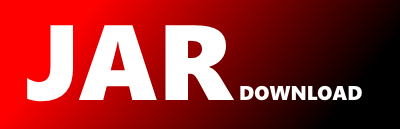
org.smallibs.concurrent.promise.impl.SolvablePromise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hpas Show documentation
Show all versions of hpas Show documentation
Functional ADT And Asynchronous library in Java
/*
* HPAS
* https://github.com/d-plaindoux/hpas
*
* Copyright (c) 2016 Didier Plaindoux
* Licensed under the LGPL2 license.
*/
package org.smallibs.concurrent.promise.impl;
import org.smallibs.concurrent.promise.Promise;
import org.smallibs.data.Try;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.function.Consumer;
public class SolvablePromise extends AbstractPromise {
private final SolvableFuture future;
private final List> onSuccess;
private final List> onError;
public SolvablePromise() {
this.future = createFuture(this::notifyResponse);
this.onSuccess = new ArrayList<>();
this.onError = new ArrayList<>();
}
@Override
public Future getFuture() {
return future;
}
@Override
public Promise onSuccess(Consumer consumer) {
Objects.requireNonNull(consumer);
final T value;
synchronized (this.future) {
if (future.isDone() || future.isCancelled()) {
try {
value = future.get();
} catch (InterruptedException | ExecutionException e) {
return this;
}
} else {
this.onSuccess.add(consumer);
return this;
}
}
consumer.accept(value);
return this;
}
@Override
public Promise onFailure(Consumer consumer) {
Objects.requireNonNull(consumer);
Throwable value = null;
synchronized (this.future) {
if (future.isDone() || future.isCancelled()) {
try {
this.future.get();
return this;
} catch (InterruptedException e) {
value = e;
} catch (ExecutionException e) {
value = e.getCause();
}
} else {
this.onError.add(consumer);
return this;
}
}
consumer.accept(value);
return this;
}
@Override
public Promise onComplete(final Consumer> consumer) {
Objects.requireNonNull(consumer);
return this.onSuccess(t -> consumer.accept(Try.success(t)))
.onFailure(throwable -> consumer.accept(Try.failure(throwable)));
}
public void solve(final Try response) {
synchronized (this.future) {
this.future.solve(response);
}
this.notifyResponse(response);
}
//
// Protected behaviors
//
protected SolvableFuture createFuture(Consumer> callbackOnComplete) {
return new SolvableFuture<>(callbackOnComplete);
}
//
// Private behaviors
//
private void notifyResponse(Try response) {
response.onSuccess(s -> {
onSuccess.forEach(c -> c.accept(s));
onSuccess.clear();
}).onFailure(t -> {
onError.forEach(c -> c.accept(t));
onError.clear();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy