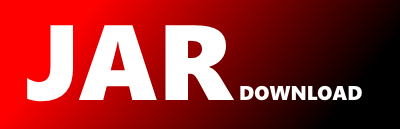
org.sklsft.commons.rest.security.context.SecurityContextHolder Maven / Gradle / Ivy
package org.sklsft.commons.rest.security.context;
import org.sklsft.commons.rest.security.exception.CredentialsConflictException;
import org.sklsft.commons.rest.security.exception.NoBoundCredentialsException;
/**
* A security context is handled by a ThreadLocal
*
* @author Nicolas Thibault
*
*/
public class SecurityContextHolder {
private static ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy