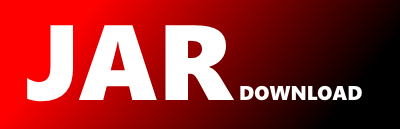
org.sitoolkit.wt.domain.operation.selenium.DbVerifyOperation Maven / Gradle / Ivy
/*
* Copyright 2013 Monocrea Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sitoolkit.wt.domain.operation.selenium;
import java.io.File;
import java.io.IOException;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javax.annotation.Resource;
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import javax.json.JsonValue;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.sitoolkit.wt.domain.operation.DbVerifyLog;
import org.sitoolkit.wt.domain.operation.HtmlTable;
import org.sitoolkit.wt.domain.operation.Value;
import org.sitoolkit.wt.domain.operation.VerifyObj;
import org.sitoolkit.wt.domain.testscript.TestStep;
import org.sitoolkit.wt.infra.TestException;
import org.sitoolkit.wt.infra.VerifyException;
import org.sitoolkit.wt.infra.template.TemplateEngine;
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.namedparam.NamedParameterJdbcTemplate;
import org.springframework.stereotype.Component;
/**
*
* @author yu.takada
*/
@Component("dbverifyOperation")
public class DbVerifyOperation extends SeleniumOperation {
@Resource
NamedParameterJdbcTemplate jdbcTemplate;
@Resource
HtmlTable htmlTable;
@Resource
DbVerifyLog dbVerifyLog;
@Resource
TemplateEngine templateEngine;
private static final String PARAMETER = "param";
private static final String VERIFY = "verify";
@Override
public void execute(TestStep testStep, SeleniumOperationContext ctx) {
JsonReader reader = Json.createReader(new StringReader("{" + testStep.getValue() + "}"));
JsonObject obj = reader.readObject();
JsonObject paramObj = obj.getJsonObject(PARAMETER);
JsonObject verifyObj = obj.getJsonObject(VERIFY);
reader.close();
String verifySql = writeSqlToString(testStep.getLocator().getValue()).toUpperCase();
Map paramMap = newUppercaseKeyMap(paramObj);
Map result = runVerifySql(verifySql, paramMap);
Map verifyMap = newUppercaseKeyMap(verifyObj);
setUpLogInfo(verifyMap, result, verifySql, paramMap);
String startLog = buildLog();
ctx.info(startLog, "");
verify();
String errorLog = buildErrorLog();
String resultTable = buildHtmlTable(result, verifyMap);
if (errorLog.length() == 0) {
replaceLog(ctx, resultTable);
} else {
throw new VerifyException(StringUtils.join(new String[] { errorLog, resultTable }));
}
}
private void replaceLog(SeleniumOperationContext ctx, String resultTabel) {
int currentIdx = ctx.getRecords().size() - 1;
String currentLog = ctx.getRecords().get(currentIdx).getLog();
String log = StringUtils.join(new String[] { currentLog, resultTabel });
ctx.getRecords().get(currentIdx).setLog(log);
}
private void setUpLogInfo(Map verifyMap, Map result,
String locatorSql, Map paramMap) {
List verifyParams = new ArrayList<>();
for (Entry entry : verifyMap.entrySet()) {
VerifyObj obj = new VerifyObj();
obj.setVerifyCol(entry.getKey());
obj.setExpected(entry.getValue());
obj.setActual(result.get(entry.getKey()));
verifyParams.add(obj);
}
dbVerifyLog.setVerifyColList(verifyParams);
dbVerifyLog.setVerifySql(locatorSql);
dbVerifyLog.setVerifyParams(paramMap);
}
private void verify() {
List invalidList = new ArrayList<>();
List errorList = new ArrayList<>();
List mismatchedList = new ArrayList<>();
for (VerifyObj obj : dbVerifyLog.getVerifyColList()) {
if (obj.getActual() == null) {
invalidList.add(obj.getVerifyCol());
} else if (!(obj.getActual().equals(obj.getExpected()))) {
errorList.add(obj);
mismatchedList.add(obj.getVerifyCol());
}
}
dbVerifyLog.setInvalidCols(invalidList);
dbVerifyLog.setVerifyErrs(errorList);
dbVerifyLog.setMismatchedCols(mismatchedList);
}
private String buildLog() {
dbVerifyLog.setTemplate("/evidence/evidence-template-dbverify-expected-list.vm");
dbVerifyLog.setVar("expected");
return templateEngine.writeToString(dbVerifyLog);
}
private String buildErrorLog() {
dbVerifyLog.setTemplate("/evidence/evidence-template-dbverify-error-list.vm");
dbVerifyLog.setVar("error");
return templateEngine.writeToString(dbVerifyLog);
}
private String writeSqlToString(String sqlPath) {
try {
File sqlFile = new File(sqlPath).getAbsoluteFile();
log.info("SQLファイルを読み込みます。[{}]", sqlFile);
return FileUtils.readFileToString(sqlFile, "UTF-8");
} catch (IOException e) {
throw new TestException(e);
}
}
private Map newUppercaseKeyMap(JsonObject jsonObj) {
Map map = new HashMap<>();
for (Entry entry : jsonObj.entrySet()) {
map.put(entry.getKey().toString().toUpperCase(), jsonObj.getString(entry.getKey()));
}
return map;
}
private Map runVerifySql(String sql, Map paramMap) {
Map tmpResult = new HashMap<>();
try {
log.info("検証SQLを実行します。[{}] [{}]", sql, paramMap);
tmpResult = jdbcTemplate.queryForMap(sql, paramMap);
} catch (DataAccessException e) {
throw new TestException(e);
}
Map result = new LinkedHashMap<>();
for (Entry entry : tmpResult.entrySet()) {
result.put(entry.getKey().toUpperCase(), entry.getValue().toString());
}
return result;
}
private String buildHtmlTable(Map result, Map verifyMap) {
List columns = new ArrayList<>();
List values = new ArrayList<>();
for (Entry entry : result.entrySet()) {
String style = "";
if (verifyMap.containsKey(entry.getKey())) {
if (dbVerifyLog.getMismatchedCols().indexOf(entry.getKey()) > -1) {
style = "mismatched";
} else {
style = "verified";
}
}
columns.add(entry.getKey());
values.add(new Value(entry.getValue().toString(), style));
}
htmlTable.setColumns(columns);
htmlTable.setValues(values);
return templateEngine.writeToString(htmlTable);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy