecConverter.class);
System.exit(converter.execute());
}
public int execute() {
File scriptDir = new File(
System.getProperty(SYSPROP_TEST_SCRIPT_DIR, "src/test/resources/pagespec"));
if (!scriptDir.exists()) {
scriptDir = new File(".");
}
for (File scriptFile : FileUtils.listFiles(
scriptDir,
new PrefixFileFilter("画面定義書_"),
DirectoryFileFilter.DIRECTORY)) {
convert(scriptFile);
}
return 0;
}
/**
* 画面定義書をテストスクリプトに変換します。
*
* @param pageSpecPath 画面定義書のパス
* @return 保存したテストスクリプトファイル
*/
public File convert(File pageSpec) {
String pageSpecPath = pageSpec.getAbsolutePath();
String inputTestScript = StringUtils.substringBefore(StringUtils.substringAfter(pageSpecPath, "_"), ".")
+ "TestScript.xlsx";
List pageItemSpeclist = loadPageSpec(pageSpecPath, ITEM_DEF_SHEET_NAME);
List testScriptList = new ArrayList();
int no = 1;
for (PageItemSpec pageItemSpec : pageItemSpeclist) {
testScriptList.add(convertPageItemSpec(no++, pageItemSpec));
}
TableDataCatalog catalog = testScriptConvertUtils.getTableDataCatalog(testScriptList);
String scriptDir = System.getProperty(SYSPROP_TEST_SCRIPT_DIR, "src/test/resources/testscript");
File testScriptFile = new File(scriptDir, inputTestScript);
repo.write(TEMPLATE_PATH, testScriptFile.getPath(), catalog);
return testScriptFile;
}
/**
* 画面定義書ファイル(xlsx)を読込みむ
* @param pageSpecPath
* @param sheetName
* @return
*/
public List loadPageSpec(String pageSpecPath, String sheetName) {
List pageItemSpecList = new ArrayList();
DocumentMapper dm = appCtx.getBean(DocumentMapper.class);
for (RowData row : repo.read(pageSpecPath, sheetName).getRows()) {
PageItemSpec pageItemSpec = dm.map("pageItemSpec", row, PageItemSpec.class);
pageItemSpecList.add(pageItemSpec);
}
return pageItemSpecList;
}
/**
* PageItemSpecオブジェクトをTestScriptオブジェクトに変換します。
* @param list
* @return
*/
private TestScript convertPageItemSpec(int no, PageItemSpec pageItemSpec) {
TestScript testScript = appCtx.getBean(TestScript.class);
testScript.setNo(Integer.toString(no));
testScript.setCurrentCaseNo(CASE_NO);
// 項目名
testScript.setItemName(pageItemSpec.getName());
// ロケーター
Locator locator = buildLocator(pageItemSpec.getName(), pageItemSpec.getPname());
testScript.setLocator(locator);
// 操作
String operationName = pageSpecConverterMap.get(pageItemSpec.getControl());
testScript.setOperationName(operationName);
if ("click".equals(operationName)) {
testScript.setScreenshotTiming("前");
}
// ケースデータ
Map testData = new HashMap();
testScript.setTestData(testData);
// TODO セレクトボックスに対する操作でケースデータ"y"はテスト実行時にエラーとなる。
// これを避けるためにselect操作はスキップさせる。
String caseData = "select".equals(operationName) ? "" : "y";
testData.put(CASE_NO, caseData);
return testScript;
}
private Locator buildLocator(String name, String pName) {
if (pName == null) {
return Locator.build("id", name);
} else {
return Locator.build("id", pName);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy