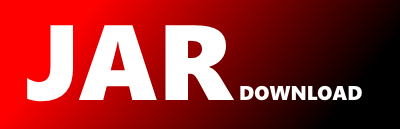
org.smallibs.data.Try Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hpas Show documentation
Show all versions of hpas Show documentation
Functional ADT And Asynchronous library in Java
/*
* HPAS
* https://github.com/d-plaindoux/hpas
*
* Copyright (c) 2016-2017 Didier Plaindoux
* Licensed under the LGPL2 license.
*/
package org.smallibs.data;
import org.smallibs.control.Filter;
import org.smallibs.exception.FilterException;
import org.smallibs.type.HK;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
public interface Try extends Filter>, HK> {
static Try success(T value) {
if (Throwable.class.isInstance(value)) {
return new Failure<>(Throwable.class.cast(value));
}
return new Success<>(value);
}
static Try failure(Throwable value) {
return new Failure<>(value);
}
@Override
default Try filter(Predicate super T> predicate) {
return this.flatmap(t -> predicate.test(t) ? this : Try.failure(new FilterException()));
}
@Override
default R accept(Function>, R> f) {
return f.apply(this);
}
@Override
default Try self() {
return this;
}
default Try map(Function super T, B> mapper) {
return this.flatmap(t -> success(mapper.apply(t)));
}
default B fold(Function super T, B> success, Function super Throwable, B> failure) {
return this.map(success).recoverWith(failure);
}
default T recoverWith(T t) {
return this.recoverWith(x -> t);
}
default T orElseThrow(Supplier extends X> exceptionSupplier) throws X {
return this.orElseThrow(x -> exceptionSupplier.get());
}
default T orElseThrow() throws Throwable {
return this.orElseThrow(x -> x);
}
default boolean isSuccess() {
return this.fold(t -> true, f -> false);
}
T recoverWith(Function super Throwable, T> t);
T orElseThrow(Function super Throwable, ? extends X> exceptionSupplier) throws X;
Try flatmap(Function super T, Try> mapper);
Try onSuccess(Consumer super T> onSuccess);
Try onFailure(Consumer super Throwable> onFailure);
/**
* Success implementation
*/
final class Success implements Try {
private final T value;
private Success(T value) {
this.value = value;
}
@Override
public Try flatmap(Function super T, Try> mapper) {
return mapper.apply(this.value);
}
@Override
public Try onSuccess(Consumer super T> onSuccess) {
onSuccess.accept(this.value);
return this;
}
@Override
public Try onFailure(Consumer super Throwable> onFailure) {
return this;
}
@Override
public T orElseThrow(Function super Throwable, ? extends X> exceptionSupplier) throws X {
return this.value;
}
@Override
public T recoverWith(Function super Throwable, T> t) {
return this.value;
}
}
/**
* Failure implementation
*/
final class Failure implements Try {
private final Throwable value;
private Failure(Throwable value) {
this.value = value;
}
@Override
public Try flatmap(Function super T, Try> mapper) {
return Try.failure(this.value);
}
@Override
public Try onSuccess(Consumer super T> onSuccess) {
return this;
}
@Override
public Try onFailure(Consumer super Throwable> onFailure) {
onFailure.accept(this.value);
return this;
}
@Override
public T orElseThrow(Function super Throwable, ? extends X> exceptionSupplier) throws X {
throw exceptionSupplier.apply(this.value);
}
@Override
public T recoverWith(Function super Throwable, T> t) {
return t.apply(this.value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy