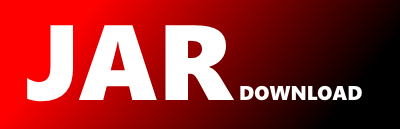
org.sitoolkit.wt.gui.infra.MavenUtils Maven / Gradle / Ivy
package org.sitoolkit.wt.gui.infra;
import java.io.File;
import java.util.logging.Level;
import java.util.logging.Logger;
public class MavenUtils {
private static final Logger LOG = Logger.getLogger(MavenUtils.class.getName());
private static String mvnCommand = "";
public static String getCommand() {
while (mvnCommand == null || mvnCommand.isEmpty()) {
LOG.info("wait for installing Maven...");
try {
Thread.sleep(3000L);
} catch (InterruptedException e) {
LOG.log(Level.WARNING, "", e);
}
}
return mvnCommand;
}
public static void findAndInstall() {
mvnCommand = find();
if (mvnCommand == null || mvnCommand.isEmpty()) {
mvnCommand = install();
}
LOG.info("mvn command is '" + mvnCommand + "'");
}
public static String find() {
LOG.info("finding installed maven");
if (SystemUtils.isOsX()) {
File mvnFile = new File("/usr/local/bin/mvn");
if (mvnFile.exists()) {
return mvnFile.getAbsolutePath();
}
}
String mavenHome = System.getenv("MAVEN_HOME");
String mvn = mavenHome + "/bin/mvn";
if (SystemUtils.isOsX()) {
if (new File(mvn).exists()) {
return mvn;
}
}
if (SystemUtils.isWindows()) {
File mvnFile = new File(mvn + ".cmd");
if (mvnFile.exists()) {
return mvnFile.getAbsolutePath();
} else {
mvnFile = new File(mvn + ".bat");
if (mvnFile.exists()) {
return mvnFile.getAbsolutePath();
}
}
}
return "";
}
public static String install() {
File sitRepo = SystemUtils.isWindows() ? new File(System.getenv("ProgramData"), "sitoolkit/repository")
: new File(System.getProperty("user.home"), ".sitoolkit/repository");
// TODO 外部化
String url = "https://archive.apache.org/dist/maven/maven-3/3.3.9/binaries/apache-maven-3.3.9-bin.zip";
String fileName = url.substring(url.lastIndexOf("/"));
File destFile = new File(sitRepo, "maven/download/" + fileName);
if (destFile.exists()) {
LOG.info("Maven binary is already downloaded : " + destFile.getAbsolutePath());
} else {
FileIOUtils.download(url, destFile);
}
File destDir = new File(sitRepo, "maven/runtime");
FileIOUtils.unarchive(destFile, destDir);
File[] children = destDir.listFiles();
if(children.length != 0){
String mvn = children[0].getAbsolutePath() + "/bin/mvn";
if (SystemUtils.isOsX()) {
if (new File(mvn).exists()) {
return mvn;
}
}
if (SystemUtils.isWindows()) {
File mvnFile = new File(mvn + ".cmd");
if (mvnFile.exists()) {
return mvnFile.getAbsolutePath();
} else {
mvnFile = new File(mvn + ".bat");
if (mvnFile.exists()) {
return mvnFile.getAbsolutePath();
}
}
}
}
return "";
// File[] children = destDir.listFiles();
// return children.length == 0 ? "" : children[0].getAbsolutePath();
}
public static void main(String[] args) {
System.out.println(install());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy