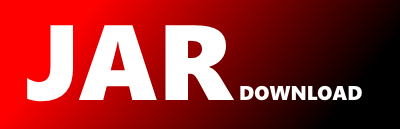
org.smallibs.concurrent.promise.Promise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hpas Show documentation
Show all versions of hpas Show documentation
Functional ADT And Asynchronous library in Java
/*
* HPAS
* https://github.com/d-plaindoux/hpas
*
* Copyright (c) 2016-2017 Didier Plaindoux
* Licensed under the LGPL2 license.
*/
package org.smallibs.concurrent.promise;
import org.smallibs.control.Filter;
import org.smallibs.data.Try;
import org.smallibs.type.HK;
import org.smallibs.util.FunctionWithError;
import java.util.concurrent.Future;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* A promise is a component denoting an asynchronous computation. Such component can be mapped in order to chain
* transformations.
*/
public interface Promise extends Filter>, HK> {
/**
* Provides the underlying future able to capture and returns the result or the error for a given execution
*
* @return a future
*/
Future getFuture();
/**
* Callback called when the computation succeed
*
* @param consumer The callback to be activated on success
* @return the current promise
*/
Promise onSuccess(Consumer consumer);
/**
* Callback called when the computation fails
*
* @param consumer The callback to be activated on error
* @return the current promise
*/
Promise onFailure(Consumer consumer);
/**
* Callback called when the computation terminates
*
* @param consumer The callback to be activated on completion
* @return the current promise
*/
Promise onComplete(Consumer> consumer);
/**
* Method use to map a function. This mapping is done when the operation is a success. The result of this mapping
* is a new promise component.
*
* @param the promised value type
* @param function The function to applied on success which can raise an error
* @return a new promise
*/
Promise map(FunctionWithError function);
/**
* Method use when a new computation must be done when the current one succeed. The current one and the chained one
* are done sequentially in the same context.
*
* @param the promised value type
* @param function The function to applied on success
* @return a new promise
*/
default Promise and(FunctionWithError function) {
return this.map(function);
}
/**
* Method use to flatmap a function. This mapping is done when the operation is a success. The result of this mapping
* is a new promise component.
*
* @param the promised value type
* @param function The function to applied on success
* @return a new promise
*/
Promise flatmap(Function> function);
/**
* Method use when a new asynchronous computation must be done when the current one succeed. The current one and the
* chained one are not done sequentially in the same context.
*
* @param the promised value type
* @param function The function to applied on success
* @return a new promise
*/
default Promise then(Function> function) {
return this.flatmap(function);
}
}