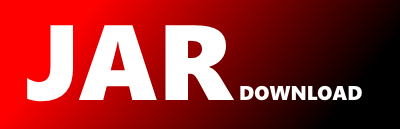
org.slf4j.spi.NOPLoggingEventBuilder Maven / Gradle / Ivy
package org.slf4j.spi;
import java.util.function.Supplier;
import org.slf4j.Logger;
import org.slf4j.Marker;
import org.slf4j.event.Level;
/**
* A no-operation implementation of {@link LoggingEventBuilder}.
*
* As the name indicates, the methods in this class do nothing. In case a return value is expected, a singleton,
* i.e. the unique instance of this class, is returned.
*
* Note that the default implementations of {@link Logger#atTrace()}, {@link Logger#atDebug()} , {@link Logger#atInfo()},
* {@link Logger#atWarn()} and {@link Logger#atError()}, return an instance of {@link NOPLoggingEventBuilder}
* when the relevant level is disabled for current logger. This is the core optimization in the SLF4J fluent API.
*
* @author Ceki Gülcü
* @since 2.0.0
*
*/
public class NOPLoggingEventBuilder implements LoggingEventBuilder {
static final NOPLoggingEventBuilder SINGLETON = new NOPLoggingEventBuilder();
private NOPLoggingEventBuilder() {
}
/**
* Returns the singleton instance of this class.
* Used by {@link org.slf4j.Logger#makeLoggingEventBuilder(Level) makeLoggingEventBuilder(Level)}.
*
* @return the singleton instance of this class
*/
public static LoggingEventBuilder singleton() {
return SINGLETON;
}
/**
* NOP implementation that does nothing.
*
* @param marker a Marker instance to add.
* @return
*/
@Override
public LoggingEventBuilder addMarker(Marker marker) {
return this;
}
/**
* NOP implementation that does nothing.
*
* @param p
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder addArgument(Object p) {
return this;
}
/**
* NOP implementation that does nothing and thus skips calling get() call on the object supplier.
*
* @param objectSupplier
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder addArgument(Supplier> objectSupplier) {
return this;
}
/**
* NOP implementation that does nothing and thus skips calling get() call on the object supplier.
*
* @param objectSupplier
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(Supplier> objectSupplier) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param b a value of type boolean
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
public LoggingEventBuilder arg(boolean b) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param c a value of type char
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(char c) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param b a value of type byte
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(byte b) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param s a value of type short
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
public LoggingEventBuilder arg(short s) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param i a value of type int
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(int i) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param l a value of type long
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(long l) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param f a value of type float
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(float f) {
return this;
}
/**
* NOP implementation that does nothing and thus skips the type cast.
*
* @param d a value of type float
value to add.
* @return a LoggingEventBuilder, usually this.
* @since 2.1.0
*/
@Override
public LoggingEventBuilder arg(double d) {
return this;
}
/**
* NOP implementation that does nothing.
*
* @param key the key of the key value pair.
* @param value the value of the key value pair.
* @return a LoggingEventBuilder, usually this.
*/
@Override
public LoggingEventBuilder addKeyValue(String key, Object value) {
return this;
}
/**
* NOP implementation that doesnothing.
*
* @param key the key of the key value pair.
* @param value the value of the key value pair.
* @return a LoggingEventBuilder, usually this.
*/
@Override
public LoggingEventBuilder kv(String key, Object value) {
return this;
}
/**
* NOP implementation that doesnothing.
*
* @param key the key of the key value pair.
* @param value a supplier of a value for the key value pair.
* @return a LoggingEventBuilder, usually this.
*/
@Override
public LoggingEventBuilder addKeyValue(String key, Supplier